In the previous section, you created a simple page with links to a page displaying category details. In this section, you'll see how to provide an AlternatingItem template, how to add embedded controls, and how to react to events of those controls. Along the way, you'll create the category detail page shown in Figure 18.3. Figure 18.3. Clicking a link on this page retrieves the units in stock for the selected item.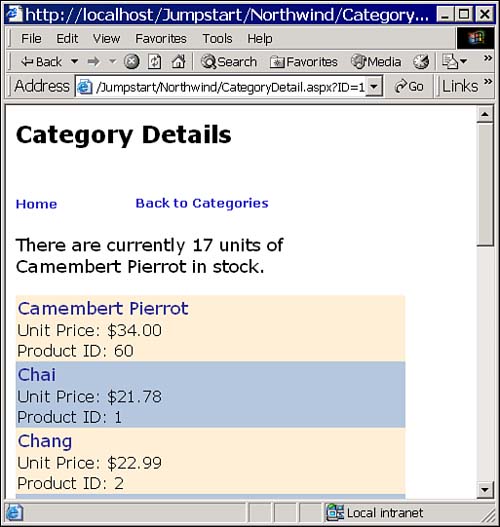 Creating the Detail Page To get started investigating more advanced features, you'll need to create the detail page. Follow these steps to create the base page you'll need: -
Select the Project, Add Web Form menu item and name the new page CategoryDetail.aspx. -
Set the page's pageLayout property to FlowLayout. -
Add controls and set their properties as described in Table 18.6. When you're done, the page should look like Figure 18.4. Figure 18.4. The CategoryDetails.aspx page should look something like this when you're done laying out the controls.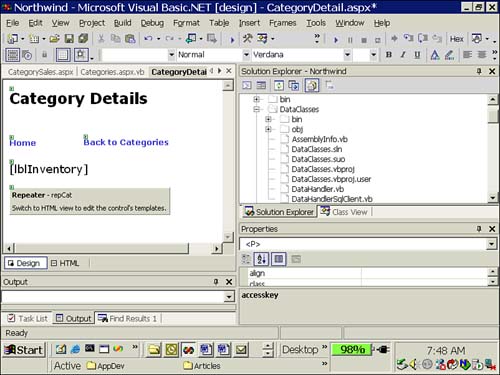 Table 18.6. Add Controls and Set Their Properties Control | Property | Value | Label | Text | Category Details | HyperLink | Text | Home | | NavigateURL | Main.aspx | HyperLink | Text | Back to Categories | | NavigateURL | Categories.aspx | Label | ID | lblInventory | | Text | (Delete the text in this property) | Repeater | ID | repCat | -
Press F7 to load the page's code-behind file. -
Add this procedure to the page's class: Private Sub RepeaterBind() Dim ds As DataSet Dim strSQL As String Dim strConn As String ' Build Connect String strConn = Session("ConnectString").ToString ' Build SQL and Connection strings strSQL = String.Format( _ "SELECT ProductID, ProductName, " & _ "UnitPrice, UnitsInStock " & _ "FROM Products " & _ "WHERE CategoryID = {0} " & _ "ORDER BY ProductName", _ Request.QueryString("ID")) ds = DataHandler.GetDataSet(strSQL, strConn) ' Set DataSource and display data repCat.DataSource = ds repCat.DataBind() End Sub In this case, the RepeaterBind procedure retrieves the connection string and then builds a SQL string indicating the rows to retrieve from SQL Server. Because this page receives an ID value in the query string sent from the previous page, the code uses Request.QueryString to retrieve the ID value and inserts it into the SQL string. The code then calls the DataHandler.GetDataSet method to create the data set based on the SQL string. The Repeater control's DataSource property is then bound to that data set. Finally, the code calls the DataBind method in order to fill the Repeater. -
Modify the Page_Load procedure so that it calls the RepeaterBind procedure you've just added: Private Sub Page_Load( _ ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles MyBase.Load If Not Page.IsPostBack Then RepeaterBind() End If End Sub -
Press Shift+F7 to load the page's designer and then click the HTML tab at the bottom of the window to switch to HTML view. -
Find the </asp:Repeater> tag that ends the Repeater control's definition and insert the following HeaderItem and FooterItem elements before the Repeater control's ending tag: <HEADERTEMPLATE> <TABLE border="0"> </HEADERTEMPLATE> <FOOTERTEMPLATE> </TABLE> </FOOTERTEMPLATE> NOTE The header and footer templates you've just added constitute the beginning and ending tags for a table. All that's left to add is the contents for each row. Creating the ItemTemplate Element  | To create the item template, follow these steps: | -
Between the HeaderItem and FooterItem elements you've just added, insert the following ItemTemplate element: NOTE Rather than typing the following large block of HTML, load Jumpstart\Repeater\RepeaterDtlHTML.txt into any text editor. Copy and paste the contents of the file into the HTML designer between the HeaderItem and FooterItem elements. <ITEMTEMPLATE> <TABLE style="WIDTH: 400px; HEIGHT: 63px" cellSpacing="1" cellPadding="1" width="600" bgColor="papayawhip" border="0"> <TR> <TD colSpan="3"> <FONT face="Verdana" size="4"> <asp:LinkButton Runat="server" Text='<%# Databinder.Eval( Container.DataItem, "ProductName") %>' CommandName='<%# Databinder.Eval( Container.DataItem, "ProductName") %>'> CommandArgument='<%#Databinder.Eval( Container.DataItem,"UnitsInStock")%>' </asp:LinkButton> </FONT> </TD> </TR> <TR> <TD> <FONT face="Verdana">Unit Price: <%# FormatCurrency(Databinder.Eval( Container.DataItem, "UnitPrice")) %> </FONT> </TD> </TR> <TR> <TD> <FONT face="Verdana">Product ID: <%# Databinder.Eval( Container.DataItem, "ProductID") %> </FONT> </TD> </TR> </TABLE> </ITEMTEMPLATE> -
Press F5 to run the project. Verify that you can select the categories on the first page and see the products selected on the second page. -
Close the browser and save your project. What's going on here? If you look carefully, you'll see that the ItemTemplate element consists of a table with three rows. The first row contains a LinkButton control, with three bound attributes: Text='<%# Databinder.Eval( Container.DataItem, "ProductName") %>' CommandName='<%# Databinder.Eval( Container.DataItem, "ProductName") %>'> CommandArgument='<%#Databinder.Eval( Container.DataItem,"UnitsInStock")%>' The Text attribute describes what appears in the display of the control. (In this case, the code retrieves the ProductName field from the Repeater's data source.) The CommandName and CommandArgument attributes contain the ProductName and UnitsInStock fields, respectively. These attributes won't come into play until the next section, but for now, just realize that when you select a LinkButton control within the Repeater, you trigger the Repeater control's ItemCommand event. The LinkButton control allows you to specify any text you like in its CommandName and CommandArgument attributes, and you can retrieve these values from the procedure that handles the ItemCommand event. The other two rows within the table display the UnitPrice and ProductID fields from the data source, using the DataBinder.Eval method to retrieve the value at runtime. The AlternatingItem Template So far, you've created a table containing items within a category, but you might like to have alternating items displayed in different colors. To do that, you must add an AlternatingItemTemplate element to the page. Follow these steps to add the AlternatingItem template: -
With the CategoryDetail.aspx designer open in HTML view, select the entire ItemTemplate element, including its tags. Press Ctrl+C to copy the element to the Clipboard. -
Move the insertion point immediately following the closing </ItemTemplate> tag and press Ctrl+V to paste the copied element into the document. -
Modify the beginning and ending tags of the new element, changing the name from ItemTemplate to AlternatingItemTemplate. -
Change the bgColor attribute of the row's style attribute to a different color. You can try Thistle or any other color of your choice. TIP For help selecting colors, you can use tools provided by Visual Studio. Delete the bgColor attribute and its value and type bgColor =. Visual Studio will provide a "Pick Color" tip, and you can then select from the colors in the dialog box shown in Figure 18.5. Figure 18.5. Choose colors using this helpful dialog box.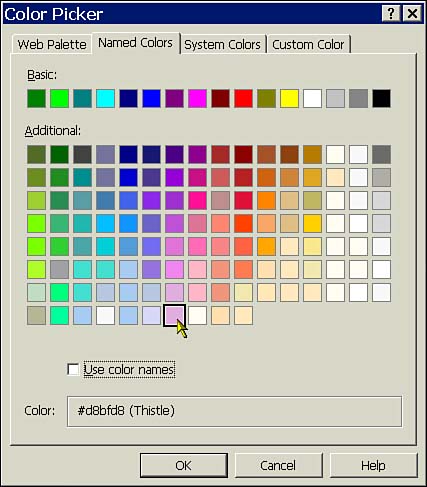 NOTE Although you've only changed the background color for the alternating items in this example, you could change other attributes as well. -
Run the project, as before, and verify that you now see alternating colors on the CategoryDetail.aspx page. -
When you're done, close the browser and save your project. Adding the ItemCommand Event Procedure It would be great if you could click an item and have its current availability (units in stock) displayed. Although the Repeater control raises several events as ASP.NET renders and destroys the page containing the control, the only event you're likely to react to is the control's ItemCommand event. The control raises this event when you click a button (or link) within the control. In this demonstration, you've created a hyperlink within the Repeater control. To finish the project, you need to add code to display the inventory amount for the selected item. Besides the standard source As System.Object parameter, the procedure for handling the ItemCommand event receives a parameter defined As RepeaterCommandEventArgs. This object provides four properties, as described in Table 18.7. Table 18.7. The RepeaterCommandEventArgs Object Provides These Useful Properties Property | Description | CommandArgument | Retrieves the value placed into the CommandArgument attribute in the Repeater control | CommandName | Retrieves the value placed into the CommandName attribute in the Repeater control | CommandSource | Retrieves the source of the command | Item | Retrieves a reference to the RepeaterItem associated with the event | Although you might normally set the CommandName attribute in the Repeater control to represent the name of a command (such as "Sort") and the CommandArgument to contain a subcommand (such as "Descending"), in this example the CommandName attribute contains the ProductName field, and the CommandArgument attribute contains the UnitsInStock field. Because you filled in these attributes (see the previous listing) in the Repeater control, you can retrieve them from within the code-behind file in the ItemCommand event handler. Follow these steps to add event handling to your sample page: -
Make sure that CategoryDetail.aspx is open in the designer window. -
Press F7 to view the code-behind file for the page. -
In the Class Name combo box, at the left top of the window, select repCat. -
In the Method Name combo box, at the right top of the window (see Figure 18.6), select ItemCommand. (At this point, the editor inserts the stub of the event handler.) Figure 18.6. Select the repCat control from the list of objects that raise events.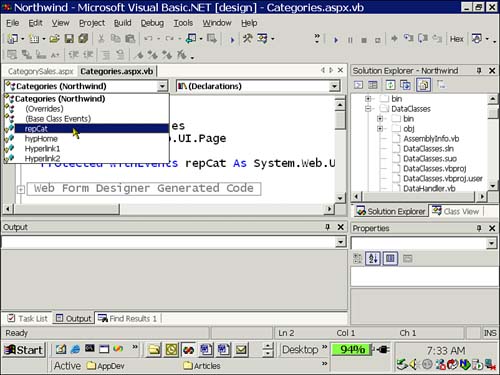 -
Modify the repCat_ItemCommand procedure so that it looks like this: Private Sub repCat_ItemCommand( _ ByVal source As System.Object, _ ByVal e As _ System.Web.UI.WebControls.RepeaterCommandEventArgs) _ Handles repCat.ItemCommand lblInventory.Text = _ String.Format("There are currently {0} units " & _ "of {1} in stock.", _ e.CommandArgument, e.CommandName) End Sub -
Run the project again, select a category, and then select an item by clicking its link. You should see the available inventory in the label control on the Category Details page. -
Close the browser and save your project. |