Variable scope is a tricky but important concept. Every variable in PHP has a scope to it, which is to say a realm in which the variable (and therefore its value) can be accessed. For starters, variables have the scope of the page in which they reside. So if you define $var, the rest of the page can access $var, but other pages generally cannot (unless you use special variables). Since included files act as if they were part of the original (including) script, variables defined before the include() line are available to the included file (as you've already seen with $page_title and header.html). Further, variables defined within the included file are available to the parent (including) script after the include() line. All of this becomes murkier when using your own defined functions. These functions have their own scope, which means that variables used within a function are not available outside of it, and variables defined outside of a function are not available within it. For this reason, a variable inside of a function can have the same name as one outside of it and still be an entirely different variable with a different value. This is a confusing concept for most beginning programmers. To alter the variable scope within a function, you can use the global statement. function function_name() { global $var; } $var = 20; function_name(); // Function call. In this example, $var inside of the function is now the same as $var outside of it. This means that the function $var already has a value of 20, and if that value changes inside of the function, the external $var's value will also change. Another option for circumventing variable scope is to make use of the superglobals: $_GET, $_POST, $_REQUEST, etc. These variables are automatically accessible within your functions (hence, they are superglobal). To use global variables 1. | Open calculator.php (refer to Script 3.10) in your text editor.
| 2. | Change the function definition to (Script 3.11)
function calculate_total ($tax = 5) { global $total; $taxrate = $tax / 100; $total = ($_POST['quantity'] * $_POST['price]) * ($taxrate + 1); $total = number_format ($total, 2); } Script 3.11. Since $_POST is a superglobal variable, its values can be accessed within functions. The $total variable is made global within the function using the global keyword. 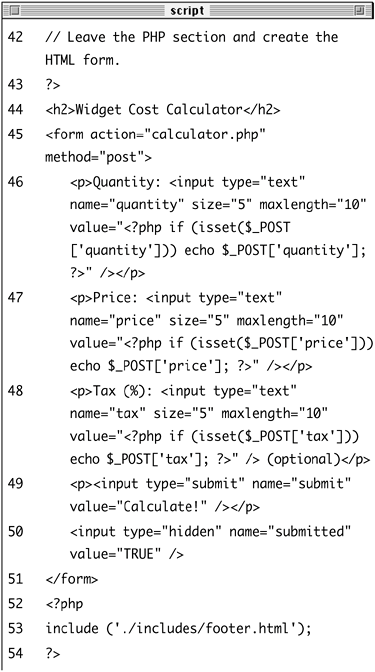
Since I've been using the superglobals anyway, I can rewrite the function so that it automatically uses these variables, ($_POST['quantity'] and $_POST['price']), instead of passing variables to it. Similarly, instead of returning the $total value, I can give it global scope.
| 3. | Initialize the $total variable outside of the function.
$total = NULL; As a matter of good programming form, the $total variable is defined with a NULL value before the function call will make it global.
| 4. | Change the function call lines to
if (is_numeric($_POST['tax'])) { calculate_total ($_POST['tax']); } else { calculate_total (); } Since the function will automatically access the $_POST variables, the function call lines now only need to send the function a tax value if one has been submitted.
| 5. | Change the echo() statement to read
echo '<p>The total cost of purchasing ' . $_POST['quantity] . ' widget(s) at $' . number_format ($_POST['price], 2) . ' each is $' . $total . '.</p>'; Instead of referring to $total_cost (which was previously returned from the function), the global $total variable is printed here.
| 6. | Save the file, upload to your server, and test in your Web browser (Figure 3.18).
Figure 3.18. Again, the same end result (what the user sees) can be created using variations on your function definition. 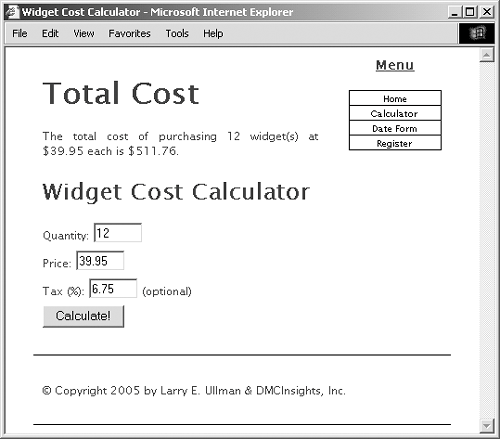
| Tips Another confusing aspect of variable scope and functions comes from where in a script you define a function. A function's code is executed when that function is called, not when it is defined. Thus, you only need to define a global variable prior to calling a function that references it. A static variable is one that belongs to a function but whose value is initialized the first time that function is called and remembered by the function for each subsequent call. The following function will print the number 1 the first time called by the script, the number 2 the second time, and so forth. function counter () { static $var = 1; echo $var++; }
Another method for circumventing scope is to pass a variable by reference rather than by value, allowing any changes made to that variable within the function to be applied to the variable outside of it. See the PHP manual for more information on passing values by reference. To adjust for scope, you can also use the $GLOBALS array, which contains all of the script's global variables. The $_SESSION and $_COOKIE variables can have values that are accessible by multiple pages. You'll learn more about both in Chapter 9, "Cookies and Sessions." Even though you can use the global statement and superglobals to rewrite your functions as I've done here, it won't always make the most sense to do so. The preceding version of the function was actually better because it received three values and returned one, without caring what the form input names (corresponding to the $_POST variables) were.
|