This Web site will make use of two configuration-type scripts. One, config.inc.php, will manage errors and could be used for other purposes, such as defining functions and establishing constants. The other, mysql_connect.php, will store all of the database-related information. Making a configuration file The sole purpose of the configuration file will be to establish the error-management policy for the site. The technique involvedcreating your own error handling functionwas covered in Chapter 6, "Error Handling and Debugging." In this chapter I'll modify the original version in a couple of ways. During the development stages, I'll want every error reported in the most detailed way (Figure 13.3). Along with the specific error message, all of the existing variables will be shown, as will the current date and time. This will be formatted so that it fits within the site's template (in other words, there will be a dedicated error formatting style defined in the CSS file). Figure 13.3. During the development stages of the Web site, I want all errors to be made as obvious and as informative as possible. 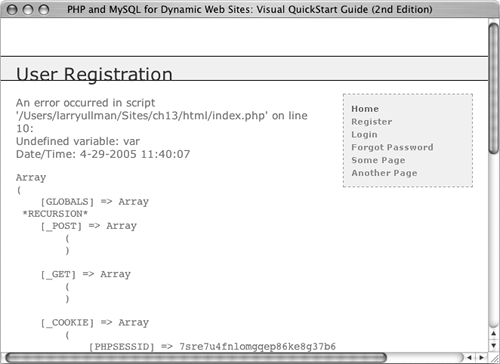 During the production, or live, stage of the site, I'll want to handle errors more gracefully (Figure 13.4). At that time, the detailed error messages will not be printed in the Web browser, but instead sent to an email address. Because certain errors (called notices) may or may not be indicative of a problem (see Chapter 6), such errors will be reported in an email but ignored in the Web browser. Figure 13.4. If errors occur while a site is live, the user will only see a message like this (but a detailed error message will be emailed to the administrator). 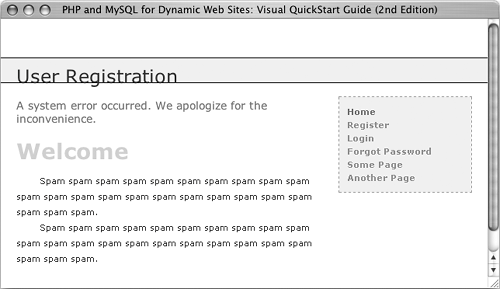 To write config.inc 1. | Create a new document in your text editor (Script 13.3).
<?php # Script 13.3 - config.inc.php Script 13.3. This configuration script dictates how errors are handled. 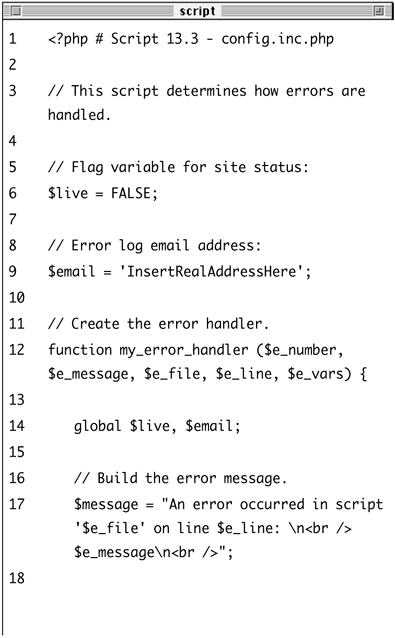 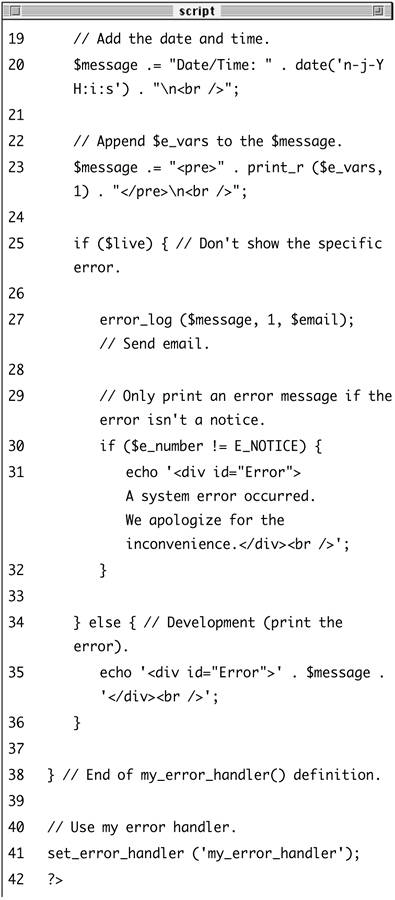
| 2. | Define the required variables.
$live = FALSE; $email = 'InsertRealAddressHere'; The $live variable is the most important one. If it is FALSE, detailed error messages are sent to the Web browser (Figure 13.3). Once the site goes live, this variable should be set to trUE so that detailed error messages are never revealed to the Web user (Figure 13.4). The $email variable is where the error messages will be sent when the site is live. You would obviously use your own e-mail address for this value.
| 3. | Begin defining the error-handling function.
function my_error_handler ($e_number, $e_message, $e_file, $e_line, $e_vars) { global $live, $email; $message = "An error occurred in script '$e_file on line $e_line: \n<br />$e_message\n<br />"; The function definition begins like the one in Chapter 6. It expects to receive five arguments: the error number, the error message, the script in which the error occurred, the line number on which PHP thinks the error occurred, and an array of variables that exist.
Next, I make the two variables defined earlier accessible by invoking the global statement. Then I begin defining my $message variable.
| 4. | Add the current date and time.
$message .= "Date/Time: " . date('n-j-Y H:i:s) . "\n<br />"; To make the error reporting more useful, I'll include the current date and time in the message. A newline character and an HTML <br /> tag are included to make the resulting display more legible.
| 5. | Append all of the existing variables.
$message .= "<pre>" . print_r ($e_vars, 1) . "</pre>\n<br />"; The $e_vars variable is an array of all variables that exist at the time of the error, along with their values. I can use the print_r() function to append the contents of $e_vars onto $message. To make it easier to read this section of the message in the Web browser, I use the HTML <pre> tags.
| 6. | Handle the error according to the value of $live.
if ($live) { error_log ($message, 1, $email); if ($e_number != E_NOTICE) { echo '<div >A system error occurred. We apologize for the inconvenience. </div><br />'; } } else { echo '<div >' . $message . '</div><br />'; } As I mentioned earlier, if the site is live, the detailed message should be sent in an email and the Web user should only see a generic message. To take this one step further, the generic message will not be printed if the error is of a specific type: E_NOTICE. Such errors occur for things like referring to a variable that does not exist, which may or may not be a problem. To avoid potentially inundating the user with error messages, I check if $e_number is not equal to E_NOTICE, which is a constant defined in PHP (see the PHP manual).
If the site isn't live, the entire error message is printed, for any type of error message. In both cases, I surround the message with <div >, which will format the message per the rules defined in my CSS file.
| 7. | Complete the function definition and tell PHP to use your error handler.
} set_error_handler ('my_error_ handler); ?> You have to use the set_error_handler() function to tell PHP to use your own function for errors.
| 8. | Save the file as config.inc.php, and upload to the Web server, placing it in the includes directory.
| Making the database script The second configuration-type script will be mysql_connect.php, a variation on the database connection file used multiple times in the book already. Its primary purpose is to connect to MySQL and select the database. The script will also define the escape_data() function, which is used to process all form data before using it in a SQL query. If a problem occurs, this page will make use of the error-handling tools established in config.inc.php. To do so, I'll use the trigger_error() function. This function lets you tell PHP that an error occurred. Of course PHP will handle that error using the my_error_hanlder() function, as established in the configuration script. Database Permissions As a security matter, this Web application should use its own specific MySQL user and password. Instead of accessing the database as a generic Web user or as the root user (a very, very bad idea), establish a new username and password with access only to the database here (sitename). The MySQL user should have permission to insert, update, and select records but nothing more. By creating a new user with very particular permissions, the potential security risk is minimized should that access information be compromised. |
To write mysql_connect.php 1. | Create a new document in your text editor (Script 13.4).
<?php # Script 13.4 - mysql_connect. php Script 13.4. The database connection script creates a function for escaping data and manages MySQL-related errors. 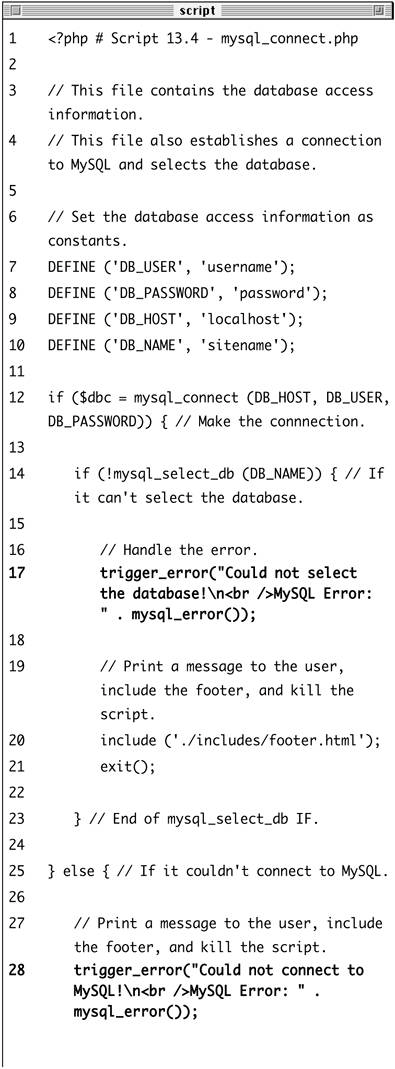 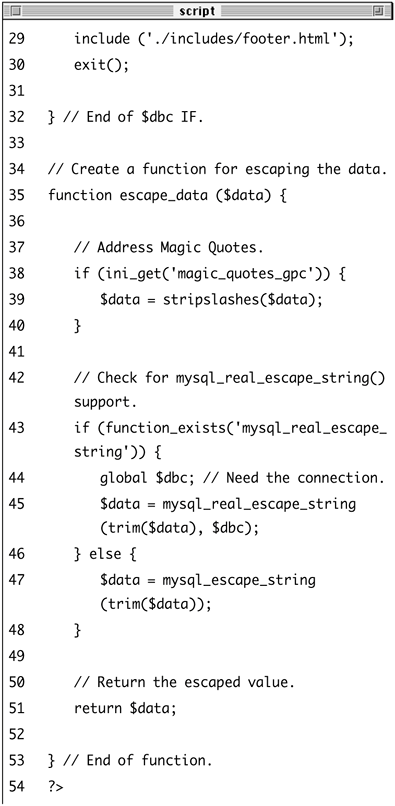
| 2. | Set the database access information.
DEFINE ('DB_USER', 'username'); DEFINE ('DB_PASSWORD', 'password'); DEFINE ('DB_HOST', 'localhost'); DEFINE ('DB_NAME', 'sitename');
| 3. | Attempt to connect to MySQL and select the database.
if ($dbc = mysql_connect (DB_HOST, DB_USER, DB_PASSWORD)) { if (!mysql_select_db (DB_NAME)) { In previous scripts, these two steps each took place in a single line. In those scripts, if the function didn't return the proper result, the die() function was called. Since I will be using my own error-handling function and not simply killing the script, I'll rewrite these steps as conditionals.
| 4. | Handle any errors if the database could not be selected.
trigger_error("Could not select the database!\n<br />MySQL Error: " . mysql_error()); include ('./includes/footer.html'); exit(); } If the script could not select the database, I want to send the error message to the my_error_handler() function. By doing so, I can ensure that the error is handled according to the currently set management technique (live stage versus development). Instead of calling my_error_handler() directly, I use trigger_error(), whose first argument is the error message.
Because an inability to select the database will most likely undermine the functionality of the script, I'll also include the footer file to complete the HTML page and terminate the script. Figure 13.5 shows the end result if a problem occurs during the development stage.
Figure 13.5. A database selection error occurring during the development of the site. 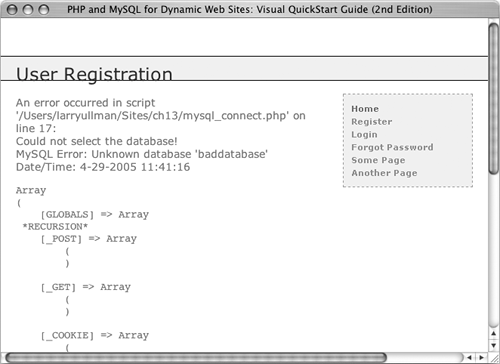
| 5. | Repeat the process if a connection could not be established.
} else { trigger_error("Could not connect to MySQL!\n<br />MySQL Error: " . mysql_error()); include ('./includes/footer. html); exit(); } These lines are permutations of the ones in Step 4 and will be executed if the script could not connect to MySQL. As in that example, the mysql_error() function is used as part of the error message.
| 6. | Create the escape_data() function.
function escape_data ($data) { if (ini_get('magic_quotes_gpc')) { $data = stripslashes($data); } if (function_exists('mysql_real_ escape_string)) { global $dbc; $data = mysql_real_escape_ string (trim($data), $dbc); } else { $data = mysql_escape_string (trim($data)); } return $data; } For an explanation of this syntax, see the original version in Chapter 8, "Web Application Development."
| 7. | Complete the PHP code.
?>
| 8. | Save the file as mysql_connect.php, and upload to the Web server, outside of the Web document directory.
| Tips On the one hand, it might make sense to place the contents of both configuration files in one script for ease of reference. Unfortunately, doing so would add unnecessary overhead (namely, connecting to and selecting the database) to scripts that don't require a database connection (e.g., index.php). For the error management file, I used .inc.php as the extension, indicating that the script is both an included file but also a PHP script. For the MySQL connection page, I just used .php, as it's clear from the file's name what the script does. These are minor, irrelevant distinctions, but I would strongly advocate that both files end with .php, for security purposes.
|