A typical user interface for an application consists of a menu, labels, text boxes, buttons, and perhaps a few controls for specific pieces of data. Without graphics, however, an otherwise functional interface can be quite boring and unintuitive. Graphics can be used to enhance the user interface in the following ways: Highlighting specific information on the screen Providing a different view of information, such as using a graph Providing a more intuitive link to the application's functions The subject of design and use of graphics is large and complex. Obviously, then, this single chapter cannot cover all the bases. However, it will provide you with enough information so that you can begin building a more visually pleasing user interface. Note The Windows API also contains many graphics-related functions. There are two general ways to enhance your forms with graphics: You can add images to controls that accept them, such as PictureBox controls, or even to a form. You can utilize the graphics methods exposed via .NET's Graphics class, which in turn exposes the GDI+ (Graphics Device Interface) of the operating system, providing a means of displaying graphics on screens and printers. Each of these techniques, including combinations of both, will be explored in this section. Note Previous versions of Visual Basic provided Line and Shape controls, which were used to place lines and various shapes (rectangles, ovals, and so on) on a form. Visual Basic .NET no longer supports these controls; using the methods provided by the Graphics class is now the preferred way of drawing shapes on a form. Using Images in Controls and Forms Many of Visual Basic .NET's controls, as well as forms themselves, support the inclusion of an image of some type. As you will see in this section, this functionality is provided by two properties that are common to several objects the Image property and the BackgroundImage property. Each of these properties expects to be provided with some type of image file that contains the desired graphic. Some possible sources for these images are drawings you have created in a graphics software package such as Windows Paint or something more robust, pictures taken by a digital camera and saved to graphics files, or actual photographs converted to graphics files by a scanner. The types of graphics files you can display are listed in Table 14.1. Table 14.1. Graphics File Formats Compatible with Visual Basic .NET File Extension | Type of File |
---|
.BMP | Windows bitmap file | .ICO | Icon | .WMF | Windows metafile | .EMF | Enhanced Windows metafile | .JPG, .JPEG | JPEG images, named after the Joint Photographic Experts Group. Similar to GIF but uses compression to reduce file size; used extensively on Internet Web pages. | .GIF | Graphics Interchange Format. A file format originally developed by CompuServe and used on Internet Web pages. | .PNG | Portable Network Graphics. Designed as the successor to GIF, PNG is gaining acceptance as a good tradeoff between image quality and file size. | Using the Image Property The Image property allows you to specify a graphic to be contained within a control. The Image property is supported by the following controls: PictureBox Button Label LinkLabel CheckBox RadioButton The Image property is most commonly used in PictureBox controls, whose main purpose is to display a picture. The other controls often use images as an alternate means of conveying the purpose of the control; for example, a Button control whose purpose is to initiate a printing procedure may show a picture of a printer in addition to (or instead of) the word Print. Figure 14.13 illustrates the use of the Image property for each of the types of controls listed previously. Figure 14.13. Many controls use the Image property to provide visual enhancements that help identify the control's function. 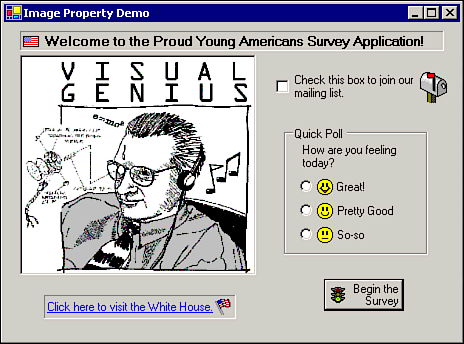 When using the Image property for a control, you can also set the control's ImageAlign property to specify which of the nine available positions within the control (TopLeft, TopCenter, TopRight, MiddleLeft, MiddleCenter, MiddleRight, BottomLeft, BottomCenter, or BottomRight) you want the image to be aligned to. The default alignment is MiddleCenter. The Image property can be set either at design time or runtime, as discussed next. Image Objects The Image property accepts as its value an Image object, which is part of a base class that provides functionality for various types of graphics, such as bitmaps, metafiles, and icons. The .NET framework provides a wealth of image manipulation capabilities that you can use in your Visual Basic programs. Once you have loaded a picture into an Image object, you can resize it or convert to another format with just a few lines of code. You can see an example of this technique in Chapter 17, "Using Active Server Pages.NET." p. 468 Setting the Image Property at Design Time To set the Imageproperty of a control at design time, simply select the control in the Designer window, click the ellipsis ( ... ) next to the Image property in the Properties window, and use the Open dialog box to browse to a file containing a supported graphic. The picture you selected will be displayed in the control both at design time and runtime. Figure 14.14 illustrates a Button control that includes a printer icon to give the user a visual clue as to the button's purpose. Figure 14.14. Adding images to standard controls can help the user understand your program's interface more quickly. 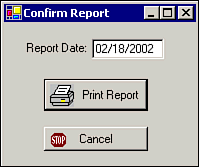 Tip If you are adding an image to a control that also includes text (a Button control, for instance), be sure to manipulate the TextAlign and ImageAlign properties so that the text is not hard to read. Figure 14.15 shows how the text and image on a control can conflict with each other. Figure 14.15. Take care when mixing text and graphics that they do not cause confusion. 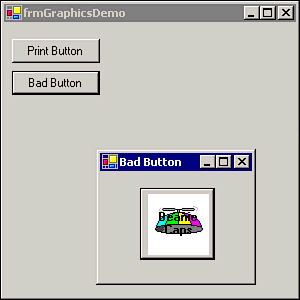 Setting the Image Property at Runtime To set a control's Image property at runtime, you invoke the FromFile method of the Image class, which creates an image from a specified file and loads it into the control. The following is an example of this technique: PictureBox1.Image = Image.FromFile("c:\images\logo4.gif") Clearing the Image Property To remove a loaded picture from a control's Image property at design time, simply right-click the Image property in the Properties window, then click Reset. At runtime, you can use code like the following: PictureBox1.Image = Nothing Considerations of Design-Time Versus Runtime Image Loading There are some things to consider as you decide whether to place images in your controls at design time or at runtime. When you populate the Image property of a control at design time, the image file information is stored as a part of your project (and compiled program); if you load many images in this manner, your program files can get very large. On the other hand, if you load your images dynamically at runtime, your image files are not compiled into your application; in addition, you gain the capability to change images at will. However, if you attempt to load an image file that is corrupt or has been deleted, your program will generate an error that you must deal with. PictureBox Control Image Considerations As the workhorse for adding images to forms, you will likely use the PictureBox control quite often. Because the picture box is a container for an image, you can control the image's position on a form simply by changing the PictureBox control's Location property. You should also be aware of the PictureBox control's SizeMode property. This property determines whether and how the control or the image contained in it are resized based upon the relative sizes of the control and the image. The following table lists the four possible values provided through the PictureBoxSizeMode enumeration and their effect: PictureBoxSizeMode Member | Button Clicked |
---|
Normal | Neither the image nor the control is resized. If the image is larger than the control, it is cropped; if the control is larger than the image, the image is positioned at the upper-left corner of the control. | AutoSize | The control is resized to match the size of the image. | StretchImage | The image is resized to match the size of the control. | CenterImage | If the control is larger than the image, the image is centered inside the control; if the image is larger than the control, the image is placed at the center of the control and the outside edges are cropped. | Figure 14.16 shows the effect the SizeMode property has on the PictureBox control. Figure 14.16. The SizeMode property changes the way images are displayed in PictureBox controls. 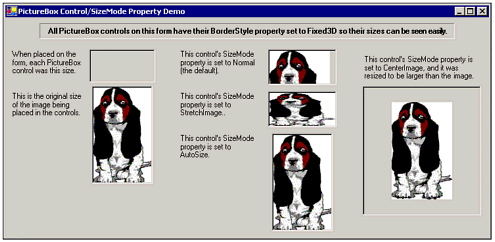 Note Previous versions of Visual Basic allowed you to set the Picture property for a form, which would place a single copy of an image in the upper-left corner of the form, with no tiling or resizing available. If you need to duplicate this functionality, simply place the image in a PictureBox control and position the control appropriately. Using the BackgroundImage Property Several controls, as well as the Form class itself, support a BackgroundImage property. As its name implies, this property specifies an image that is to be used for the background of the object that contains it. Images that are smaller than the object are tiled both vertically and horizontally as necessary to fill it. Typically, background images are used to enhance the visual appeal of a control or form, as opposed to identifying its functionality (as the Image property does). Figure 14.17 shows a form whose background property is used to enhance the application's appearance; the CheckBox controls' BackColor property is set to Transparent so the background image can show through. Figure 14.17. Background images change the appearance of forms and controls. 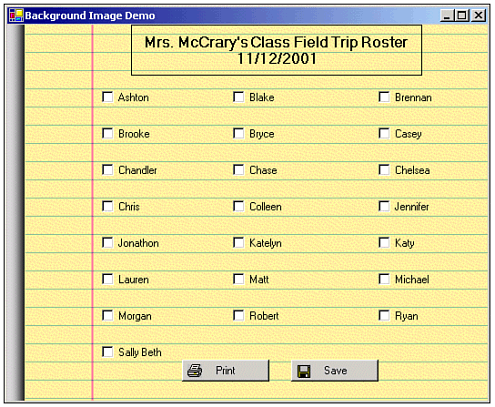 Tip Using a form's BackgroundImage property is an excellent way to provide a texture behind the rest of the form's objects. The following controls (as well as the Form class itself) support the BackgroundImage property: Button CheckBox RadioButton GroupBox PictureBox Panel Setting and Clearing the BackgroundImage Property You can set or clear the BackgroundImage property of a form or control at design time or at runtime using the same techniques discussed for the Image property in the previous section. Using Both the Image and BackgroundImage Properties Several controls, such as the Button control and the Checkbox control, support both the Image and BackgroundImage properties. In such cases, as you might expect, the image contained in the Image property appears on top of the background image, which is tiled behind the other contents of the control. If you use both the Image property and the BackgroundImage property for the same object, take care that the two images do not look bad together. Figure 14.18 depicts two Button controls exhibiting both the BackgroundImage and Image properties. Notice how the two images complement each other in the first control, while they do not look good together at all in the second control. Figure 14.18. For some controls, you can have both an image and a background image. 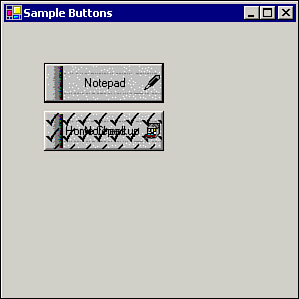 Using Graphics Methods Using controls is not the only way to add graphics to your application. The Graphics class, provided via the System.Drawing namespace, also provides several methods that you can use to draw directly on a form. Some of these methods are listed here: DrawLine. This method draws a straight line between two points. DrawRectangle. This method draws a rectangle or square. DrawEllipse. This method draws a circle or oval. DrawPolygon. This method draws a closed figure with a number of sides. Clear. This method clears the output area of the target object. The topic of drawing graphics in this manner could easily fill up a book on its own. To get your feet wet, we will demonstrate a simple example of using the graphics methods. The easiest way to demonstrate the use of the graphics methods is to write code for a form's Paint event. This event occurs when the form is drawn. It is easy to write code that invokes the graphics methods in this event's event handler because it receives an argument of the PaintEventArgs class, which contains data for the Paint event. To demonstrate some of the graphics methods, create the Paint event handler shown in Listing 14.2 for a form. Comments in the code explain each line. When you run your program, the graphics methods will draw directly on the form. Figure 14.19 shows the form after it has been drawn upon. Figure 14.19. The graphics methods can draw directly on a form. 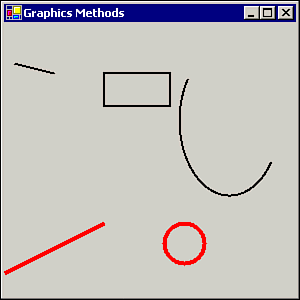 Listing 14.2 Drawing Directly on a Form with the Paint Event Handler Private Sub frmGraphicsMethods_Paint(ByVal sender As Object, _ ByVal e As System.Windows.Forms.PaintEventArgs) Handles MyBase.Paint 'Create an instance of the Graphics class ' to provide graphics functionality. ' Uses the instance of Graphics provided through ' the event arguments. Dim g As Graphics = e.Graphics 'Create two Pen objects to draw in black and red. Dim BlackPen As New Pen(Color.Black) Dim RedPen As New Pen(Color.Red) 'Set the Pen objects' width to 2 and 4 pixels. BlackPen.Width = 2 RedPen.Width = 4 'Invoke the DrawLine method of the Graphics class. ' Calls for a Pen object followed by a starting ' and ending Point object. g.DrawLine(BlackPen, New Point(50, 50), New Point(10, 40)) g.DrawLine(RedPen, New Point(100, 200), New Point(0, 250)) 'Invoke the DrawArc method. g.DrawArc(BlackPen, 175, 22, 100, 150, 45, 180) 'Invoke the DrawRectangle method. ' Calls for a Rectangle object. g.DrawRectangle(BlackPen, New Rectangle(100, 50, 66, 33)) 'Invoke the DrawEllipse method. ' Calls for a Rectangle object that defines the boundary ' of the ellipse. g.DrawEllipse(RedPen, New Rectangle(160, 200, 40, 40)) End Sub |