To write almost any program in Visual Basic, you need to know how to work with text. In earlier chapters, you learned something about displaying text in labels and text boxes. You also learned about string functions used to manipulate text in code. This section will expand on that knowledge by showing you how to display text in a way that is most intuitive to the users of your programs. Text Box Behavior In Chapter 5, "Visual Basic Building Blocks," you were introduced to the text box. You already know that the Text property is used to store and retrieve information that the user may change. In addition to the standard properties discussed in that chapter, several other properties make the TextBox control even more versatile: ReadOnly. This property prevents the users from entering information in the text box. MaxLength. This property limits the number of characters that the text box can accept. PasswordChar. This property causes the text box to hide the information typed by the users. SelectionLength, SelectionStart, and SelectionText. These properties allow the users to manipulate only the selected (highlighted) part of the text in the text box. Preventing Users from Changing Text First, take a look at the ReadOnly property, which allows you to use a text box for display only, taking away the user's ability to change text in that text box. The obvious question is "Why would you want to do that instead of just using a Label control?" One answer is that Windows-savvy users may want to copy text from a TextBox control to the Clipboard to be pasted elsewhere. Although a read-only text box does not allow users to update it, they still can select and copy the text contained in the control, as illustrated in Figure 14.20. Figure 14.20. Using the ReadOnly property prevents undesired editing of text. In the pop-up menu (automatically implemented by Windows), notice that only the Copy, Paste, and Select All options are available for a locked text box. 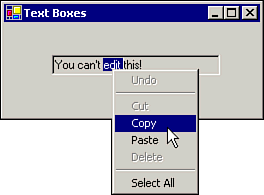 To make a text box read-only, simply set its ReadOnly property to True, as in the following examples: 'Sets a single text box to be read-only txtTest.ReadOnly = True txtTest.Text = "You can't edit this!" 'Sets every text box on the form to be read-only Dim objControl As Control Dim txtTextBox As TextBox For Each objControl In Me.Controls If TypeOf objControl Is TextBox Then txtTextBox = objControl txtTextBox.ReadOnly = True End If Next objControl Tip Use of the ReadOnly property can make it easy to design a database access form. The same form can be used for either viewing or editing a record. When you want to allow your user to only view a record, simply set the ReadOnly property of all the text boxes to True, as shown in the last code example shown previously. Note Do not confuse the ReadOnly property with the Enabled property. Both properties cause the background of the text box to be grayed out; however, the ReadOnly property does not gray out the text contained in the text box. Additionally, it allows the users to select and copy text from the text box, but the Enabled property does not. The MaxLength Property When you're designing a data entry form, one of the tasks you must perform is data validation. One type of data validation is making sure the data entered will fit in the designated database field. For example, consider a text box whose purpose is to input a seven-digit account number. Although this check can be performed in code with the Len function, you can avoid the extra code by using the TextBox control's MaxLength property. The MaxLength property allows you to specify the maximum number of characters that can be entered in a text box, regardless of its size on the form. If your user attempts to type more characters into the text box than the MaxLength property will allow, the text box will not accept the extra characters, and a system beep will be heard. Tip You can programmatically put more text into a TextBox control than its MaxLength property would normally allow. Simply use code to set the Text property to the desired string, as in txtLastName = "Murgatroyd". The PasswordChar Property If you are using a text box as part of a login form, you will want to be able to hide the password entered by the user. To do so, simply enter a character in the PasswordChar property of the text box. This property changes the text box's display behavior so that the password character is displayed in place of each character in the Text property. You may have seen this effect many times when logging in to applications or in to Windows itself. Note that the contents of the Text property still reflect what was actually typed by the users, and your code always sees the "real" text. Although you can enter any character, using the asterisk (*) character for hiding text is customary. Editing Text in a Text Box A standard textbox allows the user to highlight text either by using the mouse or by using Ctrl or Shift in combination with the cursor keys. Your code can then manipulate the selected text with the SelectionText, SelectionLength, and SelectionStart properties. You can use these properties to work with a selected piece of text in your program. Look at the example in Figure 14.21. In this case, the SelectionText property would contain just the phrase "jumped over the lazy dog." The SelectionLength property would contain the integer value 25, which is the length of that string. And the SelectionStart property would contain the integer value 20, which means the selected phrase starts with the 20th character in the text box. Figure 14.21. Your users can copy text from one text box and paste it elsewhere. 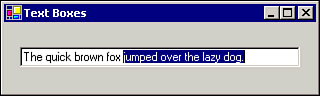 In addition to determining what has been selected, you can also set the properties from code to alter the selection. First you set the SelectionStart property, then set the SelectionLength property to highlight some characters. The following code would select the first three characters of the text contained in the TextBox control txtTest when the control gets the focus: Private Sub txtTest_GotFocus(ByVal sender As Object, _ ByVal e As System.EventArgs) Handles txtTest.GotFocus txtTest.SelectionStart = 0 txtTest.SelectionLength = 3 End Sub The SelectionLength property can be changed multiple times. This causes the selection to increase or decrease in size, automatically updating the SelectionText property. Setting the SelectionText property from code causes the currently selected text to be replaced with a new string for example: txtTest.SelectionText = "jumped into oncoming traffic." Note One classic use of the properties that govern the selected text in a TextBox control has been code like the following, which is placed in the control's GotFocus event procedure to cause the entire contents of the text box to be selected when it gets the focus: Private Sub txtSelect_GotFocus() txtSelect.SelectionStart = 0 txtSelect.SelectionLength = Len(txtSelect.Text) End Sub Beginning with Visual Basic .NET, however, this functionality is built into the TextBox control. All of your text boxes will have their text automatically selected when they get the focus, without your having to write code to accomplish this task. Working with Fonts and Colors Although drawings and pictures add a definite visual impact to your applications, the heart and soul of many of your programs will likely be the text fields used for data entry and information display. Often, adding an image to enhance onscreen text is not possible or even useful. Instead, you must make sure that you use appropriate fonts and colors to get your point across. Using the Font Object If you have used a word processor program, then you are already familiar with fonts. At design time, you assign fonts to controls and forms by using the Properties window. If you change the font of a form from the default setting, any controls subsequently drawn on the form as well as any existing controls whose Font property has not been modified will use the new font. You may remember from earlier chapters that the Font property of an object is actually an object that has its own properties. The following Font object properties can be used to control the appearance of fonts in your program: Name. String identifier for one of the fonts installed on your system for example, "Arial"or "Times New Roman". Unit. Specifies the unit of measurement of the font's size. Used in conjunction with the Size property. The default value is Point; one point is 1/72 of an inch; therefore, capital letters in a 72-point font are about one inch high. Other possible values include Pixel, Inch, and Millimeter. Size. Specifies the size of the font, based on the value selected for the Unit property. Note that if you change the Unit property, the Size property is not automatically modified. Therefore, for example, if a Font object's Unit property is set to Point and its Size property is set to 12, and then you change the Unit property to Inch, you will end up with 12-inch tall characters! Bold. True/False property that controls whether characters are shown in boldface. The bold characters appear darker and heavier than non-bold characters. Italic. True/False property that determines whether characters are italicized. Underline. True/False property that controls whether the text is displayed with a thin line under each character. Strikeout.True/False property that controls whether a thin horizontal line is drawn through the middle of the text. Adding a Splash of Color A form designed with totally battleship-gray components can be dull and plain. However, you can easily assign color to the form and controls on it by using the ForeColor and BackColor properties. To set these properties at design time, you can use one of the colors defined for components of the system (Control, Desktop, Menu, and so on); a member of the Web collection of colors; or a customized color. To view the possible choices, bring up the Properties window for a control or form, and click on the ForeColor or BackColor property. The color picker window that appears lets you choose between one of the three tabs System, Web, or Custom. The Custom tab is shown in Figure 14.22. Figure 14.22. You can specify an object's foreground or background color in one of several ways. 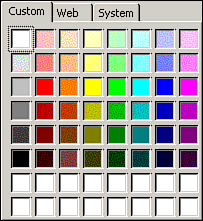 Using Fonts and Colors with a RichTextBox Control The text box is great for general use, but the RichTextBox control allows even greater control over how text displayed. The strength of the RichTextBox control, introduced in Chapter 12, "Advanced Controls," is that it can display multiple formats and colors within the same text area. The standard TextBox control allows you to change the font used to display all the text in the control. The RichTextBox control, however, lets you use multiple fonts and font properties within the same control, as depicted in Figure 14.23. Figure 14.23. The RichTextBox control lets you use many fonts in one control. 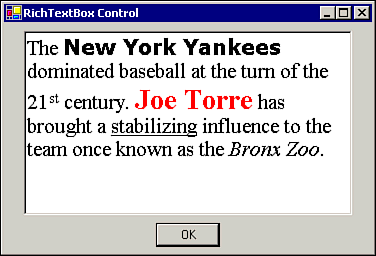 |