A big part of any programming project is providing information to your users about the program's progress and status. Although the forms and controls of your program provide the main interface to the users, they are not necessarily the best vehicles for providing bits of information that require immediate attention, such as warnings or error messages. For providing this type of information, a message box is often the best means of communication. Understanding the Message Box A message box is a simple form that displays a message and at least one command button to the user. The button is used to acknowledge the message and close the form. Because message boxes are built in to the .NET framework, you do not have to worry about creating or showing a form for this purpose. Although message boxes are quite useful, they do have a few limitations: The message box cannot accept text input from the user. It can only display information and handle the selection of a limited number of choices. You can use only one of a set of predefined icons and one of several predefined button sets in the message box. You cannot define your own icons or buttons. By design, the message box requires a user to respond to a message before any part of the program can continue. This means that the message box cannot be used to provide continuous status monitoring of the program because no other part of the program can be executing while the message box is waiting for the user's response. A Simple Message Box To use a message box, you call the Show method of the MessageBox class and supply at least one argument. The first argument, text, specifies the message that you want to show to the user. To see an example of the simplest form of a message box, follow these steps: -
Add a Button control to the top left of your sample application's main form. Set its Name property to cmdMsgBox1 and set its Text property to Simple MessageBox. -
Add the following line of code to the Click event handler for the cmdMsgBox1 button: MessageBox.Show("You clicked me!") -
Save and run your sample program. When you click the button you will see a simple message box displayed, as shown in Figure 13.1. Figure 13.1. A simple message box can be displayed with one line of code. 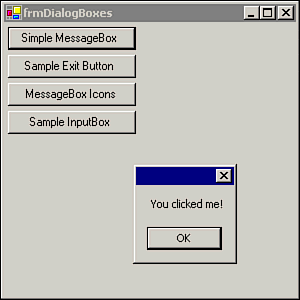 -
Click OK to close the dialog box, then close the form to end the program. Note that the message box displays the string that we supplied as the Show method's first (and only) argument. Note If the text that you want to display in the message box is long, line breaks will be inserted automatically. You can control where the line breaks will appear by including a carriage return/line feed combination (using the predefined constant vbCrLf) at the appropriate point(s) in your text, as follows: MessageBox.Show("Copyright 2001" & vbCrLf & "Mike Watson Enterprises") Adding a Title You may have noticed that the title bar of the message box was empty. If you like, you can enhance the message box's Show method to include a caption in the title bar. As you saw in the first example, the text argument of the Show method is the actual message to be displayed in the message box. The (optional) second argument, caption, is used to specify the caption that will appear in the title bar. To see how this works, modify the Click event handler for cmdMsgBox1to look like the following: MessageBox.Show("You clicked me!", "My First Message Box") Test the simple message box again, and notice the caption that appears in its title bar, as illustrated in Figure 13.2. Figure 13.2. You can add a title to your message box by supplying a second argument. 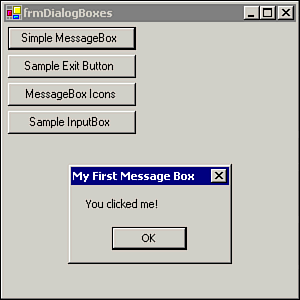 Using Multiple Buttons for Two-Way Communication The simple message box that you have seen so far works well for one-way communication to the user. With a couple of simple changes, you can enhance the message box to allow the user to return information to your program once he has read the message. A classic example of this technique would be asking the user to confirm an action he has requested, such as deleting information or exiting the program. To use a message box for two-way communication, you need to do two extra things instruct the message box to display multiple buttons, and capture the value generated by the message box. These techniques are discussed next, then you will incorporate them into an example. Showing Multiple Buttons To cause the messagebox to display multiple buttons from which the user may choose, you need to use a member of the MessageBoxButtons enumeration as the buttons (third) argument of the Show method. You have several choices, as follows: OK. Displays a single button with the caption OK. This button simply directs the user to acknowledge receipt of the message before continuing. OK, Cancel. Displays two buttons in the message box, letting the user choose between accepting the message and requesting a cancellation of the operation. Abort, Retry, Ignore. Displays three buttons, usually along with an error message. The user can choose to abort the operation, retry it, or ignore the error and attempt to continue with program execution. Yes, No, Cancel. Displays three buttons, typically with a question. The user can answer yes or no to the question, or choose to cancel the operation. Yes, No. Displays two buttons for a simple yes or no choice. Retry, Cancel. Displays the two buttons that allow the user to retry the operation or cancel it. A typical use is reporting that the printer is not responding. The user can either retry after fixing the printer or cancel the printout. You can indicate your choice of button configuration by using one of the following members of the MessageBoxButtons enumeration as the Buttons argument: OK, OKCancel, AbortRetryIgnore, YesNoCancel, YesNo, or RetryCancel. Capturing the Value Much like a normal function, the MessageBox class returns a value indicating which button the user clicked to close the box. Typically, you will use a variable to store this value; your program can then examine the contents of the variable when processing continues. Visual Basic .NET provides a DialogResult enumeration that contains the possible return values, as follows: DialogResult Member | Button Clicked |
---|
Abort | The user clicked the Abort button. | Cancel | The user clicked the Cancel button. | Ignore | The user clicked the Ignore button. | No | The user clicked the No button. | OK | The user clicked the OK button. | Retry | The user clicked the Retry button. | Yes | The userclicked the Yes button. | Adding a Two-Way Button to the Sample Program To add a button demonstrating two-way communication to our project, let's create a button that emulates a typical command to end a program. Follow these steps: -
Add a second Button control just below the first button on the sample project's frmDialogBoxes. -
Set the second button's Name property to cmdMsgBox2, and set its Text property to Sample Exit Button. -
Place the following code in cmdMsgBox2's Click event handler: Dim nTemp As Integer Dim sMsg As String sMsg = "Are you sure you want to exit the program?" nTemp = MessageBox.Show(sMsg, "Confirm Exit", MessageBoxButtons.YesNo) If nTemp = DialogResult.Yes Then Application.Exit() End If This code uses an Integer variable named nTemp to store the value generated by the message box's Show method when the user clicks a button. It also uses the YesNo member of the MessageBoxButtons enumeration to cause Yes and No buttons to appear in the message box. After the user has closed the dialog box, the code compares the value of nTemp to the Yes member of the DialogResult enumeration. If it matches, then the user has clicked the Yes button to close the dialog box, and the code ends the application. After adding this code, save and run the program. Click the Sample Exit button, and you will see a dialog box with Yes and No buttons, as shown in Figure 13.3. Figure 13.3. A message box can include multiple buttons to allow the user to make a choice. 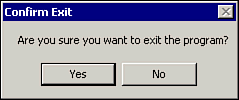 Note This example also illustrates the use of a String variable (sMsg) to contain the message displayed by the message box. The previous example used a literal string; either is acceptable. Adding an Icon The message boxes you have seen so far have included a message and a button. You can use the third argument, icon, of the Show method to add an icon to your message box. There are eight icons available for you to choose from; the MessageBoxIcon enumeration is provided to assist you in specifying which one you want. You should choose an icon that helps communicate the situation being reported by the message box. Table 13.1 lists the members of the MessageBoxIcon enumeration and typical uses: Table 13.1. Icons Indicate the Type of Message Being Shown Icon | MessageBoxIcon Member | Typical Purpose |
---|
 | Asterisk | Informs the user of the status of the program. This message is often used to notify the users of the completion of a task. |  | Error | Indicates that a severe error has occurred. Often a program is shut down after this message. |  | Exclamation | Indicates that a program error has occurred; this error may require user correction or may lead to undesirable results. |  | Hand | Same as the Error icon. |  | Information | Same as the Asterisk icon. | | None | No icon is displayed. |  | Question | Indicates that the program requires additional information from the user before processing can continue. |  | Stop | Same as the Error icon. |  | Warning | Same as the Exclamation icon. | To test the icons, add a third Button control named cmdMsgBox3 to your test program. Set its Text property to MessageBox Icons. Add the following line of code to its Click event handler: MessageBox.Show("I see an icon!", "Icon Test", _ MessageBoxButtons.OK, MessageBoxIcon.Asterisk) When you run the program and click the MessageBox Icons button, you will see a message box with an Information icon, as illustrated in Figure 13.4. Figure 13.4. You can add icons to enhance the appearance of your message boxes. 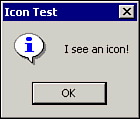 Experiment with different values from the MessageBoxIcon enumeration for the icon argument so you can see what the different icons look like. Note The possible values for the icon argument not only affect the icon that is displayed, but also the sound produced by Windows when a message appears. Your user can set sounds for different message types in the Windows Control Panel. Setting the Default Button If you are using more than one button in the message box, you can specify which button is the default. The default button is the one that has focus when the message box is displayed. This button is the one that the user is most likely to choose so that he or she can just press the Enter key to select it. For example, if you display a message box to have the user confirm the deletion of a record from a database, you probably should set up the default button so that the No button is the default; therefore, the record would not be deleted if the user just presses Enter. This way, the user must make a conscious choice to delete the record. To specify which button is the default, you need to specify the fourth argument, defaultButton, for the Show argument. The MessageBoxDefaultButton enumeration supplies three values that correspond to the three possible buttons that may be displayed, as follows: MessageBoxDefaultButton Member | Default Button |
---|
Button1 | The first button | Button2 | The second button | Button3 | The third button | Try experimenting with the defaultButton argument on your own. Note The MessageBox.Show method supports one more argument, MessageBoxOptions, which allows you to specify some special-purpose functionality of the message box. See the "MessageBoxOptions enumeration" topic in the Help system for more information. |