Many times in a program, you need to get a single piece of information from the user. You might need the user to enter a name, the name of a file, or a number for various purposes. Although the message box lets your user make choices, it does not allow him to enter any information in response to the message. Therefore, you have to use some other means to get the information. Visual Basic provides a built-in dialog box for exactly this purpose: the input box. The input box displays a prompt to tell the user what to enter, a text box where the user can enter the requested information, and two command buttons OK and Cancel that can be used to either accept or abort the input data. A typical input box in use is shown in Figure 13.5. Figure 13.5. An input box lets the user enter a single piece of information in response to a prompt. 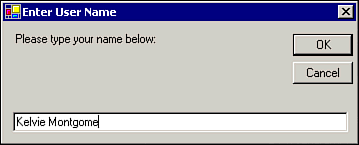 Setting Up the InputBox Function Programmatically, a call to the InputBox function works much like calling the MessageBox class and utilizing its return value. You can specify a variable to receive the information returned from the input box and then supply the input box's message (prompt) and, optionally, a title and default value. To see the InputBox in action, perform these steps: -
Add another Button control on the sample project's frmDialogBoxes. -
Set this button's Name property to cmdInputBox, and set its Text property to Sample InputBox. -
Place the following code in cmdInputBox's Click event handler: Dim sMsg As String, sUserName As String sMsg = "Please type your name below:" sUserName = InputBox(sMsg, "Enter User Name", "Anonymous") MessageBox.Show("Hi, " & sUserName & "!") Save and run the sample project, then click the Sample InputBox button. Figure 13.6 shows the input box as initially presented, including the default value in the input area. Figure 13.6. The InputBox function allows the user to enter information. 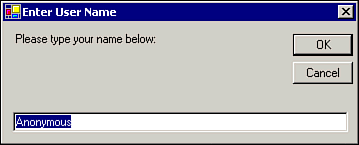 You can see that the user is presented with the prompt that you specified, and he is given a text box in which to type his response. In addition, the text box is populated with the default value (Anonymous). In this example, the information returned by the InputBox function is stored in the variable sUserName. The first argument, the Prompt parameter, represents the message that is displayed to the user to indicate what should be entered in the box. The prompt can display up to approximately 1,024 characters before it is truncated. As with the message box, word wrapping is performed automatically on the text in the prompt so that it fits inside the box. You can insert a carriage return/line feed combination (represented by the predefined constant vbCrLf) to force the prompt to show multiple lines or to separate lines for emphasis. After the prompt comes the Title argument, which specifies the text in the input box's title bar. The other argument in the preceding example is the Default argument. If included, it appears as an initial value in the input box. The user can accept this value, modify it, or erase it and enter a completely new value. The minimum requirement for the InputBox function is simply a prompt parameter, as in this statement: sReturnVal = InputBox("How's the weather?") In addition to the input box's optional parameters to specify a window title and default value, other optional parameters allow you to set its initial screen position. Refer to the complete syntax of the InputBox function in Visual Basic's help system. Note Unlike the MsgBox function, no option in the InputBox function specifies any command buttons other than the defaults of OK and Cancel. Values Returned by InputBox When the input box is used, the user can enter text in the input box's entry area, which resembles a text box. If the user types more text than will fit in the displayed entry area, the text he or she has already typed scrolls to the left. After the user is done, he or she can choose the OK or Cancel button. If the user chooses the OK button, the input box returns whatever is in the text box, whether it is new text or the default text. If the user chooses the Cancel button, the input box returns an empty string, regardless of what is in the text box. To be able to use the information entered by the user, you must determine whether the data meets your needs. First, you probably should make sure that the user actually entered some information and chose the OK button. You can do so by using the Len function to determine the length of the returned string. If the length is zero, the user clicked the Cancel button or left the input field blank. If the length of the string is greater than zero, you know that the user entered something. You might also need to check the returned value to make sure it is of the proper type. If you are expecting a number that will subsequently be compared to another number in an If statement, your program should present an error message if the user enters letters. To make sure that you have a numerical value with which to work, you can use the IsNumeric function. The IsNumeric function's purpose is to indicate whether an expression can be evaluated as a number. If the entire expression is recognized as a number, the function returns True; otherwise, it returns False. The following code illustrates additional processing of the returned value of an input box with IsNumeric and Len: Dim sInputVal As String sInputVal = InputBox("Enter your age") If Len(sInputVal) = 0 Then MessageBox.Show("No age was entered") Else If IsNumeric(stInputVal) = 0 Then MessageBox.Show("Congratulations for surviving this long!") Else MessageBox.Show("You entered an invalid age.") End If End If |