Although some Pocket PCs are equipped with wireless network cards, most of them can only exchange data with another PC when connected in their docking station. This process is known as synchronizing your PC with your Pocket PC. Microsoft includes a program called ActiveSync, pictured in Figure 27.9, which performs this process. Figure 27.9. Microsoft ActiveSync, available for free download, updates databases, e-mail, and offline Web pages on your Pocket PC. 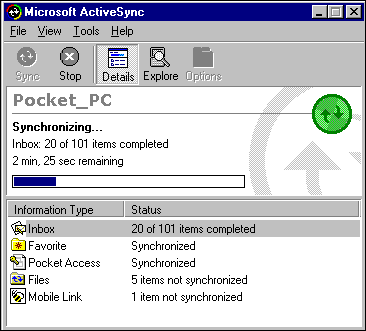 If you have the ActiveSync program installed, it will automatically appear and begin exchanging information with your Pocket PC each time you dock it. For our sample application, we'll need to move the inventory data we gathered to the desktop PC for processing. As you might imagine, you can manage this process manually by allowing ActiveSync to transfer the information to an Access database. However, in order to make the process as easy as possible for the end user, we will show how to use API calls to control it from within a VB .NET application. Understanding the API Calls When you transfer information between the Pocket PC and another PC using ActiveSync, you are essentially just transferring files. ActiveSync has filters that convert a specific file type on the Pocket PC to its Desktop PC counterpart. In the case of an ADOCE database, a filter is provided that converts a cdb file to the mdb (Access) file format. To transfer the inventory database, we will make use of this filter via API calls. To begin, start Visual Studio .NET and create a new Windows Application project. Enter the following API declarations at the top of your form class: Private Declare Function DEVICETODESKTOP Lib "c:\program files\Microsoft ActiveSync\adofiltr.dll" _ (ByVal desktoplocn As String, _ ByVal tablelist As String, _ ByVal sync As Boolean, _ ByVal overwrite As Integer, _ ByVal devicelocn As String) As Long Private Declare Function DESKTOPTODEVICE Lib_ "c:\program files\Microsoft ActiveSync\adofiltr.dll" _ (ByVal desktoplocn As String, _ ByVal tablelist As String, _ ByVal sync As Boolean, _ ByVal overwrite As Integer, _ ByVal devicelocn As String) As Long The purpose of these two API calls is self-explanatory. You simply call the appropriate function depending on the desired direction of data transfer. Note As with any API declaration, a reference to an external DLL is required. If you installed ActiveSync in a directory other than the default, you will need to search your hard drive for adofiltr.dll and modify the path appropriately. Keep in mind the following notes regarding use of the parameters: The desktoplocn and devicelocn parameters describe the paths to the mdb and cdb files, respectively. Note that the devicelocn parameter will never contain a drive letter. By specifying the tablelist parameter, you can choose to copy only certain tables within a database. Sending an empty string will copy all tables. Setting the sync parameter to True will register the process with the ActiveSync program under the Pocket AccessSync Options tab. To overwrite an existing database file, set the overwrite parameter to 1. More information on the DESKTOPTODEVICE and DEVICETODESKTOP API calls is available in the eMbedded Visual Basic help files. Coding the Data Transfer Synchronizing our inventory database will be a three-step process. First, we will copy the data from the Pocket PC to a folder on the PC hard drive. Next we will process the data and delete old records. Finally, we will send the updated database back to the Pocket PC. To set up the data transfer application, perform the following steps. -
Open Windows Explorer and create a new folder on your hard drive called C:\InventoryInfo. This folder will store the Access mdb file. -
Add a Button control to your form. Set its Name property to btnSyncData and its Text property to Transfer Inventory Database. -
Add the code in Listing 27.4 to your form. Listing 27.4 PPCDEMO.ZIP Database Transfer with the Pocket PC Private Sub UpdateInventoryDatabase() Const sMDBPath As String = "C:\InventoryInfo\Inventory.mdb" Const sCDBPath As String = "\My Documents\InventoryDB.cdb" Dim result As Long Dim Answer As DialogResult 'Show a message box to confirm that they really want to do this Answer = Messagebox.Show("Transfer Inventory data from Pocket PC?",_ "Are you sure?", MessageBoxButtons.OKCancel, MessageBoxIcon.Question) If Answer = DialogResult.OK Then Me.Text = "Copying Data from device..." result = DEVICETODESKTOP(sMDBPath, "", False, 1, sCDBPath) Me.Text = "Processing Data..." Call ProcessNewInventory() Me.Text = "Copying data to device..." result = DESKTOPTODEVICE(sMDBPath, "", False, 1, sCDBPath) Me.Text = "Done!" End If End Sub Private Sub ProcessNewInventory() Dim cn As OleDbConnection Dim cmd As OleDbCommand Dim dtPurgeDate As Date = Today.Now.AddHours(-1) Const sMDBPath As String = "C:\InventoryInfo\Inventory.mdb" cn = New OleDbConnection(_ "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" & sMDBPath) cn.Open() cmd = cn.CreateCommand() cmd.CommandType = CommandType.Text 'This command deletes records more than an hour old cmd.CommandText =_ "Delete from InventoryInfo Where DateEntered < #" &_dtPurgeDate.ToString & "#" cmd.ExecuteNonQuery() cn.Close() End Sub Private Sub btnSyncData_Click(ByVal sender As System.Object,_ ByVal e As System.EventArgs) Handles btnSyncData.Click Call UpdateInventoryDatabase() End Sub The UpdateInventoryDataBase function uses the two ADOCE API calls to transfer data to and from the Pocket PC. During this process, the form caption is updated with status information so the user knows what is happening. When the data has been loaded to the PC, the code calls the ProcessNewInventory function. In our sample application, this function uses ADO .NET to delete records from the table that are more than an hour old. However, you could also upload data to a central SQL database, print reports, or any other desired tasks. Testing the Data Transfer If you have not already entered some inventory items on your Pocket PC, do so now. After you have finished, click the OK button to close the application on the Pocket PC. Finally, dock your Pocket PC and perform the following steps: -
Press the Start button in Visual Basic .NET to start your data transfer application. -
When the form appears, click the Transfer button. -
Answer OK to the confirmation message. -
The data will be transferred to your PC and back. After completing the transfer process, connect to the file C:\InventoryInfo\Inventory.mdb using Access or the Visual Studio .NET Server Explorer. Open the InventoryInfo table and you should see the data you entered on the Pocket PC. If you had any data that was an hour old, it should be deleted from both the Pocket PC and the PC database table. Automatically Running the Transfer Program As a final enhancement to the transfer program, you can automatically start it each time the Pocket PC is docked. This is easily accomplished by performing the following steps: -
Remove the button control from your form and add the following lines of code to the end of the form's New method: Call UpdateInventoryDatabase Messagebox.Show("Done") Application.Quit -
Compile your program by choosing Build from the Build menu. -
Copy your program's executable to the C:\InventoryInfo folder. -
From the Windows Start menu, choose Run and type Regedit to display the Registry Editor. -
Using the Registry Editor, navigate to the following key: HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows CE Services\AutoStartOnConnect -
Right-click the key and choose to add a new string value. -
Name the string MySync program and for the value enter the path to your executable, C:\InventoryInfo\PocketSync.exe, as shown in Figure 27.10. Figure 27.10. You can automatically launch programs when a PC is docked or undocked by simply adding a registry value. 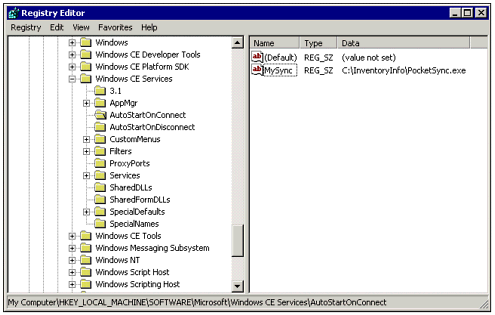 By adding additional registry entries, you can cause any number of programs to be executed when a Pocket PC is connected or disconnected. |