As you can see from Figure 9.1, there are four primary stages in the life cycle of a JNDI application. Some stages in the life cycle occur on the server side and some on the client side. Register with the naming service. The first stage in any JNDI application is for the server component to register with the naming service. To do this, the server constructs the InitialContext object initialized with WebLogic Server's implementation of the InitialContext. The server component registers itself with the naming service using the following methods: bind() The bind() method accepts two parameters for registering a server component: The first parameter is a logical name that can be used by clients to query the naming service to find the server component, and the second parameter is the actual server component object reference. The bind() method is an overloaded method that can accept the server component's logical name wrapped in a javax.naming.Name object. If a server component with the same name already exists when the server component calls the bind() method to register into the naming service, the bind() method throws a javax.naming.NamingException. rebind() The rebind() method is similar to the bind() method with one difference: The server component can overwrite an existing reference bound in the naming service with the new object reference and does not throw an exception. It is always a safer and better practice to use the rebind() method instead of the bind() method. The rebind() method, like the bind() method, is overloaded. This method is useful when a server component is taken down and needs to re-register itself with the naming service with a new object reference without restarting the naming service. Look up the object in the naming service. Once a server component's reference is registered in the naming service, the JNDI client application can contact the naming service to obtain a reference to the server component's object reference. This is done using the lookup() method. lookup() The lookup() method is used by a JNDI client application to obtain a server component's object reference. The lookup() method takes the logical name of the server component whose reference is required, as a param eter. The lookup() method returns a java.lang.Object object that is the reference of the server component stored in the naming service. Cast the object to its appropriate type. After obtaining the object reference, the client application converts the object returned by the naming service. The object reference obtained from the naming service must always be cast to its appropriate type. The returned reference of the server object is now ready to be used by your client application. Termination. The final stage in a JNDI application is the removal of the server component's object reference from the naming service using the unbind() method. If a JNDI client application tries to look up the server component in the naming service after the server component's object reference has been removed from the naming service using the unbind() method, a javax.naming.NamingException is thrown. unbind() The unbind() method is used at the end of the life cycle of the server component to remove the server component's reference from the naming service. The unbind() method takes the logical name of the server component as a parameter. The unbind() method throws a javax.naming.NamingException if the logical name is not found in the naming service. close() When the client application is done using the server object, it can free up resources by invoking the close() method. The close() method is also used by the server object after the server object is unbound from the naming context. Figure 9.1. Life cycle of a JNDI application server and client.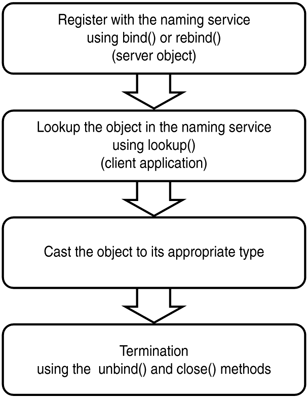 Figure 9.2 lists the steps involved in developing a JNDI application. Because a JNDI application is composed of server-side as well as client-side interaction with the naming service using the JNDI API, you will look at both while writing the JNDI application. Figure 9.2. Flowchart for developing a JNDI application server and client.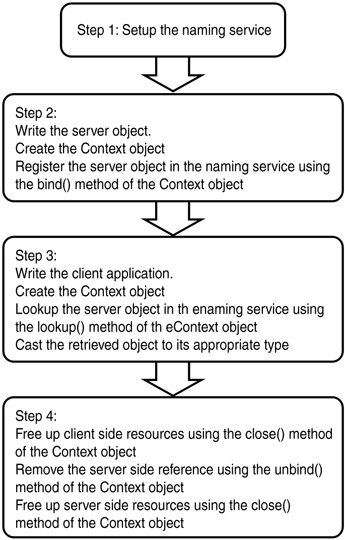 The first step in a JNDI application is, of course, to set up a naming service and have a driver compatible with the JNDI API that can interact with the naming service. The naming service is the focal point in a JNDI application. The next part of the JNDI application is writing a server-side component and registering it with a naming (or directory) service. To register with a naming service, the server-side object needs to create a Context object by instantiating an InitialContext object (provided by the naming-service provider's JNDI driver) and then to pass any values specific to the naming-service (or directory service) provider to initialize the Context object. Hashtable ht = new Hashtable(); ht.put(Context.PROVIDER_URL, "JNDI service provider URL"); ht.put(Context.INITIAL_CONTEXT_FACTORY, "JNDI service provider driver classes"); Context ctx = new InitialContext(ht); The Context object provides the means for the server object to register itself with the naming service using a name identifier (and attributes for a directory service) with the bind() method. ServerObj myServerObj = new ServerObj(); ctx.bind("bind_name", myServerObj); The server object is now ready to be accessed by client applications using the JNDI API. Once the server object is registered, a client application uses the JNDI API to connect to the naming service and access the server object. To connect to the naming service, the client application constructs the Context object by instantiating an InitialContext object as was done in the previous step. After creating the Context object, the client application uses the lookup() method with the binding name as a parameter to retrieve the object reference from the naming service. The retrieved object reference is then cast to the appropriate server object type. The client is now all set to use the server object! //... construct the context object in the same way as was done for the server object ServerObj serverObjRef = (ServerObj)ctx.lookup("bind_name"); //... use the server object in the client application After using the services of the server object, the final step is to free the resources used by the Context object reference by invoking the close() method in the client application. ctx.close(); |