A sequence is a string of characters (letters, numbers, and special characters) placed in a specific order or formatted in a special way. Following are some sample sequences: Telephone number (xxx-xxxx) Credit card number (xxxx xxxx xxxx xxxx) Date (xx/xx/xxxx) URL (http://www.xxxxxx.xxx) Although the characters within sequences can change, they must still follow certain formatting rules. Sequence validation is typically a bit more involved than other types of data validation, primarily because there are numerous validation points to check. By breaking down the process, you can more readily understand how it works. The following are some validation points for a typical email address: It cannot contain more than one @ symbol. It must include at least one period (separating the actual domain name from the domain extension, such as in mydomain.com or mydomain.net). The last period must fall somewhere after the @ symbol, but it can't be either of the last two characters. The @ symbol cannot be the first or second character. There must be at least two characters between the @ symbol and the first period that precedes it. The email address must include at least eight characters (aa@bb.cc). 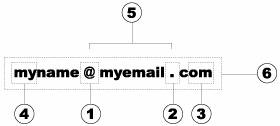 Note If an email address has been checked for the six points listed previously, you performed a reasonable validation. Although we could add many more validation points (for example, characters following the last period must be com, net, org, or something similar), your code would become extremely long and you would need to update it frequently to keep pace with changing Internet standards. It's crucial to determine the most important validation points and check for them. In this exercise, we'll check only the following three validation points for text entered into the email_ti instance of our registration form: The @ symbol must be included somewhere after the second character. The email address must include a period at least two characters after the @ symbol. The email address must consist of at least eight characters. Note Be sure to provide users with clear instructions about how to format data. It's common practice to provide an example of correctly formatted data either above or below the text box where it's to be entered, providing a quick reference for the user. 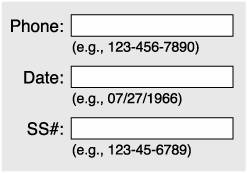 1. | Open validate3.fla.
We will continue building on the project from the last exercise.
| 2. | With the Actions panel open, select Frame 1 on the Actions layer and add the following function definition at the end of the current script:
function validateEmail() { if (email_ti.text.indexOf("@") < 2) { errors.push("@ missing in email or in the wrong place."); email_ti.setStyle("color", 0x990000); } if (email_ti.text.lastIndexOf(".") <= (email_ti.text.indexOf("@") + 2)) { errors.push(". missing in email or in the wrong place."); email_ti.setStyle("color", 0x990000); } if (email_ti.text.length < 8) { errors.push("Email address not long enough."); email_ti.setStyle("color", 0x990000); } } The validateEmail() function validates the text entered into the email_ti instance and is made up of three conditional statements, each of which checks one of the individual validation points we outlined at the beginning of this exercise. Because these are separate if statements, rather than if/else, if/else if groupings, all of them will be evaluated. Let's look at each one in depth. The first statement reads as follows:
if (email_ti.text.indexOf("@") < 2) { errors.push("@ missing in email or in the wrong place."); email_ti.setStyle("color", 0x990000); } You'll remember from Lesson 5, "Built-in Classes," that the indexOf() method returns the position (character number) where the value in the parentheses is first found in a string.
Using this method, the previous statement determines whether the first @ symbol appears before the third character in the email_ti instance. Because the first character in a string has an index number of 0, this statement evaluates to true, and an error message is pushed into the errors array if the @ symbol is found at position 0 or 1. If the @ symbol doesn't occur anywhere within the email_ti instance, this statement returns a value of -1, which is still less than 2; this result causes the statement to evaluate to true and the error message to be pushed into the array. In addition to an error message being pushed into the errors array whenever this error occurs, the text in the email_ti is styled as red, which is helpful for emphasizing the location of the error.
Note Using the indexOf() method, you can also check for the existence of strings longer than one character. For example, you can check for http:// in a string by using something similar to the following syntax: string.indexOf("http://"). The number returned is the character number of the first letter in the string. Let's look at the second statement in this function, which reads as follows:
if (email_ti.text.lastIndexOf(".") <= (email_ti.text.indexOf("@") + 2)) { errors.push(". missing in email or in the wrong place."); email_ti.setStyle("color", 0x990000); } This statement uses the lastIndexOf() methodsimilar to the indexOf() method except that it returns the character number of the last occurrence of the character in the parentheses. The following example returns 28:
email_ti.text = "derek.franklin@derekfranklin.com",¬ email_ti.text.lastIndexOf(".") Using this method, the statement looks at the position of the last period in relation to the @ symbol. If the period is less than two characters to the right of the @ symbol, this statement proves TRue, and an error message is pushed into the errors array. Again, if this error occurs, the text in the email_ti instance is styled in red, indicating the location of the error.
The third statement in this function is the easiest one to comprehend. It reads as follows:
if (email_ti.text.length < 8) { errors.push("Email address not long enough."); email_ti.setStyle("color", 0x990000); } The smallest reasonable email address is aa@bb.cceight characters, including the @ symbol and the period. If the length of the text entered into the email_ti instance is fewer than eight characters, an error message stating that the email address is too short is pushed into the error array and the text in the email_ti instance is styled as red.
After all these statements have been evaluated, you might find that the information entered into the email_ti instance is invalid on all counts. In this case, three different error messages would be pushed into the errors array.
| 3. | Add the following function call just below the validateName() function call in the validateForm() function definition:
validateEmail(); This is a call to the function we just defined. Placing this function call here adds email validation capability to the main validateForm() function. This function call is placed just above the statement that checks the length of the errors array because the validateEmail() function can push error messages into the array, thus affecting its length and the way the statement is evaluated. In the end, if either the validateName() or validateEmail() function finds errors, the corresponding messages will be displayed in the errorLog_lb instance.
Note The Submit button already calls the validateForm() function when clicked; therefore, any new functionality we add to that function (as we have just done) is automatically executed when the button is released. | 4. | Choose Control > Test Movie to test the project up to this point.
Enter an invalid email address into the email_ti instance or an invalid name into the name_ti instance to see what the validation process turns up. Clicking the Clear button resets the visual and data elements in the scene.
| 5. | Close the test movie to return to the authoring environment, and save this file as validate4.fla.
We will build on this file in the following exercise.
| |