8.1. Create Themes and Skins Most web designers use Cascading Style Sheets (CSS) to give their web sites a consistent look and feel. In ASP.NET 2.0, you can now create themes and skins that extend a consistent look and feel to your server controls. In this lab, you will learn how to create skins for your web controls and how to group them into themes. Themes Versus CSS Stylesheets While both themes and CSS stylesheets let you apply common sets of attributes to web pages, they do have their differences: Themes can define properties of controls, such as the images to be used for an ImageMap control. CSS stylesheets, on the other hand, can define only certain sets of style properties. Theme property values always override local property values, while stylesheets can cascade. Note that you can use themes and CSS together. Themes and skins allow you to cleanly separate your UI into layers, separating form from function. This greatly increases the manageability of your project. |
8.1.1. How do I do that? In this lab, you'll create some skin files and group them into a theme. Then, you'll apply this theme to a page to change its look and feel and observe the result. Launch Visual Studio 2005 and create a new web site project. Name the project C:\ASPNET20\chap08-Themes. Populate the default Web Form (Default.aspx) with the Label, Calendar, and Button controls shown in Figure 8-1. Figure 8-1. Populating the form with various controls 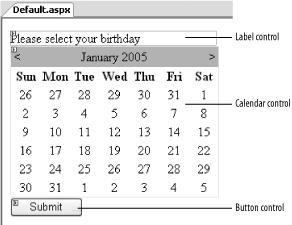
Create a new folder in your project by right-clicking the project name in Solution Explorer and then selecting Add Folder Themes Folder. The folder will be used to hold various themes folders for your application. Note that a new folder named Theme1 will be created under App_Themes. Change the name of this folder to Classic. You'll use this folder to store the skin files for the Classic theme (the name of the folder is also the theme name). Note: The names of the folders under the App_Themes folder are also the theme names.
Add three .skin files to the Classic folder by right-clicking the Classic folder and selecting Add New Item.... Select Skin File and name the new files Button.skin, Calendar.skin, and Label.skin, respectively. A skin file contains formatting information used to change the look and feel of a control. The Solution Explorer should now look like Figure 8-2. Figure 8-2. Adding skin files to a theme 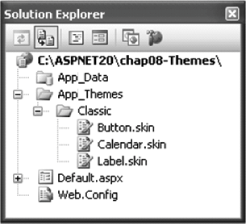
Populate the Button.skin file with the following line of code: <asp:Button runat="server" Width="90px" Font-Bold="True" /> Populate the Calendar.skin file as follows: <asp:Calendar runat="server" Width="400px" Font-Names="Times New Roman" Font-Size="10pt" Height="220px" BorderColor="Black" ForeColor="Black" BackColor="White" NextPrevFormat="FullMonth" TitleFormat="Month" DayNameFormat="FirstLetter"> <SelectedDayStyle ForeColor="White" BackColor="#CC3333" /> <SelectorStyle ForeColor="#333333" Font-Names="Verdana" Font-Size="8pt" Font-Bold="True" Width="1%" BackColor="#CCCCCC" /> <OtherMonthDayStyle ForeColor="#999999" /> <TodayDayStyle BackColor="#CCCC99" /> <DayStyle Width="14%" /> <NextPrevStyle ForeColor="White" Font-Size="8pt" /> <DayHeaderStyle ForeColor="#333333" Height="10pt" Font-Size="7pt" Font-Bold="True" BackColor="#CCCCCC" /> <TitleStyle ForeColor="White" Height="14pt" Font-Size="13pt" Font-Bold="True" BackColor="Black" /> </asp:Calendar> Populate the Label.skin file as follows: <asp:Label runat="server" Width="256px" Font-Bold="True" Font-Names="Arial Narrow" Font-Size="Medium" /> Tips for Creating Skin Files Since skin files are nothing more than just the Source View of controls (with the exception of attributes such as id), it is very easy to create skin files. One good way to do so is to give your controls the look and feel you want them to have on a normal Web Form in Design View. Then switch to Source View, copy the control element from the source code created for the control, and paste it into the skin file. Finally, remove the id attribute and add a skinid attribute in its place. |
For the default Web Form, switch to Source View and add in the following Theme attribute: <%@ Page Language="VB" AutoEventWireup="false" CodeFile="Default.aspx.vb" Inherits="Default_aspx" Theme="Classic" %> To test your application, press F5. You will notice that the controls on the page look different from their original design (see Figure 8-3). This is because the settings in the .skin files have been applied to the controls on the page. Figure 8-3. Page with theme applied 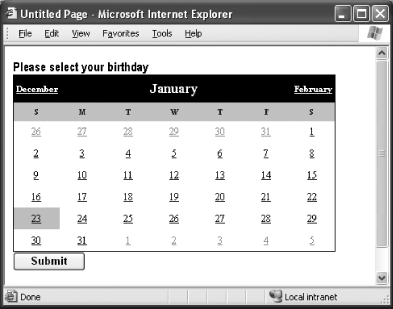
8.1.2. What just happened? You have just created three skin files and grouped them together as a single theme. Each skin file is used to define the look and feel of a particular control. Note: A theme contains one or more skins. A skin file defines the look and feel of a control. For example, the Button.skin file under the Classic theme defines the style of a Button control: <asp:Button runat="server" Width="90px" Font-Bold="True" /> Note: Remember, a skin definition cannot contain the id attribute. Including it will trigger a compile-time error. While the Button.skin file used in this lab contains only one Button definition, you can, if you wish, include multiple Button definitions in the same file, as shown in the following code snippet: <asp:Button runat="server" Width="90px" Font-Bold="True" /> <asp:Button skin runat="server" Width="90px" Font-Bold="True" Font-Italic="True" /> <asp:Button skin runat="server" Width="90px" Font-Bold="True" Font-Italic="True" Font-Underline="True" /> You identify each skin in a .skin file by the value of its skinid attribute. The first button style defined in a .skin file typically does not contain a skinid attribute and is regarded as the default skin for the Button control. If you examine the Button control's properties by displaying its Properties window, you will find a SkinID property. By default, the SkinID property is not set, but if a skin file that is part of a selected theme contains multiple definitions, you can select a particular definition from the list of skins that it displays (see Figure 8-4). If the SkinID property of a control is not set, the default skin will be applied during runtime. Figure 8-4. Specifying a SkinID for a control 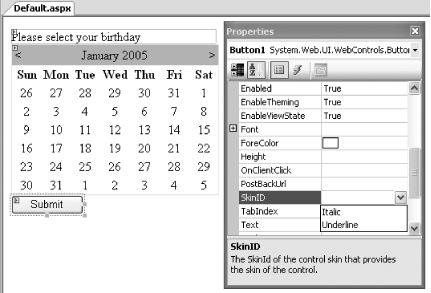 By specifying a theme to use for the page, the default skins in all the skin files will apply to all the relevant controls on the page, unless a particular skin is specified in the skinid attribute of each control. Tip: While you need not necessarily create multiple skin files for your project (in fact, a single skin file can contain all the style definitions for all controls), the recommended practice is that you hold the styles for each control in a separate skin file. A good practice is to name each skin file after the control that it defines. Disabling Skinning for Controls When a theme is applied to a page, all controls will be affected if there are styles defined in the various skin files. To prevent a control from being applied a theme, set the EnableTheming property of the control to False, like this: <asp:Button Runat="server" Text="Get Title" EnableTheming="False" /> |
8.1.3. What about... ...applying a theme programmatically? To programmatically change the theme of a page, you use the Theme property exposed by the Page class. However, you need to set the theme before the page is loaded (before the Page_Load event is fired). In fact, you have to set it in the PreInit event, which is fired before all the controls are loaded and initialized: Protected Sub Page_PreInit(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles Me.PreInit Page.Theme = "Classic" End Sub 8.1.4. Where can I learn more? Microsoft has a good quick-start guide to themes and skins at http://beta.asp.net/quickstart/aspnet/. |