Thus far in this lesson, we've dealt mostly with numbers. Now it's time to learn about the powerful methods of the String object, which you were introduced to in Lesson 4. Using String object methods, you can find specific characters in a string, change the case of the string, reverse the characters in the string, and more. The following are some of the most commonly used String object methods: length This String object property returns the number of characters in a string. Example: numCharacters = userName.length; Here the value of numCharacters based on the number of characters in the userName variable. If userName is "Jobe" the result is 4. substr(start, length) The substring method, which returns part of a string, accepts two parameters: starting index and the length (or number) of characters to count (including starting character). If the length parameter is omitted, this method will by default count until the end of the string. Example: name.substr(1, 2); If the value of name is "Kelly" the result would be "el". The letter e has an index of 1, and the number 2 tells the substring to include two letters. toLowerCase() This method forces all of the letters in a string to be lowercase. Example: message.toLowerCase(); If the value of message is "Hello" the result is "hello". toUpperCase() This method forces all of the letters in a string to be uppercase. Example: message.toUpperCase(); If the value of message is "Hello" the result is "HELLO". NOTE As mentioned in Lesson 4, the text property of a text field instance can also be considered an instance of the String object and thus can be manipulated as such. For example, myTextField.text.toUpperCase(); will cause all the text in the myTextField text field to appear in uppercase letters. In this exercise, you will create a simple silly word-game application. With it, you can enter words in a basic form and a paragraph will be generated. -
Open madlibs1.fla in the Lesson08/Assets folder. This file includes three layers: Background, Text Fields, and Actions. The Background layer contains the main site graphics. The Text Fields layer contains five input-text fields, a dynamic-display text field, and a Submit button. (We'll use the Actions layer in a moment.) The five input-text fields are (from the top down) verb1, properNoun, verb2, adjective, and noun. These will allow the user to enter words that will be used to generate the paragraph. The bigger text field, at the bottom-right of the stage, is named paragraph and will be used to display the paragraph. 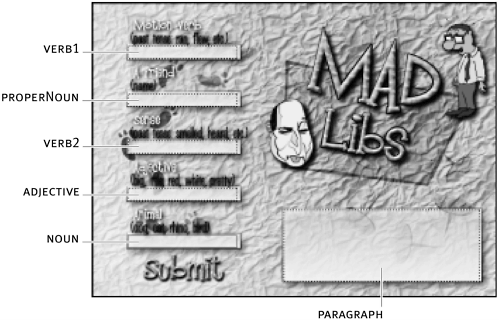 -
With the Actions panel open, select Frame 1 of the Actions layer and add the following script: function generate () { } This is the beginning of a function definition. This function will be called when the Submit button is pressed. The final function will take all of the user-input words and modify them when needed. A sentence of text will then be generated that includes these words. -
With Frame 1 still selected, add the following four lines of script to the generate() function definition: verb1.text = verb1.text.toLowerCase(); verb2.text = verb2.text.toLowerCase(); adjective.text = adjective.text.toLowerCase(); noun.text = noun.text.toLowerCase(); 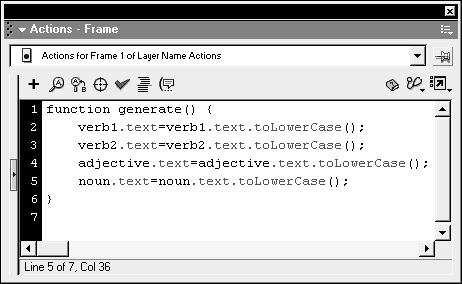 These four actions force the text entered into the corresponding text fields to be lowercase primarily for grammatical reasons (none of these fields will include proper nouns or represent the first word in the sentence). -
Add the following script to the end of the current generate() function, just below noun.text = noun.text.toLowerCase() : properNoun.text = properNoun.text.substr(0,1).toUpperCase() + properNoun.text.substr(1).toLowerCase(); In the above script, properNoun.text makes reference to the properNoun text field on the stage, which is used to enter a person's name. For grammatical reasons, the leading character in a person's name (that is, the character at index 0) should be capitalized and the rest of the letters lowercased. To ensure that this is the case, we use the substr() method in our expression. As you can see from the above, you can use two methods concurrently and in a contiguous fashion. First, properNoun.text.substr(0, 1) returns the first letter of the value of properNoun.text , which it is then forced to uppercase using the toUpperCase() method. The string concatenation operator (+) is then used to concatenate the first half of the expression with the second. The second half also uses the substr() method. The difference here is that it begins counting with the character at index 1 (the second letter). Because the length value (the second parameter of the substr() method) is not specified, it finds the rest of the characters in the string and uses the toLowerCase() method to make those letters lowercase. Thus, "kelly" would be changed to "Kelly" or "kElLy" to "Kelly." 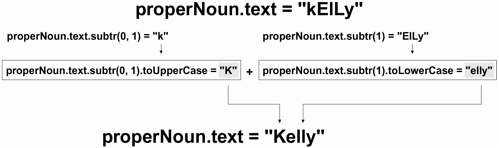 -
Add the following final line of script to this generate() function: paragraph.text = "You " + verb1.text + " into love with " + properNoun.text + " when you " + verb2.text + " " + properNoun.text + " eating a " + adjective.text + " " + noun.text + "."; This line of script uses the concatenation operator several times to insert various variable values in hard-coded text strings to build a complete sentence. TIP Be careful when concatenating several strings that each string section has opening and closing quotes; if any are missing, an error will result. The Actions panel cues you to errors in code by providing red highlighting above the Parameters setting area of the panel to indicate an error in that line's syntax.  -
With the Actions panel open, select the Submit button and add the following script: on (release, keyPress "<Enter>") { generate(); } When the button is released or the Enter key pressed, the generate() function you just created will be called. -
Choose Control > Test Movie. Enter text into each of the input-text boxes, then press the Submit button and read the sentence you created. Try it again, this time entering text with varying case. You can see how easy it is to manipulate strings with ActionScript. -
Close the test movie and save your work as madlibs2.fla. Now you know something about using methods of the String object an important aspect of learning ActionScript since text plays an important role of many projects and you need to know how to manipulate it. The exercise in this section introduced you to the basics; as you progress through the book, you'll find additional examples of ways to control text to suit your project's needs. |