In the previous exercise you learned how to enhance an existing method of the MovieClip object class. But what do you do if you need a method that hasn't been invented? You create your own! As with enhancing methods, there are two ways of creating new methods, depending on whether you're creating one for a built-in object (Mouse, Key, Math, Selection) or a class of objects (MovieClip, TextField, and so on). To create a new method for a built-in object (we'll use the Math object again), you would use the following syntax: Math.totalWithTax = function(total, taxPercent){ convertedPercent = taxPercent / 100; totalTax = total * convertedPercent; return total + totalTax; } trace(Math.totalWithTax(24.00, 6)); // Outputs 25.44 Here you can see that to add a method (like the totalWithTax() method) to the Math object, you simply attach a function to it as shown. This method is now available for use anywhere in your movie. You would use this same approach to attach new methods to other built-in objects. In the following exercise, we'll show you how to add a new method to a class of built-in objects (primarily the MovieClip object, though you can use the same approach to create new methods for any built-in object class). The method that we create will allow a movie clip instance to be flipped horizontally or vertically functionality ActionScript doesn't currently provide through a built-in method. -
Open objectProject3.fla in the Lesson06/Assets folder. Since we worked on this project in the previous exercise, you should be familiar with its elements. In this exercise, we'll place scripts on Frame 1 as well as on the double-headed arrow buttons on the top-left part of the screen. -
With the Actions panel open, select Frame 1 and add the following script at the end of the current script: MovieClip.prototype.flip = function(mode){ if (mode.toLowerCase() == "h"){ this._xscale = -this._xscale; }else if (mode.toLowerCase() == "v"){ this._yscale = -this._yscale; } } 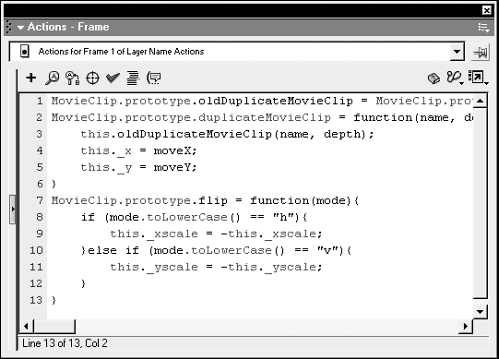 We've placed this script on Frame 1 so that it will execute as soon as the movie begins to play and the flip() method will be added to the MovieClip.prototype object and available for use by movie clip instances from that point forward. As you can see, this new method is nothing more than a function that accepts a single parameter named mode . This will be a value of either "h" or "v", indicating whether flipping should occur horizontally or vertically. The function uses an if statement to determine the value of mode , so that it knows how to react. The if part of the statement first converts the value of mode to a lowercase character then compares that value to "h". If that value is "h", the function knows to do a horizontal flip (which is what the line of script that follows does). It does this by reversing the current xscale value of the currently referenced movie clip instance (this ). Thus, if that value is currently 75, it will be set to -75; if it's 34, it will be set to 34. The function reverses these values by using the minus operator (-). When used as shown, that operator turns a negative value into a positive and vise-versa. Scaling a movie clip instance in this fashion gives the effect of flipping. The else part of the statement deals with what happens if mode has a value of "v", indicating a vertical flip. In this case, the yscale property of the currently referenced instance is reversed, which gives the effect of flipping it vertically. NOTE We converted the value of mode to a lowercase character to deal with a situation in which we might accidentally use "H" or "V" instead of "h" or "v". "H" does not equal "h"; thus, the conversion helps minimize errors that might occur when attempting to use the new method. -
With the Actions panel open, select the up-down arrow button under the smaller of the double-square buttons and attach this script: on(release){ smallHead.flip("v"); } When the button is released, the smallHead instance is flipped vertically. -
With the Actions panel still open, select the left-right arrow under the button you just added a script to and attach this script: on(release){ smallHead.flip("h"); } This will flip the smallHead instance horizontally. -
Attach the same actions discussed in the previous two steps to the up-down, and left-right buttons under the larger of the double-square buttons, replacing the smallHead reference in the script with bighead . -
Choose Control > Test Movie to test the project up to this point. Pressing any of these buttons will cause the appropriate movie clip instance to flip. 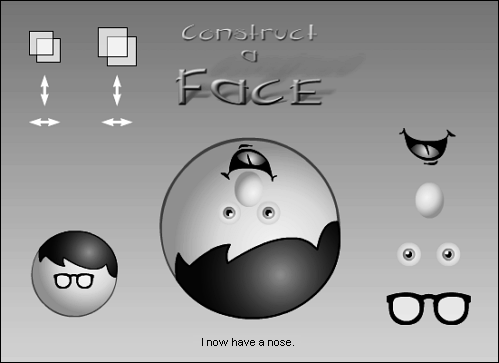 This is just a simple example of how you can add a custom method to a class of objects. You can create methods for other classes (for example, TextField, Sound, Color, XML, and so on) in similar fashion to that shown in Step 2. -
Close the test movie and save your work as objectProject4.fla. This completes this exercise. We will build on a slightly modified version of this project in the following exercise. TIP For a great resource for custom and enhanced ActionScript methods, visit http://www.layer51.com/proto/. TIP You can create a library of custom methods (or classes of objects for that matter) by writing the necessary code in a standard text editor then saving the file as something like customScript.as (an .as file is understood by Flash to contain Actionscript code). Then, if you needed to use any of these classes or custom methods, you would simply place an #include action on Frame 1 of your movie, indicating the file name of the .as file containing the script you would like to include (something like #include "customScript.as" ) . This action automatically imports and inserts the code in the .as file at the same point as the #include action (a transparent process). You can create as many different .as files as you want to organize your reusable custom code. |