18.2. Defining Form Elements HTML form elements allow you to create simple user interfaces for your JavaScript programs. Figure 18-1 shows a complex form that contains at least one of each of the basic form elements. In case you are not already familiar with HTML form elements, the figure includes a numbered key identifying each type of element. This section concludes with an example (Example 18-1) that shows the HTML and JavaScript code used to create the form pictured in Figure 18-1 and to hook up event handlers to each form element. Figure 18-1. HTML form elements 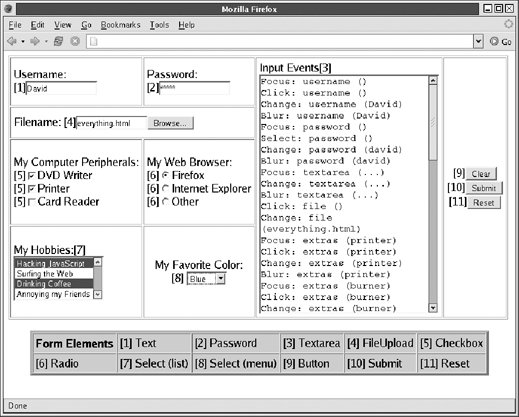 Table 18-1 lists the types of form elements that are available to HTML designers and JavaScript programmers. The first column of the table names the type of form element, the second column shows the HTML tags that define elements of that type, and the third column lists the value of the type property for each type of element. As shown earlier, each Form object has an elements[] array that contains the objects that represent the form's elements. Each element has a type property that can distinguish one type of element from another. By examining the type property of an unknown form element, JavaScript code can determine the type of the element and figure out what it can do with that element. Finally, the fourth column of the table provides a short description of each element and also lists the most important or most commonly used event handler for that element type. Table 18-1. HTML form elementsObject | HTML tag | type property | Description and events |
---|
Button | <input type="button"> or <button type="button"> | "button" | A push button; onclick. | Checkbox | <input type="checkbox"> | "checkbox" | A toggle button without radio-button behavior; onclick. | File | <input type="file"> | "file" | An input field for entering the name of a file to upload to the web server; onchange. | Hidden | <input type="hidden"> | "hidden" | Data submitted with the form but not visible to the user; no event handlers. | Option | <option> | none | A single item within a Select object; event handlers are on the Select object, not on individual Option objects. | Password | <input type="password"> | "password" | An input field for password entrytyped characters are not visible; onchange. | Radio | <input type="radio"> | "radio" | A toggle button with radio-button behavioronly one selected at a time; onclick. | Reset | <input type="reset"> or <button type="reset"> | "reset" | A push button that resets a form; onclick. | Select | <select> | "select-one" | A list or drop-down menu from which one item may be selected; onchange. (See also Option object.) | Select | <select multiple> | "select-multiple" | A list from which multiple items may be selected; onchange. (See also Option object.) | Submit | <input type="submit"> or <button type="submit"> | "submit" | A push button that submits a form; onclick. | Text | <input type="text"> | "text" | A single-line text entry field; onchange. | Textarea | <textarea> | "textarea" | A multiline text entry field; onchange. |
Note that the names Button, Checkbox, and so on from the first column of Table 18-1 may not correspond to an actual client-side JavaScript object. Complete details about the various types of elements are available in Part IV, under the entries Input, Option, Select, and Textarea. These form elements are discussed in more depth later in this chapter. Now that you've taken a look at the various types of form elements and the HTML tags used to create them, Example 18-1 shows the HTML code used to create the form shown in Figure 18-1. Although the example consists primarily of HTML, it also contains JavaScript code that defines event handlers for each form element. You'll notice that the event handlers are not defined as HTML attributes. Instead, they are JavaScript functions assigned to the properties of the objects in the form's elements[] array. The event handlers all call the function report(), which contains code that works with the various form elements. The next section of this chapter explains everything you need to know to understand what the report() function is doing. Example 18-1. An HTML form containing all form elements <form name="everything"> <!-- A one-of-everything HTML form... --> <table border="border" cellpadding="5"> <!-- in a big HTML table --> <tr> <td>Username:<br>[1]<input type="text" name="username" size="15"></td> <td>Password:<br>[2]<input type="password" name="password" size="15"></td> <td rowspan="4">Input Events[3]<br> <textarea name="textarea" rows="20" cols="28"></textarea></td> <td rowspan="4" align="center" valign="center"> [9]<input type="button" value="Clear" name="clearbutton"><br> [10]<input type="submit" name="submitbutton" value="Submit"><br> [11]<input type="reset" name="resetbutton" value="Reset"></td></tr> <tr> <td colspan="2"> Filename: [4]<input type="file" name="file" size="15"></td></tr> <tr> <td>My Computer Peripherals:<br> [5]<input type="checkbox" name="extras" value="burner">DVD Writer<br> [5]<input type="checkbox" name="extras" value="printer">Printer<br> [5]<input type="checkbox" name="extras" value="card">Card Reader</td> <td>My Web Browser:<br> [6]<input type="radio" name="browser" value="ff">Firefox<br> [6]<input type="radio" name="browser" value="ie">Internet Explorer<br> [6]<input type="radio" name="browser" value="other">Other</td></tr> <tr> <td>My Hobbies:[7]<br> <select multiple="multiple" name="hobbies" size="4"> <option value="programming">Hacking JavaScript <option value="surfing">Surfing the Web <option value="caffeine">Drinking Coffee <option value="annoying">Annoying my Friends </select></td> <td align="center" valign="center">My Favorite Color:<br>[8] <select name="color"> <option value="red">Red <option value="green">Green <option value="blue">Blue <option value="white">White <option value="violet">Violet <option value="peach">Peach </select></td></tr> </table> </form> <div align="center"> <!-- Another tablethe key to the one above --> <table border="4" bgcolor="pink" cellspacing="1" cellpadding="4"> <tr> <td align="center"><b>Form Elements</b></td> <td>[1] Text</td> <td>[2] Password</td> <td>[3] Textarea</td> <td>[4] FileU</td> <td>[5] Checkbox</td></tr> <tr> <td>[6] Radio</td> <td>[7] Select (list)</td> <td>[8] Select (menu)</td> <td>[9] Button</td> <td>[10] Submit</td> <td>[11] Reset</td></tr> </table> </div> <script> // This generic function appends details of an event to the big Textarea // element in the form above. It is called from various event handlers. function report(element, event) { if ((element.type == "select-one") || (element.type == "select-multiple")){ value = " "; for(var i = 0; i < element.options.length; i++) if (element.options[i].selected) value += element.options[i].value + " "; } else if (element.type == "textarea") value = "..."; else value = element.value; var msg = event + ": " + element.name + ' (' + value + ')\n'; var t = element.form.textarea; t.value = t.value + msg; } // This function adds a bunch of event handlers to every element in a form. // It doesn't bother checking to see if the element supports the event handler, // it just adds them all. Note that the event handlers call report(). // We're defining event handlers by assigning functions to the // properties of JavaScript objects rather than by assigning strings to // the attributes of HTML elements. function addhandlers(f) { // Loop through all the elements in the form. for(var i = 0; i < f.elements.length; i++) { var e = f.elements[i]; e.onclick = function( ) { report(this, 'Click'); } e.onchange = function( ) { report(this, 'Change'); } e.onfocus = function( ) { report(this, 'Focus'); } e.onblur = function( ) { report(this, 'Blur'); } e.onselect = function( ) { report(this, 'Select'); } } // Define some special-case event handlers for the three buttons. f.clearbutton.onclick = function( ) { this.form.textarea.value=''; report(this,'Click'); } f.submitbutton.onclick = function ( ) { report(this, 'Click'); return false; } f.resetbutton.onclick = function( ) { this.form.reset( ); report(this, 'Click'); return false; } } // Finally, activate our form by adding all possible event handlers! addhandlers(document.everything); </script> | |