The include Action The include action resembles the include directive superficially in that both of them cause information from an external source to be included into the current JSP page. They differ in other aspects of their processing in the following ways: The include action inserts the contents of the other file into the JSP at runtime. This information is reloaded every time the page is run. The include directive only inserts the information at translation time. The include action can dynamically insert the name of the page to include. This enables the actual page to be selected based on user input. The include action can accept parameters. The include directive is a textual substitution that can reference variables local to the page. The include action transfers control to the other JSP or servlet that does not have access to the local variables of the calling JSP. Because the processing done to support the include action is so much more complex than that done to support the include directive, the performance of the include directive is superior. If you have a situation where only static HTML or JSP is to be included, you should use the directive. If, however, you need the features mentioned previously in the bulleted list, you must use the include action. You can think of the include action as a type of subroutine call. Your program (JSP) runs to a certain point. It then transfers control to another JSP that runs to completion. Upon completion, control is passed back to the calling program (JSP), which resumes processing at the line following the call (the include line). The syntax of the include action is as follows: <jsp:include page='xyz.jsp' flush="true" /> The page is the name of the page relative to the location of the calling JSP. The normal double quotes can be used instead of the single quotes, if you prefer. The flush attribute is required, although it must always be set to true. This indicates the programmer's desire to flush the buffer prior to transferring control to the included program. Listing 23.6 shows how the include action can be used to include another JSP at runtime. Listing 23.6 The IncludeAction.jsp File <html> <head> <title>Include Action Test</title> <body> <h2>Include Action Test</h2> This is before the included page. <jsp:include page='includes/IncludedPage1.jsp' /> This is after the included page. <br> </body> </html> Notice that the include action uses the page attribute to designate the page to transfer control to temporarily. The page's location is calculated relative to the location of the calling page. The result of running this program is shown in Figure 23.4. Figure 23.4. The JSP include action transfers control to another JSP or servlet, and then returns control after it completes. 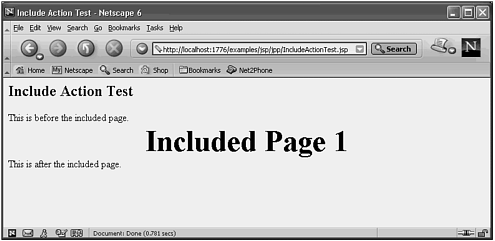 Listing 23.4 showed how the include action can be used to insert the contents of a static JSP file into another. The result was correct, but unexciting because you could have accomplished the same thing using the include directive. Listing 23.7 shows a more sophisticated use of the include action where user input is used to determine which JSP to include. Listing 23.7 The IncludeActionTest2.jsp File <html> <head> <title>Include Action Test2</title> <body> <h2>Include Action Test2</h2> This is before the included page. <% String pageNum; pageNum = request.getParameter("pageNum"); %> <jsp:include page='<%= "includes/IncludedPage" + pageNum +".jsp" %>' /> This is after the included page. <br> </body> </html> In this example, the page number to be included is retrieved by a call to the request object. pageNum = request.getParameter("pageNum"); The page attribute of the include action is created dynamically using a JSP expression. <jsp:include page='<%= "includes/IncludedPage" + pageNum +".jsp" %>' /> The HTML file that displays the form is shown in Listing 23.8. Listing 23.8 The PageNum.html File <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <HTML> <HEAD> <TITLE>Passing Parameters to JSPs</TITLE> </HEAD> <H1 align="CENTER"> Enter the Page that you want to see </H1> <FORM action='/examples/jsp/jpp/IncludeActionTest2.jsp'> Page Number: <INPUT TYPE="TEXT" NAME="pageNum"><BR><BR> <CENTER> <INPUT TYPE="SUBMIT"> </CENTER> </FORM> </BODY> </HTML> The parameter named pageNum is the one that is accessed via the request object. The JSP pages to be displayed are very simple. Listing 23.9 shows one of them. The others are nearly identical, so only one of them needs to be shown. Listing 23.9 The IncludedPage3.jsp File <center> <font size=10> <b> Included Page 3 </b> </font> </center> This file is kept simple to make the use of the include easy to understand. Your included files can be of arbitrary complexity, which may take several forms, and can contain their own include actions. Listing 23.10 shows an example that has a number of additional features beyond simple insertion of static HTML. Listing 23.10 The IncludeActionTest3.jsp File <html> <head> <title>Include Action Test3</title> <body> <h2>Include Action Test3</h2> This is before the included page. <% String newPageNum; newPageNum = request.getParameter("pageNum"); %> <jsp:include page="IncludeActionTest4.jsp" flush="true" > <jsp:param name ="forwardedPageNum" value="<%= newPageNum %>" /> </jsp:include> This is after the included page. <br> </body> </html> The purpose of this JSP is to show the passing of the parameter from a form to an included JSP. The first step in doing this is to retrieve the pageNum variable from the request object and store it in a local variable. <% String newPageNum; newPageNum = request.getParameter("pageNum"); %> The next step is to add the jsp:param action to the jsp:include tag. <jsp:include page="IncludeActionTest4.jsp" flush="true" > <jsp:param name ="forwardedPageNum" value="<%= newPageNum %>" /> </jsp:include> Notice that the nature of the closing tag </jsp:include> is different from the /> that we used when there were no params being passed. The /> ending doesn't work when you have nested tags. The "flush" attribute indicates that the output buffer should be flushed before the include is executed. As of JSP 1.1, this value must always be "true." The HTML that calls this JSP is included here in Listing 23.11 to show the complete example. Listing 23.11 The PageNum3.HTML File <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <HTML> <HEAD> <TITLE>Passing Parameters to JSPs</TITLE> </HEAD> <H1 align="CENTER"> Enter the Page that you want to see </H1> <FORM action='/examples/jsp/jpp/IncludeActionTest3.jsp'> Page Number: <INPUT TYPE="TEXT" NAME="pageNum"><BR><BR> <CENTER> <INPUT TYPE="SUBMIT"> </CENTER> </FORM> </BODY> </HTML> Note that the original parameter name is "pageNum". The IncludeActionTest4.jsp file is the most interesting part of this example. It is shown here in Listing 23.12. Listing 23.12 The IncludeActionTest4.jsp File <center> <font size=10> <b> IncludeActionTest4 <br> <% String fpn = request.getParameter("forwardedPageNum"); if (fpn.equals("1")) { %> <jsp:include page='includes/IncludedPage1.jsp' /> <% } if (fpn.equals("2")) { %> <jsp:include page='includes/IncludedPage2.jsp' /> <% } if (fpn.equals("3")) { %> <jsp:include page='includes/IncludedPage3.jsp' /> <% } %> The pageNum parameter = <%= request.getParameter("pageNum") %> </b> </font> </center> The parameter forwardedPageNum was declared in IncludedActionTest3 and passed as a parameter. This program gains access to it by using the same request object as always. String fpn = request.getParameter("forwardedPageNum"); The local variable fpn now contains a string value that can be tested and branched off of. if (fpn.equals("1")) { %> <jsp:include page='includes/IncludedPage1.jsp' /> <% Notice that we had to terminate the scriptlet and start another one after the include action line. Notice also that the fact that this file was included by IncludeActionTest3.jsp does not prevent it from doing its own jsp:include actions. The included files are the same ones that we showed in Listing 23.9. The fact that we have added a parameter to the request object doesn't interfere with the other fields that it already contains. The pageNum parameter = <%= request.getParameter("pageNum") %> The pageNum parameter is the one that was declared in the HTML form shown earlier in Listing 23.11. It continues to exist as we move down into the included files. The result of running this is shown here in Figure 23.5. Figure 23.5. The JSP include action can make up parameters and pass them to the included page. 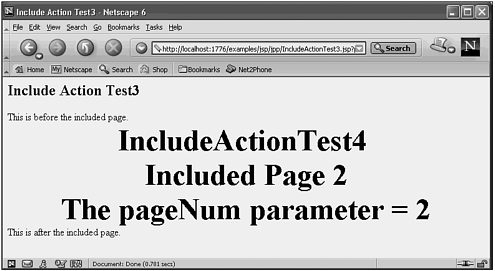 This figure shows output from three different JSPs. The IncludeAction3.jsp generated the Include Action Test 3 line, as well as the before and after lines. IncludeAction4.jsp generated the IncludedActionTest4 line as well as the The pageNum parameter = 2 line. The line that reads Included Page 2 was generated by the IncludedPage2.jsp file. Including other files with or without parameter passing is a powerful capability. In the next section you will learn another way to use components that don't involve the include action at all. |