The most powerful way to use component programming in JSPs is to call JavaBeans from within them. JavaBeans are normal Java classes that can be created using any editing tools. They don't extend any special classes or implement any particular interfaces. What makes JavaBeans different from ordinary Java classes is that they are designed according to a specific pattern. Normally, JavaBeans are associated with Graphical User Interfaces (GUIs). If the JavaBean pattern is followed, visual tools such as Forte, JBuilder, and Visual Cafe can place these Beans on the toolbar and allow users to drag them into their applications. On the server side, the requirements are not as strict because these JavaBeans don't have a GUI representation. The rules for creating server-side JavaBeans are fairly simple: A JavaBean must have an empty constructor, which is a constructor that takes no arguments. It might have other constructors as well, but one constructor must accept no arguments. If no constructors are declared, the empty constructor that is automatically generated by the JVM satisfies this requirement. A JavaBean can have no variables declared as public. You probably follow this rule already because the declaration of public variables in a class definition is considered bad form. A JavaBean may have properties. These properties are private variables that are accessed via get and set methods. If a variable has only a get, but not a set method associated with it, it is considered a read-only property. If a variable has only a set method, it would be a write-only property. If it has neither, it is not a property at all, but only a private variable of the class. Using JavaBeans in your JSPs has a number of advantages over using include files. The primary advantage comes from the division of labor that is possible using JSPs and JavaBeans in combination. JavaBean development is virtually identical to ordinary Java class development. JavaBeans can extend any superclass and implement any interface that you want it to. This means that a Bean can be multithreaded, it can access a database via JDBC, it can use the Java Cryptography Extension (JCE), and any other standard extension that you know how to program. Programmers who are capable of programming with all these extensions are expensive and hard to find and hire. To make the best use of their time, it is wise to give them the responsibility of developing the JavaBeans that contain the nondisplay-oriented logic of your application. JSP developers are often more junior and, in some cases, are not programmers at all. Many JSP developers are Web page designers who have migrated to JSP to gain more power over their content. They describe their needs to the JavaBean developers who provide them with the Beans that they need as well as instructions on how to use them. Creating JavaBeans and calling them from within a JSP is fairly simple. The complexity of the task comes from the challenge of trying to program a Bean to solve your business problems, not from the structure of the Bean itself. Listing 23.14 shows a simple server-side JavaBean. Listing 23.14 The TicketRequestBean Class /* * TicketRequestBean.java * * Created on July 9, 2002, 11:22 AM */ package com.samspublishing.jpp.ch23; import java.sql.*; import java.util.*; /** * * @author Stephen Potts * @version */ public class TicketRequestBean implements java.io.Serializable { //information about the customer private int custID; private String lastName; private String firstName; public TicketRequestBean() { } /** Constructor */ public TicketRequestBean(int custID, String lastName, String firstName, int cruiseID, String destination, String port, String sailing, int numberOfTickets, boolean isCommissionable) { //set the information about the customer this.custID = custID; this.lastName = lastName; this.firstName = firstName; } public int getCustID() { return this.custID = custID; } public String getLastName() { return this.lastName = lastName; } public String getFirstName() { return this.firstName = firstName; } public void setCustID(int custID) { this.custID = custID; } public void setLastName(String lastName) { this.lastName = lastName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String toString() { String outString; outString = "-------------------------------------------" + "\n"; //information about the customer outString += "custID = " + this.custID + "\n"; outString += "lastName = " + this.lastName + "\n"; outString += "firstName = " + this.firstName + "\n"; outString += "-------------------------------------------" + "\n"; return outString; } } This class contains the usual complement of getters and setters, as well as the required empty constructor. In addition, it contains a nonempty constructor and a toString() method to dump the contents for debugging purposes. This class will give you some fields to learn with. Conceptually, this Bean is very simple. The definition of the fields is shown here: custID The customer's ID in our system. lastName The last name of the customer. firstName The first name of the customer. Making calls to this JavaBean from within a JSP is fairly simple. A special action, called jsp:useBean, provides us with a way of instantiating a JavaBean inside our JSP. The syntax of the useBean action is shown here: <jsp:useBean scope="page" /> The id is the name of this instance in your JSP and it is used to reference the bean within the page. The class is the full name of the class, including the package. The scope specifies what pages will be able to access this instance. (Page, the default, specifies that only this page will see this instance.) This JavaBean can be populated by a JSP. Listing 23.15 shows the form that we will use to do this. Listing 23.15 The AddCustomertForm.html File <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <HTML> <HEAD> <TITLE>Add a Customer</TITLE> </HEAD> <H1 align="CENTER"> Enter the new customer's information </H1> <FORM action='/examples/jsp/jpp/TicketRequestProcessor.jsp'> Customer ID: <INPUT TYPE="TEXT" NAME="custID"><BR><BR> Customer Last Name: <INPUT TYPE="TEXT" NAME="lastName"><BR><BR> Customer First Name: <INPUT TYPE="TEXT" NAME="firstName"><BR><BR> <CENTER> <INPUT TYPE="SUBMIT"> </CENTER> </FORM> </BODY> </HTML> There is nothing special about this form. It gives the user a place to enter information about the customer, and then calls the TicketRequestProcessor.jsp page when the SUBMIT button is clicked. <FORM action='/examples/jsp/jpp/TicketRequestProcessor.jsp'> The TicketRequestProcessor.jsp file is shown in Listing 23.16. Listing 23.16 The TicketRequestProcessor.jsp File <jsp:useBean scope="page" /> <html> <head> <title>Ticket Request Processor</title> <body> <h2>Ticket Request Processor</h2> <br> Customer ID: <%= request.getParameter("custID") %> <br> Customer First Name: <%= request.getParameter("firstName") %> <br> Customer Last Name: <%= request.getParameter("lastName") %> <br> <jsp:setProperty name="trb" property="firstName" value='<%= request.getParameter("firstName") %>' /> <jsp:setProperty name="trb" property="lastName" value='<%= request.getParameter("lastName") %>' /> Now get the property to see if it is there <br> <jsp:getProperty name="trb" property="firstName"/> <br> <jsp:getProperty name="trb" property="lastName"/> </body> </html> It is customary to place the jsp:usebean tag first in a JSP. The id field is the name that this Bean will be known by in this scope. The class name is the full name of the class, complete with the package. The scope tells the translator whether this id will exist outside of this page. The scope="page" parameter indicates that the scope is limited to this page. <jsp:useBean scope="page" /> First, we display the values in the request using JSP expressions. Then, we set the name properties in the JavaBean using the jsp:setProperty action. <jsp:setProperty name="trb" property="firstName" value='<%= request.getParameter("firstName") %>' /> <jsp:setProperty name="trb" property="lastName" value='<%= request.getParameter("lastName") %>' /> Following that we use the jsp:getProperty action to retrieve the values from the JavaBean for display. We do this to prove that the setProperty action worked. <jsp:getProperty name="trb" property="firstName"/> <br> <jsp:getProperty name="trb" property="lastName"/> The only tricky part of this example is the location of the JavaBean's class file. Because this application is part of the examples application, Apache Tomcat requires that the file be placed in the ...\webapps\examples\WEB-INF\classes directory. However, because this class is part of a package, according to the rules of Java it must be placed in the directory that matches the package name. Therefore, in this example, the .class file must be placed in a directory named ...\webapps\examples\WEB-INF\classes\com\samspublishing\jpp\ch23 To run this example, place the JavaBean's .class file in this directory. Place the TicketRequestProcessor.jsp file in the ...\webapps\examples\jsp\jpp directory and the AddCustomerForm.html file in the ...\webapps\examples directory. Type the following URL in the address bar of your browser: http://localhost:1776/examples/AddCustomerForm.html The result that you see should resemble Figure 23.6: Figure 23.6. The JSP useBean action gives a JSP page access to Java code stored in a JavaBean. 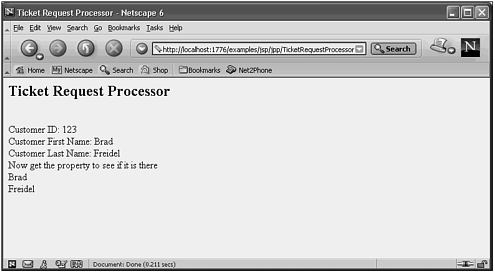 Notice that we avoided processing the custID field in the previous example. We separated out this field because the type of the custID in the JavaBean is not String, but int. This complicates things because we will get a type-mismatch message if we try to store the string into an integer field. To solve this problem, we need to do a conversion on the numeric field before performing the setProperty action. Listing 23.17 shows the HTML code for an example that performs this conversion. Listing 23.17 The AddCustomerForm2.html File <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <HTML> <HEAD> <TITLE>Add a Customer 2</TITLE> </HEAD> <H1 align="CENTER"> Enter the new customer's information </H1> <FORM action='/examples/jsp/jpp/TicketRequestProcessor2.jsp'> Customer ID: <INPUT TYPE="TEXT" NAME="custID"><BR><BR> Customer Last Name: <INPUT TYPE="TEXT" NAME="lastName"><BR><BR> Customer First Name: <INPUT TYPE="TEXT" NAME="firstName"><BR><BR> <CENTER> <INPUT TYPE="SUBMIT"> </CENTER> </FORM> </BODY> </HTML> The only difference in this file is the name of the JSP page. <FORM action='/examples/jsp/jpp/TicketRequestProcessor2.jsp'> Listing 23.18 shows the version of the JSP that does the conversion. Listing 23.18 The TicketRequestProcessor2.jsp File <jsp:useBean scope="session" /> <html> <head> <title>Ticket Request Processor 2</title> <body> <h2>Ticket Request Processor 2</h2> <br> Customer ID: <%= request.getParameter("custID") %> <br> Customer First Name: <%= request.getParameter("firstName") %> <br> Customer Last Name: <%= request.getParameter("lastName") %> <br> <% String custIDString = request.getParameter("custID"); int custIDValue = Integer.parseInt(custIDString); %> <jsp:setProperty name="trb" property="custID" value='<%= custIDValue %>' /> <jsp:setProperty name="trb" property="firstName" value='<%= request.getParameter("firstName") %>' /> <jsp:setProperty name="trb" property="lastName" value='<%= request.getParameter("lastName") %>' /> Now get the property to see if it is there <br> <jsp:getProperty name="trb" property="custID"/> <br> <jsp:getProperty name="trb" property="firstName"/> <br> <jsp:getProperty name="trb" property="lastName"/> </body> </html> The parameter custID is passed in as a String, so we need to assign it to a String in the scriptlet. String custIDString = request.getParameter("custID"); Before we can store the custID value in a JavaBean, we must convert it to an int using the parseInt() static method from the java.lang.Integer class. int custIDValue = Integer.parseInt(custIDString); Now we can set the property using the custIDValue. <jsp:setProperty name="trb" property="custID" value='<%= custIDValue %>' /> The result of running this is shown in Figure 23.7. Figure 23.7. A conversion must take place before you can store a String value in a JavaBean using the jsp:setProperty action. 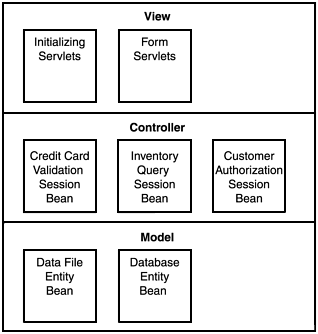 This conversion is a bit cumbersome, especially for nonprogrammers who are learning JSP. Fortunately, JSP provides an automatic way to associate a parameter with a property in a JavaBean. The param argument of the setProperty action will perform this association automatically, along with any conversion that may be needed. The syntax for this is shown here: <jsp:setProperty name="trb" property="custID" param="custID" /> Using this new syntax, we can simplify the TicketRequestProcessor2.jsp file as shown here in Listing 23.19. Listing 23.19 The TicketRequestProcessor3.jsp File <jsp:useBean scope="session" /> <html> <head> <title>Ticket Request Processor 3</title> <body> <h2>Ticket Request Processor 3</h2> <br> Customer ID: <%= request.getParameter("custID") %> <br> Customer First Name: <%= request.getParameter("firstName") %> <br> Customer Last Name: <%= request.getParameter("lastName") %> <br> <jsp:setProperty name="trb" property="custID" param="custID" /> <jsp:setProperty name="trb" property="firstName" param="firstName" /> <jsp:setProperty name="trb" property="lastName" param="lastName" /> Now get the property to see if it is there <br> <jsp:getProperty name="trb" property="custID"/> <br> <jsp:getProperty name="trb" property="firstName"/> <br> <jsp:getProperty name="trb" property="lastName"/> </body> </html> This JSP is identical to TicketRequestProcessor, except for the method of associating a value with a property. <jsp:setProperty name="trb" property="custID" param="custID" /> <jsp:setProperty name="trb" property="firstName" param="firstName" /> <jsp:setProperty name="trb" property="lastName" param="lastName" /> In this JSP, the parameter names are passed in directly to the setProperty actions, and the type conversions are done automatically. |