The fact that the HTTP protocol is stateless is one of its finest features. The simplicity of this approach makes it an ideal tool for delivering content quickly. This statelessness is also a hindrance when you need to perform a task that has to span several Web pages. It would be nice if you could identify yourself to the server so that it could pick up where it left off in the previous transaction, instead of having to start over every time. The browser writers addressed this issue first when they introduced the idea of cookies. Cookies are small files that live on the client's hard drive, which identify the user to a specific Web application. The server sends the cookie to the browser in response to the initial request. Subsequent requests send the same cookie back to the server, which enables the server to identify these requests as being from the same requester. Java supports the creation and manipulation of cookies via the javax.servlet.http.Cookie class. About a dozen methods are defined by this class, but the constructor, setValue() and getValue(), are the most commonly used. Listing 21.14 shows how cookies are used to store the state of an application. Listing 21.14 The SpecialDiet.java Class /* * SpecialDiet.java * * Created on June 25, 2002, 1:27 PM */ import javax.servlet.*; import javax.servlet.http.*; import java.util.*; import java.io.*; /** * * @author Stephen Potts * @version */ public class SpecialDiet extends HttpServlet { /** Initializes the servlet. */ public void init(ServletConfig config) throws ServletException { super.init(config); } /** Destroys the servlet. */ public void destroy() { } /** Handles the HTTP <code>GET</code> method. * @param request servlet request * @param response servlet response */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { } /** Handles the HTTP <code>POST</code> method. * @param request servlet request * @param response servlet response */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { response.setContentType("text/html"); Cookie myCookie = new Cookie("null", "null"); Enumeration keys; String key, value; keys = request.getParameterNames(); while (keys.hasMoreElements()) { key = (String)keys.nextElement(); value = request.getParameter(key); if(!key.equals("btnSubmit")) { System.out.println("value= " + value + " key= " + key); myCookie = new Cookie(value,key); response.addCookie(myCookie); } } response.sendRedirect( "http://localhost:1776/examples/servlet/SpecialDiet2"); } /** Returns a short description of the servlet. */ public String getServletInfo() { return "Short description"; } } This is a typical servlet until you get into the doPost() method. A Cookie object is declared and used to store several cookies. Cookie myCookie = new Cookie("null", "null"); We step through each parameter and create a cookie that stores the current value. Enumeration keys; String key, value; keys = request.getParameterNames(); while (keys.hasMoreElements()) { key = (String)keys.nextElement(); We get the parameters from the request object. value = request.getParameter(key); The only key that we don't want to create a cookie for is the OK button. if(!key.equals("btnSubmit")) { System.out.println("value= " + value + " key= " + key); We store the value and the key in the cookie and add it to the response object. myCookie = new Cookie(value,key); response.addCookie(myCookie); Finally, we call the sendRedirect() method to another servlet that displays all the cookies. response.sendRedirect( "http://localhost:1776/examples/servlet/SpecialDiet2"); This line causes a different servlet, SpecialDiet2, to be called. Because we don't specify a method, doGet() will be called. Listing 21.15 shows this program. Listing 21.15 The SpecialDiet2 Class /* * SpecialDiet2.java * * Created on June 25, 2002, 1:27 PM */ import javax.servlet.*; import javax.servlet.http.*; import java.util.*; import java.io.*; /** * * @author Stephen Potts * @version */ public class SpecialDiet2 extends HttpServlet { /** Initializes the servlet. */ public void init(ServletConfig config) throws ServletException { super.init(config); } /** Destroys the servlet. */ public void destroy() { } /** Handles the HTTP <code>GET</code> method. * @param request servlet request * @param response servlet response */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { response.setContentType("text/html"); PrintWriter out = response.getWriter(); Cookie cookies[]; cookies = request.getCookies(); System.out.println("Creating the SpecialDiet HTML"); // output your page here out.println("<html>"); out.println("<head>"); out.println("<title>" + "Your Diet Choices" + "</title>"); out.println("</head>"); out.println("<body BGCOLOR=\"#FDF5E6\"\n>"); out.println("<h1 ALIGN=CENTER>"); out.println("Here are your choices"); out.println("</h1>"); for (int i=0; i< cookies.length; i++) { System.out.println(cookies[i].getName()+ " " + cookies[i].getValue() + "<BR>"); out.println(cookies[i].getName()+ " " + cookies[i].getValue() + "<BR>"); out.println(" "); } out.println("</body>"); out.println("</html>"); out.close(); } /** Handles the HTTP <code>POST</code> method. * @param request servlet request * @param response servlet response */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { } /** Returns a short description of the servlet. */ public String getServletInfo() { return "Short description"; } } This servlet is ordinary also, except for the cookie-handling code. We declared an array of Cookie objects called cookies, and then called the HttpServletRequest class's getCookies() method. Cookie cookies[]; cookies = request.getCookies(); In the midst of creating the HTML code, we loop through and print every cookie that was sent. for (int i=0; i< cookies.length; i++) { System.out.println(cookies[i].getName()+ " " + cookies[i].getValue() + "<BR>"); out.println(cookies[i].getName()+ " " + cookies[i].getValue() + "<BR>"); out.println(" "); } The HTML that is used to gather the user input is shown in Listing 21.16. Listing 21.16 The ChooseDiet.HTML File <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <HTML> <HEAD> <TITLE>Choose Your Dietary Preference</TITLE> </HEAD> <BODY BGCOLOR="#FDF5E6"> <H1 align="CENTER"> Do you have special dietary needs?: </H1> <FORM action='/examples/servlet/SpecialDiet' method='post'> <TABLE cellspacing='5' cellpadding='5'> <TR> <TD align='center'><B>Choose one or more</B></TD> <TD align='center'><B></B></TD> <TD align='center'><B></B></TD> </TR> <TR> <TD align='center'><INPUT TYPE='Checkbox' NAME="losodium" VALUE="loso117"></TD> <TD align='center'>Delicious Low-Sodium Pizza</TD> </TR> <TR> <TD align='center'><INPUT TYPE='Checkbox' NAME="lofat" VALUE="lofat118"></TD> <TD align='center'>Delicious Lo-Fat Chicken</TD> </TR> <TR> <TD align='center'><INPUT TYPE='Checkbox' NAME="vegan" VALUE="vegan119"></TD> <TD align='center'>Delicious Vegetarian Lasagna</TD> </TR> </TABLE> <HR><BR> <CENTER> <INPUT type='SUBMIT' name='btnSubmit' value='OK'> <BR><BR> <A href='/examples/servlet/SpecialDiet2'>View Current Choices</A> </CENTER> </FORM> </BODY> </HTML> Notice that the method on the Form is Post. This is so that doPost() will be called on the servlet. <FORM action='/examples/servlet/SpecialDiet' method='post'> We also included a link to the output servlet from this page: <A href='/examples/servlet/SpecialDiet2'>View Current Choices</A> The result of running the ChooseDiet.html is shown here in Figure 21.11. Figure 21.11. The ChooseDiet.html form uses check boxes to gather user input. 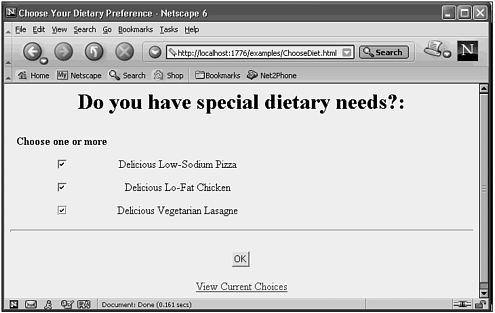 The SpecialDiet servlet has no visible result. It calls the SpeciaDiet2 servlet, which displays the values and the keys that were stored in the cookies. Cookies provide a simple way to store small amounts of data using the browser. You can see how this approach might become unmanageable if the amount of state data were to become large. The Session object uses cookies to provide a more elegant solution for larger amounts of data. Figure 21.12. The SpecialDiet2 servlet displays the contents of the cookies. 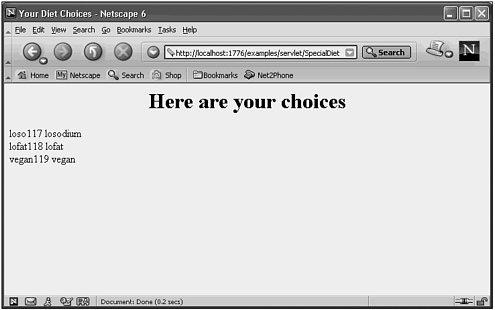 |