As with any other field of human endeavor, Web applications come with a lexicon of jargon. The rest of this chapter is devoted to explaining some of this jargon, as well as putting it into the context of the three-tiered architecture particularly the middleware and data layer portions. Server-Side Scripting If you've done any JavaScript coding, you understand client-side scripting. Client-side scripting is handled by a visitor's Web browser and can be different depending on the browser they're using. You know that, in some cases, you have to put in certain checks to make sure your code will work in Internet Explorer and Netscape Navigator. Server-side scripting happens on the server. When a visitor requests a dynamic page from a Web server, the server processes the page. If it finds any special processing instructions (such as including a certain file, checking for a certain variable, or querying a database) the server completes its processing and then displays the results back to the visitor's browser. In addition to being handled by the server, server-side scripting languages generally have more features and capabilities. For example, with a server-side scripting language such as JSP or PHP, you can extract files from and write files to the server's file system, run more sophisticated encryption programs (for e-commerce security), and complete other tasks. These tasks can be fulfilled on a high-end server machine instead of being handled by the end user. Databases A database is a collection of information stored in logical containers called tables. Each table normally contains related information, such as personal statistics, product data, or inventory. When you peek inside a table, you'll see that it is made up of the following: Rows A row is a complete data record, such as a company's complete shipping address or a person's vital statistics. Fields A field is a particular piece of information in a data record, such as a first name, a title, or a phone number. Figure 16.2. Database fields and rows.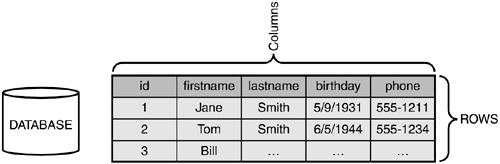 For example, a database table for your customers might have the following fields: customerid firstname lastname address city state zip phone email notes Together, the fields of a database table make up its schema, or structure. Why Databases Are Better Than Flat Files What's the point of having database tables? Why not just have flat text files that contain long lines (rows) each divided by commas into fields: 1,Tom,Smith,123 Main St.,Anytown,TX,12345,512-555-1212,tom@smith. com, New customer. This approach might work, but imagine what would happen each time a user added information to the file. The Web application would have to open the file, go down to the end of the file, and add a line. If more than one person at a time wanted to add information to the file, most everyone would have to wait in line. Multiply this problem if a user needed to update a line of information or delete a line. Databases were invented to help solve these problems. Modern database systems offer fast data retrieval, easily managed information, and features such as data locking to prevent users from walking all over each other. | Data Types But just having a list of fields isn't enough. You have to tell the database what data type can be contained by these fields. Data types define what kind of data is acceptable to that field and can include the following: Autocreated ID numbers, used to uniquely identify a record Characters, such as letters, numbers, and special characters Integers, or whole numbers Floating numbers, or numbers with decimals Binary information, such as images and spreadsheets Date and time stamps, to record when something happened or when something is due Options, such as on/off, yes/no and so on Data Lengths Knowing what fields are in a database table and the data types that are allowed in those fields still isn't enough. A database table also needs to know how much data to allow in each field. You can define different kinds of data lengths, but here are some suggested data lengths: Names (character data) 64 characters is usually sufficient. IDs (integer data) 10 characters. Passwords (character data) 10 16 characters. Addresses (character data) 128 characters. Dates (date stamps) 6 12 characters. TIP Each database has different options for length on each data type. Be sure to read the user documentation that comes with your database software. Adding Information to a Table There are a variety of ways to add information to a database from a Web application, but the most widely used is an HTML form. When a user enters information into an HTML form and clicks the Submit button, each element in the form (fields, lists, and check boxes, for instance) is converted to a variable that matches the name given to that element in the HTML code. NOTE For example, if an input field is named Address, whatever data input is in that field is assigned to the $Address variable. These variables are then processed by the middleware, which connects to the database and inserts the information from the form using SQL (Standard Query Language). The information is inserted into the database, one row at a time. Figure 16.3. The process of inserting information into a database from a Web form.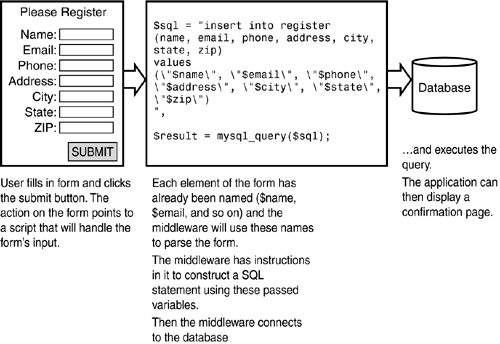 Therefore, to continue with the example, your first customer data record might look like this: Because we are using a customerid to uniquely identify this customer, another Tom Smith even one that lives on the same street in the same town would never be confused for this Tom Smith. This kind of ID is called a primary key, which becomes important when we start talking about relational databases (more on this later). Queries A query is used to insert, update, delete, and extract data from a database. Queries can be very specific, such as extracting all rows in which TX is in the state field (using the preceding example), or they can extract (or delete) all records in a table. Database Management Systems and Relational Systems A database isn't just a table, though it can be a number of tables in the same database. To continue the preceding example, you might have other tables in your database besides a customer database. You might also have a table that holds product information (if you have an e-commerce site, for example). All these tables reside in a database management system, or DBMS. Popular DBMS packages include Microsoft Access, MySQL, and Oracle. A relational database is a series of database tables that relate to each other. Although this may not seem very important, think about what happens when you start adding tables to your database. To pursue the example, you add a product table to your database and store information in that table about each of your products. Now comes the tough part: you need a table to store information about each sale you make on the site. In the old-fashioned way of doing things, you'd have to build a table (let's call it Sales) and use it to record every single piece of information about the customer and every single piece of information about the product they bought. Not only is this a terrible waste of space, but it can create havoc every time you change information (such as a customer's address or product description), you'd have to keep track of all the places you need to make changes, every time you have to make changes. Not very pretty. Using a relational database is much easier. If you wanted to track which customers purchased which products on your site, you would need only a simple table that tracked two numbers: a customerid and a productid. The customerid would tell you which customer made the purchase, and the productid would tell you what product was purchased. Each ID would point back to a unique ID in the customer and product tables. This simple notation keeps you from having to repeat (and keep track of) information such as telephone numbers and addresses. Connecting to a Database From the Web application's standpoint, it has to connect to a database before it can do any extracting, adding, updating, or deleting of information. To connect to a database, it must know the following information: Where the database server resides The username and password to get into the database server The name of the database to open In some cases, as with Microsoft ODBC data sources, you can set up a Data Source Name (see the following section) that can simplify the connection process. With PHP, JSP, and other languages, you can create connection objects that handle connecting to a database for you. Structured Query Language Structured Query Language, or SQL (pronounced "sequel") was designed as a data operations language in a relational environment. Each SQL command, or statement, can do any of a number of operations, including the following: Select rows from a table (or tables). Add a row to a table. Update a row in a table. Delete a row from a table. Create or delete a table. Chapter 27, "SQL Primer," covers SQL syntax in more detail, but for right now, I'd like to cover the basics of selecting rows from a table. Starting with Select The easiest way to learn SQL is to start with a simple SELECT statement. Let's continue with the customer table example. If you recall, the table had the following fields, or schema: customerid firstname lastname address city state zip phone email notes If you want to see all the records in the database, you would use this command: select * from customer For most Web applications, though, doing a SELECT * on a database table could lead to trouble, as this means the visitor must patiently wait for the entire database to load in the browser. Even with a fast connection and heavy-duty server, this operation could take some time especially if the site gets a lot of visitors. To filter some of the records that get extracted, you can modify your SELECT statement in various ways. The first is to replace the * with actual field names in the first clause of the statement. select customerid,city,state from customer The previous SELECT statement would retrieve only three fields from each record in the database table. If you want to further filter what gets retrieved from the database, you can add a where clause to the end of the SELECT statement. A where clause defines a condition that must be met. Only those records that match the condition in the where clause get extracted: select customerid,city,state from customer where state = 'tx' The previous SELECT statement would retrieve only three fields from each record in the table, but only if the state field had "tx" in it. Effectively, this operation restricts the recordset, or records returned, to those customers in Texas. You can make your where clauses fairly complex, stringing them together with ands and ors: select customerid,city,state from customer where state = 'tx' and email like '%.org' The previous example not only shows that two conditions have to be met, but it also introduces the like operator and the wildcard (%). In the first case, both conditions have to be met for a record to be returned that is, the customer must have a Texas address and an email address that ends in ".org." Using or instead of and would have extracted records that matched either condition. In the second case, the like operator allows you to pull out records for which you have only partial information. For example, you may know only part of an email address, or just the first few letters of a person's name or street address. Using the = operator would have returned no records because = is expecting to match exactly what you tell it to match. The wildcard symbol (%) instructs the database to match any number of characters, or any length. In the preceding example, the SQL statement matched any number of characters and ".org" in the email field of the customer table. In this case, ".org" would come at the end because the wildcard symbol fills in the front part of the field. To match a specific set of letters at the front of a field and then anything else at the end, use this notation: select customerid,firstname,lastname,email from customer where email like 'tom@%' The previous example would have extracted all records for customers with 'tom' in their email address. This means that your recordset would have included customers with emails such as tom@abc.com, tom@xyz.com, tom@tom.com, and so on. SQL statements are not case sensitive, nor are they whitespace sensitive. This fact allows us to write SQL statements without regard for line breaks. In fact, the preceding SQL statement could have been written like this: SELECT customerid,city,state FROM customer WHERE email LIKE 'tom@%' For more information on SQL, refer to Chapter 27. It includes further detail on select statements, Data Source Name When you are trying to access an ODBC (Open DataBase Connectivity) data source, such as a Microsoft SQL Server database or Excel spreadsheet, you can significantly speed up operations by setting up a Data Source Name (DSN). A Data Source Name (DSN) is a logical name that refers to not only the data source, but also the drive it sits on. On Windows machines, you use the Control panel to access a tool to create a DSN. Creating a DSN can simplify the commands you give to connect to that database. |