Arrays are often the most useful types in any programming language and the most frustrating to grasp. Different programming languages provide different rules for arrays, so it's easy to become confused. The .NET Framework simplifies this process and creates one set of rules for all arrays. Arrays are groups of variables stored together that can be referenced individually by their indices. Imagine an egg carton. The carton itself is the container, and each egg is a variable. You can reference each egg by its index: egg 1, egg 2, and so on. (Figure 3.3 demonstrates this concept.) This allows you to store similar elements together. Figure 3.3. An array is much like an egg carton.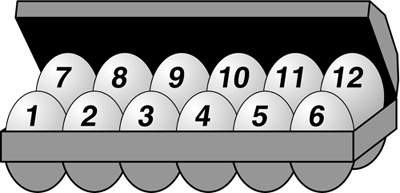 Arrays in VB.NET and C# are zero-based, meaning that the first item in an array is stored at index 0. Thus, the last item's index array is always one minus the number of items in the array. All variables in an array must be of the same data type you can't mix and match. Let's look at a simple declaration of an array in VB.NET: Dim MyArray(6) As Integer Dim MyArray2() As String = {"dog", "cat", "horse", _ "elephant", "llama"} On the first line you simply declare an array with six total slots (that are empty) for Integers. This means the last item in the array will be at index 5. The number in parentheses on line 1 tells you how many elements should be in the array. This number is also referred to as the length of the array. If you want to explicitly declare the array, as on line 2, you must leave this number off and use curly brackets ({ } ) to set the values. The length of this second array is 5. "Dog" is at index 0 and you would refer to it as MyArray2(0), while "llama" is MyArray2(4). The code in C# would be int[] MyArray = new int[6]; string[] MyArray2 = new string[5] {"dog", "cat", "horse", "elephant", "llama"}; There are a few differences here. First, instead of using parentheses for arrays, we use square brackers [...]. Next, the brackets must go after the data type rather than the variable name. Finally, to initialize the array you must use the new keyword, followed by the data type with brackets and the number of elements in the array. Listing 3.4 shows an example of using arrays in VB.NET, and Listing 3.5 shows the same example in C#. Listing 3.4 Declaring and Referencing Array Elements 1: <%@ Page Language="VB" %> 2: 3: <script runat="server"> 4: dim arrColors(5) as String 5: 6: sub Page_Load(obj as object, e as eventargs) 7: arrColors(0) = "green" 8: arrColors(1) = "red" 9: arrColors(2) = "yellow" 10: arrColors(3) = "blue" 11: arrColors(4) = "violet" 12: 13: Response.Write("The first element is: ") 14: Response.Write(arrColors(0) & "<p>") 15: 16: Response.Write("The third element is: ") 17: Response.Write(arrColors(2) & "<p>") 18: 19: Response.Write("The 5-3 element is: ") 20: Response.Write(arrColors(5-3) & "<p>") 21: end sub 22: </script> 23: 24: <html><body> 25: 26: </body></html> Listing 3.5 Declaring and Referencing Array Elements in C# 1: <%@ Page Language="C#" %> 2: 3: <script runat="server"> 4: string[] arrColors = new string[5]; 5: 6: void Page_Load(Object Sender, EventArgs e) { 7: arrColors[0] = "green"; 8: arrColors[1] = "red"; 9: arrColors[2] = "yellow"; 10: arrColors[3] = "blue"; 11: arrColors[4] = "violet"; 12: 13: Response.Write("The first element is: "); 14: Response.Write(arrColors[0] + "<p>"); 15: 16: Response.Write("The third element is: "); 17: Response.Write(arrColors[2] + "<p>"); 18: 19: Response.Write("The 5-3 element is: "); 20: Response.Write(arrColors[5-3] + "<p>"); 21: } 22: </script> 23: 24: <html><body> 25: 26: </body></html>  | On line 4 of either listing you create your array of strings, and you assign values to it on lines 7 11. Lines 14, 17, and 20 show various ways of referencing the elements. Figure 3.4 shows the output of this code. | Figure 3.4. Different methods to access array elements.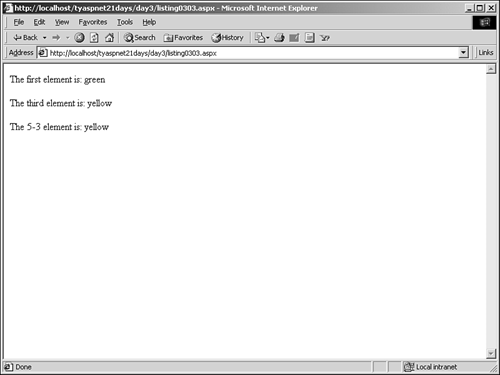 There are two functions in VB.NET designed specifically to manipulate arrays: Redim and Erase. Once you declare an array, you're limited to the size you've specified. However, Redim can be used to change the array size. For example: Redim arrColors(6) Your colors array now has a size of 6. The existing values are destroyed in the process, however. You can use the keyword preserve to save these values: Redim Preserve arrColors(6) If the new array size is smaller, the values that fall outside the array's dimensions are discarded. If the new size is larger, the extra indices are simply initialized to the default values.  | The Erase statement sets each value in the array to Nothing, which is VB.NET's way of saying there's no value stored in the variable: | Erase arrColors Note The Array class in the .NET Framework provides more functions for array manipulation. See the .NET Framework SDK documentation for more information. |