Visual Basic .NET provides a broad range of data types that you can use when defining variables and working with data. These data types are slightly different from those provided by VB6 or VBScript. Table 7.1 lists the available types, along with the corresponding .NET Framework data types (each .NET language, such as Visual Basic .NET or C# .NET, maps its specific data types to data types provided by the .NET Framework). TIP Note that the VB6 Currency data type is gone. Currency was a strange type: In order to avoid some round-off errors, Visual Basic multiplied the data values you entered by 10,000 for storage in memory and then divided by 10,000 again for display. In the .NET Framework, use the Decimal type instead, if you want floating-point values that don't exhibit round-off errors when you perform calculations on the values. Table 7.1. Visual Basic .NET Supplies a Broad Range of Data Types VB Type | CLR Type | Storage Size | Value Range | Boolean | System.Boolean | 2 bytes | True or False. | Byte | System.Byte | 1 byte | 0 to 255 (unsigned). | Char | System.Char | 2 bytes | 0 to 65,535 (unsigned). | Date | System.DateTime | 8 bytes | January 1, 0001 to December 31, 9999. | Decimal | System.Decimal | 16 bytes | +/-79,228,162,514,264,337,593,543,950,335 with no decimal point; +/-7.9228162514264337593543950335 with 28 places to the right of the decimal. | Double | System.Double | 8 bytes | -1.79769313486231E+308 to -4.94065645841247E-324 for negative values; 4.94065645841247E-324 to 1.79769313486231E+308 for positive values. | Integer | System.Int32 | 4 bytes | -2,147,483,648 to 2,147,483,647. | Long | System.Int64 | 8 bytes | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. | Object | System.Object | 4 bytes | Any type can be stored in a variable of type Object. | Short | System.Int16 | 2 bytes | -32,768 to 32,767. | Single | System.Single | 4 bytes | -3.402823E+38 to -1.401298E-45 for negative values; 1.401298E-45 to 3.402823E+38 for positive values. | String | System.String | Depends on the implementing platform | Zero to approximately 2 billion Unicode characters. | User-defined type (structure) | Sum of the sizes of its members | | Each member of the structure has a range determined by its data type and is independent of the ranges of the other members. | Load VBLanguage.aspx in the page designer. This page, shown in Figure 7.2, allows you to try out all the sample code for the remainder of this chapter. Double-click VB.NET Data Types to load the following code. This procedure provides examples of how to declare and assign a value to each of the different data types: Figure 7.2. This sample page allows you to try out all the code.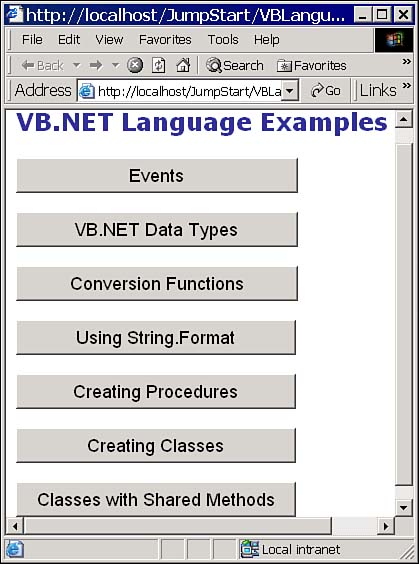 NOTE You may be wondering why some of the assignments in the sample code require extra method or function calls. For example, to assign a value into a Decimal variable, we must call the CDec function. To print out the value of an Object variable, we had to call the ToString method. It's the Option Strict directive that forces this extra work see the next section for more information. Listing 7.3 shows code from the sample project that uses many of the basic data types. Listing 7.3 This Code Shows Examples of the Types Available in VB .NET Private Sub DataTypesSample() Dim blnValue As Boolean Dim bytValue As Byte Dim chrValue As Char Dim dtValue As Date Dim decValue As Decimal Dim dblValue As Double Dim intValue As Integer Dim lngValue As Long Dim oValue As New Object() Dim srtValue As Short Dim sngValue As Single Dim strValue As String blnValue = True bytValue = 32 chrValue = CChar("A") dtValue = #1/1/2002# decValue = CDec(100.5) dblValue = 10.25 intValue = 10 lngValue = 1000000 oValue = "A String" srtValue = 0 sngValue = 1.5 strValue = "This is a string" With Response .Write("blnValue = " & blnValue & "<BR>") .Write("bytValue = " & bytValue & "<BR>") .Write("chrValue = " & chrValue & "<BR>") .Write("dtValue = " & dtValue & "<BR>") .Write("decValue = " & decValue & "<BR>") .Write("dblValue = " & dblValue & "<BR>") .Write("intValue = " & intValue & "<BR>") .Write("lngValue = " & lngValue & "<BR>") .Write("oValue = " & oValue.ToString() & "<BR>") .Write("srtValue = " & srtValue & "<BR>") .Write("sngValue = " & sngValue & "<BR>") .Write("strValue = " & strValue & "<BR>") End With End Sub Option Strict Visual Basic has, in its past versions, allowed data type conversions that other languages never permitted. For example, in Visual Basic 6.0, it was quite possible to assign an integer into a string and to perform mathematical operations on strings, like this: Dim x As String x = 25 + "2" After running this code, the variable x would contain the string "27". Because this type of programming can lead to errors, when variables can contain data that you might not have intended, Visual Basic .NET supports an option that forces you to explicitly convert from one type to another: Option Strict. You can add this statement to the top of each module to turn on strict conversions for the module: Option Strict On You can also right-click the project in the Solution Explorer window, select Properties from the context menu, and then select the Build tab in the left pane of the window. In the Option Strict drop-down list, select On to turn the option on for the entire project. With Option Strict set to On, you won't be able to make the type of "loose" conversions you might have made in Visual Basic 6.0. Attempting to compile code that doesn't meet the stringent requirements of Option Strict will fail. HANDLING OPTION STRICT In VB .NET, Option Strict is off by default. This is a poor decision, in our eyes, because it allows the same sort of code you might have written in VB6, including potentially dangerous type conversions. With Option Strict turned on, you can catch these errors at compile time, instead of at runtime basically, there's less to worry about at runtime, with the tradeoff being more to worry about at coding time. It also forces you into a better understanding of the objects you are working with, and it enables code to be reused in a project where Option Strict is on. As it is, you must open the project properties for each new project you create, in order to turn on Option Strict (or you can manually add it to the top of each file, which is certainly an onerous task). You can set Option Strict on in VB .NET for all new projects you create, but it's a nontrivial task. To do this, find the folder containing all the project templates. For example, this might be a folder such as H:\Program Files\Microsoft Visual Studio.NET\Vb7\VBWizards. Starting in that folder, use Windows Explorer to search for *.vbproj. Load all the files named *.vbproj into a text editor and modify the XML at the top of the file so that it looks something like this (what we're showing here is just the beginning of the vbproj file; leave all the existing tags alone and simply insert the OptionStrict attribute within the Settings element): <VisualStudioProject> <VisualBasic> <Build> <Settings OptionStrict = "On" -- more attributes and elements follow -- Save each file, and the next time you create a project based on one of these templates, Option Strict will be on, by default. Most likely, you want Option Strict on for development in VB .NET, and you'll forget to turn it on manually. | Conversion Functions Once you've enabled Option Strict in Visual Basic .NET, you'll need to take advantage of a group of functions that allow you to convert from one data type to another. You can use either the CType function (which can convert any type to any other compatible type) or the individual conversion functions (CStr, CChar, CLng, and so on). The CType function takes two arguments: The first argument is the variable or value you wish to convert, and the second argument is the data type you wish to convert the value to. The general syntax looks like this: var1 = CType(var2, DataType) Visual Basic .NET also provides a group of distinct functions (the names all begin with C) that you can use to convert data types from one type to another. In fact, these functions have not changed much from the similar functions available in Visual Basic 6.0. The list of conversion functions includes CBool, CByte, CDate, CDbl, CDec, CInt, CLng, CSng, CShort, and CStr. The procedure shown in Listing 7.4, from the file VBLanguage.aspx, demonstrates using several of the conversion functions: Listing 7.4 Converting from One Data Type to Another Is Common in Many Applications Private Sub ConversionSample() Dim srtValue As Short = 0 Dim intValue As Integer = 10 Dim lngValue As Long = 100 Dim sngValue As Single = 1.5 Dim dblValue As Double = 10.25 ' Convert up using CType sngValue = CType(srtValue, Single) dblValue = CType(sngValue, Double) lngValue = CType(intValue, Long) ' Convert up using C* functions sngValue = CSng(srtValue) dblValue = CDbl(sngValue) lngValue = CLng(intValue) End Sub TIP This example takes advantage of one of VB .NET's new features: the ability to initialize a variable at the time you declare it. Although we don't recommend overusing this privilege both in terms of scoping issues and in terms of the errors that can occur when code runs as you declare a variable it works well for simple constant values. Constructing Text Using the String.Format Method You'll often find that you need to construct strings either by concatenating bits and pieces of text or by inserting values into placeholders within an existing string. To make this task simpler, the .NET Framework supplies the String.Format method. This method allows you to supply a template string containing numbered placeholders for replacements. You provide the replacements as the rest of the parameters for the String.Format method, and at runtime the .NET Framework replaces each placeholder with its value. (You can also supply the replacements as an array of objects rather than as individual values, as we've done here. It's up to you.) To try out the String.Format method, double-click Using String.Format on the sample page. This leads you to this procedure: Private Sub StringFormatSample() Response.Write(String.Format( _ "Customer {0} spent {1:C} in {2}.", _ 12, 14.56, "January")) End Sub In this example, the template string (Customer {0} spent {1:C} in {2}.) provides three placeholders, which must begin numbering at 0. The second one ({1:C}) provides additional formatting information (:C indicates currency formatting). The documentation lists the complete set of possible formatting options. At runtime, the code replaces each of the placeholders with one of the last three parameters. Running the code in this example provides the following result: Customer 12 spent $14.56 in January. As you can see, the code replaces each of the placeholders in turn with the corresponding value from the remainder of the parameter list. You'll see another example of using the String.Format method in the next section. |