ASP seemed great a few years ago, but now ASP.NET provides a simpler, faster, more powerful way to create Web applications. Instead of using a scripting language, you may now create real, fully compiled applications. You can write these applications using any of the .NET-compliant languages available, and you can use the great tools provided by Visual Studio .NET to do your work. Any developer can now create full-featured, powerful Web applications. Creating a New ASP.NET Application Enough talking about it how about actually creating a simple Web application? To get started, you'll create a project with a Web Form that allows users to input first and last names. After entering the data into these two text controls on the Web page, a user clicks a button and sees the entered information appear in a label below the button. Figure 5.1 shows the sample Web Form you will create. Figure 5.1. This simple page allows you to enter values, and clicking the button runs code to process those values.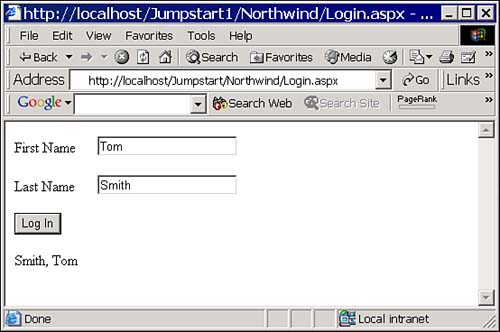 Creating the Login Form To get started, you'll need to create a new Web Application project in Visual Studio .NET: -
Start Visual Studio .NET and select the File, New, Project menu item. -
In the New Project dialog box, select Visual Basic Projects in the Project Types pane. -
In the Templates pane, select ASP.NET Web Application. -
In the Location text box, enter http://www.localhost/Jumpstart/Northwind as the location for the project. -
Click OK to allow Visual Studio .NET to create the virtual root, add the project template, and prepare for you to begin working on the project. This may take a few moments. -
By default, Visual Studio .NET creates a page named WebForm1.aspx. Although you could develop your application using this page, you'll generally want to rename the page, the code file, and the programming class contained within the code file. It's easier to simply delete the whole page and create a new one, using your own name. -
Select WebForm1.aspx in the Solution Explorer window (most likely, the Solution Explorer window will appear in the upper-right corner of the Visual Studio .NET environment), right-click, and select Delete from the context menu. -
Select the Project, Add Web Form menu item. -
Set the name of this new page to Login.aspx and then click Open to add the page. -
Use the View, Toolbox menu item to ensure that the Toolbox window is visible. Then add controls and set properties as shown here. Control Type | Property | Value | Label | ID | Label1 | | Text | First Name | TextBox | ID | txtFirst | Label | ID | Label2 | | Text | Last Name | TextBox | ID | txtLast | Button | ID | btnLogin | | Text | Login | Label | ID | lblName | | BorderStyle | Inset | | Text | (Delete the text, so you see just the label's name.) | -
To view the layout information you've created, choose the View, HTML Source menu item (or click the HTML tab at the bottom of the designer window). You'll see HTML but no programming code that goes into a separate location. -
Select the View, Design menu item to get back to the normal design view. -
Select File, Save All to save your project. Running the Login Form At this point, you can run this application and see the Web Form appear in your browser. Although this page does not have any functionality yet, this exercise is a good test to make sure everything is running up to this point. Here are the steps to follow: -
Select Login.aspx in the Solution Explorer window. -
Right-click and select Set as Start Page from the context menu. -
Press F5 to run this sample application. TIP If you have trouble running the application, refer back to the instructions in Chapter 1, "Getting Started with the Sample Application." You may need to configure your project to allow debugging. You should now see the Web Form displayed in your browser, and you can enter data into the two text fields. If you click the button, nothing will happen because you have not told it to do anything yet. You need to add some code in order for the button to have any effect. TIP While your page is open in the browser window, right-click and select View Source from the context menu. Although there will be a bunch of stuff in the page that you didn't put there (ASP.NET adds some useful support that you'll learn about later), you should see standard HTML for the controls you did place on the page. Adding Code to the Button If you want the button to actually "do" anything, you need to add some code. For this example, you need to add code so that the button posts the data you entered in the text boxes and fills in the appropriate data in the label below the button control. Follow these steps to add the Visual Basic .NET code you need: -
Stop the program from running by closing down the browser. -
While the page is open in the Visual Studio .NET page designer, double-click the LogIn button. You will now see a code window appear with the procedure btnLogin_Click already created for you. -
Modify this procedure, which will run when a user clicks the button, so that it looks like this: Private Sub btnLogin_Click( _ ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles btnLogin.Click lblName.Text = txtLast.Text & ", " & txtFirst.Text End Sub NOTE In your editor, the code will look slightly different. The first long line of code, the procedure definition, will appear all on a single line. To make the code fit on the printed page, we've wrapped this one logical line of code into multiple physical lines, ending each line with Visual Basic .NET's line continuation characters (a space followed by an underscore). You needn't make this same change in your own code, unless you want to. We do this throughout this book to make the code visible within the limited range of printed space. -
The code you just wrote retrieved the Text property from both the txtLast and txtFirst text boxes, and it places the data into the Label control on the page. If you've ever programmed in any flavor of Visual Basic, this should look awfully familiar. In fact, the whole point of programming in .NET is that you should be able to use familiar techniques, like these, no matter what type of application you're creating. Finally, it's time to test your masterpiece. -
Run the application by pressing F5 again. -
Enter a first and last name and click Login. -
If you have done everything correctly, you should see the entered name appear in the label below the button. Where did you put your code? When you created the Web Form, you created a file with an .aspx extension (Login.aspx). This file contains only layout information, generally. When you added code, you modified a corresponding file, the "code-behind" file (believe us, we don't make these things up), named Login.aspx.vb. All the programming code goes into this separate file, as part of a class named Login: Public Class Login ... Private Sub btnLogin_Click( _ ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles btnLogin.Click lblName.Text = txtLast.Text & ", " & txtFirst.Text End Sub End Class This class defines the programmatic behavior for this page. When you press F5 to run the project, Visual Studio .NET compiles your class into a DLL. When you browse to the page, ASP.NET loads the layout information from the ASPX page as well as the code from the compiled DLL. This separation provides for all sorts of exciting flexibility (you can update the code on your site, even while users are currently viewing pages on the site, for example) and makes it simpler to create sites. It's important to consider what happens when you click the button on the sample page: The form posts its data back to the server. The server recomposes the page using the data you typed in and places it back into the page. The server then sends the page back to the browser. The browser renders the page again. It's interesting (and important) to note that if you were using ASP (rather than ASP.NET), it would be up to you to place the values back into the appropriate HTML tags. In this case, even after you click the button, the first name and last name values you entered still appear in the text box controls. If you were using a standard ASP page, that wouldn't be the case. |