Testing the OutlookBar Control Testing the final control is a victory that should be savored. The various controls, components, and designers for the OutlookBar control and its related controls demonstrate the basic requirements for creating custom controls for Windows Forms. Figure 9.3 shows the test application displaying a MessageBox indicating which item was clicked on. Figure 9.3. The OutlookBar client.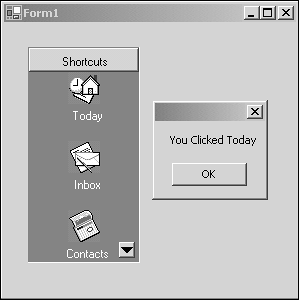 To test the OutlookBar control, compile the solution containing all the code developed thus far. This will result in the dll SAMS.ToolKit.dll being created. This dll houses all the components, controls, and designers developed to this point. Creating the client application requires starting a new Windows Forms project and adding the OutlookBar and ImageListView controls to the Toolbox. Adding the controls to the Toolbox requires customizing the Toolbox and browsing to the location of the newly compiled dll. To create the test application, drag the OutlookBar control onto the main form. Next, drag an ImageListView control onto the OutlookBar control. A new tab is automatically created. Associate an ImageList component with the ImageListView control containing a set of large images. The images can be of anything. The images shown in Figure 9.3 were borrowed from Microsoft Outlook. After the ImageList has been associated with the ImageListView control, use the Items property editor to add ImageListViewItems and set the text and image index for them. Listing 9.5 shows the result of the test client code. Listing 9.5 OutlookBar Client 1: using System; 2: using System.Drawing; 3: using System.Collections; 4: using System.ComponentModel; 5: using System.Windows.Forms; 6: using System.Data; 7: 8: namespace OutlookBar_Test 9: { 10: /// <summary> 11: /// Summary description for Form1. 12: /// </summary> 13: public class Form1 : System.Windows.Forms.Form 14: { 15: private SAMS.ToolKit.Controls.OutlookBar outlookBar1; 16: private System.Windows.Forms.ImageList LargeImages; 17: private SAMS.ToolKit.Controls.ImageListView imageListView1; 18: private SAMS.ToolKit.Controls.OutlookBarTab outlookBarTab1; 19: private SAMS.ToolKit.Controls.ImageListViewItem imageListViewItem1; 20: private SAMS.ToolKit.Controls.ImageListViewItem imageListViewItem2; 21: private SAMS.ToolKit.Controls.ImageListViewItem imageListViewItem3; 22: private SAMS.ToolKit.Controls.ImageListViewItem imageListViewItem4; 23: private SAMS.ToolKit.Controls.ImageListViewItem imageListViewItem5; 24: private SAMS.ToolKit.Controls.ImageListViewItem imageListViewItem6; 25: private SAMS.ToolKit.Controls.ImageListViewItem imageListViewItem7; 26: private System.ComponentModel.IContainer components; 27: 28: public Form1() 29: { 30: // 31: // Required for Windows Form Designer support 32: // 33: InitializeComponent(); 34: 35: // 36: // TODO: Add any constructor code after InitializeComponent call 37: // 38: } 39: 40: /// <summary> 41: /// Clean up any resources being used. 42: /// </summary> 43: protected override void Dispose( bool disposing ) 44: { 45: if( disposing ) 46: { 47: if (components != null) 48: { 49: components.Dispose(); 50: } 51: } 52: base.Dispose( disposing ); 53: } 54: 55: #region Windows Form Designer generated code 56: /// <summary> 57: /// Required method for Designer support - do not modify 58: /// the contents of this method with the code editor. 59: /// </summary> 60: private void InitializeComponent() 61: { 62: this.components = new System.ComponentModel.Container(); 63: System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(Form1)); 64: this.outlookBar1 = new SAMS.ToolKit.Controls.OutlookBar(); 65: this.LargeImages = new System.Windows.Forms.ImageList(this.components); 66: this.imageListView1 = new SAMS.ToolKit.Controls.ImageListView(); 67: this.outlookBarTab1 = new SAMS.ToolKit.Controls.OutlookBarTab(); 68: this.imageListViewItem1 = new SAMS.ToolKit.Controls.ImageListViewItem(); 69: this.imageListViewItem2 = new SAMS.ToolKit.Controls.ImageListViewItem(); 70: this.imageListViewItem3 = new SAMS.ToolKit.Controls.ImageListViewItem(); 71: this.imageListViewItem4 = new SAMS.ToolKit.Controls.ImageListViewItem(); 72: this.imageListViewItem5 = new SAMS.ToolKit.Controls.ImageListViewItem(); 73: this.imageListViewItem6 = new SAMS.ToolKit.Controls.ImageListViewItem(); 74: this.imageListViewItem7 = new SAMS.ToolKit.Controls.ImageListViewItem(); 75: this.outlookBar1.SuspendLayout(); 76: this.SuspendLayout(); 77: // 78: // outlookBar1 79: // 80: this.outlookBar1.Location = new System.Drawing.Point(24, 24); 81: this.outlookBar1.Name = "outlookBar1"; 82: this.outlookBar1.Size = new System.Drawing.Size(112, 216); 83: this.outlookBar1.TabIndex = 0; 84: this.outlookBar1.Tabs.AddRange(new SAMS.ToolKit.Controls.OutlookBarTab[] { 85: this.outlookBarTab1} ); 86: this.outlookBar1.Text = "outlookBar1"; 87: // 88: // LargeImages 89: // 90: this.LargeImages.ColorDepth = System.Windows.Forms.ColorDepth.Depth8Bit; 91: this.LargeImages.ImageSize = new System.Drawing.Size(32, 32); 92: this.LargeImages.ImageStream = ((System.Windows.Forms.ImageListStreamer)(resources.GetObject("LargeImages.ImageStream"))); 93: this.LargeImages.TransparentColor = System.Drawing.Color.Transparent; 94: // 95: // imageListView1 96: // 97: this.imageListView1.BackColor = System.Drawing.SystemColors.ControlDark; 98: this.imageListView1.ForeColor = System.Drawing.SystemColors.ControlLightLight; 99: this.imageListView1.Items.AddRange(new SAMS.ToolKit.Controls.ImageListViewItem[] { 100: this.imageListViewItem1, 101: this.imageListViewItem2, 102: this.imageListViewItem3, 103: this.imageListViewItem4, 104: this.imageListViewItem5, 105: this.imageListViewItem6, 106: this.imageListViewItem7} ); 107: this.imageListView1.LargeIcons = true; 108: this.imageListView1.LargeImageList = this.LargeImages; 109: this.imageListView1.Location = new System.Drawing.Point(1, 25); 110: this.imageListView1.Name = "imageListView1"; 111: this.imageListView1.Size = new System.Drawing.Size(110, 190); 112: this.imageListView1.SmallImageList = null; 113: this.imageListView1.TabIndex = 0; 114: this.imageListView1.ItemClicked += new SAMS.ToolKit.Controls.ItemClickedEventHandler(this.imageListView1_ItemClicked); 115: 116: this.outlookBarTab1.Alignment = System.Drawing.StringAlignment.Center; 117: this.outlookBarTab1.Child = this.imageListView1; 118: this.outlookBarTab1.ForeColor = System.Drawing.SystemColors.ControlText; 119: this.outlookBarTab1.Text = "Shortcuts"; 120: 121: this.imageListViewItem1.ImageIndex = 0; 122: this.imageListViewItem1.Text = "Today"; 123: 124: this.imageListViewItem2.ImageIndex = 1; 125: this.imageListViewItem2.Text = "Inbox"; 126: 127: this.imageListViewItem3.ImageIndex = 2; 128: this.imageListViewItem3.Text = "Contacts"; 129: 130: this.imageListViewItem4.ImageIndex = 3; 131: this.imageListViewItem4.Text = "Calendar"; 132: 133: this.imageListViewItem5.ImageIndex = 4; 134: this.imageListViewItem5.Text = "Tasks"; 135: 136: this.imageListViewItem6.ImageIndex = 5; 137: this.imageListViewItem6.Text = "Notes"; 138: 139: this.imageListViewItem7.ImageIndex = 6; 140: this.imageListViewItem7.Text = "Trash"; 141: 142: this.AutoScaleBaseSize = new System.Drawing.Size(5, 13); 143: this.ClientSize = new System.Drawing.Size(292, 273); 144: this.Controls.AddRange(new System.Windows.Forms.Control[] { 145: this.outlookBar1} ); 146: this.Name = "Form1"; 147: this.Text = "Form1"; 148: this.outlookBar1.ResumeLayout(false); 149: this.ResumeLayout(false); 150: 151: } 152: #endregion 153: 154: /// <summary> 155: /// The main entry point for the application. 156: /// </summary> 157: [STAThread] 158: static void Main() 159: { 160: Application.Run(new Form1()); 161: } 162: 163: private void imageListView1_ItemClicked(object sender, SAMS.ToolKit.Controls.ImageListViewEventArgs e) { 164: string msg = string.Format("You Clicked { 0} ", this.imageListView1.Items[ e.ItemIndex ].Text ); 165: MessageBox.Show( this, msg ); 166: } 167: } 168: } Listing 9.5 is 99% generated during the design process of the test application. The only hand-coded method is the imageListView1_ItemClicked event handler on line 163, and even the stub for this was genereated by double-clicking on the ImageListView control. The imageListView1_ItemClicked event handler uses the ImageListViewEventArgs parameter to get the text of the ImageListView item and displays a message box stating which item was clicked on. Of 168 lines of code, only 2 lines have to be typed. Isn't code generation wonderful? |