When filling out a form online, the answer given to one question can often determine the data that needs to be provided for another question. For example, answering "yes" to a question on a form may require one set of options being provided, while answering "no" requires a different set of options be presented. Rather than trying to load all of the data for any situation, using Ajax we can simply go back to the server and load data contextual to the answers provided by the visitor. In this example (Figure 22.3), when visitors select a country (Figure 22.4), the state/province list underneath is dynamically updated with data from the server, depending on whether they chose "United States" or "Canada" (Figure 22.5). Figure 22.3. When the page first loads, the State/Province field is empty. 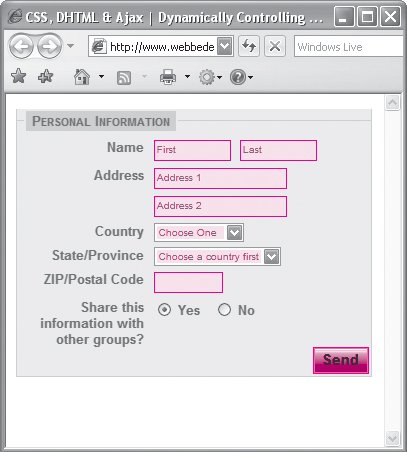 Figure 22.4. After selecting the country… 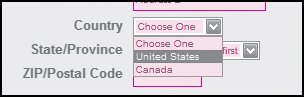 Figure 22.5. …the State/Province drop-down menu has the correct data loaded into it. 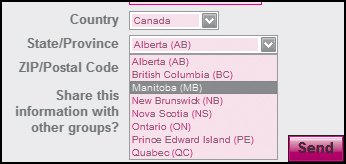 To create dynamically updated form data: | | 1. | dynamicForm.js
Start a new JavaScript file and save it as dynamicForms.js (Code 22.5). Steps 2 and 3 apply to this file.
Code 22.5. dynamicForms.js: Functions used for updating form data using Ajax. [View full width] function clearField(obj) { if (obj.defaultValue==obj.value) obj.value = ''; } function chooseCountry(requestedData, objectID) { fetchData('dataPage.php', requestedData,objectID); } function filterData(pageRequest, objectID){ if (pageRequest.readyState == 4 && (pageRequest.status==200 || window.location. href.indexOf ("http")==-1)) { var object = document.getElementById (objectID); object.options.length = 0; if(pageRequest.responseText != '') { var arrSecondaryData = pageRequest.responseText.split(','); for(i = 0; i < arrSecondaryData.length; i++) { if(arrSecondaryData[i] != '') object.options[object.options.length] = new Option(arrSecondaryData[i], arrSecondaryData[i]); }}}} | | 2. | function chooseCountry(requestedData, objectID) {…}
Add the function chooseCountry() to your JavaScript.
When triggered from Step 10, this function uses the basic Ajax function fetchData(), passing it the value for the selected country (requestedData), the ID for where the returned data should be placed (objectID), and the page on the server that this data should be passed to (dataPage.php).
| | | 3. | function filterData(pageRequest, objectID){…}
Add the function filterData() to your JavaScript. This function replaces the default version of filterData() that will be imported in Step 7.
This version of the function takes a comma separated list, splits it into an array (secondaryData[]) and then places each item into it's own <option> element in the <select> element specified by objectID.
| 4. | dataPage.php
Set up a page on your server that holds and processes the data you will be placing in your HTML page (Code 22.6).
Code 22.6. dataPage.php: A server page using PHP that takes the data sent to it and delivers back the relevant list of U.S. states or Canadian provinces. [View full width] <?php $dataOptions = array(); $dataOptions["United States"] = array("Alabama (AL)", "Alaska (AK)", "Arizona (AZ)", "Arkansas (AR)", "California (CA)", "Colorado (CO)", "Connecticut (CT)"); $dataOptions["Canada"] = array("Alberta (AB)", "British Columbia (BC)", "Manitoba (MB)", "New Brunswick (NB)", "Nova Scotia (NS)", "Ontario (ON)", "Prince Edward Island (PE)", "Quebec (QC)"); if(isset($_POST["dataRequest"]) && isset($dataOptions[$_POST["dataRequest"]])) { foreach($dataOptions[$_POST["dataRequest"]] as $secondaryOptions) { printf("%s,", $secondaryOptions); }} ?> | In this example, I'm using a simple PHP file to create an array ($dataOptions[]) that uses a country name as its index to hold a list of states or provinces.
The script takes the value passed to it through the dataRequest variable in the XMLHttpRequest call, in order to determine which list of comma separated values to deliver back to the filter script in Step 3.
| | | 5. | dynamicForms.css
Create an external style sheet that holds your styles for this page and save it as dynamicForms.css. I used styles similar to those I set up in "Styling Forms" in Chapter 21.
| 6. | index.html
Set up an HTML file and save it as index.html (Code 22.7). Steps 7 through 12 apply to this file.
Code 22.7. index.html: The page with the dynamic form. | 7. | src="/books/3/292/1/html/2/ajaxBasics.js"
Add a call to the ajaxBasics.js file created in Chapter 20 (Code 20.6). You will need to add a copy of this file to the directory or change the src value to the path where your Ajax basics file is located. This file includes the fetchData() and filterData() functions.
| | | 8. | src="/books/3/292/1/html/2/dynamicForm.js"
Add a call to the dynamicForms.js file you set up in Step 1.
| 9. | href="dynamicForm.css"
Add a link to the dynamicForms.css file you set up in Step 5.
| 10. | onchange="chooseCountry(this.options [this.selectedIndex].text, 'state');"
In the form on your page, add a <select> element with an ID value of country and an onchange event handler that triggers the function chooseCountry() from Step 2. Pass the function the currently selected value: this.options
[this.selectedIndex].text
and the ID of the <select> element you want the dynamically gathered options to be placed into, which in this example is state.
| 11. | <option>United States</option>
In the <select> element from Step 10, set up <option> elements that have text and values corresponding to the values used in the$dataOptions[] array from Step 4.
| 12. | <select name="state" size="1" tabindex="5">…</select>
Add the select element into which the filterData() function will place the dynamic data, giving the element an ID that corresponds to the value passed to the function in Step 10 (such as "state").
| |