As you can see in Figure 4.8, there are a lot of windows open during a typical Visual Studio .NET session. Each window has a different purpose related to the design or execution of your project. In the very early days of VB, each of these windows was a top-level window within the operating system and could be stacked or moved independently. Recent versions of Visual Basic have used a more structured, Multiple Document Interface (MDI) environment in which child windows are docked within the boundaries of a parent window. VB.NET continues to take the organized approach but, like its predecessors, can be adjusted to suit individual tastes. This section explores the different windows you will encounter within Visual Studio. Figure 4.8. Visual Basic .NET has several styles of windows. To change the appearance of a window, right-click its title bar to display the context menu. 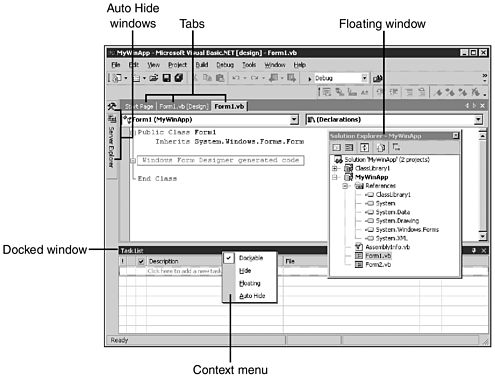 Understanding Window Behavior Figure 4.8 shows examples of the different window styles available in Visual Studio .NET. Each type of window has a different behavior in relation to other open windows. For example, some windows obscure parts of other windows when visible, instead of merging with the parent window. As you work with windows in the Visual Studio environment, you may want to try different window settings to find the window arrangement with which you are most comfortable. Auto-Hide Windows Auto-hide windows are new to VB .NET. They appear as flat buttons on the outer edge of the screen (for example, the Server Explorer and Toolbox in Figure 4.8). Hovering over the icon causes the window to slide out and display its contents. When an auto-hide window loses focus, it slides off the screen automatically. The title of the most recently displayed auto-hide window is displayed adjacent to the icon. Only the Server Explorer and Toolbox are set to auto-hide mode by default. Tip Auto-hide windows seem like they will be great for tasks that are mouse-oriented, such as drawing controls on a form. Because the Toolbox window is used during design only, more screen real estate is available for code and other windows to display debugging information. Note  | Click the thumbtack icon to make an auto-hide window remain visible on the screen. |
Dockable Windows Dockable windows are resizable windows that can be anchored in a fixed position within the development environment. When docked windows are resized, other docked windows take up the unused space. The advantage of a docked window is that it can be positioned to remain visible at the same time as other docked windows. In order to free a dockable window, right-click the title bar and choose Floating from the menu. (If you do not select floating, then the window will jump to the tab box.) To dock a window within the development environment, first make sure Dockable is selected. Next, drag the window to the title bar of another window to dock it. As with previous versions of VB, docking can be a bit tricky; it may require practice before you get comfortable with it. Floating Windows You can position floating windows on top of other windows within the development environment. The advantage of a floating window is that it can be displayed quickly without a lot of resizing. One example is using the Properties window during form design. If the Properties window is docked, you may have to resize it to read all of the text. By using floating mode, you can display it by pressing its shortcut key (function key F4), set the properties, and then close the window. Note A floating window can be especially useful when you have memorized the shortcut key to display it. For example, pressing Ctrl+Alt+L (or Ctrl+R, depending on your profile settings) displays the Solution Explorer, which can be used as a starting point to navigate to any other window in your project. Tabbed Document Area You may notice in Figure 4.8 that the code windows are organized using tabs, much like the tabs on a file folder. This is a new feature in Visual Studio .NET, which allows you to quickly access the documents in your project, such as Web pages, code, and forms. To select a tab, just single-click the tab's title and the corresponding window is displayed. Note You can use Ctrl+F6 to quickly cycle through the tab windows, or Ctrl+F4 to close the current window. (These have been standard Windows keystrokes for a long time. In addition, Alt+F4 will close an active top-level window.) If you look closely at the tabbed section in Figure 4.8, you will notice three small buttons on the far right of the tabs. If you have opened so many documents that all of their tabs will not fit on the screen, you can use the arrow buttons to scroll the tabs left or right. The third button closes the current window. You can customize the look of your tabbed documents by re-ordering the tabs and creating additional tab groups. To create a new tab group, right-click on the title of a window you want to include in the new group and a context menu will appear. You may choose to create a new tab group with either horizontal or vertical orientation. Figure 4.9 shows a screen with two horizontal tab groups. Figure 4.9. Visual Studio allows you to organize code and design windows using multiple tab groups. 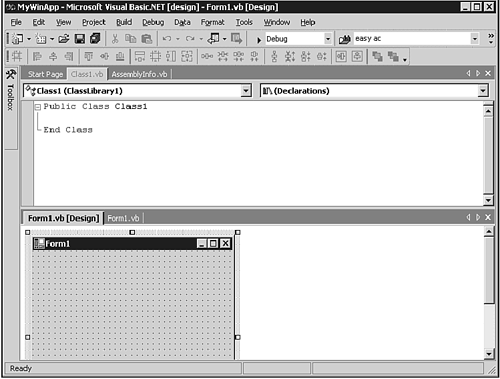 By dragging tabs with your mouse, you can re-order documents within a tab group or move a document window to another tab group. To remove a tab group, either drag all documents out of it or repeatedly click the close button until all tabs have disappeared. Note that while you can have multiple tab groups, they all must have the same orientation; that is, you can have horizontal or vertical groups but not both. Note An asterisk (*) next to the title of a document tab indicates changes have been made but not yet saved to disk. Making the Most of Available Screen Space Power users of the Visual Studio .NET environment will want to have a lot of windows open at once. For example, as you type in the code window, the dynamic help and task list continuously update themselves with pertinent information. You may also want to display immediate or watch windows while simultaneously viewing code. To use all of the information effectively, we suggest that you increase desktop video resolution to 1,024 x 768 or higher. (For readability purposes, photos in this book are taken at a resolution of 800 pixels x 600 pixels.) Right-click the background of your Windows desktop and choose Properties from the context menu. Select the Settings tab, as pictured in Figure 4.10, and change your screen area to a size with which you are comfortable. Some developers even go so far as to use Windows' multiple monitor support, which allows you to create a desktop that spans more than one monitor! Figure 4.10. For the best possible development experience, set your desktop area to a high resolution. 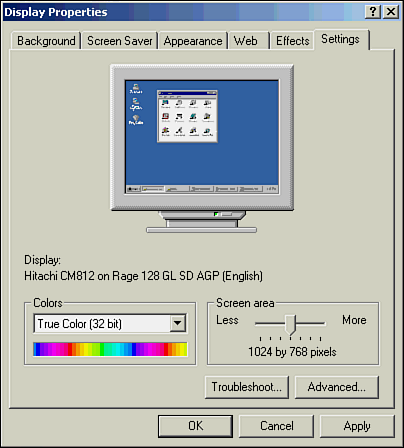 Designing Visual Components One special type of window in the Visual Studio work area is a Designer window. Designer windows are where you draw the look of your Visual Studio .NET applications, just as an artist paints on a canvas. Designing the look of your program is often the most fun part of using Visual Basic. Unlike the code, which is hidden from the user, the design allows the programmer to show off his or her creativity. Note If you have been paying attention, you probably realize by now that many objects in your applications have both a visual component as well as a code component. These two components may be part of the same object, such as a Windows form. A Windows form designer is indicated by the word [Design] next to the document title. If you read the introductory chapter, then you already know the basic process of adding controls to a form. In this section, we will discuss some of the finer points, such as working with groups of controls. If you are not familiar with the basics of drawing controls, p. 21 To perform the exercises in this section, you will need to set up a sample project. Using knowledge you have acquired from Chapter 2, "Creating Your First Windows Application," and earlier in this chapter, perform the following tasks: -
Start Visual Studio and create a new Windows Application project. -
Place three TextBox controls on the form. -
Place a Label control on the form beside each text box. -
Place a Button control on the form. Finally, align the various controls so they look similar to Figure 4.11. Creating this example will allow you to easily follow along with the concepts described in the text. Figure 4.11. Making controls line up is easy thanks to the grid of dots in the background of the form. 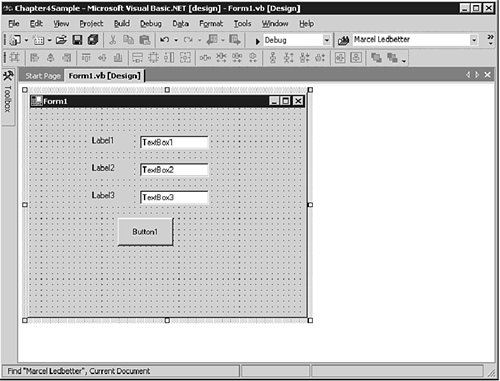 Moving and Sizing Controls To arrange controlson a form, you must move and resize them. These activities are almost second nature to a Visual Basic programmer, but worth mentioning because of some changes in Visual Studio .NET. To demonstrate, single-click the topmost textbox on the sample form. Notice the dashed line that appears at the outer edge of the textbox, indicating that it is the selected control. After you have selected the control, try pressing the arrow keys on your keyboard while holding down the Ctrl key. Notice that the control moves around on the screen, providing a more precise control of movement than the mouse. This is a new feature in Visual Studio .NET. (You can also move controls in the traditional manner as we did in Chapter 2 by clicking and dragging with the mouse.) When you move a control with the mouse, its default behavior is to align itself with the grid of dots displayed on the background of the form. (This grid is not displayed when your program is running, but instead is merely a design aid.) Often developers will want to position objects with more or less more precision than that provided by the default grid settings. Even in previous versions of Visual Basic, developers have been able to change the size of the grid or disable the grid to allow free-form control alignment. However, a great new addition to this version of Visual Studio is the ability to set these options on a per-form basis! This is accomplished through the Form Properties window. To change the grid settings, first select the form by single-clicking in an area that is on the form but outside a control. Next, press F4 to display the Properties window and find the GridSize property. The default setting of 8 pixels between dots provides a lot of precision, but the setting can be changed as low as 2x2 if desired. Another property, SnaptoGrid, determines whether or not the controls automatically align themselves with the grid. Note The Windows Forms Designer options folder (under the Tools, Options menu) lets you set the grid size for new forms. Even though the grid size can be set on a per-form basis, you may want to set this option to specify a default size for new forms. In addition to changing a control's position, you will usually end up changing either its height or width to make it look the way you want. You can resize controls using the keyboard arrows in combination with the Ctrl and Shift keys, or by dragging the mouse. To demonstrate keyboard resizing, first single-click any text box on our sample form. Next, press either the right or left arrow key while simultaneously holding down the Shift and Ctrl keys to change the width of the text box. Before we leave the topic of resizing controls, let's take a closer look at what happens when you select a control. Figure 4.12 shows our sample form with the TextBox1 control selected. Figure 4.12. When sizing objects in a Designer window, the color of the sizing boxes indicates the directions in which they can be resized. 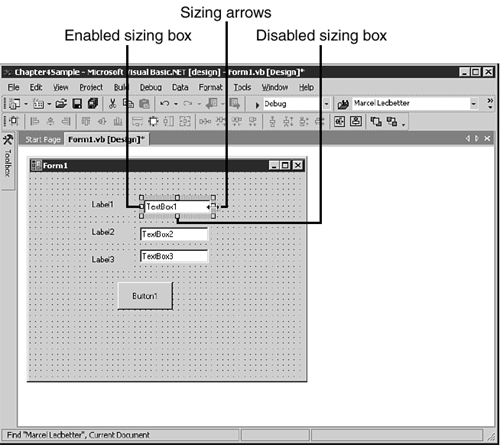 Note that some of the boxes around the edge of the text box are white in the center while others are gray. This is a new feature in Visual Studio .NET that indicates whether or not to resizing is allowed in a particular dimension. When you position the mouse over an active sizing box, the pointer will change to arrows pointing in just two directions. Hold the mouse button down and drag the mouse to resize the object in those directions. In the case of our text box, the MultiLine property is set to False so the TextBox control can only be resized in the horizontal direction. To demonstrate how the sizing boxes are affected by this property, press F4 to display the Properties window. Find the MultiLine property in the list and change its value to True. Notice that the sizing boxes now all appear white, and positioning the mouse over them changes the mouse cursor to the appropriate resize arrows. Working with Multiple Controls As you already know, before you can change something about a control, you have to select the control. Thus far we have selected controls on a form by single-clicking the desired control with the mouse. However, there are times when you will need to make changes to a group of controls at once and therefore need to select a group of controls. Selecting multiple controls requires a little more dexterity, but is still accomplished very easily within the Visual Studio environment. There are two basic methods of doing this: Hold down the Shift or Control key and single-click each control with the mouse that you want to include in the control group. To remove a control from your group, simply click it again. Using the mouse pointer, draw a box that touches all the controls you want to include in the group. To do this, click an empty area of the form and drag the mouse. A box with dashed edges will appear. When you release the mouse button, any controls within the box's boundaries will be included in your control group. Go ahead and create a control group that includes all of the textboxes on our sample form. Notice that selection boxes appear around all of the controls, as pictured in Figure 4.13. Figure 4.13. When selecting a group of controls, the primary control in the group has sizing boxes of a different color. 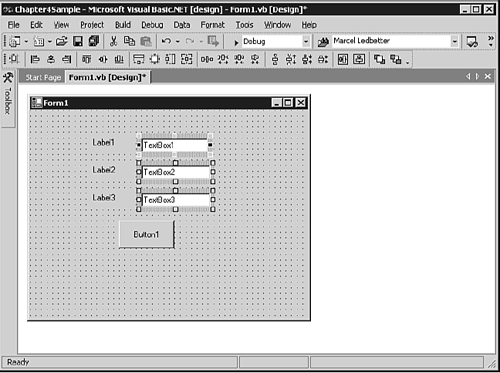 After you have selected a group of controls, you can move or resize them at the same time. However, even more useful is the ability to set common control properties. In the case of TextBox controls, developers frequently want them to appear empty at program startup. Let's clear the text from our textboxes by clearing the text in the Text property. First, press the F4 button to display the Properties window. Note that when displaying the Properties window for a group, no control name is shown and some of the properties are missing. Find the Text property in the list and place the cursor in the value column. Press the Spacebar and then the Enter key. The contents of all three textboxes will be cleared. Using the Format Menu If you have a lot of controls on a form that are haphazardly arranged, your form will look messy. To arrange your controls you could just move and size them with the mouse so that they visually look similar. However, Visual Studio provides the Format menu to make this process easier. Before using most functions on the Format menu, you first have to select a group of controls. Let's call one of the controls in a group the primary control. By using the options on the Format menu, you can make the other controls in the group change to look like the primary control. Note The primary control in a group of controls can be selected by single-clicking the desired control after selecting the rest of the group. The format menu provides the following options for working with groups of controls: Align Use these options to make all the selected controls have the same relative positions on the grid. Make Same Size Causes every control in the group to take on the same height, width, or both. Spacing Changes the amount of empty form space between each control in a group, or removes it all together. Center in Form Centers the selected controls on the form. The final two options on the Format menu apply to individual controls, as well as control groups. Order refers to the layering of controls. In other words, if you have two controls drawn on top of each other, use the options in this submenu to determine which one shows up on top. The Lock Controls option can be used to prevent you from accidentally moving or sizing controls on a form. By selecting a control and choosing this option, the sizing/movement indicator will change and you will be unable to move the control until you have unlocked by clicking the option again. In this section we have discussed arranging controls during form design. To learn more about control alignment during program execution, please p. 357 Working with Invisible Controls We have seen how controls such as textboxes can be arranged on a form. However, there are some controls that the developer cannot position, such as the Timer control. The Timer control has been included in many previous versions of Visual Basic. Like other controls, it provides a piece of preprogrammed functionality you can use in your applications. However, it has no user interface when a program is running and is therefore invisible to the user. No matter where you try to draw them, invisible controls will be placed at the bottom of the Designer window, as shown in Figure 4.14. Figure 4.14. Staring with Visual Studio .NET, Windows controls that are only visible at design time are not drawn on the form itself. 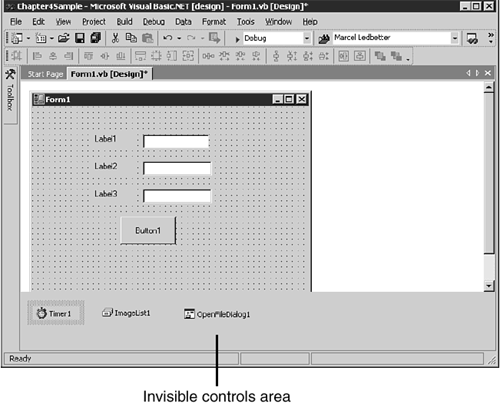 For more information about the use of the timer control, p. 309 Special Considerations for the Web Throughout this section, all of our examples have been using Windows applications. However, Visual Studio .NET also allows you to design Web applications in much the same manner. Instead of using a Windows form designer window, you use a Web form designer window to place and arrange controls. Many of the features and principles we have discussed in this section also apply to Web forms, but often in a more limited or slightly different fashion. The main reason for these limitations is simply the nature of the Web itself. Windows applications are ultimately compiled into executable code and all the user interface elements the operating system has to offer; but Web applications are restricted to a standard group of HTML elements that must be rendered by a remote user's Internet browser. The degree of control available to the developer is just not the same. Because of this, we have devoted an entire chapter to building Web applications. Note The Web designer allows you to select a group of controls, but you must surround them with the selection box rather than just touch them. To learn more about designing Web applications, p. 475 In addition to creating a snazzy user interface, you have to make your program actually do something by adding Visual Basic code. For example, in Chapter 2, you wrote code that took values from textboxes and used them to make a sample loan calculator program work. In the example, you typed Visual Basic code into Visual Studio's Code Editor. The Code Editor works much like a word processing program in that you just type text into it. However, instead of checking your spelling and grammar, the Visual Basic Code Editor checks to make sure you have entered syntactically correct code statements. In this section, we will discuss some special features you will find useful when entering code. To review the loan calculator sample application, please p. 21 To provide an example that we can refer to from the text, you will need to create a sample application. Using knowledge you have acquired from Chapter 2 and earlier in this chapter, perform the following tasks: -
Start Visual Studio and create a new Windows Application project. -
Place a Button control on the form. -
Double-click the Button control to display the code window for the form. -
In the Click event for Button1, enter the following two lines of code exactly: 'The following is a silly sample program! MessageBox.Show("The current date and time is " & Now) Your screen should look similar to the one shown in Figure 4.15. Figure 4.15. The Visual Basic .NET Code Editor not only gives you access to your own code, but also the code generated by the form designer used to create your forms and controls. 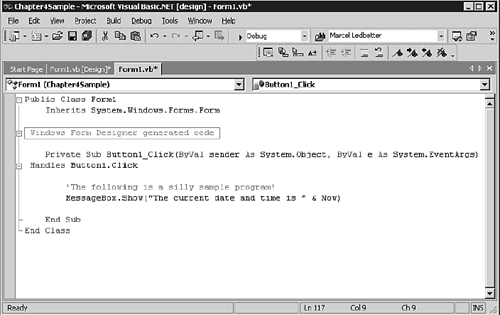 Note One of the ways the Code Editor helps make the coding task easier is by providing a feature called Intellisense. As you type your code, you will be presented with lists of function parameters and object properties so you won't have to remember them. By pressing Ctrl+Space you can have Visual Studio attempt to guess a partial word you have typed. Navigating to Specific Functions The code behind a form is really just one big file. To open it without any specific point in mind, you can right-click the form in the Solution Explorer and choose View Code. However, many times you will want to navigate to a specific point inside the Code Editor. To create the sample Click event procedure shown in Figure 4.15, you used one of the most common ways to navigate inside the Code Editor window double-clicking a control. Double-clicking a Button control takes you to the Button's Click event procedure. Similarly, double-clicking another type of control will take you to one of its many events. If an event procedure does not exist, a stub procedure is created so you can enter new code. However, this method is not always efficient because you may want to access some of the other events associated with a control, or add subroutines that aren't associated with any control. To navigate to the code associated with specific objects on your form, you use the two dropdown boxes at the top of the Code Editor window. Use the drop-down box on the left to pick the class name and the box on the right to pick the method name. To further understand how these drop-down boxes work, look again at Figure 4.15. The selected class is Form1 and the selected method is Button1_Click. As you can see from the way the code is arranged in the Code Editor window, the Button1_Click subroutine procedure is completely contained within the declaration of the form, which begins with Public Class Form1 and ends with the End Class statement. The hierarchical nature of the code in Visual Basic .NET forms may cause some initial confusion for existing VB programmers. However, these code navigation boxes operate in much the same way they always have. Simply pick the object you want to work with on the left, and the event you want to work with on the right. Visual Basic .NET's more object-oriented approach to code layout actually makes sense when you think about it; your forms are classes that contain other classes, such as textboxes and buttons. Note To see the list of all events for the form, including those for which you have not written code, select (Base Class Events) from the class name box. Note During informal discussions it is accepted practice to use the terms object and class interchangeably. However, it is important to understand the distinction. A class is like a template. Objects are created based on a class and objects of the same class behave the same way. To learn more about objects and classes, please see Chapter 5, "Visual Basic Building Blocks." To access other classes contained within the Form1 class, such as controls you have placed on the form, expand the class drop-down box and select the appropriate class name. For example, go ahead and select the Button1 class. Note The icons in the class drop-down box indicate the accessibility of the class. For example, the icon for private classes includes a padlock. Whenever a class is selected, you can expand the Method drop-down box to display available methods for the selected object. The Method box in Figure 4.16 shows some of the methods associated with the Button class. Figure 4.16. In a method listing, a boldface method name indicates that code has been written for that method. 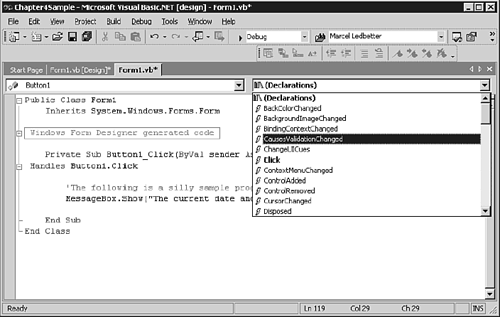 Notice that Visual Studio .NET also includes an icon next to the name of the method. The lightning bolt icons indicate the method is an event procedure. Searching for Code If you have used a Web site search engine, you are familiar with the concept of searching for information by entering a search word. Similarly, if you are looking for a specific variable or function name in your Visual Basic code, there are several ways to get to it. Visual Basic's Edit menu, located under the Find and Replace submenu, contains commands for doing just that. However, serious Visual Basic programmers will want to commit the most important search-related shortcut keys to memory (see Table 4.1). Table 4.1. Shortcut Keys for Finding Code Keystroke | Purpose |
---|
Ctrl+F | Initiate a search | F3 | Repeat a search | Ctrl+H | Find and replace | Pressing Ctrl+F will display the Find dialog box, which is pictured in Figure 4.17. Figure 4.17. In Visual Studio .NET, regular expressions and wildcard characters can be used when searching your code. 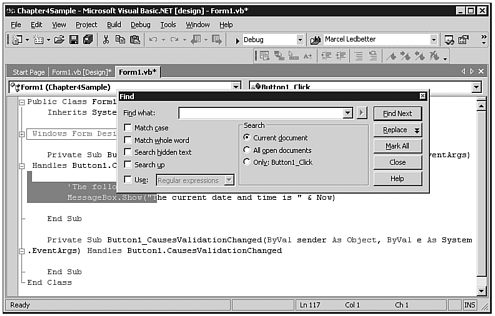 Basic searching for a specific word in your code is easy; just type the word in the Find What box and press Enter. To repeatedly search for the same text, press the F3 key. If the text is found, it will be selected in the Code Editor window. Note A drop-down box on the main toolbar also keeps track of your recent searches and can be accessed without displaying the Find dialog box. This type of searching capability should be familiar to users of previous editions of Visual Basic. However, Visual Studio .NET adds several new powerful capabilities. One of these new features is the addition of wildcards and regular expressions, which allow you to search for a pattern of characters, not just a specific character. To tell Visual Basic the desired pattern, you create an expression by combining normal letters, numbers, and special characters. To demonstrate, let's perform wildcard and regular expression searches on our sample project. Press Ctrl+F to display the Find dialog box, and enter the following text: Button?_Click Next, click the Use check box and select Wildcards from the drop-down list. (This instructs the search engine to evaluate expressions rather than searching for just the literal text.) Click the Find Next button, and notice that the text Button1_Click is highlighted in your code. This is because the 1 matched the question mark in the wildcard expression. As you might have guessed, the question mark (?) is used to represent any single character in an expression. If your code contained a Click event for Button2, the previous search would have found that code as well. (Note that Button10 would not be found by the preceding wildcard expression, because the question mark is used to indicate only a single character. To find one or more characters with a wildcard, use an asterisk [*] instead.) Note A printable list of regular expressions and wildcards, with examples of their use, can be found in the help files. Regular expressions work like wildcards but are a lot more powerful. Visual Studio .NET supports a large number of regular expression characters that can be used to build complex patterns. For demonstration purposes, suppose we want to find code where the letters s or l occur together, but only when followed by an a or o. First, press Ctrl+F to display the Find dialog box, and enter the following characters in the Find What box: [l|s]^2[a|o] Click the Use check box and choose Regular Expressions from the drop-down list. Then, click the Find Next button repeatedly. Notice that the search finds only the llo in following and the ssa in MessageBox. Starting from right to left, the sample regular expression has three main parts. The first part, enclosed by brackets, indicates a group of letters to find, either an l or an s. The second part, ^2, means only match when the previous expression occurs two times. The rightmost part of the expression is another bracketed letter group that includes the letters a and o. Because the last part includes only these two letters, the search does not find the lly in silly. Note Regular expressions have been a mainstay of the UNIX community for years. Tutorials and examples to help you understand them can be found on the Internet. Marking Code with Bookmarks Another button you may have noticed on the Find dialog is the Mark All button, which is used to create bookmarks in your code. Bookmarks in the Code Editor can be used to mark text that you want to refer to at a later time, similar to bookmarks in real life. Note Bookmarks are different than breakpoints, which have meaning during the debugging process and are discussed in a later chapter. When you perform a search and click the Mark All button, a bookmark is placed on each line of code that matches the search. Bookmarks are represented by small blue squares in the margin of the Code Editor. Figure 4.18 shows the results of adding bookmarks to all lines of code with the word End in them. Figure 4.18. Bookmark navigation commands can be found in the Bookmarks submenu under the Edit menu or on the Bookmarks toolbar. 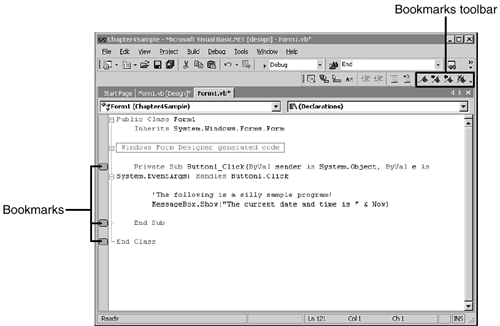 The easiest way to jump from bookmark to bookmark is by using the Bookmarks toolbar, also pictured in Figure 4.18. Note The Find command is not the only way to add bookmarks. You can add them manually with the toolbar, or add a task list shortcut as described later in this chapter. Copying and Pasting Code There are some shortcut keys that every Windows developer should know (see Table 4.2). Although some might argue the most important of these is the key used to undo mistakes, the Cut, Copy, and Paste keys are equally important. Table 4.2. Keys to Memorize Keystroke | Purpose |
---|
Ctrl+X | Cut selected text | Ctrl+C | Copy selected text | Ctrl+V | Paste selected text | Ctrl+Z | Undo a mistake | These keystrokes are probably already second nature to you because they are shared across a variety of Windows applications. However, Visual Studio .NET adds a new concept to the cut and paste world you know and love: the Clipboard Ring. Traditional copy and paste operations allow for a single item in the buffer. However, Visual Studio .NET keeps track of each piece of text you copy in an area of the Toolbox called the Clipboard Ring, pictured in Figure 4.19. Figure 4.19. You can drag code snippets from the Toolbox to the Code Editor with the mouse. 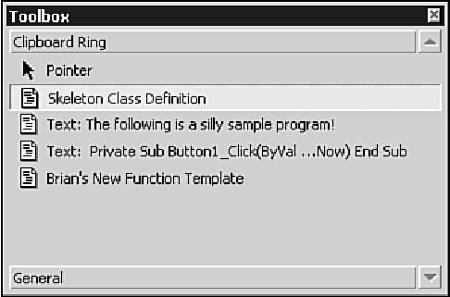 The easiest way to use the Clipboard Ring is to press the Shift key when you paste text. By repeatedly pressing Ctrl+Shift+V instead of the familiar Ctrl+V, you can cycle through text that you have recently copied to the clipboard. By default, text displayed in the Clipboard Ring is just displayed as with the word Text followed by a few characters of the actual text. By right-clicking one of these items, you can name it. For example, the clipboard item called Brian's New Function Template might contain a standard function template that I can use repeatedly by dragging it to the Code Editor with the mouse. Note The Clipboard Ring is temporary; items will disappear from it when you exit Visual Studio. Using the Task List One of the exciting new Visual Studio .NET features is the addition of the Task List window. The Task List window simplifies coding by consolidating errors and other programming tasks into a centralized list. In previous versions of Visual Basic, typing errors in your code triggered nasty message boxes that interrupted the flow of coding, sometimes even after you realized the error and were trying to correct it. Visual Studio .NET's syntax error checking is much less intrusive and operates in the background, very similarly to the automatic spell check feature of Microsoft Word. Although these errors are indicated in your code with wavy lines, they are also placed on the Task List. Clicking on an error in the Task List automatically opens the appropriate code window and jumps to the associated line of code. In addition to tracking your errors, the Task List can also track important sections of code that represent future to-do items or other important project notes. Viewing the Task List To view the Task List window, choose Show Tasks from the View menu and select one of the options. Each option in the menu represents a different view of the project's current tasks. For example, errors are added automatically to the task list by Visual Basic, but you may also add manual comments. Depending on what you are working on, you might want to temporarily hide some of the tasks. Table 4.3 describes the options in the Task List menu and how they affect the subset of tasks that is displayed. Table 4.3. Task List Options Option | Tasks Displayed |
---|
All | All types of open tasks | Comment | Tasks generated from program comments | Build Errors | Errors reported from the compiler | User | Tasks you have manually entered | Shortcut | Shortcuts to lines of code | Additional filters can be applied in combination with the preceding ones, such as the following: Current File Restricts tasks to current code file only Checked If user tasks are shown, includes checked tasks only Unchecked If user tasks are shown, includes unchecked tasks only Note The word filtered will appear in the Task List window caption if you are not displaying all tasks. The Task List, as shown in Figure 4.20, displays several columns of information. By clicking on the gray column headers you can sort the Task List as desired. From left to right, these columns are as follows: Figure 4.20. The Task List provides centralized management of your errors, to-do items, and bookmarks. 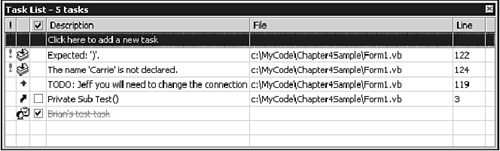 Priority You can set this to high, medium, or low, and an appropriate icon will be displayed. Type A type icon indicates the type of task: a user task, a program error, a shortcut, or a comment. Checked You can check user tasks to provide a visual indicator that they have been completed. Description Provides a brief description of the task. File The path to the file pertaining to the task, if applicable. Line The line of code pertaining to the task, if applicable. Understanding the Different Types of Tasks  | Errors are added and removed from the Task List by Visual Basic. Seasoned VB users will appreciate the fact that they can hurriedly type lines of code without being interrupted by an in-your-face message box with every typo. A quick glance at the Task List allows you to jump to and correct any errors on your own schedule. |  | Comment tasks are tasks that Visual Basic generates after scanning the comments in your program. If your comments contain certain key words such as TODO or HACK, the comment is added to the Task List. When you send code to someone else, adding a TODO comment is a good way to inform him of any changes he needs to make to get the code to work on his PC. Removing the TODO from the comment will remove it from the Task List. | Note If you want to store different types of comment-related tasks in the Task List, you can change the key words by choosing Options from the Tools menu and selecting Task List from the Environment folder.  | User tasks are a type of task that you manually enter. An example of such a task might be "Send a new copy of this executable to Marge" or a task that does not have a line of code associated with it. To enter a new task, just single-click the words "Click here to add a new task" and type a description. You can also set a priority, or click in the check mark column to indicate that the task has been completed. To delete user tasks from the list, single-click the task and press the Delete key on your keyboard. |  | Shortcut tasks are exactly what they sound like: shortcuts to a specific line of code. For example, if you are working with a particularly large project over a period of several days, you can mark a section of code you want to revisit. Just choose the Edit menu, the Bookmarks submenu, and click Add Task List Shortcut (You can also get to this menu by right-clicking in the Code Editor.) When you select this option, the line of code will be added to the Task List. | Using Dynamic Help Help in Visual Basic has always been just a click away, provided you have the help files installed or accessible on CD. The easiest way to access help is to click what you need help with and press the F1 key to ask the system for help. You can click objects, windows, or even words in the Code Editor. Pressing F1 causes a list of appropriate topics to be displayed. In addition, all the search capabilities of the MSDN library (Microsoft Developer's Network) have been integrated into Visual Studio. Several different types of help windows can be displayed in Visual Studio such as Contents, Search, and Index. By using the mouse and docking techniques described earlier, you can position each of these windows as desired. These Help windows can also be separated by tabs within a single window for easy access, as shown in Figure 4.21. Figure 4.21. All of the Help windows pictured here are accessible from the Help menu or via shortcut keys and can be arranged any way you like. 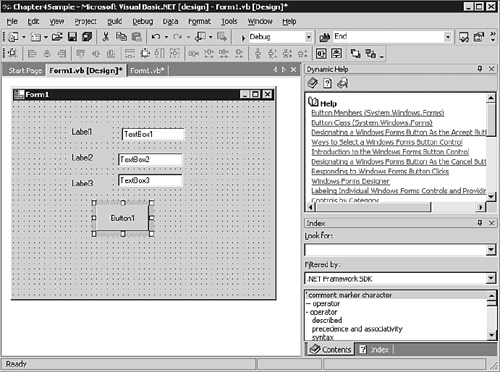 Although readers are probably familiar with searching a help index or reading a table of contents, Visual Studio .NET's new Dynamic Help feature merits additional explanation. Dynamic Help works silently in the background (much like the Task List) to provide the developer with continuous assistance. In the example shown in Figure 4.21, the developer has drawn a Button control on a Windows form. The Dynamic Help window automatically updated itself with information pertaining to buttons, control creation, and other related topics. Clicking on one of these links opens the selected article within the Help files. Tip The Help files will often include sample code, which you can easily copy and paste back into your own projects. If you are new a new user of Visual Studio .NET, I suggest keeping the Dynamic Help window visible while you work. If you see a topic that interests you, simply click on the hyperlink to read more about it. Other Useful Windows in the IDE In this chapter we have described some of the most important windows that you will be using as you develop applications with Visual Basic .NET. However, there are many additional windows available that you will encounter as you develop applications. Because these windows are generally more specialized and require knowledge of coding practices, they are covered later in the book. However, the following is a quick review, along with reference to chapters where they are covered in more detail: The Object Browser is used to explore the properties and methods of objects. It can be accessed by pressing F2. For more detailed information, see Chapter 5. Internet-related windows such as Internet Explorer and the Favorites window should be familiar to users of the Internet. Debug-related windows such as the Immediate window, Watch window, and Command window are used to trace and troubleshoot your programs. |