5.1. Create a Login Page Using the New Security Controls In ASP.NET 1.x, you can use form-based authentication to authenticate web users through a custom login page. While this is a useful and straightforward technique, it still requires you to write your own code to perform the authentication, most often through the use of a data store such as SQL Server. However, this mundane task has now been simplified by the introduction of new security controls in ASP.NET 2.0. 5.1.1. How do I do that? In this lab, you will build a login page that employs the built-in security controls that ship with ASP.NET 2.0 to authenticate users. You will use the various new Login controlsin particular, Login, LoginView, LoginStatus, and LoginNameto help you accomplish tasks such as user authentication, display of login status and login name, and more. You will create two forms: one to display the login status of the user and another to allow the user to log into your application. Note: Using the various Login controls, you can now perform user authentication with ease! The Web Forms created in this lab will lay the groundwork for the next lab, Section 5.2, which will add users to the site for you to test the security controls. First, we'll create a new site to use as a test bed for the new security controls. Launch Visual Studio 2005 and create a new web site project. Name the project C:\ASPNET20\chap-5-SecurityControls. You'll find the security controls you'll be using in this chapter in the Toolbox, under the Login tab, as shown in Figure 5-1. Figure 5-1. The security controls in ASP.NET 2.0 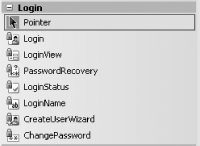
Populate the default Web Form with the control shown in Figure 5-2. The LoginView control is a container that displays different information depending on whether the user is logged in or not. This page will be used to display the status of the user (whether she is logged in or not). This Default.aspx form will be used to display the login status of a user. Figure 5-2. The LoginView control 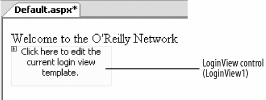
Configure the LoginView control by clicking on it and selecting the AnonymousTemplate views in the LoginView Tasks menu (see Figure 5-3). Type the following text into the LoginView control: "You must Login to the O'Reilly Network to post a talkback." The Anonymous view displays this content when a user is not yet authenticated. Figure 5-3. Configuring the LoginView control for Anonymous view 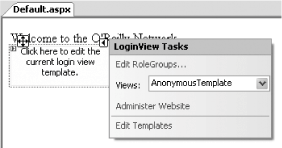
Drag and drop a LoginStatus control onto the LoginView control, as shown in Figure 5-4. The LoginStatus control displays a hyperlink that shows "Login" before the user is authenticated and "Logout" when the user is logged in. Using the LoginStatus control, you can let the user click on the link to either log into or log out of the site. Figure 5-4. Using the LoginStatus control to display login status 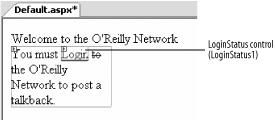
Configure the LoginView control for Logged In View by selecting the LoggedInTemplate views in the LoginView Tasks menu (see Figure 5-3). Type the following text into the LoginView control: "You are logged in as." The LoggedIn view displays this content when a user is authenticated. Drag and drop a LoginName control and an additional LoginStatus control onto the LoginView control in Default.aspx, as shown in Figure 5-5. When a user logs into the site, the LoginName control will display the user's name that was used to log into the application. The user will also be able to log out of the site by clicking on the LoginStatus control. Figure 5-5. Using the LoginName control to display a username 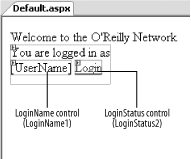
Add a new Web Form to the project and name it Login.aspx. This form will be used to let users log into the site. Drag and drop a Login control (see Figure 5-6) onto the Login.aspx Web Form. The Login control provides user interface elements for logging into a web site. Figure 5-6. Add the Login control to the Web Form 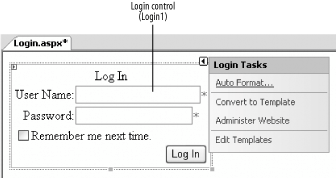
Default Login Page in ASP.NET 2.0 Note that in ASP.NET 2.0, the default login page is named Login.aspx (this is the default "burned" into ASP.NET 2.0 and can be verified by looking at machine.config.comments). However, if you do wish to use a different name for your login page, you can modify the Web.config file by adding the following lines (shown in bold). This will change the authentication mode from the default Login.aspx to Authenticate.aspx: <system.web> <authentication mode="Forms"> <forms name=".ASPXAUTH" loginUrl="Authenticate.aspx" protection="Validation" timeout="999999" /> </authentication> ... Here are the attributes of the <forms> element:
- name
-
Specifies the name of the HTTP cookie to use for authentication
- loginUrl
-
Specifies the name of the page to use for login
- protection
-
Specifies the type of encryption, if any, to use for cookies
- timeout
-
Specifies the amount of time, in integer minutes, after which the cookie expires |
Note that ASP.NET forms authentication does not perform any type of encryption on the credentials that are submitted via the login page, so any login page should be protected by SSL encryption so that credentials can't be stolen. Tip: To learn how to configure SSL on IIS, check out http://www.microsoft.com/resources/documentation/iis/6/all/proddocs/en-us/nntp_sec_securing_connections.mspx.
It's time to dress up the Login control. Apply the Elegant scheme to the control by clicking on the Auto Format... link in the Login Tasks menu. Your Login control should now look like the one shown in Figure 5-7. Figure 5-7. Applying autoformat to the Login control 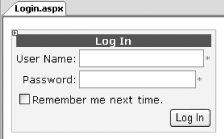
Add a Web.config file to your project (right-click the project name in Solution Explorer and select Add New Item..., and then select Web Configuration File). In Web.config, change the authentication mode from Windows to Forms by adding the following line of code. You use forms authentication so that you can add users to your web site without needing to create the user accounts in Windows: <system.web> <authentication mode="Forms"/> You are now ready to test your application. But before you do that, you have to add a user to your project. You will see how to do this in the next lab. Tip: You should set the DestinationPageUrl property of the Login control to one of your sites pages so that if the Login.aspx page is loaded directly, it can direct the user to a default page once the user is authenticated. (The DestinationPageUrl property applies only when Login.aspx is loaded directly.)
5.1.2. How does it work? ASP.NET 2.0 uses a new security model known as the Membership Provider Model. The Provider Model allows for maximum flexibility and extensibility by enabling developers to choose the way they add security features to their applications. As an example of the extensibility of the Provider Model, consider the new set of Login controls, some of which you've put to work in this lab. The controls, APIs, and providers that make up this new model are shown in Figure 5-8. Figure 5-8. The Membership Provider Model 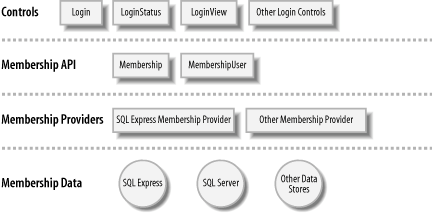 At the top level are the various web server controls, such as the Login, LoginStatus, and LoginView controls. Underlying the controls are the APIs that perform the work required to implement their functionality. The Membership class takes care of tasks such as adding and deleting users, while the MembershipUser class is responsible for managing users' information, such as passwords, password questions, and so on. The Membership APIs use the Membership Providers to saveor persist, in today's jargonuser information in data stores. Visual Studio 2005 ships with one default Membership Provider: the SQL Express 2005 Membership Provider. The role of the Membership Provider is to act as a bridge between the Membership APIs and the data stores, so that information can be persisted without requiring a developer to write the low-level code needed to access data. If the provider supplied by Microsoft does not meet your needs, you can either extend them or simply write your own. For instance, if you want to save the membership information for your site in an XML document rather than a relational database (such as SQL Server), you can write your own provider to talk to the XML file. 5.1.3. What about... ...authenticating users without the use of cookies? Forms authentication in ASP.NET 1.x uses an HTTP cookie to store the encrypted username. In ASP.NET 2.0, it now supports an additional mode of forms authentication: cookieless forms authentication. To use cookieless forms authentication, you just need to set the <cookieless> attribute within the Web.config file: <authentication mode="Forms"> <forms name=".ASPXAUTH" loginUrl="Login.aspx" protection="Validation" timeout="999999" cookieless="UseUri"/> </authentication> The cookieless attribute supports four modes:
- AutoDetect
-
Automatically detects whether the browser supports cookies and will automatically use cookies if the browser supports them
- UseCookies
-
Uses cookies for forms authentication (default behavior)
- UseDeviceProfile
-
Uses cookies (or not) based on device profile settings in machine.config
- UseUri
-
Uses cookieless forms authentication through the URL Note: If you use cookieless forms authentication, you will notice a long string of text embedded within your URL. ...configuring the LoginView control in Source View? If you do not like using the LoginView wizard, you can switch to Source View and manually add the attributes you need to configure the control. Here is the source view of the LoginView control that will achieve the same result as discussed earlier: <asp:LoginView runat="server"> <LoggedInTemplate> You are logged in as <asp:LoginName runat="server" /> <asp:LoginStatus runat="server" /> </LoggedInTemplate> <AnonymousTemplate> You must <asp:LoginStatus runat="server" /> to the O'Reilly Network to post a talkback. </AnonymousTemplate> </asp:LoginView> 5.1.4. Where can I learn more? For more information about the various parameters in the <forms> element in Web.config, check out the MSDN Help topic "<forms> Element." For an overview of the Membership feature in ASP.NET 2.0, check out the MSDN Help topic "Introduction to Membership." |