5.2. Add Users with WAT Once you have set up your site to authenticate users, you need a way to add user accounts to your site. In ASP.NET 2.0, you can do this by using the ASP.NET Web Site Administration Tool (WAT). The user accounts you add will be stored in a SQL Server 2005 Express database by default (located under the App_Data folder in your project), as specified by the default Membership Provider. There is no longer any need for you to create your own custom databases for this purpose or to store the user account information in Web.config (which is insecure). Note: The ASP.NET Web Application Administration Tool (WAT) will help you create new users for your web site and then store the users' information in the database. 5.2.1. How do I do that? In this lab, you will use the ASP.NET WAT to add new user accounts to your web application. The user accounts will be stored in the database specified by the Membership Provider (see the Section 5.1.2 of Section 5.1). By default, the WAT adds new users to a SQL 2005 Express database. Open the project created in the last lab (C:\ASPNET20\chap-5-SecurityControls). To add a new user to your web site, you will use the ASP.NET Web Site Administration Tool (found in the Website ASP.NET Configuration menu item; see Figure 5-9). Figure 5-9. Launching the ASP.NET Configuration tool 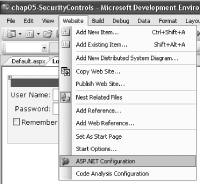
Alternatively, you can invoke the ASP.NET WAT by clicking on the ASP.NET Configuration icon in Solution Explorer (see Figure 5-10). Figure 5-10. Invoking the ASP.NET Configuration tool in Solution Explorer 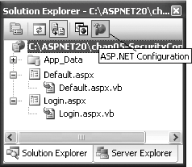
To create a new user, click on the Security tab (see Figure 5-11). Figure 5-11. The ASP.NET Web Site Configuration Tool 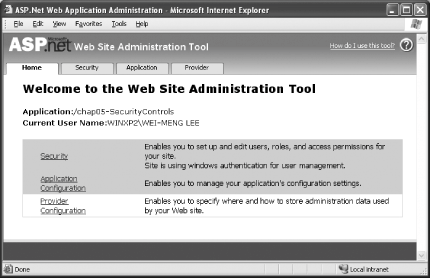
Under the Users group, click on the "Create user" link to create a new user account (see Figure 5-12). Figure 5-12. Creating a new user 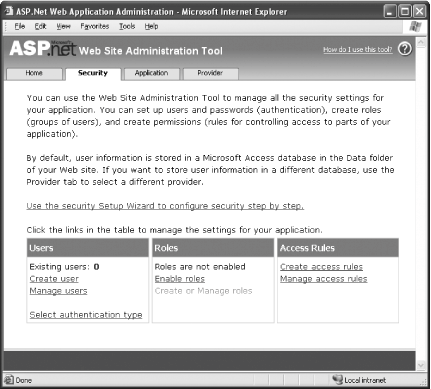
Enter the information needed to create a new user account (see Figure 5-13). Click the Create User button to complete the account creation. Figure 5-13. Entering information for a new user 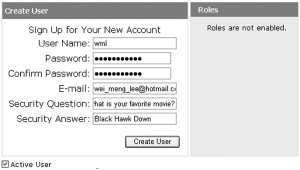
Finally, you are now ready to test drive your application. In Solution Explorer, select Default.aspx and press F5. You should see the screen shown in Figure 5-14. Figure 5-14. The opening page: not logged in yet 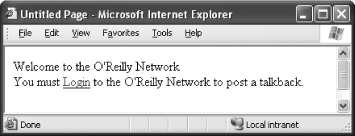
Click on the Login link to go to the Login.aspx page. Enter the account details of the account just created and click Log In (see Figure 5-15). Figure 5-15. Logging in 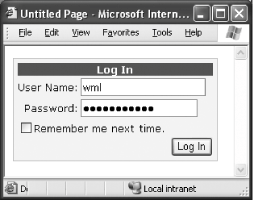
If the account is authenticated, you should see a screen like Figure 5-16. Figure 5-16. User authenticated 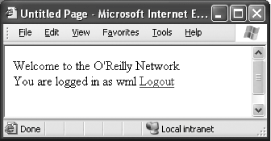 5.2.2. What just happened? When you add a user to your application, the information is saved in two tables in SQL 2005 Express (located in the App_Data folder of the project): aspnet_Membership aspnet_Users Tip: The aspnet_Membership table stores detailed information about a user (such as password, email, last login date, and more), whereas the aspnet_Users table stores information such as the name of the web application to which the user belongs. Tables Table 5-1 and Table 5-2 show the values that are created in the two tables when I create an account for myself. Table 5-1. Content of aspnet_Membership Field | Value |
---|
ApplicationId | {B6677867-19E8-409B-B5CD-9D584B5F5647} | UserId | {03D7299A-2C80-4A3C-B6B7-818369526BEE} | Password | TyPAwmdjkASXxZAn+Kxy3U59qQ0= | PasswordFormat | 1 | PasswordSalt | ZsmfGb76Jflh2+xmxcyXcA== | MobilePIN | | Email | wei_meng_lee@hotmail.com | LoweredEmail | wei_meng_lee@hotmail.com | PasswordQuestion | What is your favorite movie? | PasswordAnswer | Black Hawk Down | IsApproved | 1 | IsLockedOut | 0 | CreateDate | 2/5/2005 6:58:05 PM | LastLoginDate | 2/5/2005 6:58:05 PM | LastPasswordChangedDate | 2/5/2005 6:58:05 PM | LastLockoutDate | 1/1/1753 | FailedPasswordAttemptCount | 0 | FailedPasswordAttemptWindowStart | 1/1/1753 | FailedPasswordAnswerAttemptCount | 0 | FailedPasswordAnswerAttemptWindowStart | 1/1/1753 | Comment | |
Table 5-2. Content of aspnet_Users Field | Value |
---|
ApplicationId | {B6677867-19E8-409B-B5CD-9D584B5F5647} | UserId | {03D7299A-2C80-4A3C-B6B7-818369526BEE} | UserName | wml | LoweredUserName | wml | MobileAlias | | IsAnonymous | 0 | LastActivityDate | 2/5/2005 6:58:05 PM |
Notice that the password has been salt hashed before it is stored in the Password field of the aspnet_Membership table (see Sidebar 1-2). Password Hashing There are many ways to store a user's password in the database. The simplest (and most insecure) way is to store the user's password in clear text. However, doing so will expose all your users' passwords in the event that your database is compromised. A better way would be to encrypt your password (using an encryption key or, better still, with keyless encryption). The best choice would be to apply a one-way function to the password so that no key is needed, reducing the chance that the key would be discovered by a potential hacker. Hashing is a cryptographic algorithm that takes in an input and produces a hash output. It does not require a key and, technically, there is little chance that two different inputs will produce the same hash value. Therefore, the hashed values of passwords are often stored in the database. When a user logs in, the given password is hashed and its value is compared with that of the value stored in the database. If they are the same, the user is authenticated. One pitfall of hashing is that a potential hacker may use a dictionary attack. He may generate a list of hash values of commonly used passwords and then compare them with the hash values stored in the database. Once a match is found, the attacker would know the password of a user. To slow attackers down, a random string of values (known as a salt ) is generated for each user and added to the password before it is hashed. The hashed value and the salt is then stored in the database. The advantage of this is that an attacker will now have to generate a separate list of hash values for each user (taking into consideration the random salt for each user), making it more difficult and time-consuming to get the exact password. Also, using a salted hash, two users having the same password will now have two different hash values, since a random salt is generated for each user. |
5.2.3. What about... ...finding out whether a page is being viewed by an authenticated user? You determine if a page is being viewed by an authenticated user by using the GetUser( ) method from the Membership class. Take the example of the Default.aspx page. You might want to display some customized welcome messages once a user has logged in successfully. You can do that by calling the GetUser( ) method in the Form_Load event-handling code. If the user viewing the page is not logged in, the GetUser( ) method will return null. The following code checks to see if an authenticated user is viewing the page, and if that's the case, it prints out the current time. Protected Sub Page_Load(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles Me.Load If Membership.GetUser IsNot Nothing Then Response.Write("Time is " & Now) End If End Sub ...preventing a potential hacker from trying too many times to guess a user's password? You can limit the number of unsuccessful attempts by setting the passwordAttemptThreshold and passwordAttemptWindow attributes of the Membership Provider in the Web.config file, as shown in Example 5-1. Example 5-1. Limiting password attempts ... <membership defaultProvider="SqlProvider" userIsOnlineTimeWindow="20"> <providers> <add name="SqlProvider" type="System.Web.Security.SqlMembershipProvider" connectionStringName="LocalSqlServer" requiresQuestionAndAnswer="true" passwordAttemptThreshold="5" passwordAttemptWindow="30" applicationName="MyApplication" /> </providers> </membership> Tip: The LocalSqlServer connection string points to the SQL Server 2005 Express database in your project. It is defined in the machine.config.comments file. The code in Example 5-1 limits a user to five password attempts within 30 minutes. If the user exceeds those numbers, her account will be locked. 5.2.4. Where can I learn more? To learn more about how to use salted hashes in your own .NET applications, check out this MSDN article: http://msdn.microsoft.com/msdnmag/issues/03/08/SecurityBriefs/. For more information on the passwordAttemptThreshold and passwordAttemptWindow attributes, check out the MSDN Help topics on "Membership.passwordAttemptThreshold property" and "Membership.passwordAttemptWindow property." |