As should be obvious by now, both the UIObject and UIComponent classes have methods that are inherited by all component instances. In addition, different component types have methods that are unique to themselves. For brevity, we'll only mention a few examples here before moving on to the exercise for this section. Common Methods The following methods are common to all component instances: move(x, y) moves a component instance to the specified x and y coordinates. For example: myButton_pb.move(100, 200); setSize(width, height) resizes a component instance to the specified width and height values. For example: myButton_pb.setSize(250, 150); getFocus() returns a value of the current object that has focus. For example, var myVariable:String = myButton_pb.getFocus(); assigns myVariable a string value representing the name of the component instance that currently has focus. setFocus() sets the focus to a particular component instance. For example, myButton_pb.setFocus() gives focus to the myButton_pb instance. NOTE For more information on what focus means and how it's used, see "Using the FocusManager Component" later in this lesson. There are other methods that are inherited by all instances, but these are the most common. Component-Specific Methods While most components have methods specific to themselves, most of these methods are used to do one of the following: Add something to a component instance, such as a piece of data or a graphic Get (return) information about a component instance; for example, what item is currently selected in a combobox Tell (set) the component instance to do something, such as scroll up or down, or highlight a specific piece of data Sort the component's data in a specific manner NOTE There are too many component-specific methods to list here. For a complete listing of the methods of a component class, look up its entry in the ActionScript dictionary. Component-specific methods can be found under each component listing in the Actions Toolbox section of the Actions panel. In the following exercise, we'll use component methods to dynamically insert, delete, and manipulate the data within our List component as well as to dynamically insert icon graphics. In addition, we'll use component methods to control and communicate with several other component instances. Open Components3.fla. In the preceding exercise, we set up the framework for using component events via Listener objects; however, we didn't script our Listener objects to do anything when events were triggered. In this exercise, we'll insert scripts that cause the application to perform an action when these events occur.
With the Actions panel open and Frame 1 selected, insert the following script just below inputURL_tiListener.focusIn = function () {: deleteURL_pb.enabled = false; openURL_pb.enabled = false; windowOption.enabled = false; enableWindowGraphics(false); addURL_pb.enabled = true; 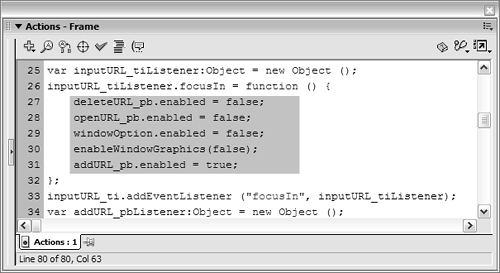 In the preceding exercise, we registered the inputURL_tiListener object to listen for the focusIn event in relation to the inputURL_ti component instance. The script we've just inserted tells the Listener object what to do when this event occurs in relation to that instance. When the user clicks inside the inputURL_ti instance (gives it focus), this script will execute. The purpose of this script is to reset various elements to their initial state. The deleteURL_pb and openURL_pb Button instances are disabled, the radio buttons within the windowOption group are disabled, the enableWindowGraphics() function is called (making the graphics associated with the radio buttons transparent), and the addURL_pb instance is enabled. We're resetting these various elements to their initial states. Other scripts we'll add shortly will change these states as the user interacts with the application; this script places these elements into the appropriate state for inputting a new URL. This will become clearer as we progress. It's important to understand that the addURL_pb button is enabled (as shown in the last line of the script) when the inputURL_ti instance is given focus for inputting a new URL. This occurs because the two instances work in tandem. When typing a URL, the user adds it to the list by clicking the addURL_pb button, requiring that instance to be enabled. We'll script the functionality that adds the URL in a moment, but first let's take a look at some of the items in the library that play an important role in one of the following steps.
Choose Window > Library to open the Library panel. 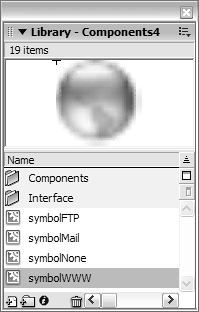 You'll find two folders within the library that contain movie elements, as well as four additional movie clips not contained within a folder. These movie clips represent icon graphics. Here's how they'll be used by our application. When the user enters a URL containing www, not only will that URL be added to the listURL_lb instance, but our application will be scripted to detect that a www address has been entered. The appropriate icon graphic will be shown next to the URL in the list, which in this case would be the movie clip named symbolWWW. If the user enters a URL containing ftp, the symbolFTP movie clip will be used. Entering mailto causes symbolMail to be used. If none of the aforementioned URL types is entered, our app will assume that an errant URL has been added and the symbolNone movie clip will be shown next to that URL. 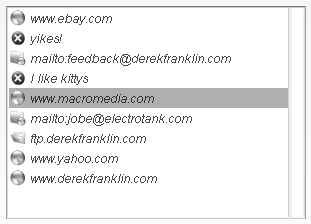 This functionality is made possible as the result of the capability of List component instances to dynamically attach icon graphics, which are nothing more than movie clips that have been given linkage identifier names. Let's look at how one of these movie clips is set up.
Right-click (Control-click on a Macintosh) the symbolWWW movie clip in the library and choose Linkage from the menu that appears. This opens the Linkage Properties dialog box, which shows that this movie clip has been given a linkage name of symbolWWW. (Yes, it's the same name as the movie clip itself; it was done this way for simplicity.) 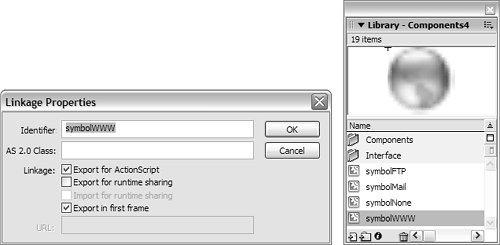 The remaining three movie clips have also been given identifier names representative of their movie clip names. Giving movie clips identifier names allows us to dynamically insert them into our project as it plays, something we'll script next.
Click OK to close the Linkage Properties dialog box. With the Actions panel open, insert the following script just below addURL_pbListener.click = function () {: listURL_lb.enabled = true; listURL_lb.addItemAt (0, inputURL_ti.text); listURL_lb.selectedIndex = 0; listURL_lb.iconFunction = function (item:Object):String { var tempString:String = item.label; if (tempString.indexOf ("www.") >= 0) { return "symbolWWW"; } else if (tempString.indexOf ("mailto:") >= 0) { return "symbolMail"; } else if (tempString.indexOf ("ftp.") >= 0) { return "symbolFTP"; } else { return "symbolNone"; } }; inputURL_ti.text = ""; 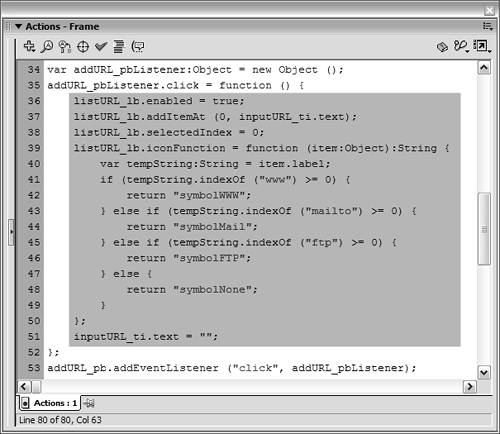 Because this script is inserted within the click handler of the addURL_pbListener object, it gets executed when the addURL_pb instance is clicked. Let's look at how this script works. The first line enables the listURL_lb instance, just in case it has been disabled (such as when the application is initially opened). The next line of script uses the addItemAt() method, which is available to List component instances. This method adds an item (line of information) to the specified List component instance, which in this case is the one named listURL_lb. NOTE A disabled component cannot be manipulated by the user or via ActionScript. To understand how this method works, you need to understand that each item displayed in a List component instance is actually an object with two properties named label and data. The label property holds a value representing the text displayed for the item; the data property is a hidden value associated with that label. For example, if you had a List component that displayed computer parts, the label property could be used to hold a text description of the piece of hardware, while the associated data property could be used to hold the associated part number (hidden from the user) like this: label: Monitor data: Mon359a4 label: Keyboard data: Key4e94f and so on. If the user later selected one of these items from the list, you could use a script to retrieve either the label or data value of the selected item. The data item is the preferred choice because it contains more specific information; the label property is used mostly for readability. With this understanding, let's look at the syntax for using the addItemAt() method: nameOfListInstance.addItemAt(index, label, data); The index parameter indicates where in the list to add the new item, with 0 being the top of the list. The label parameter represents the text you want to display for the new item. The data parameter represents any hidden value you would like to associate with the new item. The data parameter is optional, and omitting it will cause the label and data properties of the newly added item to contain the same value. As you can see in the script we inserted, the method is set up to add a new item to index 0 (which, again, is the topmost position on the list), and the value assigned to the label property of this newly added item is the text currently displayed in the inputURL_ti instance (what the user has entered). Because we're not using the optional third parameter in the method call, the label and data properties contain the same value when the item is added to the list. NOTE Items shown in List component instances have index numbers (beginning with 0), indicating their position in the list. Thus, the first item in the list has an index of 0, the second has an index value of 1, and so on. 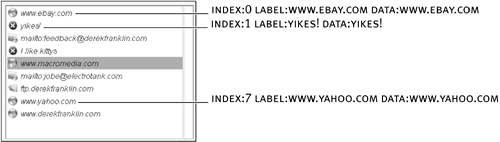 The third line of the script assigns a value to the selectedIndex property of the listURL_lb instance. This will set and highlight the item at index 0, which will always be any newly added item. The next several lines of script set the iconFunction property of the listURL_lb instance. The value of this property specifies a function to execute when a new item is added to the list. This function is used to add an icon to the newly added item by returning a string value, representative of the identifier name of the icon to use (as discussed in Step 3). Let's look at how this function works. When this function is executed, it's passed an object that we've named item. This object has two properties named label and data. The values of these properties are the same as the label and data properties as defined in the addItemAt() method call we just discussed. If our method call looked similar to this: myList.addItemAt(0, "fruit", 47); the object passed to iconFunction would have a label property value of "fruit" and a data property value of 47. The value of one or both of these passed properties is used by the function to determine the name of the icon to attach. The function's first action creates a variable named tempString. This variable is assigned a value based on the label property of the object passed to the function. If the user is adding the URL www.derekfranklin.com, for example, tempString will be assigned that URL as its value. Next, a series of conditional statements is used to determine whether the value contained in tempString includes www., mailto:, ftp., or none of these. As a result, the function will return a string value representing the linkage identifier name of the movie clip in the library to use. 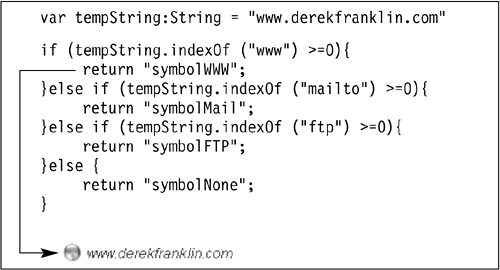 The last line in the script clears the inputURL_ti instance of the URL the user has entered, allowing the user to quickly add another URL. Let's test our project.
Choose Control > Test Movie. When the application appears, click inside the inputURL_ti instance. Notice that the addURL_pb instance immediately becomes enabled, as scripted in Step 2. Enter a URL and click the Add button to enable the listURL_lb URL and add it to the list. The URL is highlighted, the appropriate icon is attached, and the inputURL_ti instance is cleared of text and ready for the next entry, as we scripted in Step 5. Let's return to the authoring environment to add a few more scripts.
Close the test movie to return to the authoring environment. With the Actions panel open and Frame 1 selected, insert the following script just below listURL_lbListener.focusIn = function () {: addURL_pb.enabled = false; deleteURL_pb.enabled = true; openURL_pb.enabled = true; windowOption.enabled = true; enableWindowGraphics(true); 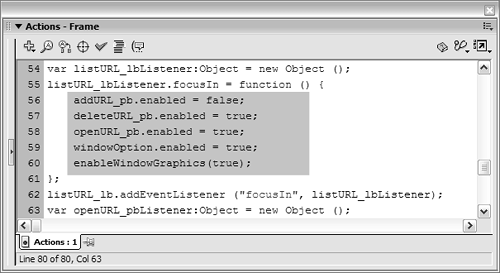 Because this script is inserted within the focusIn handler of the listURL_lbListener object, it gets executed when the listURL_lb instance is given focus (the user interacts with it). This script does nothing more than disable the addURL_pb instance, while enabling the other elements specified within the script. The bottom half of our app comes to life as a result. These elements are enabled because they have a purpose when the user gives focus to the listURL_lb instance (selects a URL from it). Let's test this functionality.
Choose Control > Test Movie. When the application appears, enter a URL and then click the Add button to add the URL to the list. Next, click the URL you just entered in the list. When you do, all the elements in the bottom half of the application become enabled as a result of the script we added in Step 7. These enabled elements don't do anything yet, but that will change in a moment. If you click inside the inputURL_ti instance at this point to add another URL, the script we added in Step 2 is executed, resulting in the Add button being enabled and the elements in the bottom half of the application becoming disabled. This interactivity is all being managed through component events that make creating a responsive application simple and straightforward. Let's return to the authoring environment to script the Delete and Open buttons and wrap up this exercise.
Close the test movie to return to the authoring environment. With the Actions panel open and Frame 1 selected, insert the following script just below openURL_pbListener.click = function () {: getURL (listURL_lb.value, windowOption.getValue ()); Because this script is inserted within the click handler of the openURL_pbListener object, it gets executed when the openURL_pb instance is clicked. As you can see, the getURL() action is used to perform a single task opening the specified URL. Typically, the getURL() action is used in the following manner: getURL("http://www.derekfranklin.com", "_blank"); The first parameter specifies the URL to open; the second specifies the HTML target. Our use of the getURL() action involves using two dynamic values in place of hard-coded values, as shown in this sample script. The URL parameter of the getURL() action is determined by looking at the current value of the listURL_lb instance. This property reflects the currently selected item in the instance. If the currently selected item is http://www.electrotank.com, that's considered the value of the instance. The value of the second parameter of the getURL() action is derived by getting the current value of the windowOption radio button group. You'll remember that in the first exercise of this lesson, we assigned data values to both of the radio button instances in our application, with the top radio button instance being given a data value of _self and the other instance a data value of _blank. We then assigned both of those radio buttons to the windowOption group. Depending on which radio button is currently selected when this getURL() action is executed, the method call of windowOption.getValue() will return a value of either _self or _blank. This determines whether the specified URL is opened in the current browser window or a new one.
NOTE URLs entered containing www must be preceded by http:// for the getURL() action to work properly. Insert the following script just below deleteURL_pbListener.click = function () {: listURL_lb.removeItemAt (listURL_lb.selectedIndex); listURL_lb.selectedIndex = listURL_lb.length - 1; if (listURL_lb.length <= 0) { deleteURL_pb.enabled = false; openURL_pb.enabled = false; windowOption.enabled = false; enableWindowGraphics(false); listURL_lb.enabled = false; } 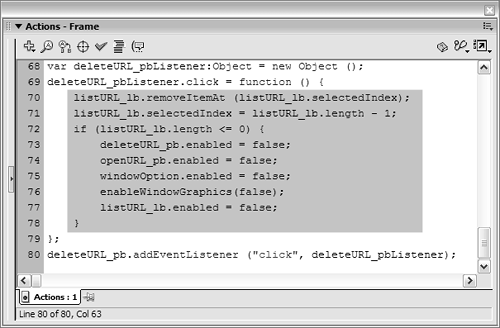 Because this script is inserted within the click handler of the deleteURL_pbListener object, it gets executed when the deleteURL_pb instance is clicked, and takes care of deleting items from the list. The first line of the script uses the removeItemAt() method to remove an item from the list contained in the listURL_lb instance. This method accepts a single parameter, representing the index number of the item to be deleted. In our use of this method, this index value is dynamically determined by retrieving the index of the currently selected item in the list. If the currently selected item in the list is fourth from the top, it will have an index value of 3. As a result, item 3 on the list is deleted. The next line of the script sets the selectedIndex property of the listURL_lb instance to select and highlight the last item in the list after a deletion occurs. The value of this property is dynamically assigned by determining the length of the listURL_lb (how many items it contains) minus 1. If the instance contains five items, for example, after the deleted item is removed the instance length property will be 5 and the item selected will have an index of 4. The extra step of subtracting 1 is done because although the length property is determined with a count starting at 1 (one item in the list means a length of 1), index values start from 0 (the first item in the list has an index of 0). This functionality is added simply from a usability standpoint, allowing the user to continue clicking the Delete button, removing the last item in the list automatically. The last part of this script uses a conditional statement to react in the event that all items in the list have been deleted. If this occurs, the actions within the statement again disable the elements in the application used for managing and navigating URLs, because they have no meaning when no URLs exist. Let's do one final test.
Choose Control > Test Movie. When the application appears, add some URLs; then select a URL and either delete it or open it to see how the application handles these activities. Delete all the URLs from the list to see how the application reacts. Component properties, events, and methods allow you to quickly create useful and responsive applications.
Close the test movie to return to the authoring environment. Save this file as Components4.fla. In the sections that follow, we'll tweak the application's usability and appearance.
|