In user interfaces, it's sometimes useful to add drag-and-drop behaviors to movie clip instances a term that refers to the process of clicking and dragging movie clip instances around the stage, so that when they're released (dropped), they'll perform a set of actions (determined by the location where they're dropped). The easiest way to conceptualize this type of behavior is by thinking of the trashcan on your computer desktop: If you drag a file over the trashcan and let go of it, that file is deleted; however, if you're not over the trashcan when you let go, you've simply moved the file icon. The most common way to create drag-and-drop items in Flash is by using _droptarget or hitTest() . Checking the _droptarget property of a movie will return the path in slash syntax to the highest movie clip instance that the currently dragged movie clip instance is over. Using the hitTest() method, you can determine whether the bounding box of one instance is touching the bounding box of another (for more on this, see Lesson 9, Using Conditional Logic) and take action accordingly. The hitTest() method is used more frequently because it's more versatile than the _droptarget property. In this exercise, you'll extend our project to dynamically create a row of icons (simple graphical movie clip instances) that can be dragged and dropped onto the canvas using hitTest() . When dropped, a copy of the movie clip instance will be created using duplicateMovieClip() and the original will be sent back to its initial position. -
Open the draw3.fla file in the Lesson14/Assets folder. This file is as you left it at the end of the previous exercise. You will add ActionScript to the controller and icon movie clip instances. First, you'll create a function that creates the row of icons below the canvas. Then, you'll add the ActionScript that makes them draggable and the ActionScript that detects if they were dropped onto the canvas. -
With the Actions panel open, select the controller movie clip instance and add this function definition to the load event: function buildIconList () { spacing = 85; iconY = 360; iconX = 70; var i = -1; while (++i < _root.icon._totalFrames) { newName = "icon" + i; _root.icon.duplicateMovieClip(newName, 10000 + i); _root[newName]._x = iconX + i * spacing; _root[newName]._y = iconY; _root[newName].gotoAndStop(i + 1); } } There's nothing in this function you haven't seen before. The icon movie clip instance contains a certain number of frames on its timeline, each of which includes a different icon graphic. This ActionScript will duplicate the icon clip one time for every frame in that movie clip. It will send each duplicated movie clip instance to a unique frame and align it along the bottom of the screen. The result is a row of icons at the bottom of the screen. You can add or remove icons (that is, add or remove frames) in the icon movie clip instance, and this ActionScript loop will adapt based on the _totalFrames property (used in the while loop). The spacing variable represents the vertical space between the duplicated icon. iconY and iconX represent the coordinates of the first duplicate. 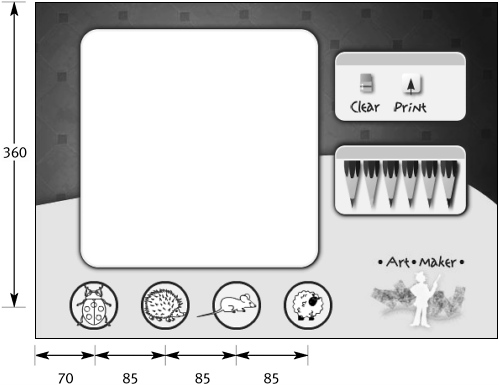 -
Add buildIconList(); to the bottom of the load event. This will call the function you created in the previous step to create the icon list. -
Double-click the icon movie clip instance to edit it in place. With the Actions panel open, select the invisible button and add this script: on (press) { startDrag (this); } As you saw in the previous two steps, the icon movie clip instance (which contains the button to which this script is attached) will be duplicated four times, and each of those duplicates will be moved to one of the four frames on its timeline. This will place four icons (with different graphics) just below the canvas. Each of these icons must be able to be dragged onto the canvas, where on release, a copy (yes, a duplicate of a duplicate) will be placed on the canvas producing the effect of dragging a copy of the icon onto the stage in order to color over it. The invisible button triggers the dragging process when pressed, as you can see by the startDrag() action that's attached to it. It's important to note that this invisible button has an instance name of iconButton. Here's why. When the icon is dropped onto the canvas for duplication, the duplicate will inherit the invisible button as well as the actions it contains. This means that the duplicate (which would appear on the canvas) would also be draggable, which is not what we want. We want the duplicates to remain stationary once they've been dropped onto the canvas). Giving the button an instance name allows you to disable it in duplicates placed on the canvas. The function that handles the drag-and-drop functionality will be set up so that when the duplicate is created, the button inside of it is disabled, preventing it from executing the startDrag() action. This button will only be enabled (the default behavior for all buttons) on the instances of this clip below the canvas which means those instances can be dragged, facilitating the first part of the drag-and-drop functionality. 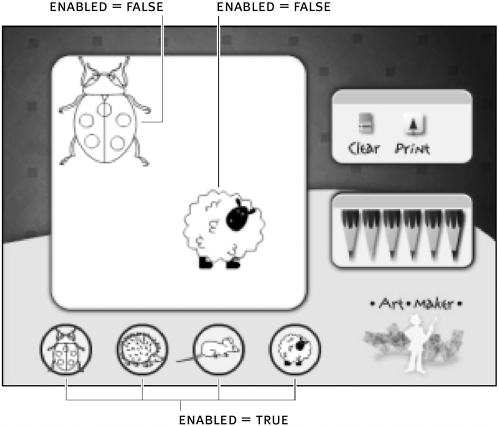 -
With the Actions panel still open, add the following script to the button: on (release) { stopDrag (); _root.controller.iconReleased(_name); } The first action in this script simply stops the drag action when the button is released, making it appear to be "dropped." The second action calls the iconReleased() function (which we have yet to define) on the controller movie clip instance. This function will duplicate the instance that has been dragged onto the canvas and place it back in its original position (just below the canvas), where it's ready to be dragged and dropped again. You'll notice that the function call sends the _name property of the dropped instance to the function: This tells the function which of the four icons has been dragged onto the canvas and thus which one needs to be duplicated. -
Navigate back to the main timeline and select the icon movie clip instance. With the Actions panel open, add the following script: onClipEvent (load) { homeX = _x; homeY = _y; } Using a load event, two variables, homeX and homeY , are created to store the location of the instance when first loaded. Each duplicate will inherit this script so that its original x and y positions are stored on its own timeline using these variable names. Knowing these values will let the icons that are dragged and dropped onto the canvas and duplicated be returned to their original position on the stage, so that they can be dragged and dropped again and again. Now let's set up the function that will bring everything together. -
Select the controller clip and add this function to the load event: function iconReleased (name) { if (_root.canvas.hitTest(_root._xmouse,_root._ymouse)) { ++v; newName="object" + v; _root[name].duplicateMovieClip(newName, v); _root[newName].gotoAndStop(_root[name]._currentFrame); _root[newName].iconButton.enabled = false; root[newName]._xscale = 250; _root[newName]._yscale = 250; } _root[name]._x = _root[name].homeX; _root[name]._y = _root[name].homeY; } This function is set up to receive one parameter, which is identified as name . As described in Step 5, this will be the instance name (_name property) of the icon that is dragged and dropped onto the canvas. The value of this parameter plays an important role in how the rest of the function works. First, a conditional statement checks to see if the mouse is over the canvas movie clip instance when the function is called, indicating that an icon has actually been dropped on the canvas. If the mouse is over the canvas, a copy of the movie clip instance that is dragged and dropped is created using duplicateMovieClip() . The name given to the duplicate is derived by concatenating "object" with the current value of v (which is incremented with each new duplicate created). Based on this functionality, the current value of v will always reflect the number of duplicate icons that have been dragged and dropped onto the canvas an important thing to remember for the next exercise. Once created, the duplicate is sent to the same frame as the original instance (so that the same icon that was dragged appears on the canvas). The next action is used to disable the invisible button inside the duplicate named iconButton (as discussed in Step 4). In addition, the duplicate is scaled vertically and horizontally by 250 percent so that it will appear on the canvas as a larger representation of the icon instance from which it was created. NOTE A duplicated instance inherits the exact x and y positions of the original (at the time of its duplication). Thus, unless you use a simple script to move it, a duplicate upon its creation is placed right on top of the original (which means you won't even be able to tell that a duplicate has been created because nothing will have changed visually). The last two lines of ActionScript in this function send the dragged movie clip instance back to its original position, based on the values of homeX and homeY on its timeline. You'll notice that these actions are placed outside the conditional statement, meaning they're executed regardless of whether the statement proves true and a duplicate is created. Thus, if the user tries to drop an icon anywhere other than on top of the canvas movie clip instance, a duplicate will not be created and the icon will simply snap back to its original position below the canvas. 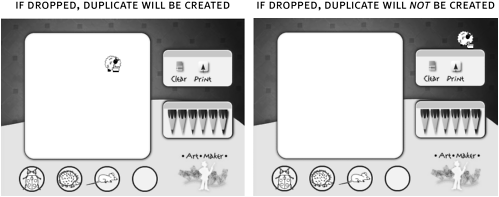 -
Choose Control > Test Movie to test your work. Drag the icons onto the canvas. If you drag an icon and drop it anywhere other than on the canvas instance, it will return to its original location, unduplicated. If you release the icon when your mouse is over the canvas movie clip instance, a duplicate will be created and the original will be sent back to its starting position. -
Close the test movie and save your work as draw4.fla. You've now created a simple, medium-sized drawing application. By applying the concepts you've learned here, you can create an application that includes many more features than this one. |