There are several common integration patterns that can be applied using the Platform Unification strategy. Front Controller Front Controller implemented with ASP.NET front end and EJB back end is one pattern, see [MS_FrontController] and [J2EE_FrontController]. In the WS-I example application, the .NET Front Controller represents MVC-II type presentation layer and the Java EE component implements a Session Façade and Data Transfer object business layer patterns. This pattern addresses systems where the presentation layer exists or is most efficiently developed using ASP.NET, and the back-end enterprise requirements are best met using the Java EE infrastructure. COM+ Adapter Component .NET-serviced components that require enterprise services, such as transaction management and declarative security, can be deployed in the Platform Unification approach as an EJB. The EJB framework provides a COM+ component with Java EE enterprise services. This is implemented by wrapping the serviced component as an EJB that exposes a home and remote (or local home and local) interface. Example The challenges of interoperability management are illustrated in the Service Interoperability (WS-1) Supply Chain Management (SCM) Retailer System. As described earlier, this article considers an implementation of the Retailer system where the front-end components are implemented in .NET and the back-end components in Java EE as EJBs. The Retailer system is a subsystem of the SOA-based SCM application. This configuration would be typical of organizations where the front-end Web development team is proficient in ASP.NET and the integrated Visual Studio .NET development environment and the back-end development team is proficient in Java EE. The IT management team is faced with the challenges of system and application management across a mixed platform. The front-end/back-end interoperability can be addressed by several of the interoperability technologies described previously: Bridging Solutions Both proprietary and CORBA-based solutions can provide point-to-point connectivity between the .NET front end and the back-end EJBs. The application is deployed on a mixed .NET/Java EE platform. Web services The back-end components provide a Web services interface to clients such as the .NET front end. This is the configuration that is implemented in the WS-I reference implementations. Using application server support for EJB 2.1, Web service endpoints are easily implemented using EJBs. The .NET front end uses these Web services, provided that the Web service interface types are interoperable with .NET supported types. The application is deployed on a mixed .NET/Java EE platform. Mono Mono enables the ASP.NET front end to be migrated to a non-Windows platform. This would allow the front and back ends to both run on a Unix or Linux platform but would not solve the interoperability management issues as described. Platform Unification The platform unification approach enables the .NET front end to access the back-end EJB components as .NET objects. The application is deployed on a unified Java EE platform. The choice of interoperability technology should address the architectural and organizational requirements. If one assumes that the Retailer subsystem is contained within a common organizational boundary, the subsystem may be best implemented using a tight-coupling approach. Platform Unification provides the most complete management functionality for a tightly-coupled system and simplifies the complexity of mixed platform management. The Platform Unification Solution Using the Platform Unification approach, the Retailer sub-system is implemented using the .NET web front end and an EJB implementation of the Retailer functionality. Both the .NET front end and the EJB are deployed on a common Java EE platform. This delivers a tightly coupled solution for the subsystem. The Web service interfaces of the other subsystems can be deployed as .NET Web services on Java EE using Platform Unification or as Java EE or .NET platforms on other systems. Figure 15-8 outlines the deployment architecture of this example. Figure 15-8. .NET Components deployed in a Java EE Servlet Container and EJB Components on a Java EE Server 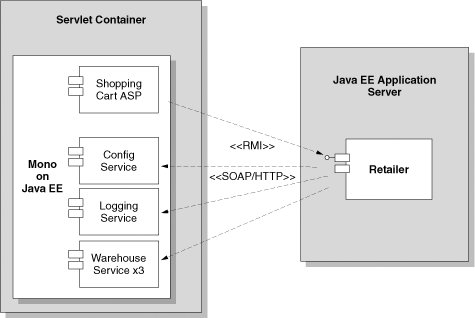 The components described in the deployment diagram in Figure 15-8 are deployed on a unified Java EE platform. The Shopping Cart component in the Web front end is implemented in .NET using ASP, and the Configuration Service, the Logging Service, and the three Warehouse Services are implemented as .NET Web services. Through Platform Unification, all of the .NET components are deployed on a Java EE servlet container. The Retailer component is implemented as an EJB, and its functionality is exposed through its remote interface and accessed using RMI. In the following discussion, the tightly coupled interface between the .NET front end and the Retailer EJB are detailed. Listing 15-1. Retailer EJB Remote Interface public interface Warehouse extends javax.ejb.EJBObject { public interface Retailer extends javax.ejb.EJBObject { public Collection getCatalog() throws java.rmi.RemoteException; public SubmitOrderResponse SubmitOrder( String configURL, String userId, SubmitOrderRequest submitOrderIn) throws java.rmi.RemoteException; }; } | The Mainsoft Visual MainWin development environment exposes the semantics of EJB use, such as JNDI lookup of the home interface and EJB remote interface invocation through a .NET proxy class. Listing 15-2. Generated .NET C# Proxy for the Retailer EJB public class Retailer : object, IDisposable, ISerializable { private static org.ws_i.www.retailer.RetailerHome home; private org.ws_i.www.retailer.Retailer ejbObj; static Retailer() { Context context; context = vmw.j2ee.Java EEUtils.CreateContext(null, null); object homeObj; homeObj = context.lookup( "java:comp/env/ejb/org/ws_i/www/retailer/RetailerHome"); home = ((org.ws_i.www.retailer.RetailerHome) (javax.rmi.PortableRemoteObject.narrow(homeObj, java.lang.Class.forName( "org.ws_i.www.retailer.RetailerHome")))); } public Retailer(org.ws_i.www.retailer.Retailer ejbObj) { this.ejbObj = ejbObj; } public Retailer(SerializationInfo info, StreamingContext ctx) { home = ((org.ws_i.www.retailer.RetailerHome) (info.GetValue("home", typeof(org.ws_i.www.retailer.RetailerHome)))); ejbObj = ((org.ws_i.www.retailer.Retailer) (info.GetValue("ejbObj", typeof(org.ws_i.www.retailer.Retailer)))); } public Retailer() { this.ejbObj = home.create(); } public virtual java.util.Collection Catalog { get { return this.ejbObj.getCatalog(); } } public virtual void GetObjectData(SerializationInfo info, StreamingContext ctx) { info.AddValue("home", home); info.AddValue("ejbObj", ejbObj); } public virtual void Dispose() { ejbObj = null; } public virtual org.ws_i.www.retailer.SubmitOrderResponse SubmitOrder(string arg_0, string arg_1, org.ws_i.www.retailer.SubmitOrderRequest arg_2) { return this.ejbObj.SubmitOrder (arg_0, arg_1, arg_2); } } | Calling remote interface methods is done from the .NET code by invoking methods of the proxy class. The flow of the UC1 Purchase Goods use case in this implementation has the ShoppingCart ASP page calling the getCatalog method of the Retailer EJB to retrieve the catalog and then present it to the user. This is seen in Listing 15-3, which shows the .NET ShoppingCart ASP C# code behind creating an EJB proxy instance and calling the getCatalog Retailer EJB remote interface method. Listing 15-3. .NET Code Accessing the Retailer EJB and Calling the getCatalog Method WebClient.localhost.Retailer retailer = new WebClient.localhost.Retailer(); if(retailer == null){ this.Session["OrderStatusMsg"] = "retailer unavailable "; Response.Redirect("OrderStatus.aspx"); Response.End(); } else{ try { java.util.logging.ConsoleHandler handler = new java.util.logging.ConsoleHandler(); java.util.logging.Logger logger = java.util.logging.Logger.getLogger("WebClient"); logger.addHandler(handler); logger.info("calling Retailer EJB getCatalog"); //get product catalog java.util.Collection catCollection =retailer.Catalog; logger.info("after call to Retailer EJB getCatalog"); | The catalog contents are returned to the .NET caller as a java.util.Collection of CatalogItem instances. This collection is manipulated in .NET as C# data structures, as shown in Listing 15-4. The mapping of java.math.BigDecimal to .NET System.Decimal is also shown in Listing 15-4. Listing 15-4. C# .NET Code Manipulating Native Java Data Types Returned by the EJB ArrayList cat=new ArrayList(); TableRow row=null; java.util.Iterator iter = catCollection.iterator(); while(iter.hasNext()){ org.ws_i.www.retailer.CatalogItem ciwsi = (org.ws_i.www.retailer.CatalogItem)iter.next(); CatalogItem ci = new CatalogItem(); ci.brand = ciwsi.getBrand(); ci.category = ciwsi.getCategory(); ci.description = ciwsi.getDescription(); ci.name = ciwsi.getName(); ci.price = Decimal.Parse( vmw.common.PrimitiveTypeUtils.BigDecimalToDecimal( ciwsi.getPrice()).ToString()); ci.productNumber = ciwsi.getProductNumber(); cat.Add(ci); row=AddProductRow(ci); | The ShoppingCart ASP page btnPlaceOrder_Click method is invoked on the user selection of the shopping cart. This method collects the selection data and prepares the Java parameters, as shown in Listing 15-5. Listing 15-5. C# .NET Code Manipulating Native Java Parameters java.util.ArrayList partsOrderList = new java.util.ArrayList(orderList.Count); foreach(PartsOrderItem poi in orderList) { java.lang.Integer iQuantity = java.lang.Integer.valueOf(poi.quantity.ToString()); java.math.BigDecimal bPrice = vmw.common.PrimitiveTypeUtils. DecimalToBigDecimal(poi.price); org.ws_i.www.retailer.PartsOrderItem item = new org.ws_i.www.retailer.PartsOrderItem( bPrice,poi.productNumber,iQuantity); partsOrderList.add(item); } | The next logical step is to collect customer information such as Name, Street, City, State, and Country in order to submit the order. Logging is performed prior to submitting the purchase order. The most important operation here is the EJB SubmitOrder method invoked through the EJB proxy: retailer.SubmitOrder(configuratorUrl, (String)config["UserID"],order); Listing 15-6 demonstrates customer data collection, logging, and the SubmitOrder method, along with retrieving an order response. Listing 15-6. C# .NET Code Calling the Retailer EJB SubmitOrder Method order.setPartsOrder(partsOrderList); cust.setName((String)config["CustomerName"]); cust.setCustnbr((String)config["CustomerNumber"]); cust.setStreet1((String)config["CustomerStreet"]); cust.setCity((String)config["CustomerCity"]); cust.setState((String)config["CustomerState"]); cust.setCountry((String)config["CustomerCountry"]); order.setCustDetails(cust); // Logging java.util.logging.ConsoleHandler handler = new java.util.logging.ConsoleHandler(); java.util.logging.Logger logger = java.util.logging.Logger.getLogger("WebClient"); logger.addHandler(handler); logger.info("calling Retailer EJB SubmitOrder"); // Submitting Order org.ws_i.www.retailer.SubmitOrderResponse responseEJB = retailer.SubmitOrder(configuratorUrl, (String)config["UserID"],order); logger.info("after Retailer EJB SubmitOrder"); submitOrderResponse response = new submitOrderResponse(); java.util.Collection responseCol = responseEJB.getOrderResponse(); | Meeting Management Requirements The implementation of the Retailer subsystem using Platform Unification is able to meet the management requirements detailed previously. Fault Management In the examples shown, one can see integrated logging through the Java Logging Facility. Through the use of the java.util.Logging API in both the .NET and the Java EE code, all logging is integrated across the components on a common Java EE logging facility. Remote exceptions are delivered to the front end and caught as .NET exceptions. Configuration Management The .NET front end is deployed on the Java EE platform as a fully-compliant WAR file, complete with web.xml and server-specific deployment descriptors. The WAR file contains all of the ASP pages and cross-compiled .NET code for the application. The WAR file is deployed and managed in the same way as any compliant servlet WAR file. Accounting Management Metering of the .NET front end is provided by the Java EE application server through the standard statistics facilities provided to all Java EE modules by the Java EE Management Model (JSR-77). The .NET front end is a managed object represented in the model as a standard WebModule. The statistics facilities in the javax.management.j2ee.statistics API allow for collection of all of the invocation timing. These management objects (MEJBs) are accessed by administration tools such as JMX MBeans. Performance Management The tight coupling of the .NET Web front end and Retailer EJB enables higher performance and improved user response time as compared with the Web services SOAP-based model. The RMI transport and marshaling is more efficient than SOAP/HTTP. The platform unification approach enables the use of native Java data types in the .NET code, facilitating the use of RMI. Security Management Using the Java EE declarative security model, authentication, access control, and transport-level security can be defined without changing the .NET code. Web Form authentication can be enabled by adding the appropriate declaration in the web.xml deployment description for the .NET front end. The authenticated security context is propagated through RMI calls to the EJBs. Access control declarations for the Shopping Cart page are declared. Additional security requirements, such as requiring SSL for the login page, can be specified declaratively. The Java EE application server provides facilities for management of user credentials, such as through JAAS. |