A Simple Example Now we move on to a simple example of a JSF application. Our first example starts with a login screen, shown in Figure 1-3. 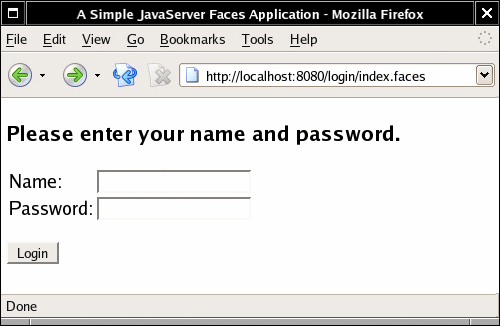 Of course, in a real web application, this screen would be beautified by a skilled graphic artist. The file that describes the login screen is essentially an HTML file with a few additional tags (see Listing 1-1). Its visual appearance can be easily improved by a graphic artist who need not have any programming skills. Listing 1-1. login/web/index.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <title>A Simple JavaServer Faces Application</title> 7. </head> 8. <body> 9. <h:form> 10. <h3>Please enter your name and password.</h3> 11. <table> 12. <tr> 13. <td>Name:</td> 14. <td> 15. <h:inputText value="#{user.name}"/> 16. </td> 17. </tr> 18. <tr> 19. <td>Password:</td> 20. <td> 21. <h:inputSecret value="#{user.password}"/> 22. </td> 23. </tr> 24. </table> 25. <p> 26. <h:commandButton value="Login" action="login"/> 27. </p> 28. </h:form> 29. </body> 30. </f:view> 31. </html>
| We discuss the contents of this file in detail later in this chapter, in the section "JSF Pages" on page 13. For now, note the following points: -
A number of the tags are standard HTML tags: body, table, and so on. -
Some tags have prefixes, such as f:view and h:inputText. These are JSF tags. The two taglib declarations declare the JSF tag libraries. -
The h:inputText, h:inputSecret, and h:commandButton tags correspond to the text field, password field, and submit button in Figure 1-3. -
The input fields are linked to object properties. For example, the attribute value="#{user.name}" tells the JSF implementation to link the text field with the name property of a user object. We discuss this linkage in more detail later in this chapter, in the section "Beans" on page 12. When the user enters the name and password, and clicks the "Login" button, a welcome screen appears (see Figure 1-4). Listing 1-3 on page 14 shows the source code for this screen. The section "Navigation" on page 16 explains how the application navigates from the login screen and the welcome screen. 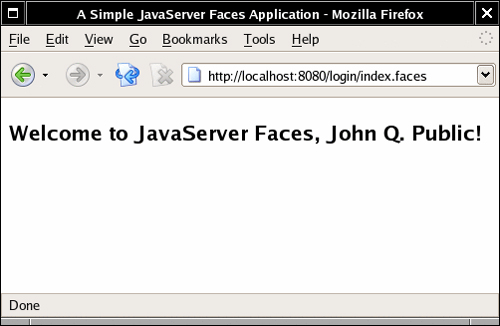 The welcome message contains the username. The password is ignored for now. The purpose of this application is, of course, not to impress anyone but to illustrate the various pieces that are necessary to produce a JSF application. Ingredients Our sample application consists of the following ingredients: -
Pages that define the login and welcome screens. We call them index.jsp and welcome.jsp. -
A bean that manages the user data (in our case, username and password). A bean is a Java class that exposes properties, usually by following a simple naming convention for the getter and setter methods. The code is in the file UserBean.java (see Listing 1-2). Note that the class is contained in the com.corejsf package. -
A configuration file for the application that lists bean resources and navigation rules. By default, this file is called faces-config.xml. -
Miscellaneous files that are needed to keep the servlet container happy: the web.xml file, and an index.html file that redirects the user to the correct URL for the login page. More advanced JSF applications have the same structure, but they can contain additional Java classes, such as event handlers, validators, and custom components. Listing 1-2. login/src/java/com/corejsf/UserBean.java 1. package com.corejsf; 2. 3. public class UserBean { 4. private String name; 5. private String password; 6. 7. // PROPERTY: name 8. public String getName() { return name; } 9. public void setName(String newValue) { name = newValue; } 10. 11. // PROPERTY: password 12. public String getPassword() { return password; } 13. public void setPassword(String newValue) { password = newValue; } 14. }
| Directory Structure A JSF application is deployed as a WAR file: a zipped file with extension .war and a directory structure that follows a standardized layout: 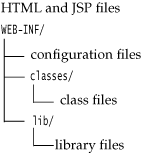 For example, the WAR file of our sample application has the directory structure shown in Figure 1-5. Note that the UserBean class is in the package com.corejsf. 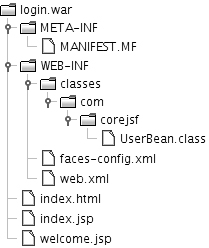 The META-INF directory is automatically produced by the jar program when the WAR file is created. We package our application source in a slightly different directory structure, following the Java Blueprints conventions (http://java.sun.com/blueprints/code/projectconventions.html). The source code is contained in an src/java directory, and the JSF pages and configuration files are contained in a web directory (see Figure 1-6). 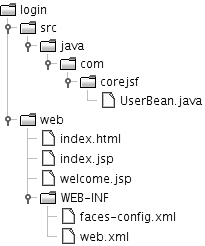 Build Instructions We now walk you through the steps required for building JSF applications with your bare hands. At the end of this chapter, we show you how to automate this process. | | 1. | Launch a command shell.
| 2. | Change to the corejsf-examples directory that is, the directory that contains the sample code for this book.
| 3. | Run the following commands:
cd ch1/login/src/java mkdir ../../web/WEB-INF/classes javac -d ../../web/WEB-INF/classes com/corejsf/UserBean.java On Windows, use backslashes instead:
cd ch1\login\web mkdir WEB-INF\classes javac -d ..\..\web\WEB-INF\classes com\corejsf\UserBean.java
| 4. | Change to the ch1/login/web directory.
| 5. | Run the following command:
jar cvf login.war . (Note the period at the end of the command, indicating the current directory.)
| 6. | Copy the login.war file to the directory glassfish/domains/domain1/autodeploy.
| 7. | Make sure that GlassFish has been started. Point your browser to
http://localhost:8080/login The application should start up at this point.
| The bean classes in more complex programs may need to interact with the JSF framework. In that case, the compilation step is more complex. Your class path must include the JSF libraries. With the GlassFish application server, add a single JAR file: glassfish/lib/javaee.jar With other systems, you may need multiple JAR files. A typical compilation command would look like this: javac -classpath .:glassfish/lib/javaee.jar -d ../../web/WEB-INF/classes com/corejsf/*.java On Windows, use semicolons to separate the path elements: javac -classpath .;glassfish\lib\javaee.jar -d ..\..\web\WEB-INF\classes com\corejsf\*.java Be sure to include the current directory (denoted by a period) in the class path. |