Action events are fired by command components buttons, links, etc. when the component is activated. As we saw in "Life-Cycle Events" on page 274, action events are fired during the Invoke Application phase, near the end of the life cycle. You typically attach action listeners to command components in JSF pages. For example, you can add an action listener to a link like this:
<h:commandLink actionListener="#{bean.linkActivated}"> ... </h:commandLink> Command components submit requests when they are activated, so there's no need to use onchange to force form submits as we did with value change events in "Value Change Events" on page 275. When you activate a command or link, the surrounding form is submitted and the JSF implementation subsequently fires action events. It's important to distinguish between action listeners and actions. In a nutshell, actions are designed for business logic and participate in navigation handling, whereas action listeners typically perform user interface logic and do not participate in navigation handling. Action listeners often work in concert with actions when an action needs information about the user interface. For example, the application shown in Figure 7-4 uses an action and an action listener to react to mouse clicks by forwarding to a JSF page. If you click on a president's face, the application forwards to a JSF page with information about that president. Note that an action alone cannot implement that behavior an action can navigate to the appropriate page, but it can't determine the appropriate page because it knows nothing about the image button in the user interface or the mouse click. Figure 7-4. The Rushmore Application 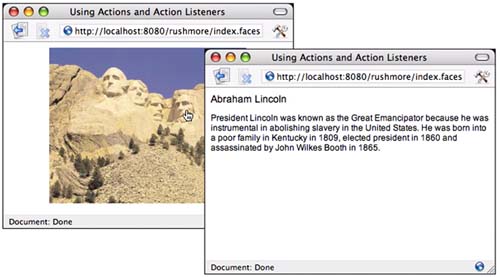 The application shown in Figure 7-4 uses a button with an image, like this:
<h:commandButton image="mountrushmore.jpg" actionListener="#{rushmore.listen}" action="#{rushmore.act}"/> When you click on a president, a listener which has access to the mouse click coordinates determines which president was selected. But the listener can't affect navigation, so it stores an outcome corresponding to the selected president in an instance field:
public class Rushmore { private String outcome; private Rectangle washingtonRect = new Rectangle(70,30,40,40); private Rectangle jeffersonRect = new Rectangle(115,45,40,40); private Rectangle rooseveltRect = new Rectangle(135,65,40,40); private Rectangle lincolnRect = new Rectangle(175,62,40,40); public void listen(ActionEvent e) { FacesContext context = FacesContext.getCurrentInstance(); String clientId = e.getComponent().getClientId(context); Map requestParams = context.getExternalContext(). getRequestParameterMap(); int x = new Integer((String)requestParams.get(clientId + ".x")).intValue(); int y = new Integer((String)requestParams.get(clientId + ".y")).intValue(); outcome = null; if(washingtonRect.contains(new Point(x,y))) outcome = "washington"; if(jeffersonRect.contains(new Point(x,y))) outcome = "jefferson"; if(rooseveltRect.contains(new Point(x,y))) outcome = "roosevelt"; if(lincolnRect.contains(new Point(x,y))) outcome = "lincoln"; } } The action associated with the button uses the outcome to affect navigation:
public String act() { return outcome; } Note that the JSF implementation always invokes action listeners before actions. NOTE  | JSF insists that you separate user interface logic and business logic by refusing to give actions access to events or the components that fire them. In the preceding example, the action cannot access the client ID of the component that fired the event, information that is necessary for extraction of mouse click coordinates from the request parameters. Because the action knows nothing about the user interface, we must add an action listener to the mix to implement the required behavior. |
The directory structure for the application shown in Figure 7-4 is shown in Figure 7-5. The code listings for the application are shown in Listing 7-6 through Listing 7-13. Listing 7-6. rushmore/index.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <link href="styles.css" rel="stylesheet" type="text/css"/> 7. <f:loadBundle basename="com.corejsf.messages" var="msgs"/> 8. <title> 9. <h:outputText value="#{msgs.windowTitle}"/> 10. </title> 11. </head> 12. <body> 13. <h:form style="text-align: center"> 14. <h:commandButton image="mountrushmore.jpg" 15. actionListener="#{rushmore.listen}" 16. action="#{rushmore.act}"/> 17. </h:form> 18. </body> 19. </f:view> 20. </html> Listing 7-7. rushmore/lincoln.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <link href="site.css" rel="stylesheet" type="text/css"/> 7. <f:loadBundle basename="com.corejsf.messages" var="msgs"/> 8. <title> 9. <h:outputText value="#{msgs.windowTitle}"/> 10. </title> 11. </head> 12. 13. <body> 14. <h:form> 15. <h:outputText value="#{msgs.lincolnPageTitle}" 16. style/> 17. <p/> 18. <h:outputText value="#{msgs.lincolnDiscussion}" 19. style/> 20. </h:form> 21. </body> 22. </f:view> 23. </html> Listing 7-8. rushmore/jefferson.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <link href="site.css" rel="stylesheet" type="text/css"/> 7. <f:loadBundle basename="com.corejsf.messages" var="msgs"/> 8. <title> 9. <h:outputText value="#{msgs.windowTitle}"/> 10. </title> 11. </head> 12. 13. 14. <body> 15. <h:form> 16. <h:outputText value="#{msgs.jeffersonPageTitle}" 17. style/> 18. <p/> 19. <h:outputText value="#{msgs.jeffersonDiscussion}" 20. style/> 21. </h:form> 22. </body> 23. </f:view> 24. </html> Listing 7-9. rushmore/roosevelt.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <link href="site.css" rel="stylesheet" type="text/css"/> 7. <f:loadBundle basename="com.corejsf.messages" var="msgs"/> 8. <title> 9. <h:outputText value="#{msgs.windowTitle}"/> 10. </title> 11. </head> 12. 13. <body> 14. <h:form> 15. <h:outputText value="#{msgs.rooseveltPageTitle}" 16. style/> 17. <p/> 18. <h:outputText value="#{msgs.rooseveltDiscussion}" 19. style/> 20. </h:form> 21. </body> 22. </f:view> 23. </html> Listing 7-10. rushmore/washington.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <link href="site.css" rel="stylesheet" type="text/css"/> 7. <f:loadBundle basename="com.corejsf.messages" var="msgs"/> 8. <title> 9. <h:outputText value="#{msgs.windowTitle}"/> 10. </title> 11. </head> 12. 13. <body> 14. <h:form> 15. <h:outputText value="#{msgs.washingtonPageTitle}" 16. style/> 17. <p/> 18. <h:outputText value="#{msgs.washingtonDiscussion}" 19. style/> 20. </h:form> 21. </body> 22. </f:view> 23. </html> Listing 7-11. rushmore/WEB-INF/classes/com/corejsf/Rushmore.java 1. package com.corejsf; 2. 3. import java.awt.Point; 4. import java.awt.Rectangle; 5. import java.util.Map; 6. import javax.faces.context.FacesContext; 7. import javax.faces.event.ActionEvent; 8. 9. public class Rushmore { 10. private String outcome = null; 11. private Rectangle washingtonRect = new Rectangle(70, 30, 40, 40); 12. private Rectangle jeffersonRect = new Rectangle(115, 45, 40, 40); 13. private Rectangle rooseveltRect = new Rectangle(135, 65, 40, 40); 14. private Rectangle lincolnRect = new Rectangle(175, 62, 40, 40); 15. 16. public void listen(ActionEvent e) { 17. FacesContext context = FacesContext.getCurrentInstance(); 18. String clientId = e.getComponent().getClientId(context); 19. Map requestParams = context.getExternalContext().getRequestParameterMap(); 20. 21. int x = new Integer((String) requestParams.get(clientId + ".x")) 22. .intValue(); 23. int y = new Integer((String) requestParams.get(clientId + ".y")) 24. .intValue(); 25. 26. outcome = null; 27. 28. if (washingtonRect.contains(new Point(x, y))) 29. outcome = "washington"; 30. 31. if (jeffersonRect.contains(new Point(x, y))) 32. outcome = "jefferson"; 33. 34. if (rooseveltRect.contains(new Point(x, y))) 35. outcome = "roosevelt"; 36. 37. if (lincolnRect.contains(new Point(x, y))) 38. outcome = "lincoln"; 39. } 40. 41. public String act() { 42. return outcome; 43. } 44. } Listing 7-12. rushmore/WEB-INF/faces-config.xml 1. <?xml version="1.0"?> 2. 3. <!DOCTYPE faces-config PUBLIC 4. "-//Sun Microsystems, Inc.//DTD JavaServer Faces Config 1.0//EN" 5. "http://java.sun.com/dtd/web-facesconfig_1_0.dtd"> 6. <faces-config> 7. <navigation-rule> 8. <from-view-id>/index.jsp</from-view-id> 9. <navigation-case> 10. <from-outcome>washington</from-outcome> 11. <to-view-id>/washington.jsp</to-view-id> 12. </navigation-case> 13. <navigation-case> 14. <from-outcome>jefferson</from-outcome> 15. <to-view-id>/jefferson.jsp</to-view-id> 16. </navigation-case> 17. <navigation-case> 18. <from-outcome>roosevelt</from-outcome> 19. <to-view-id>/roosevelt.jsp</to-view-id> 20. </navigation-case> 21. <navigation-case> 22. <from-outcome>lincoln</from-outcome> 23. <to-view-id>/lincoln.jsp</to-view-id> 24. </navigation-case> 25. </navigation-rule> 26. 27. <managed-bean> 28. <managed-bean-name>rushmore</managed-bean-name> 29. <managed-bean-class>com.corejsf.Rushmore</managed-bean-class> 30. <managed-bean-scope>session</managed-bean-scope> 31. </managed-bean> 32. 33. </faces-config> Listing 7-13. rushmore/WEB-INF/classes/com/corejsf/messages.properties 1. windowTitle=Mt. Rushmore Tabbed Pane 2. jeffersonTabText=Jefferson 3. rooseveltTabText=Roosevelt 4. lincolnTabText=Lincoln 5. washingtonTabText=Washington 6. 7. jeffersonTooltip=Thomas Jefferson 8. rooseveltTooltip=Theodore Roosevelt 9. lincolnTooltip=Abraham Lincoln 10. washingtonTooltip=George Washington 11. 12. lincolnDiscussion=President Lincoln was known as the Great Emancipator because \ 13. he was instrumental in abolishing slavery in the United States. He was born \ 14. into a poor family in Kentucky in 1809, elected president in 1860 and \ 15. assassinated by John Wilkes Booth in 1865. 16. 17. washingtonDiscussion=George Washington was the first president of the United \ 18. States. He was born in 1732 in Virginia and was elected Commander in Chief of \ 19. the Continental Army in 1775 and forced the surrender of Cornwallis at Yorktown \ 20. in 1781. He was inaugurated on April 30, 1789. 21. 22. rooseveltDiscussion=Theodore Roosevelt was the 26th president of the United \ 23. States. In 1901 he became president after the assassination of President \ 24. McKinley. At only 42 years of age, he was the youngest president in US history. 25. 26. jeffersonDiscussion=Thomas Jefferson, the 3rd US president, was born in \ 27. 1743 in Virginia. Jefferson was tall and awkward, and was not known as a \ 28. great public speaker. Jefferson became minister to France in 1785, after \ 29. Benjamin Franklin held that post. In 1796, Jefferson was a reluctant \ 30. presidential candiate, and missed winning the election by a mere three votes. \ 31. He served as president from 1801-1809. Figure 7-5. Rushmore Example Directory Structure 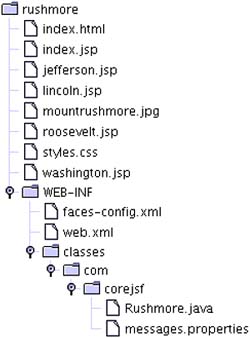
|