Using the JOptionPane Another really useful class found in the Swing library is the JOptionPane. This class provides a simple way to display pop-up dialogs in your program. JOptionPanes come in a variety of handy shapes and types: Input Dialog Contains a text field and two buttons, OK and Cancel. Confirm Dialog Contains either OK/Cancel or Yes/No buttons. Message Dialog Contains only an OK button. Option Dialog Contains a list of choices and an arbitrary number of buttons. All the types of JOptionPane work similarly. You first instantiate the object by passing in a set of parameters (some are constants) that tell the JOptionPane class what kind of message box you want to see. Listing 17.7 shows an example of a message box that accepts user input. Listing 17.7 The TestJOptionPane1.java File /* * TestJOptionPane1.java * * Created on September 5, 2002, 4:34 PM */ package ch17; import javax.swing.*; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts */ public class TestJOptionPane1 extends JFrame implements ActionListener { JOptionPane op; JTextArea ta; JButton btnEnter; /** Creates a new instance of TestJOptionPane1 */ public TestJOptionPane1() { op = new JOptionPane("What is your name?", JOptionPane.QUESTION_MESSAGE, JOptionPane.OK_CANCEL_OPTION, null); op.setSelectionValues(null); op.setWantsInput(true); ta = new JTextArea(5, 30); ta.setText(""); JPanel p1 = new JPanel(); p1.add(ta); btnEnter = new JButton("Enter Your Name"); btnEnter.addActionListener(this); JPanel p2 = new JPanel(); p2.add(btnEnter); JPanel cp = (JPanel)getContentPane(); cp.setLayout(new BorderLayout()); cp.add(p1, BorderLayout.NORTH); cp.add(p2, BorderLayout.SOUTH); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setBounds(100, 100, 400, 200); setVisible(true); setTitle("Using a JOptionPane"); } public void actionPerformed(ActionEvent ae) { Container parent = btnEnter.getParent(); JDialog jd = op.createDialog(parent, "Save"); jd.show(); String filename = (String) op.getInputValue(); System.out.println("You entered " + filename); ta.setText("You entered " + filename); } public static void main(String[] args) { TestJOptionPane1 top1 = new TestJOptionPane1(); } } The option pane generates events, so we have to implement a listener. public class TestJOptionPane1 extends JFrame implements ActionListener { We instantiate the option pane by passing in the string that we want displayed, the type of message that we want, the option type (specifies which buttons appear), and the special buttons (in this case null) that you want to see. op = new JOptionPane("What is your name?", JOptionPane.QUESTION_MESSAGE, JOptionPane.OK_CANCEL_OPTION, null); We have no predefined set of values to have the user choose from, so we enter null. op.setSelectionValues(null); We want the user to input a value, so we call this method with true as the parameter. op.setWantsInput(true); We want the button on the JFrame to work, so we implement the actionPerformed() method. public void actionPerformed(ActionEvent ae) { Container parent = btnEnter.getParent(); JDialog jd = op.createDialog(parent, "Save"); jd.show(); When the dialog is displayed, the program blocks waiting for a response. When it continues, the option pane's input value will be populated. String filename = (String) op.getInputValue(); We display it both on the JFrame and on the console. System.out.println("You entered " + filename); ta.setText("You entered " + filename); The result of running this program is shown here in Figure 17.6. Figure 17.6. A JOptionPane can accept user input. 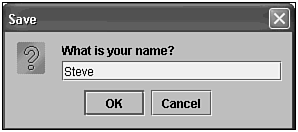 Notice that the name doesn't appear until you click the OK button. Notice also that the original name is still in the text field on the option pane when you click the "Enter your Name" button again. |