The ServiceBase Class To create a Windows service, you derive a custom class from the ServiceBase class of the System.ServiceProcess namespace. Table 7.1 lists the important members of the ServiceBase class. Table 7.1. Important Members of the ServiceBase ClassMember Name | Type | Description |
---|
AutoLog | Property | When set to true, automatically logs the calls to the OnStart(), OnStop(), OnPause(), and OnContinue() methods in the Application event log. | CanPauseAndContinue | Property | When set to true, indicates that the service can be paused and resumed. | CanShutdown | Property | When set to true, indicates that the service should be notified when the system is shutting down. | CanStop | Property | When set to true, indicates that the service can be stopped after it has started. | EventLog | Property | Specifies an event log that can be used to write customized messages to the Application event log on method calls such as OnStart() and OnStop(). | OnContinue() | Method | Specifies the actions to take when a service resumes normal functioning after being paused. This method is executed when the SCM sends a Continue message to the Windows service. | OnPause() | Method | Specifies the actions to take when a service pauses. This method is executed when the SCM sends a Pause message to the Windows service. | OnStart() | Method | Specifies the actions to take when a service starts running. This method is executed when the SCM sends a Start message to the Windows service. | OnStop() | Method | Specifies the actions to take when a service stops running. This method is executed when the SCM sends a Stop message to the Windows service. | Run() | Method | Provides the main entry point for a service executable. | ServiceName | Property | Specifies a name that identifies the service. | 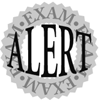 | With the help of the AutoLog and EventLog properties of the ServiceBase class, you can write messages to the Application event log only. If you want to write entries to a different event log, you should set the AutoLog property to false, and create a new EventLog component, and override the OnStart(), OnStop(), and other related methods to explicitly post entries to the custom log. |
Understanding How the SCM Interacts with a Windows Service The SCM handles the tasks involved in controlling a Windows service. Figure 7.2 illustrates how the SCM interacts with a Windows service. Figure 7.2. The ServiceBase class provides the functionality that enables a Windows service application to interact with the SCM. 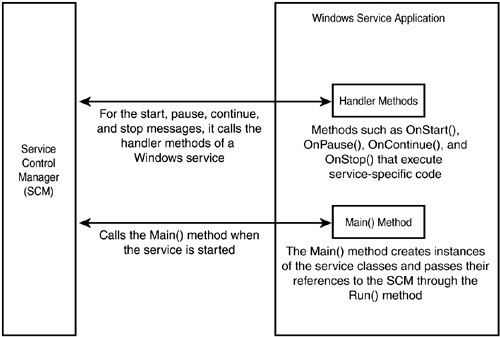 When the SCM sends a Start message to the Windows service, the following things happen: The SCM finds the path of the Windows service application's executable file from the Windows service database. The SCM creates a Windows service process by executing the Main() method defined in the Windows service executable. The Main() method of the Windows service executable creates one or more instances of the Windows service class and passes their references to the SCM through the static Run() method of the ServiceBase class. The SCM uses these references to send messages to the Windows service. The handler associated with the start message the OnStart() method is executed. The OnStart() method executes the code that is required for a Windows service to run, such as listening to a port for incoming requests, logging events to the event log, spooling print jobs, and so on. After the Windows service is started, the SCM can use its reference to send various messages, such as Pause, Continue, and Stop messages, to the Windows service. These messages invoke the OnPause(), OnContinue(), and OnStop() methods, respectively, of the Windows service. Not all services implement the OnPause(), OnContinue(), and OnStop() methods. Whether a service needs to implement these methods depends on the CanPauseAndContinue and CanStop properties of the Windows service. If these properties are true, the SCM can invoke the OnPause(), OnContinue(), and OnStop() methods. But if your service can't be paused, for example, set the CanPauseAndContinue property to false and the SCM will never call OnPause or OnContinue. Creating the Windows Service Application Take the following steps to create a Windows service application: Open Visual Studio .NET and create a new blank solution named C07 at the location C:\EC70320. Add a new Visual C# .NET Windows Service project (Example7_1) to the solution. The Windows service project contains a file named Service1.cs. Access the Properties window, set the Name and ServiceName properties to WebsiteMonitor, and set the CanPauseAndContinue property to true. Note that the AutoLog and the CanStop properties are already true. From the Components tab of the toolbox, drop an EventLog component (eventLog1) on the design area. Set its Log property to Application and set the Source property to WebsiteMonitor. From the Components tab of the toolbox, drop a Timer component (timer1) on the design area. Set its Enabled property to false and its Interval property to 60000 milliseconds. Switch to Code view and change all instances of Service1 to WebsiteMonitor. Add the following using directives to the code: using System.Net; using System.Configuration; Add a new Application Configuration file (App.Config) to the project. Add the following content to it: <?xml version="1.0" encoding="utf-8" ?> <configuration> <appSettings> <add key="Website" value="http://www.techcontent.com" /> </appSettings> </configuration> Add the following event handler for the Elapsed event of the timer1 control: private void timer1_Elapsed(object sender, System.Timers.ElapsedEventArgs e) { HttpWebRequest req = (HttpWebRequest) WebRequest.Create (ConfigurationSettings.AppSettings["Website"]); try { using (HttpWebResponse res = (HttpWebResponse)req.GetResponse()){} } catch(System.Net.WebException we) { eventLog1.WriteEntry(we.Message, EventLogEntryType.Error); } } In the code, search for the skeletons of the OnStart() and OnStop() methods, and modify them as follows: protected override void OnStart(string[] args) { timer1.Enabled = true; } protected override void OnStop() { timer1.Enabled = false; } Add the following code to override the OnPause() and the OnContinue() methods of the base class: protected override void OnPause() { timer1.Enabled = false; } protected override void OnContinue() { timer1.Enabled = true; } Build the project. This step creates the WebsiteMonitor Windows service application. Compilation of this project creates an executable file just like other Windows applications. However, you cannot directly run this executable. You need to install a Windows service application before you can start it. When you build the project, Visual Studio .NET uses the app.config file to create the actual application configuration file, which is of the form filename.exe.config (in this case, it is Example7_1.exe.config). |