Every Java programmer is familiar with the process of inserting calls to System.out.println into troublesome code to gain insight into program behavior. Of course, once you have figured out the cause of trouble, you remove the print statements, only to put them back in when the next problem surfaces. The logging API of JDK 1.4 is designed to overcome this problem. Here are the principal advantages of the API. It is easy to suppress all log records or just those below a certain level, and just as easy to turn them back on. Suppressed logs are very cheap, so that there is only a minimal penalty for leaving the logging code in your application. Log records can be directed to different handlers, for display in the console, for storage in a file, and so on. Both loggers and handlers can filter records. Filters discard boring log entries, using any criteria supplied by the filter implementor. Log records can be formatted in different ways, for example, in plain text or XML. Applications can use multiple loggers, with hierarchical names such as com.mycompany.myapp, similar to package names. By default, the logging configuration is controlled by a configuration file. Applications can replace this mechanism if desired. Basic Logging Let's get started with the simplest possible case. The logging system manages a default logger Logger.global that you can use instead of System.out. Use the info method to log an information message: Logger.global.info("File->Open menu item selected"); By default, the record is printed like this: May 10, 2004 10:12:15 PM LoggingImageViewer fileOpen INFO: File->Open menu item selected (Note that the time and the names of the calling class and method are automatically included.) But if you call Logger.global.setLevel(Level.OFF); at an appropriate place (such as the beginning of main), then all logging is suppressed. Advanced Logging Now that you have seen "logging for dummies," let's go on to industrial-strength logging. In a professional application, you wouldn't want to log all records to a single global logger. Instead, you can define your own loggers. When you request a logger with a given name for the first time, it is created. Logger myLogger = Logger.getLogger("com.mycompany.myapp"); Subsequent calls to the same name yield the same logger object. Similar to package names, logger names are hierarchical. In fact, they are more hierarchical than packages. There is no semantic relationship between a package and its parent, but logger parents and children share certain properties. For example, if you set the log level on the logger "com.mycompany", then the child loggers inherit that level. There are seven logging levels: SEVERE WARNING INFO CONFIG FINE FINER FINEST By default, the top three levels are actually logged. You can set a different level, for example, logger.setLevel(Level.FINE); Now all levels of FINE and higher are logged. You can also use Level.ALL to turn on logging for all levels or Level.OFF to turn all logging off. There are logging methods for all levels, such as logger.warning(message); logger.fine(message); and so on. Alternatively, you can use the log method and supply the level, such as logger.log(Level.FINE, message); TIP  | The default logging configuration logs all records with level of INFO or higher. Therefore, you should use the levels CONFIG, FINE, FINER, and FINEST for debugging messages that are useful for diagnostics but meaningless to the program user. |
CAUTION  | If you set the logging level to a value finer than INFO, then you also need to change the log handler configuration. The default log handler suppresses messages below INFO. See the next section for details. |
The default log record shows the name of the class and method that contain the logging call, as inferred from the call stack. However, if the virtual machine optimizes execution, accurate call information may not be available. You can use the logp method to give the precise location of the calling class and method. The method signature is void logp(Level l, String className, String methodName, String message) There are convenience methods for tracing execution flow: void entering(String className, String methodName) void entering(String className, String methodName, Object param) void entering(String className, String methodName, Object[] params) void exiting(String className, String methodName) void exiting(String className, String methodName, Object result) For example, int read(String file, String pattern) { logger.entering("com.mycompany.mylib.Reader", "read", new Object[] { file, pattern }); . . . logger.exiting("com.mycompany.mylib.Reader", "read", count); return count; } These calls generate log records of level FINER that start with the strings ENtrY and RETURN. NOTE  | At some point in the future, the logging methods will be rewritten to support variable parameter lists ("varargs"). Then you will be able to make calls such as logger.entering("com.mycompany.mylib.Reader", "read", file, pattern). |
A common use for logging is to log unexpected exceptions. Two convenience methods include a description of the exception in the log record. void throwing(String className, String methodName, Throwable t) void log(Level l, String message, Throwable t) Typical uses are if (. . .) { IOException exception = new IOException(". . ."); logger.throwing("com.mycompany.mylib.Reader", "read", exception); throw exception; } and try { . . . } catch (IOException e) { Logger.getLogger("com.mycompany.myapp").log(Level.WARNING, "Reading image", e); } The throwing call logs a record with level FINER and a message that starts with ThrOW. Changing the Log Manager Configuration You can change various properties of the logging system by editing a configuration file. The default configuration file is located at jre/lib/logging.properties
To use another file, set the java.util.logging.config.file property to the file location by starting your application with java -Djava.util.logging.config.file=configFile MainClass
CAUTION  | Calling System.setProperty("java.util.logging.config.file", file) in main has no effect because the log manager is initialized during VM startup, before main executes. |
To change the default logging level, edit the configuration file and modify the line .level=INFO You can specify the logging levels for your own loggers by adding lines such as com.mycompany.myapp.level=FINE That is, append the .level suffix to the logger name. As you see later in this section, the loggers don't actually send the messages to the console that is the job of the handlers. Handlers also have levels. To see FINE messages on the console, you also need to set java.util.logging.ConsoleHandler.level=FINE CAUTION  | The settings in the log manager configuration are not system properties. Starting a program with -Dcom.mycompany.myapp.level=FINE does not have any influence on the logger. |
NOTE  | The logging properties file is processed by the java.util.logging.LogManager class. It is possible to specify a different log manager by setting the java.util.logging.manager system property to the name of a subclass. Alternatively, you can keep the standard log manager and still bypass the initialization from the logging properties file. Set the java.util.logging.config.class system property to the name of a class that sets log manager properties in some other way. See the API documentation for the LogManager class for more information. |
Localization You may want to localize logging messages so that they are readable for international users. Internationalization of applications is the topic of Chapter 10 of Volume 2. Briefly, here are the points to keep in mind when localizing logging messages. Localized applications contain locale-specific information in resource bundles. A resource bundle consists of a set of mappings for various locales (such as United States or Germany). For example, a resource bundle may map the string "readingFile" into strings "Reading file" in English or "Achtung! Datei wird eingelesen" in German. A program may contain multiple resource bundles, perhaps one for menus and another for log messages. Each resource bundle has a name (such as "com.mycompany.logmessages"). To add mappings to a resource bundle, you supply a file for each locale. English message mappings are in a file com/mycompany/logmessages_en.properties, and German message mappings are in a file com/mycompany/logmessages_de.properties. (The en, de codes are the language codes.) You place the files together with the class files of your application, so that the ResourceBundle class will automatically locate them. These files are plain text files, consisting of entries such as readingFile=Achtung! Datei wird eingelesen renamingFile=Datei wird umbenannt ... When requesting a logger, you can specify a resource bundle: Logger logger = Logger.getLogger(loggerName, "com.mycompany.logmessages"); Then you specify the resource bundle key, not the actual message string, for the log message. logger.info("readingFile"); You often need to include arguments into localized messages. Then the message should contain placeholders {0}, {1}, and so on. For example, to include the file name with a log message, include the placeholder like this: Reading file {0}. Achtung! Datei {0} wird eingelesen. You then pass values into the placeholders by calling one of the following methods: logger.log(Level.INFO, "readingFile", fileName); logger.log(Level.INFO, "renamingFile", new Object[] { oldName, newName }); Handlers By default, loggers send records to a ConsoleHandler that prints them to the System.err stream. Specifically, the logger sends the record to the parent handler, and the ultimate ancestor (with name "") has a ConsoleHandler. Like loggers, handlers have a logging level. For a record to be logged, its logging level must be above the threshold of both the logger and the handler. The log manager configuration file sets the logging level of the default console handler as java.util.logging.ConsoleHandler.level=INFO To log records with level FINE, change both the default logger level and the handler level in the configuration. Alternatively, you can bypass the configuration file altogether and install your own handler. Logger logger = Logger.getLogger("com.mycompany.myapp"); logger.setLevel(Level.FINE); logger.setUseParentHandlers(false); Handler handler = new ConsoleHandler(); handler.setLevel(Level.FINE); logger.addHandler(handler); By default, a logger sends records both to its own handlers and the handlers of the parent. Our logger is a child of the primordial logger (with name "") that sends all records with level INFO or higher to the console. But we don't want to see those records twice. For that reason, we set the useParentHandlers property to false. To send log records elsewhere, add another handler. The logging API provides two useful handlers for this purpose, a FileHandler and a SocketHandler. The SocketHandler sends records to a specified host and port. Of greater interest is the FileHandler that collects records in a file. You can simply send records to a default file handler, like this: FileHandler handler = new FileHandler(); logger.addHandler(handler); The records are sent to a file javan.log in the user's home directory, where n is a number to make the file unique. If a user's system has no concept of the user's home directory (for example, in Windows 95/98/Me), then the file is stored in a default location such as C:\Windows. By default, the records are formatted in XML. A typical log record has the form <record> <date>2002-02-04T07:45:15</date> <millis>1012837515710</millis> <sequence>1</sequence> <logger>com.mycompany.myapp</logger> <level>INFO</level> <class>com.mycompany.mylib.Reader</class> <method>read</method> <thread>10</thread> <message>Reading file corejava.gif</message> </record> You can modify the default behavior of the file handler by setting various parameters in the log manager configuration (see Table 11-2), or by using another constructor (see the API notes at the end of this section). Table 11-2. File Handler Configuration ParametersConfiguration Property | Description | Default |
---|
java.util.logging.FileHandler.level | The handler level. | Level.ALL | java.util.logging.FileHandler.append | Controls whether the handler should append to an existing file, or open a new file for each program run. | False | Configuration Property | Description | Default |
---|
java.util.logging.FileHandler.limit | The approximate maximum number of bytes to write in a file before opening another. (0 = no limit). | 0 (no limit) in the FileHandler class, 50000 in the default log manager configuration | java.util.logging.FileHandler.pattern | The pattern for the log file name. See Table 11-3 for pattern variables. | %h/java%u.log | java.util.logging.FileHandler.count | The number of logs in a rotation sequence. | 1 (no rotation) | java.util.logging.FileHandler.filter | The filter class to use. | No filtering | java.util.logging.FileHandler.encoding | The character encoding to use. | The platform encoding | java.util.logging.FileHandler.formatter | The record formatter. | java.util.logging.XMLFormatter |
You probably don't want to use the default log file name. Therefore, you should use another pattern, such as %h/myapp.log. (See Table 11-3 for an explanation of the pattern variables.) Table 11-3. Log File Pattern VariablesVariable | Description |
---|
%h | The value of the user.home system property. | %t | The system temporary directory. | %u | A unique number to resolve conflicts. | %g | The generation number for rotated logs. (A .%g suffix is used if rotation is specified and the pattern doesn't contain %g.) | %% | The % character. |
If multiple applications (or multiple copies of the same application) use the same log file, then you should turn the "append" flag on. Alternatively, use %u in the file name pattern so that each application creates a unique copy of the log. It is also a good idea to turn file rotation on. Log files are kept in a rotation sequence, such as myapp.log.0, myapp.log.1, myapp.log.2, and so on. Whenever a file exceeds the size limit, the oldest log is deleted, the other files are renamed, and a new file with generation number 0 is created. TIP  | Many programmers use logging as an aid for the technical support staff. If a program misbehaves in the field, then the user can send back the log files for inspection. In that case, you should turn the "append" flag on, use rotating logs, or both. |
You can also define your own handlers by extending the Handler or the StreamHandler class. We define such a handler in the example program at the end of this section. That handler displays the records in a window (see Figure 11-3). Figure 11-3. A log handler that displays records in a window 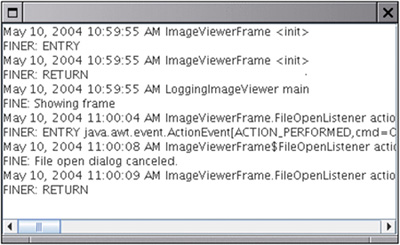
The handler extends the StreamHandler class and installs a stream whose write methods display the stream output in a text area. class WindowHandler extends StreamHandler { public WindowHandler() { . . . final JTextArea output = new JTextArea(); setOutputStream(new OutputStream() { public void write(int b) {} // not called public void write(byte[] b, int off, int len) { output.append(new String(b, off, len)); } }); } . . . } There is just one problem with this approach the handler buffers the records and only writes them to the stream when the buffer is full. Therefore, we override the publish method to flush the buffer after each record: class WindowHandler extends StreamHandler { . . . public void publish(LogRecord record) { super.publish(record); flush(); } } If you want to write more exotic stream handlers, extend the Handler class and define the publish, flush, and close methods. Filters By default, records are filtered according to their logging levels. Each logger and handler can have an optional filter to perform added filtering. You define a filter by implementing the Filter interface and defining the method boolean isLoggable(LogRecord record) Analyze the log record, using any criteria that you desire, and return true for those records that should be included in the log. For example, a particular filter may only be interested in the messages generated by the entering and exiting methods. The filter should then call record.getMessage() and check whether it starts with ENTRY or RETURN. To install a filter into a logger or handler, simply call the setFilter method. Note that you can have at most one filter at a time. Formatters The ConsoleHandler and FileHandler classes emit the log records in text and XML formats. However, you can define your own formats as well. You need to extend the Formatter class and override the method String format(LogRecord record) Format the information in the record in any way you like and return the resulting string. In your format method, you may want to call the method String formatMessage(LogRecord record) That method formats the message part of the record, substituting parameters and applying localization. Many file formats (such as XML) require a head and tail part that surrounds the formatted records. In that case, override the methods String getHead(Handler h) String getTail(Handler h) Finally, call the setFormatter method to install the formatter into the handler. With so many options for logging, it is easy to lose track of the fundamentals. The "Logging Cookbook" sidebar summarizes the most common operations. Example 11-4 presents the code for the image viewer that logs events to a log window. Logging Cookbook For a simple application, choose a single logger. It is a good idea to give the logger the same name as your main application package, such as com.mycompany.myprog. You can always get the logger by calling Logger logger = Logger.getLogger("com.mycompany.myprog");
For convenience, you may want to add static fields private static final Logger logger = Logger.getLogger("com .mycompany.myprog");
to classes with a lot of logging activity. The default logging configuration logs all messages of level INFO or higher to the console. Users can override the default configuration, but as you have seen, the process is a bit involved. Therefore, it is a good idea to install a more reasonable default in your application. The following code ensures that all messages are logged to an application-specific file. Place the code into the main method of your application. if (System.getProperty("java.util.logging.config.class") == null && System.getProperty("java.util.logging.config.file") == null) { try { Logger.getLogger("").setLevel(Level.ALL); final int LOG_ROTATION_COUNT = 10; Handler handler = new FileHandler("%h/myapp.log", 0, LOG_ROTATION_COUNT); Logger.getLogger("").addHandler(handler); } catch (IOException e) { logger.log(Level.SEVERE, "Can't create log file handler", e); } }
Now you are ready to log to your heart's content. Keep in mind that all messages with level INFO, WARNING, and SEVERE show up on the console. Therefore, reserve these levels for messages that are meaningful to the users of your program. The level FINE is a good choice for logging messages that are intended for programmers. Whenever you are tempted to call System.out.println, emit a log message instead: logger.fine("File open dialog canceled");
It is also a good idea to log unexpected exceptions, for example, try { . . . } catch (SomeException e) { logger.log(Level.FINE, "explanation", e); }
|
Example 11-4. LoggingImageViewer.java 1. import java.awt.*; 2. import java.awt.event.*; 3. import java.awt.image.*; 4. import java.io.*; 5. import java.util.logging.*; 6. import javax.swing.*; 7. 8. /** 9. A modification of the image viewer program that logs 10. various events. 11. */ 12. public class LoggingImageViewer 13. { 14. public static void main(String[] args) 15. { 16. 17. if (System.getProperty("java.util.logging.config.class") == null 18. && System.getProperty("java.util.logging.config.file") == null) 19. { 20. try 21. { 22. Logger.getLogger("").setLevel(Level.ALL); 23. final int LOG_ROTATION_COUNT = 10; 24. Handler handler = new FileHandler("%h/LoggingImageViewer.log", 0, LOG_ROTATION_COUNT); 25. Logger.getLogger("").addHandler(handler); 26. } 27. catch (IOException e) 28. { 29. Logger.getLogger("com.horstmann.corejava").log(Level.SEVERE, 30. "Can't create log file handler", e); 31. } 32. } 33. 34. Handler windowHandler = new WindowHandler(); 35. windowHandler.setLevel(Level.ALL); 36. Logger.getLogger("com.horstmann.corejava").addHandler(windowHandler); 37. 38. JFrame frame = new ImageViewerFrame(); 39. frame.setTitle("LoggingImageViewer"); 40. frame.setSize(300, 400); 41. frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); 42. 43. Logger.getLogger("com.horstmann.corejava").fine("Showing frame"); 44. frame.setVisible(true); 45. } 46. } 47. 48. /** 49. The frame that shows the image. 50. */ 51. class ImageViewerFrame extends JFrame 52. { 53. public ImageViewerFrame() 54. { 55. logger.entering("ImageViewerFrame", "<init>"); 56. // set up menu bar 57. JMenuBar menuBar = new JMenuBar(); 58. setJMenuBar(menuBar); 59. 60. JMenu menu = new JMenu("File"); 61. menuBar.add(menu); 62. 63. JMenuItem openItem = new JMenuItem("Open"); 64. menu.add(openItem); 65. openItem.addActionListener(new FileOpenListener()); 66. 67. JMenuItem exitItem = new JMenuItem("Exit"); 68. menu.add(exitItem); 69. exitItem.addActionListener(new 70. ActionListener() 71. { 72. public void actionPerformed(ActionEvent event) 73. { 74. logger.fine("Exiting."); 75. System.exit(0); 76. } 77. }); 78. 79. // use a label to display the images 80. label = new JLabel(); 81. add(label); 82. logger.exiting("ImageViewerFrame", "<init>"); 83. } 84. 85. private class FileOpenListener implements ActionListener 86. { 87. public void actionPerformed(ActionEvent event) 88. { 89. logger.entering("ImageViewerFrame.FileOpenListener", "actionPerformed", event); 90. 91. // set up file chooser 92. JFileChooser chooser = new JFileChooser(); 93. chooser.setCurrentDirectory(new File(".")); 94. 95. // accept all files ending with .gif 96. chooser.setFileFilter(new 97. javax.swing.filechooser.FileFilter() 98. { 99. public boolean accept(File f) 100. { 101. return f.getName().toLowerCase().endsWith(".gif") || f.isDirectory(); 102. } 103. public String getDescription() 104. { 105. return "GIF Images"; 106. } 107. }); 108. 109. // show file chooser dialog 110. int r = chooser.showOpenDialog(ImageViewerFrame.this); 111. 112. // if image file accepted, set it as icon of the label 113. if (r == JFileChooser.APPROVE_OPTION) 114. { 115. String name = chooser.getSelectedFile().getPath(); 116. logger.log(Level.FINE, "Reading file {0}", name); 117. label.setIcon(new ImageIcon(name)); 118. } 119. else 120. logger.fine("File open dialog canceled."); 121. logger.exiting("ImageViewerFrame.FileOpenListener", "actionPerformed"); 122. } 123. } 124. 125. private JLabel label; 126. private static Logger logger = Logger.getLogger("com.horstmann.corejava"); 127. } 128. 129. /** 130. A handler for displaying log records in a window. 131. */ 132. class WindowHandler extends StreamHandler 133. { 134. public WindowHandler() 135. { 136. frame = new JFrame(); 137. final JTextArea output = new JTextArea(); 138. output.setEditable(false); 139. frame.setSize(200, 200); 140. frame.add(new JScrollPane(output)); 141. frame.setFocusableWindowState(false); 142. frame.setVisible(true); 143. setOutputStream(new 144. OutputStream() 145. { 146. public void write(int b) {} // not called 147. public void write(byte[] b, int off, int len) 148. { 149. output.append(new String(b, off, len)); 150. } 151. }); 152. } 153. 154. public void publish(LogRecord record) 155. { 156. if (!frame.isVisible()) return; 157. super.publish(record); 158. flush(); 159. } 160. 161. private JFrame frame; 162. } 
java.util.logging.Logger 1.4 Logger getLogger(String loggerName) Logger getLogger(String loggerName, String bundleName) get the logger with the given name. If the logger doesn't exist, it is created. Parameters: | loggerName | The hierarchical logger name, such as com.mycompany.myapp | | bundleName | The name of the resource bundle for looking up localized messages |
void severe(String message) void warning(String message) void info(String message) void config(String message) void fine(String message) void finer(String message) void finest(String message) log a record with the level indicated by the method name and the given message. void entering(String className, String methodName) void entering(String className, String methodName, Object param) void entering(String className, String methodName, Object[] param) void exiting(String className, String methodName) void exiting(String className, String methodName, Object result) log a record that describes entering or exiting a method with the given parameter(s) or return value. void throwing(String className, String methodName, Throwable t) logs a record that describes throwing of the given exception object. void log(Level level, String message) void log(Level level, String message, Object obj) void log(Level level, String message, Object[] objs) void log(Level level, String message, Throwable t) log a record with the given level and message, optionally including objects or a throwable. To include objects, the message must contain formatting placeholders {0}, {1}, and so on. void logp(Level level, String className, String methodName, String message) void logp(Level level, String className, String methodName, String message, Object obj) void logp(Level level, String className, String methodName, String message, Object[] objs) void logp(Level level, String className, String methodName, String message, Throwable t) log a record with the given level, precise caller information, and message, optionally including objects or a throwable. void logrb(Level level, String className, String methodName, String bundleName, String message) void logrb(Level level, String className, String methodName, String bundleName, String message, Object obj) void logrb(Level level, String className, String methodName, String bundleName, String message, Object[] objs) void logrb(Level level, String className, String methodName, String bundleName, String message, Throwable t) log a record with the given level, precise caller information, resource bundle name, and message, optionally including objects or a throwable. Level getLevel() void setLevel(Level l) get and set the level of this logger. Logger getParent() void setParent(Logger l) get and set the parent logger of this logger. Handler[] getHandlers() gets all handlers of this logger. void addHandler(Handler h) void removeHandler(Handler h) add or remove a handler for this logger. boolean getUseParentHandlers() void setUseParentHandlers(boolean b) get and set the "use parent handler" property. If this property is TRue, the logger forwards all logged records to the handlers of its parent. Filter getFilter() void setFilter(Filter f) get and set the filter of this logger. 
java.util.logging.Handler 1.4 abstract void publish(LogRecord record) sends the record to the intended destination. abstract void flush() flushes any buffered data. abstract void close() flushes any buffered data and releases all associated resources. Filter getFilter() void setFilter(Filter f) get and set the filter of this handler. Formatter getFormatter() void setFormatter(Formatter f) get and set the formatter of this handler. Level getLevel() void setLevel(Level l) get and set the level of this handler. 
java.util.logging.ConsoleHandler 1.4 
java.util.logging.FileHandler 1.4 FileHandler(String pattern) FileHandler(String pattern, boolean append) FileHandler(String pattern, int limit, int count) FileHandler(String pattern, int limit, int count, boolean append) construct a file handler. Parameters: | pattern | The pattern for constructing the log file name. See Table 11-3 on page 585 for pattern variables. | | limit | The approximate maximum number of bytes before a new log file is opened. | | count | The number of files in a rotation sequence. | | append | true if a newly constructed file handler object should append to an existing log file. |

java.util.logging.LogRecord 1.4 Level getLevel() gets the logging level of this record. String getLoggerName() gets the name of the logger that is logging this record. ResourceBundle getResourceBundle() String getResourceBundleName() get the resource bundle, or its name, to be used for localizing the message, or null if none is provided. String getMessage() gets the "raw" message before localization or formatting. Object[] getParameters() gets the parameter objects, or null if none is provided. Throwable getThrown() gets the thrown object, or null if none is provided. String getSourceClassName() String getSourceMethodName() get the location of the code that logged this record. This information may be supplied by the logging code or automatically inferred from the runtime stack. It might be inaccurate, if the logging code supplied the wrong value or if the running code was optimized and the exact location cannot be inferred. long getMillis() gets the creation time, in milliseconds, since 1970. long getSequenceNumber() gets the unique sequence number of this record. int getThreadID() gets the unique ID for the thread in which this record was created. These IDs are assigned by the LogRecord class and have no relationship to other thread IDs. 
java.util.logging.Filter 1.4 
java.util.logging.Formatter 1.4 abstract String format(LogRecord record) returns the string that results from formatting the given log record. String getHead(Handler h) String getTail(Handler h) return the strings that should appear at the head and tail of the document containing the log records. The Formatter superclass defines these methods to return the empty string; override them if necessary. String formatMessage(LogRecord record) returns the localized and formatted message part of the log record. |