Data binding gives you extensive control over your data. You can bind nearly any type of data to any control or property of a control on an ASP.NET page. This gives you complete control over how data moves from the data store to the page and back again. You can simply display the data to the user, use it to set the style properties on a control, or even allow the user to modify the data directly and update the data store. There are two ways to bind data to an ASP.NET page: use a control's DataSource property, or use a data-binding expression. The first method is most commonly used for more complex ASP.NET server controls and will be covered in a minute. The second method can be used anywhere. The syntax for a data-binding expression is as follows: <%# property or collection %> Note Although this syntax looks very similar to code render blocks used with traditional ASP, it's not. Code render blocks are always evaluated at page runtime. Data-binding expressions are only evaluated using the DataBind() method. It's that simple. This expression will do different things depending on where it's used. Take Listing 9.4, for example, which declares a few variables and then binds them to various place in the page. Listing 9.4 Binding Data to Different Places in a Page 1: <script runat="server"> 2: dim strName as String = "Chris" 3: dim myArray() as String = {"Hello", "World"} 4: dim myString as String = "Chris" 5: 6: sub Page_Load(Sender as Object, e as EventArgs) 7: Page.DataBind() 8: end sub 9: </script> 10: 11: <html> 12: <form runat="server"> 13: ... 14: My Name is <%# strName %> 15: 16: <asp:Listbox datasource='<%# myArray %>' runat="server" /> 17: 18: <asp:Textbox text='<%# myString %>' runat="server" /> 19: ... 20: </form> 21: </html> Line 14 displays the value of strName next to the HTML output "My Name is". On line 16, the ListBox takes a collection and automatically loops through it to properly display the values of myArray as list items. Line 18 produces a text box that displays whatever is in myString. Thus, you can see that data binding offers a lot of flexibility. In lines 14 and 18, data binding doesn't provide you much benefit; after all, you could just write the text in the variables' places. However, the ListBox on line 16 is a different case. Data binding allows the ListBox to iterate over the items in the array, and fill itself up with strings. Without data binding, you'd have to manually fill up the ListBox with some kind of loop. Caution Be aware that your data-binding expressions must return types that are expected by your controls. For example, the text box control on line 18 expects a string value. If the data-binding expression doesn't produce one, you'll receive an error. It's often helpful to cast your data-binding expressions. For example, even though it might not be necessary here, changing line 18 to <asp:Textbox text='<%# myString.ToString %>' runat="server" /> ensures that the data is in the proper format. Data-binding expressions are only evaluated when the DataBind method is called ASP.NET doesn't process them for you automatically. You have a lot of choices about when and where to call this method. If you call DataBind at the page level, every data-binding expression on the page will be evaluated. This is typically accomplished in the Page_Load event: sub Page_Load(Sender as Object, e as EventArgs) DataBind() end sub You can also call DataBind for each control individually, which gives you greater control over how your application uses data. For instance, imagine that you allow users to view and modify their personal information on your Web site. You could display all of their information on one page but let them update only one field at a time. This would save you the hassle of collecting and verifying every single field on the page again. Listing 9.5 shows an example. Listing 9.5 Different Ways to Use DataBind 1: Sub Page_Load(Sender as object, e as EventArgs) 2: 'do some stuff 3: DataBind() 4: End Sub 5: 6: Sub Submit_Click(Sender as object, e as EventArgs) 7: if Text1.TextChanged then 8: Label1.DataBind() 9: end if 10: end sub  | The first Sub on lines 1 4 binds all the data on the page as soon as the page is loaded. This is a very typical situation, and often that's what you'll want to do. The second Sub, on lines 6 10, is the event handler for the Click event of a Submit button. If this subprocedure determines that the text in the Text1 control has changed, it evaluates the data-binding expression for the Label1 control only. This is very helpful when users modify data fields. | Using Data Binding This flexible syntax gives you a lot of freedom about how you use data in your pages. However, how do you create and use the data sources? The simplest way is to create a page-level variable. This is a variable that isn't contained within a method. You can then use this value anywhere within your page in a data-binding expression: <script language="VB" runat="server"> dim strName as string = "My Name" sub Page_Load(Sender as Object, e as EventArgs) DataBind() end sub </script> ... ... <asp:Label runat="server" text='<%# strName %>' /> <%# strName %> The last two lines show two examples of binding this page property. It's as simple as that. This doesn't do a lot for you, however. You could actually, in the Page_Load event, simply use the following command to fill the label: lblName.Text = "My Name" The true power of data binding comes from using dynamic values in server controls. Let's take a look at an example in Listing 9.6. Listing 9.6 Binding One Server Control to Another 1: <script language="VB" runat="server"> 2: sub Index_Changed(Sender as Object, e as EventArgs) 3: DataBind() 4: end sub 5: </script> 6: 7: <html><body> 8: <form runat="server"> 9: 10: <asp:Listbox runat="server" 11: width="150" 12: AutoPostBack=true 13: rows="1" 14: SelectionMode="Single" 15: OnSelectedIndexChanged="Index_Changed" > 16: <asp:Listitem value="1">Red</asp:Listitem> 17: <asp:Listitem value=" 2">Blue</asp:Listitem> 18: <asp:Listitem value=" 3">Green</asp:Listitem> 19: <asp:Listitem value=" 4">Yellow</asp:Listitem> 20: </asp:Listbox><p> 21: 22: <asp:Label runat="server" 23: Text='<%# List1.selectedItem.Text %>' /> 24: </form> 25: </body></html> The Label on line 22 uses a data-binding expression that binds to the SelectedItem property of the ListBox. Whenever the selected index changes, you call DataBind again to bind the new value to the Label. The effect is that the Label will show whatever is selected. This is just an example of how you can bind to any public property of the page. Figure 9.4 shows the output of this page. Figure 9.4. Displaying selected data with data binding.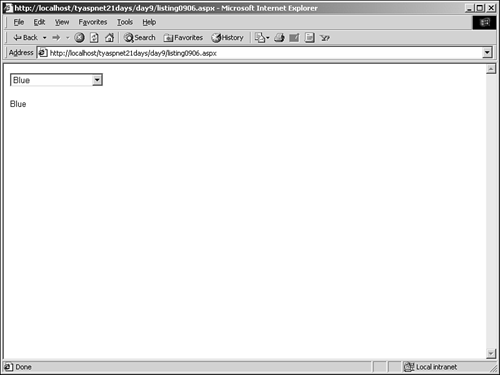 Some server controls can also bind to data classes, rather than simple properties, for more elaborate data manipulation. These controls have a DataSource property that's accessible only at design time. Simply set this property to a data class (such as an array or data view) and call DataBind, and these controls handle much of the work for you. Listing 9.7 expands on the previous example. Listing 9.7 Using the DataSource Property 1: <script runat="server"> 2: sub Page_Load(Sender as Object, e as EventArgs) 3: if not Page.IsPostBack then 4: 'create an array of colors 5: dim arrColors() as string = _ 6: {"red", "orange", "yellow", "green", _ 7: "blue", "indigo", "violet"} 8: lbColors.SelectedIndex = 0 9: lbColors.DataSource = arrColors 10: end if 11: DataBind() 12: end sub 13: </script> 14: 15: <html><body> 16: <form runat="server"> 17: <asp:Listbox runat="server" 18: width="150" 19: AutoPostBack="true" 20: SelectionMode="Single" > 21: </asp:Listbox><p> 22: 23: <asp:Label runat="server" 24: Text='<%# lbColors.SelectedItem.Text %>' /> 25: </form> 26: </body></html>  | You create a data class (an array in this case) on line 5 and set the listbox's DataSource property on line 9. Now the listbox populates itself automatically using the array. The label works exactly as it did before when the selection changes, it updates itself. There is one difference here, however. When you first load the page, the ListBox doesn't have an item selected, and therefore, lines 23 24 will cause an error; you can't bind to the SelectedItem property if nothing is selected. Therefore, on line 8, you select the first item in the ListBox. | Figure 9.5 shows this page in action. Figure 9.5. Binding data at design time.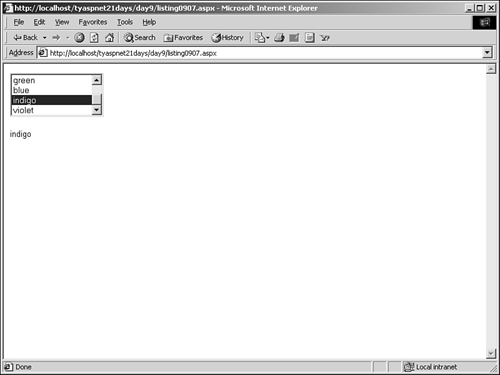 Notice the addition of the Postback check on line 2. You only need to create your array the first time the page is viewed. After that, the listbox fills itself automatically using the built-in viewstate management. Therefore, you add a check to determine if the form has been submitted. If it has, you don't need to repopulate the listbox. This check also serves another purpose if you take it out, you receive an Object reference not set to an instance of an object. error from the label. Let's examine the flow of the page to figure this one out. See Figure 9.6. Figure 9.6. On the left is the workflow without a Postback check. On the right is the workflow with a Postback check.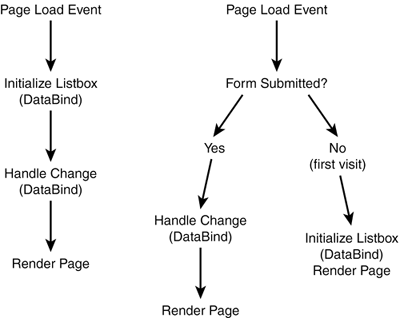 Let's assume that your page has been submitted. In your Page_Load event, you set the DataSource property of the listbox and bind it. Essentially, this reinitializes the listbox and destroys the state information. Then the Index_Changed event fires, which evaluates all data-binding expressions on the page. The data-binding expression for the label tries to use the SelectedItem property of the listbox. Because you eliminated the state information, the reference to SelectedItem returns a null object, which throws an error. Thus, you add the postback check, which prevents the page from reinitializing the listbox when the form is submitted. Beware of little things like these. Because of the way the event-driven model works, you must be careful when binding data on a page. Now that you know how data binding works, you might wonder how it benefits users of your ASP.NET pages. The answer is that it doesn't do much at all for your users, unless you count the fact that it cuts development time for you and speeds site delivery for them. The biggest benefit of data binding is the features it provides you, as the developer: decreased development time, ease of use, standardized methods, and so on. Now you're ready to take a look at some of the more complex ASP.NET server controls that use data binding. |