In this example, we will look at various techniques for inserting and deleting elements within an array. -
Open your text editor and type the code in Listing 10.4 or open the Array2.cfm file from the CompletedFiles\Examples\Step10 folder. Listing 10.4 Array2.cfm <!--- File: Array2.cfm Description: Demo the working with array elements Author: Created: ---> <HTML> <HEAD> <TITLE>Array 2</TITLE> </HEAD> <BODY> <!--- create the array ---> <CFSET aDepts=ArrayNew(1)> <TABLE BORDER="1" CELLSPACING="4"> <TR VALIGN="top"> <TD> <!--- populate the array with data ---> <CFSET aDepts[1]="Marketing"> <CFSET aDepts[2]="Operations"> <CFSET aDepts[3]="Sales"> <CFSET aDepts[4]="Technology"> <P>Array Has Been Populated</P> <!--- dump the array ---> <CFDUMP VAR="#aDepts#"> </TD> <TD> <CFSET temp=ArrayInsertAt(aDepts,3,"Legal")> <P>'Legal' Has Been Inserted</P> <!--- dump the array ---> <CFDUMP VAR="#aDepts#"> </TD> <TD> <CFSET temp=ArrayDeleteAt(aDepts,1)> <P>'Marketing' Has Been Deleted</P> <!--- dump the array ---> <CFDUMP VAR="#aDepts#"> </TD> <TD> <CFSET temp=ArrayClear(aDepts)> <P>The Array Has Been Cleared</P> <!--- dump the array ---> <CFDUMP VAR="#aDepts#"> </TD> <TD> <CFSET temp=ArraySet(aDepts,1,5,"full")> <P>Use ArraySet to prefill the array</P> <!--- dump the array ---> <CFDUMP VAR="#aDepts#"> </TD> </TR> </TABLE> </BODY> </HTML> -
Save the file as Array2.cfm in your Example\Step10 folder. -
Browse to the Array2.cfm file. You should see something similar to Figure 10.6. Figure 10.6. The Array2.cfm browser display. 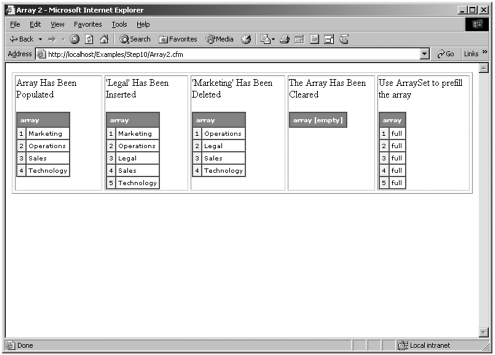 Multidimensional Arrays So far we have only been looking at one-dimensional arrays, but we can also create two- and three-dimensional arrays. Whereas a one-dimensional array simply has a row for each element value, two-dimensional arrays have rows and columns similar in layout to a spreadsheet. Figure 10.7 shows an example of a two-dimensional array. Figure 10.7. A two-dimensional array. 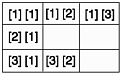 Notice in Figure 10.7 that we have several rows and columns and that each element in a two-dimensional array is referenced by two index numbers. The first number represents a row value; the second number represents a column value. Also note that the array does not have to be symmetrical, meaning we can have a different number of column values for each row. A three-dimensional array can be thought of as a cube or a stack of two-dimensional arrays, as illustrated in Figure 10.8. Figure 10.8. Three-dimensional arrays. 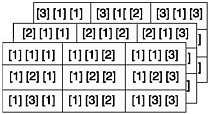 Elements within a three-dimensional array are referenced by three index numbers. The first number represents the plane or sheet in the stack, the second number represents the row in that plane, and the third number represents the column in that row. Looping Through Arrays A popular technique for both populating arrays and displaying array values is the use of loops. Using loops and nested loops, it is possible to loop through all the values in a given array. For example, the following code uses two nested loops to populate the elements of a two-dimensional array. Figure 10.9 shows the <CFDUMP> for the array created. Figure 10.9. A populated two-dimensional array. 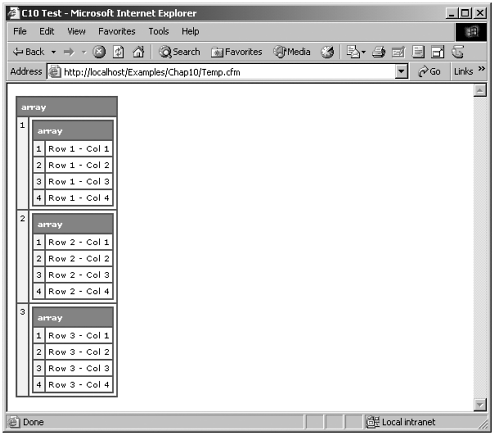 <!--- create a 2D array ---> <CFSET aMy2DArray=ArrayNew(2)> <!--- create 3 rows with 4 columns ---> <!--- outer loop, used for each row ---> <CFLOOP INDEX="x" FROM="1" TO="3"> <!--- inner loop, used for each column ---> <CFLOOP INDEX="y" FROM="1" TO="4"> <CFSET aMy2DArray[x][y]="Row #x# - Col #y#"> </CFLOOP> </CFLOOP> <!--- dump the array ---> <CFDUMP VAR="#aMy2DArray#"> NOTE Earlier in this step, I mentioned that one of the advantages of an array is that it can store complex variable types such as queries and other arrays. Although it is convenient to think of two-dimensional arrays in terms of rows and columns, strictly speaking this is not how they are organized. If you look closely at the array dump in Figure 10.9, you will see a one-dimensional array with three elements representing each of our rows. Each element within that one-dimensional array contains another one-dimensional array, this time with four elements each. These elements represent our column elements. A three-dimensional array actually has an array inside an array inside an array. As you can see, this really starts to hurt your brain after a while, and it is far easier to think of arrays in terms of columns and rows. You can also use loops to display the elements within an array. The trick here is knowing how big the array is before we start looping through it. We can use the ArrayLen() function to determine the length of a given array. The following code renders an HTML table of our aMy2DArray variable. Figure 10.10 shows the browser output for this code. Figure 10.10. An HTML table display. 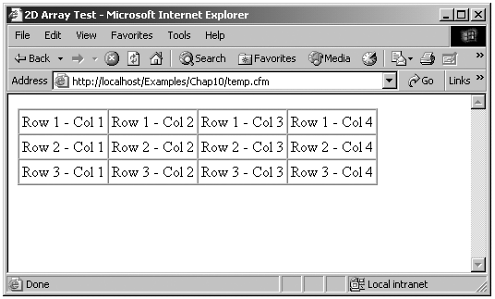 <!--- create a table ---> <TABLE BORDER="1" CELLPADDING="3" CELLSPACING="0"> <!--- outer loop, value of x for each row ---> <CFLOOP INDEX="x" FROM="1" TO="#ArrayLen(aMy2DArray)#"> <TR> <!--- inner loop, value of y for each column ---> <CFLOOP INDEX="y" FROM="1" TO="#ArrayLen(aMy2DArray[x])#"> <TD> <CFOUTPUT>#aMy2DArray[x][y]#</CFOUTPUT> </TD> </CFLOOP> </TR> </CFLOOP> </TABLE> The preceding code uses the ArrayLen() function to determine how many rows our array has and then begins to loop through them, creating a new table row for each row in the array. Then we use a nested loop for each column value. The ArrayLen() function in the nested loop checks to see how many second-dimension values each particular row has and then loops through them, creating a new table cell for each value. Queries and Arrays Repeated queries to a database to retrieve fairly static information can be costly from a performance standpoint. A popular technique to ease the burden is to query information once and then store it within an array variable stored in the Application or Session scope. By doing this, we only have to query the database once, but the information (now stored in a shared scope) is available to any template within the application without having to query the database again. The question is, how do we get the information from the query into an array? The answer is by using a special type of loop called a Query loop. The Query loop simply loops through a query result recordset in much the same way that the <CFOUTPUT> tag does. Instead of using an INDEX value within the loop, however, we simply use the CurrentRow value of the recordset. The Query loop syntax is as follows. The STARTROW and ENDROW attributes are optional. <CFLOOP QUERY="QueryName" STARTROW="Startrow" ENDROW="endrow"> <!--- loop logic ---> </CFLOOP> |