Although Web Services are formally a part of ASP.NET, you can write client applications that take advantage of the Web Services that run either on the client or on a Web server. In this section, you'll create a client-side application, using Windows Forms, to demonstrate the techniques involved in consuming an XML Web Service. When you decide to consume a Web Service as part of your application, the Web Service might be located on your local intranet, it might be located at a corporate office that's only accessible through the Internet, or it might be a public service. Whatever the scenario, the steps to consume the service are the same. In general, you'll always need to perform these steps: -
Add a Web Reference, retrieving information about the Web Service. -
Create an object in your code that corresponds to the service. -
Call a method of the object that's created in the previous step. Creating a Client Application In this section, you'll create a simple Windows Forms application that calls the Web Service you created earlier in the chapter. Follow these steps to create the new project and test the service: -
Open a second instance of Visual Studio .NET. (You're leaving the first instance open only for convenience. If you don't want to run two copies of Visual Studio .NET concurrently, you can close the previous instance.) -
Create a new project, selecting Windows Application from the list of project templates. -
Set the name of the project to WebServiceClient and the location to any convenient location (perhaps within the Jumpstart folder, to keep all your examples in one place). -
Click OK to create the project. -
Use the View, Properties Window menu item to display the Properties window. -
Set the Text property for the form to Add Two Numbers. -
Add three Label controls, three TextBox controls, and button so that the form looks like Figure 29.4. Figure 29.4. The finished form should look like this.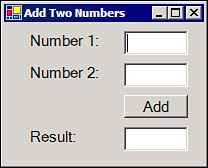 -
Set the Name properties for the TextBox controls to txtNum1, txtNum2, and txtResults, respectively. -
Set the Name property for the Button control to btnAdd. Adding the Web Reference Now that you've created the basic form, you can hook up the Web Service. Follow these steps to add the Web reference: -
In the Solution Explorer window, right-click the project. Select Add Web Reference from the context menu. (You could also select the Project, Add Web Reference menu item.) -
Visual Studio .NET displays the Add Reference dialog box, shown in Figure 29.5. You could search for a service using UDDI (as you did in the previous chapter), but this time, you'll enter the exact address of the service you created in the previous section. Enter a full address, like this, into the Address text box and then press Enter: Figure 29.5. Enter the address of the Web Service you'd like to use.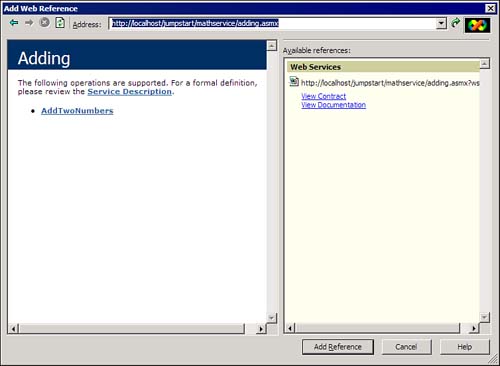 http://localhost/jumpstart/mathservice/adding.asmx -
Click Add Reference to add the reference to your project. -
Take a look at the Solution Explorer window it should look something like Figure 29.6 (we've expanded the node corresponding to the new Web reference). Note that adding the reference also added a few new files to your project most notably, the adding.wsdl file, which contains information about the methods, parameters, and return values in the Web Service. Figure 29.6. Adding a Web reference adds other files, as well.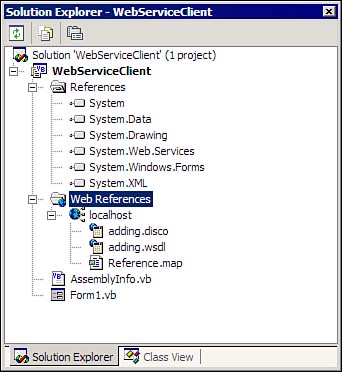 TIP Although you could rename the Web reference (you did this in the previous chapter), it's not required. If you'd like to rename localhost to some other name, feel free, although you'll need to modify any code in the rest of this example that refers to localhost explicitly. Calling the Web Service Method Once you've added the Web reference to your project, you can call the methods provided by the Web Service from within your project's code. Follow these steps to test calling the AddTwoNumbers method from your form: -
On your form, double-click the Add button. -
Modify the btnAdd_Click procedure so that it looks like this: ' Code Fragment 2. Private Sub btnAdd_Click( _ ByVal sender As System.Object, _ ByVal e As System.EventArgs) _ Handles btnAdd.Click Dim ws As New localhost.Adding() txtResults.Text = ws.AddTwoNumbers( _ CInt(txtNum1.Text), CInt(txtNum2.Text)).ToString End Sub -
Press F5 to run the application. -
Enter two values into the text boxes on the form, press Add, and, after a few seconds, you'll see the results appear on the form. When you're done, the form should look like Figure 29.7. Figure 29.7. The sample form displays the results of calling the Web Service method.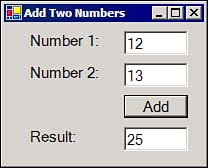 -
Close the form when you're done. What's going on in the code? The code starts by instantiating a localhost.Adding object: Dim ws As New localhost.Adding() The procedure finished by calling the AddTwoNumbers method of the object you created, passing in the Text properties of the two TextBox controls on the form. It converted the result into a String value for display in the Result text box, like this: txtResults.Text = ws.AddTwoNumbers( _ CInt(txtNum1.Text), CInt(txtNum2.Text)).ToString The remaining question is, Where did the localhost.Adding object come from? How did your project know how to find this remote object? The simple answer is, of course, that when you added the Web reference to your project, you added code that takes care of these details for you. If you use the Project, Show All Files menu item, you'll see that the Reference.map item in the Solution Explorer window includes a code-behind file named Reference.vb, as shown in Figure 29.8. This file contains a namespace that matches the name of your reference (localhost, in this case) and a class with the same name as your Web Service class (Adding, in this case). Figure 29.8. Adding a Web reference adds a new code file, as well.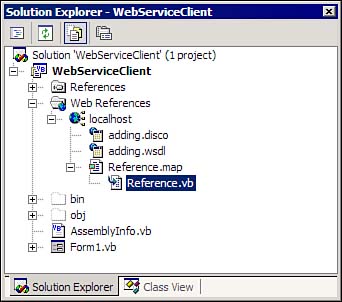 Therefore, when your code refers to localhost.Adding, you're actually working with the Adding class within the localhost namespace in the Reference.vb file in your project, not within a remote Web Service class. (This class is often called a proxy class, because it acts as a proxy for the real class in the remote Web Service.) If you examine the code within the Adding class in Reference.vb, you'll find an AddTwoNumbers procedure (which explains how your code can call the method named AddTwoNumbers). This local AddTwoNumbers procedure includes code that manages the call to the real Web Service procedure, so you don't need to worry about how it all happens. If you're interested, dig into the code in Reference.vb, although it's not important to understand all the details at this point. NOTE Perhaps you're wondering how you managed to get IntelliSense tips as you were typing the code that called the Web Service method. Because adding the Web reference adds the local proxy class containing the same information as in the Web Service, Visual Studio .NET can provide design-time help just as it does with local objects. As you've seen, the most exciting feature of Web Services is that you don't have to know who created the service, or even in what language, development environment, or operating system. To your client applications, all that matters is the WSDL the information about what methods are available, what parameters to send, and what data to expect back. If you've selected a Web Service from a reputable source, you can consume it just like any local object. TIP You've seen how to create a simple Windows application that consumes an XML Web Service. Creating a Web application that consumes a Web Service is no more difficult as a matter of fact, it takes exactly the same steps to create a Web application that consumes a Web Service. You might want to try re-creating the consumer application you just created as a Web page. You'll see that you can follow the same exact steps and achieve the same results. |