Although the Web Service you create in this section is really simple, it shows off many of the issues you'll face when creating any Web Service, and its simplicity makes it a good example to get you started. Follow these steps to create your first XML Web Service: -
In Visual Studio .NET, create a new project. Select ASP.NET Web Service from the list of project templates. -
Set the location of your service to http://localhost/Jumpstart/MathService. Click OK to create the project. -
By default, the Web Service project template creates a component named Service1.asmx. Find this component in the Solution Explorer window and rename it to Adding.asmx. NOTE If you double-click Adding.asmx to display it in the designer, you'll note that it's empty but that there is a designer surface for the object. You might wonder why, given that you can't place Web Form controls on the designer. Microsoft supplies this designer so that you can use items on the Data and Components tabs of the Toolbox window. You can drag a SqlDataAdapter object onto the designer and have access to data from within your service. The same goes for all the objects provided on the Components tab. It's worth your time to investigate what tools Microsoft has provided on this tab perhaps they can save you some time in a future application. -
In the Solution Explorer window, right-click Adding.asmx and select View Code from the context menu. The code should look like Listing 29.1. (We've removed the hidden region and reformatted the code a bit to make it fit on the printed page.) Listing 29.1 When You First Create a Web Service Component, You'll Find This Code Inside Imports System.Web.Services <WebService(Namespace := "http://tempuri.org/")> _ Public Class Service1 Inherits System.Web.Services.WebService ' WEB SERVICE EXAMPLE ' The HelloWorld() example service returns the string ' Hello World. ' To build, uncomment the following lines then save ' and build the project. ' To test this web service, ensure that the .asmx file ' is the start page ' and press F5. ' '<WebMethod()> Public Function HelloWorld() As String ' HelloWorld = "Hello World" ' End Function End Class NOTE Isn't it nice that Microsoft supplied a sample Web Service method for you? Too bad it doesn't do anything even vaguely useful. You'll always want to remove the sample template code. -
Modify the class name from Service1 to Adding. -
Modify the default namespace (http://tempuri.org/) and replace it with http://www.northwind.com/ws instead. When you're done, the attribute should look like this: <WebService(Namespace:="http://www.northwind.com/ws")> _ Public Class Adding ... End Class NOTE The WebService attribute, associated with the class itself, allows you to specify information about the Web Service class. In this case, you're specifying the namespace associated with the class. Every Web Service must have some name tied to it (its namespace the SOAP specification requires this), and by default, Visual Studio .NET uses a sample namespace, http://tempuri.org/ For any real Web Service, you should replace this with a unique name normally a name that represents your organization. If your company's URL is http://www.northwind.com, for example, you could be guaranteed that no other company would use the same URL as its own namespace. Of course, there's no rule that forces developers to use a unique name here, and it's not crucial at this point that you do, but as Web Services proliferate, it will be useful if you identify your services with some unique "tag" in the service's Namespace property. If you don't modify the default namespace name, you'll see warnings later on, as you test your Web Service. -
Delete the commented-out sample procedure, if you like. -
Add the following code to the Adding class: ' Code Fragment 1. <WebMethod()> _ Public Function AddTwoNumbers( _ ByVal Number1 As Integer, _ ByVal Number2 As Integer) As Long Return Number1 [PLUS] Number2 End Function If you take a moment to investigate the AddTwoNumbers method, you'll see that it's just standard VB .NET code. Nothing special is going on here, yet you're creating an XML Web Service that can work across the Web, using HTTP to transport values in and out of the method. How does this work? The answer is in the one extra attribute you've added to the procedure: The WebMethod attribute indicates to the compiler that your procedure is to be treated specially. It will send and receive its input and output using XML (encased in SOAP packets), and Visual Studio .NET will take extra steps to expose the functionality of the method inside an XML Web Service for you. What can you do in a Web Service method? You can do just about anything you might do in any other code, except that you can't display a user interface. Obviously, because the Web Service method will be running on a remote computer, you don't want it to have any user interaction. Other than that, however, you can use any of the .NET Framework and its objects within your Web Service method. Testing the Web Service Although you'll generally consume the Web Service methods you create from within real applications, it's easy to test your method without needing to create a new application. When you simply browse directly to the Adding.asmx page you've created, ASP.NET renders a test page for you. Try it out by following these steps: -
Press F5 to run the project. -
Visual Studio .NET displays the test page with a link for each method you've created, as shown in Figure 29.1. Figure 29.1. The test page for your Web Service allows you to try out the methods provided by the service.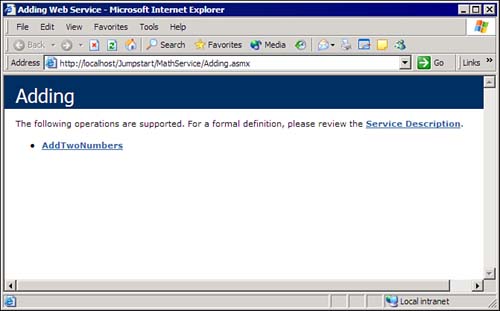 -
Click the AddTwoNumbers link, bringing up the test page shown in Figure 29.2. Figure 29.2. ASP.NET provides a test page for each Web Service method.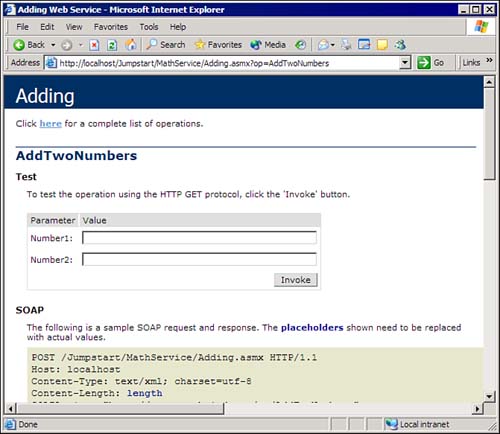 TIP You might find it interesting to scroll your test page down and view the information about the SOAP packets created for you by the .NET Framework. Isn't it nice to know about all the work you don't have to do? -
Provide the two requested numeric values and then click Invoke to test your method. -
Once you're done, you'll see a page like the one shown in Figure 29.3, containing the results of your Web Service method call. (For this example, we entered 12 and 13 into the two text boxes on the previous page.) Figure 29.3. The Web Service method returns its value in XML.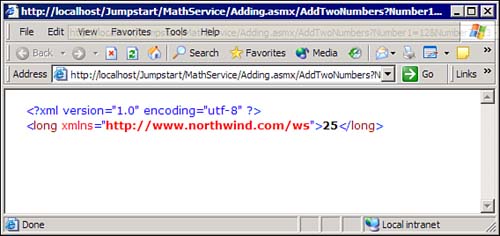 Before you close the browser window, investigate the URL in the Address text box. You should see text like this: http://localhost/Jumpstart/MathService/Adding.asmx/ AddTwoNumbers?Number1=12&Number2=13 You can test a Web Service method directly, if you know the name of the method and its parameter names, simply by entering a URL like this one. If you need to consume a Web Service from a non-.NET client, for example, you could create an HTTP request within your code, using a URL like this one as your target. The response would contain the results shown in Figure 29.3. |