Although a service that adds two numbers together has its uses (although it might be difficult to determine those uses, except for demonstration purposes), you might be more likely to create a Web Service that interacts with a data source and provides information based on that data. In this section, you'll create a Web Service that retrieves information from the Northwind sample database in SQL Server. You'll provide two methods: GetInventory. This method returns the current, available inventory for a specific product. GetAllInventory. This method returns a DataSet containing the inventory level for all products. There really isn't much new in these procedures, because the techniques you've already learned for manipulating data all apply here. To get started creating your service, follow these steps: -
Create a new project in Visual Studio .NET, selecting the ASP.NET Web Service project template. -
Set the location for your service to http://localhost/JumpStart/InventoryService. -
Rename the Service1.asmx file to Inventory.asmx. -
Select the View, Code menu item and modify the class name from Service1 to Inventory. -
Modify the default namespace (http://tempuri.org/) and replace it with http://www.northwind.com/ws instead. -
Delete the commented-out sample code within the class. When you're done, the class should look like Listing 29.2. Listing 29.2 The Starting Point for Your Web Service Looks Like This Imports System.Web.Services <WebService(Namespace:="http://www.northwind.com/ws")> _ Public Class Inventory Inherits System.Web.Services.WebService End Class -
Scroll to the top of the file and add the following Imports statement: Imports System.Data.OleDb -
Add the following code to the Inventory class: Private Const CONNECTION_STRING As String = _ "Provider=sqloledb;" & _ "Data Source=(local);" & _ "Initial Catalog=Northwind;" & _ "User ID=sa;Password=" <WebMethod()> Public Function UnitsInStock( _ ByVal ProductID As Integer) As Integer Dim strSQL As String = _ "SELECT UnitsInStock FROM Products " & _ "WHERE ProductID = " & ProductID Dim cnn As OleDbConnection Dim cmd As OleDbCommand Dim intUnits As Integer Try cnn = New OleDbConnection(CONNECTION_STRING) cnn.Open() cmd = New OleDbCommand(strSQL, cnn) UnitsInStock = cmd.ExecuteScalar() Finally If cnn.State = ConnectionState.Open Then cnn.Close() End If End Try End Function The UnitsInStock method opens a connection to the data source and executes a Command object that returns the single required value the available units in stock for the requested product. To test your Web Service method, follow these steps: -
Press F5 to run the project. -
On the test page, click the UnitsInStock link. -
Enter a value (say, 12) into the ProductID text box. Click Invoke to test the method. -
You should see the results, formatted as XML, within the browser window. Returning a DataSet from a Web Service Every sample method exposed in a Web Service that you've seen so far returns a single value. There's no reason, however, that a Web Service method can't return a complex result, such as a DataSet. To finish this chapter, you'll create a Web Service method that returns a complete DataSet, and then you'll create a simple page that displays the results of calling the Web Service method in a DataGrid control. Follow these steps to create and consume the Web Service method: -
Add the following method to the Inventory class in Inventory.asmx: <WebMethod()> Public Function GetAllInventory() As DataSet Dim strSQL As String = _ "SELECT ProductID, ProductName, " & _ "UnitPrice, UnitsInStock " & _ "FROM Products ORDER BY ProductName" Dim da As OleDbDataAdapter Dim ds As New DataSet() Try da = New OleDbDataAdapter(strSQL, CONNECTION_STRING) da.Fill(ds) Catch Throw End Try Return ds End Function -
Test your method, just as you did in the previous section. The return value should contain a large amount of XML data, as shown in Figure 29.9. This XML contains the DataSet returned by your Web Service method. Figure 29.9. A Web Service method that returns a DataSet creates output like this.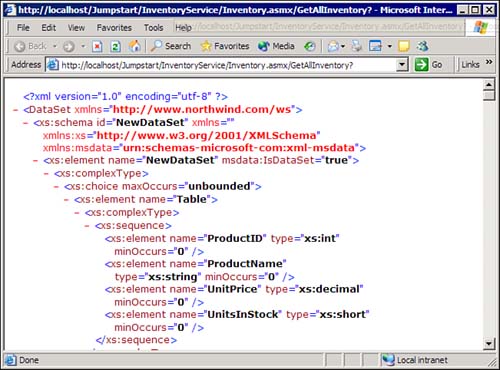 -
Save your project. -
Create a new ASP.NET Web Application project, selecting the location http://localhost/JumpStart/WebServiceConsumer for your project. -
Add a Web reference to your project using this URL: http://localhost/jumpstart/inventoryservice/inventory.asmx TIP Refer back to the previous example for complete steps and hints on adding a Web reference to your project. -
Add a DataGrid control to your page. Set the control's ID property to grdInventory. -
Double-click the page (not the control) and modify the Page_Load procedure so that it looks like this: Private Sub Page_Load( _ ByVal sender As System.Object, _ ByVal e As System.EventArgs) _ Handles MyBase.Load If Not Page.IsPostBack Then Dim ws As New localhost.Inventory() grdInventory.DataSource = ws.GetAllInventory() grdInventory.DataBind() End If End Sub -
Press F5 to run the project. You should see the inventory data displayed in the grid. What happened here? When your page loaded, it called the Web Service method, which returned a DataSet. Your code set the DataGrid control's DataSource property to the DataSet returned from the method and displayed the data. Couldn't be much easier than that, could it? Of course, between the Web Service and the consumer application, the .NET Framework serializes the DataSet as XML you saw that in Figure 29.9. Once the consumer application receives the XML, however, the proxy class indicates that the application should retrieve a DataSet object, and the .NET Framework converts the data back into its original data type, ready to be bound to the DataGrid control's DataSource property. |