File I/O in JSFL Introduced after the launch of Flash MX 2004, File I/O was one of the most requested features to be added to JSFL. A specific class, called Flfile, was created to handle this new feature. Flfile has several methods, but we are going to be using three for our example, the exists method, the remove method, and the write method. Here are those three methods with some explanation: exists (fullPath) This method checks to see if the file in the fullPath parameter exists; it returns a Boolean value TRue if the file does exist and false if the file does not exist. remove (fullPath) This method completely removes the file at fullPath and returns a Boolean value based on its success. write (fullPath, stringContent) This method creates a file at fullPath and fills it with the stringContent. Remember, all these methods require a full, absolute path to the file you are referencing. The example we are going to create, when run from within a Flash document, will create an Excel file that lists every single item in the library with some pertinent information about each one. We will first go over the command step-by-step and then show the code in its entirety. First, create a new JSFL file called createLibraryListXLS.jsfl and paste this first bit of code in it: //get the path of the doc var tPath = fl.getDocumentDOM().path; var fileName = tPath.slice(tPath.lastIndexOf("\\")+1,tPath.lastIndexOf(".")); tPath = tPath.slice(0,tPath.lastIndexOf("\\")); var filePath = "file:///"+tPath.split("\\").join("/")+"/"+fileName+"_library.xls"; This bit of code first gets the path to the open file you are calling the command on (FLA). It then extrapolates the filename from the full path to use as part of the Excel filename. Finally, it creates the new absolute file path of the file you are going to create at the end. After that bit of code, paste this small piece: //if the file already exists.. get rid of it if(FLfile.exists(filePath)){ FLfile.remove(filePath); } These three lines of code do a lot. They look to see if the file has already been made. If the file has been made before, it is destroyed so that later we can re-create it. The next section begins the content variable we are going to use to store everything we want put in the file. //start of the content var content=""; content+="<html>\n\t<body>\n"; content+="\t\t<table border='1'>\n"; content+="\t\t\t<tr>\n"; content+="\t\t\t\t<th nowrap>id</th>\n"; content+="\t\t\t\t<th nowrap>type</th>\n"; content+="\t\t\t\t<th nowrap>linkageClassName</th>\n"; content+="\t\t\t\t<th nowrap>linkageURL</th>\n"; content+="\t\t\t\t<th nowrap>linkageIdentifier</th>\n"; content+="\t\t\t\t<th nowrap>libraryPath</th>\n"; The preceding code creates the beginning of our file by creating all the header fields we will need in Excel. Next up is all the information in the library: [View full width] //now its time to run through the array of library items var itemArray= fl.getDocumentDOM().library.items; itemArray.sort(); var tLength = itemArray.length; var i=0; while(i<tLength){ var tItem = itemArray[i]; if(tItem.itemType != "folder"){ content+="\t\t\t<tr>\n"; content+="\t\t\t\t<td align='right'>"; content+=i; content+="</td>\n"; content+="\t\t\t\t<td align='right'><b>"; switch (tItem.itemType){ case "movie clip": content+="<font color='ff0000'>movie clip</font>"; break; case "bitmap": content+="<font color='006600'>bitmap</font>"; break; case "sound": content+="<font color='cc9900'>sound</font>"; break; case "graphic": content+="<font color='9933cc'>graphic</font>"; break; case "button": content+="<font color='0000ff'>button</font>"; break; case "compiled clip": content+="<font color='333300'>compiled clip</font>"; break; case "font": content+="<font color='cc9900'>button</font>"; break; case "video": content+="<font color='996600'>compiled clip</font>"; break; default: content+=tItem.itemType; } content+="</b></td>\n"; content+="\t\t\t\t<td align='right'>"; (tItem.linkageClassName)? content+="<i>"+ tItem.linkageClassName+"</i>" :content+="N/A"; content+="</td>\n"; content+="\t\t\t\t<td align='right'>"; (tItem.linkageURL)? content+="<i>"+tItem.linkageURL+"</i>":content+="N/A"; content+="</td>\n"; content+="\t\t\t\t<td align='right'>"; (tItem.linkageIdentifier)? content+="<b>"+ tItem.linkageIdentifier+"</b>" :content+="N/A"; content+="</td>\n"; content+="\t\t\t\t<td align='right'>"; content +=tItem.name; content+="</td>\n"; content+="\t\t\t</tr>\n"; } i++; } content+="\t\t</table>\n\t</body>\n</html>";
The preceding code looks like a lot, but it's mostly the switch statement (more on switch statements in Chapter 11, "Statements and Expressions"). We first grab a copy of all the items in the library as an array. Then we begin to go through them one by one, setting each row of our Excel spreadsheet with properties of each item; using the switch statement, we even color code each type of library item. When the loop is over, we close off the Excel spreadsheet and are ready to create the file with the next piece of code: //write the file FLfile.write(filePath,content); And that's the last line where we create the Excel file at filePath and write content to it. All together, the code looks like this: [View full width] //get the path of the doc var tPath = fl.getDocumentDOM().path; var fileName = tPath.slice(tPath.lastIndexOf("\\")+1,tPath.lastIndexOf(".")); tPath = tPath.slice(0,tPath.lastIndexOf("\\")); var filePath = "file:///"+tPath.split("\\").join("/")+"/"+fileName+"_library.xls"; //if the file already exists.. get rid of it if(FLfile.exists(filePath)){ FLfile.remove(filePath); } //start of the content var content=""; content+="<html>\n\t<body>\n"; content+="\t\t<table border='1'>\n"; content+="\t\t\t<tr>\n"; content+="\t\t\t\t<th nowrap>id</th>\n"; content+="\t\t\t\t<th nowrap>type</th>\n"; content+="\t\t\t\t<th nowrap>linkageClassName</th>\n"; content+="\t\t\t\t<th nowrap>linkageURL</th>\n"; content+="\t\t\t\t<th nowrap>linkageIdentifier</th>\n"; content+="\t\t\t\t<th nowrap>libraryPath</th>\n"; //now its time to run through the array of library items var itemArray= fl.getDocumentDOM().library.items; itemArray.sort(); var tLength = itemArray.length; var i=0; while(i<tLength){ var tItem = itemArray[i]; if(tItem.itemType != "folder"){ content+="\t\t\t<tr>\n"; content+="\t\t\t\t<td align='right'>"; content+=i; content+="</td>\n"; content+="\t\t\t\t<td align='right'><b>"; switch (tItem.itemType){ case "movie clip": content+="<font color='ff0000'>movie clip</font>"; break; case "bitmap": content+="<font color='006600'>bitmap</font>"; break; case "sound": content+="<font color='cc9900'>sound</font>"; break; case "graphic": content+="<font color='9933cc'>graphic</font>"; break; case "button": content+="<font color='0000ff'>button</font>"; break; case "compiled clip": content+="<font color='333300'>compiled clip</font>"; break; case "font": content+="<font color='cc9900'>button</font>"; break; case "video": content+="<font color='996600'>compiled clip</font>"; break; default: content+=tItem.itemType; } content+="</b></td>\n"; content+="\t\t\t\t<td align='right'>"; (tItem.linkageClassName)? content+="<i>"+ tItem.linkageClassName+"</i>" :content+="N/A"; content+="</td>\n"; content+="\t\t\t\t<td align='right'>"; (tItem.linkageURL)? content+="<i>"+tItem.linkageURL+"</i>":content+="N/A"; content+="</td>\n"; content+="\t\t\t\t<td align='right'>"; (tItem.linkageIdentifier)? content+="<b>"+ tItem.linkageIdentifier+"</b>" :content+="N/A"; content+="</td>\n"; content+="\t\t\t\t<td align='right'>"; content +=tItem.name; content+="</td>\n"; content+="\t\t\t</tr>\n"; } i++; } content+="\t\t</table>\n\t</body>\n</html>"; //write the file FLfile.write(filePath,content);
Now you can test this file by opening any Flash document that has items in its library and running the command, as shown in Figure 27.15. Figure 27.15. File I/O in JSFL opens up a lot of avenues for interesting and time-saving commands. 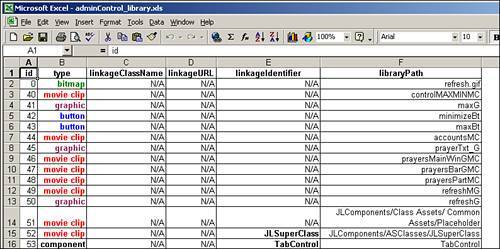 CAUTION It is important to understand that although File I/O is very powerful and fun to work with, it is also dangerous because it can in fact destroy and overwrite files. This is even more important to remember when installing and running commands from unknown third parties. Always check the code if it is not from a trusted source. And even if it is from a trusted source, check it anyway. TIP If you want to turn off the capability to have File I/O running completely, you can change the name of the file. PC users will find the file here: C:\Program Files\Macromedia\Flash 8\en\First Run\External Libraries\FLfile.dll and Mac users can find the file here: Mac:HardDrive\Applications\Macromedia Flash 8\en\First Run\External Libraries\FLfile. |