So far, we have focused on putting raw text directly into text fields and then formatting that text with either a TextFormat object or a StyleSheet object. With HTML text, you can put content and formatting in one package using supported HTML tags. Here is a list of the supported HTML tags in Flash: Anchor tag<a> Creates a hyperlink with these attributes: href The URL to be loaded target The window type to be used when opening a link This example creates a link to Macromedia in its own window: <a href="http://www.macromedia.com" target="_blank">click here</a>
Bold tag<b> Creates bold text between the tags: <b>This is what I call bold</b>
Line Break tag<br> This tag creates a new line in HTML text fields. This example creates two lines of text: This is one like<br>this is another line.
Font tag<font> This tag can control some properties of text using these attributes: color This attribute controls the hexadecimal color of text as in this example: <font color="#0000ff">I am blue</font>
face This attribute controls the actual font type of text, and can also handle multiple font names separated by commas. It will choose the first font available on the user's machine. This example uses Arial text as our first choice and Courier as our second: <font face="Arial, Courier">I am Arial</font>
size This attribute controls the size of the text in pixel points. This example sets text to a point size of 20: <font size="20">This is big text</font>
letterSpacing This attribute controls the spacing between letters. This example sets letter spacing to a point size of 2: <font letterSpacing='2'>This is spaced out</font>
Image tag<img> This tag is new to Flash and allows outside images and SWFs to be brought into HTML text fields as well as internal movie clips with their linkage set. It supports a number of tags: src This attribute is the only required attribute and is the URL to the SWF or JPG; it can also be the linkage to an internal movie clip. Both JPGs and SWFs will not display until completely loaded and Flash does not support the loading of progressive JPGs. id This attribute gives the movie clip that holds the external image or SWF (which is automatically created by Flash). It is good if you want to be able to manipulate the embedded image with ActionScript. width Used to set the width of the JPG, SWF, or internal movie clip you load in. height Used to set the height of the JPG, SWF, or internal movie clip you load in. align This attribute controls which side the content you load in to the text field will be on. left and right are the only allowable settings, and the default is left. hspace This attribute controls the horizontal space in pixels around the loaded content between the content and the text. The default is 8. vspace This attribute controls the vertical space in pixels around the loaded content between the content and the text. The default is 8. This example places a JPG on the left, with default spacing as well as an id. <img src="/books/3/419/1/html/2/local.jpg" width="320" height="240" >
For more on embedding images into text fields, look at the section titled "The Image Tag" later in this chapter. Italics tag<i> This tag italicizes text: <i>Here is some italicized text</i>
List Item tag<li> This tag is used to create bulleted lists: Software<li>Flash MX 2004 Pro<li>Dreamweaver<li>Freehand
This example would appear like this: Software Flash MX 2004 Pro Dreamweaver Freehand
Paragraph tag<p> This tag begins a new paragraph and usually holds a large amount of text. It has two attributes: align Controls the horizontal positioning of text between the <p> tags. You can use left, right, center and justify. class This attribute is used with the StyleSheet object to control format. This example creates a new paragraph and sets its alignment to center: <p align="center">New paragraph</p>
Span tag<span> This tag is only useful when setting text to StyleSheets. It used to have a different class of style within a <p> tag. It has one attribute: class Used to name the certain style within the StyleSheet to use for formatting. In this example we use a span tag to change some text in a <p> tag: <p >This is <span >really </span>cool</p>
Text format tag<textformat> Is used to allow a certain set of the TextFormat object's properties to be used with HTML text. It has many attributes: blockindent This attribute is used to control the block indentation in points. indent This attribute controls the indentation from the left side of the text field in points for the first character in the paragraph. leading This attribute controls line spacing. leftmargin This attribute controls the margin on the left in points. rightmargin This attribute controls the margin on the right in points. tabstops This attribute creates custom tabbing points (creates a mock table). An example using this attribute is provided later in this chapter. This example creates some text with 2-point line spacing and a 3-point indentation for paragraphs: <textformat leading="2" indent="3">
Underline tag<u> This tag underlines text and it has no attributes: <u>I am underlined</u>
Now that you have seen all the tags HTML text fields will support, let's start using some. First, if you are creating the text field manually, you must make sure the HTML option in the Properties Inspector is turned on. If you are creating your text fields with ActionScript, make sure you change the html property to TRue like this: myTextField_txt.html = true; Also, we have been using the text property to set text to text fields. If we did that with HTML text fields, it would display the actual HTML tags and the text, so instead we set HTML text to text fields using the htmlText property like this: myTextField_txt.htmlText = "<b>This is a bold statement.</b>"; Okay, now you know some basic rules of HTML text fields. Let's jump right into an example. In this example, we will create a text field and put some HTML into it. 1. | Create a new Flash document.
| 2. | Open the Actions panel in the first frame and place these actions in it:
[View full width] //create the text field this.createTextField("myText_txt",1,0,0,200,200); //set of its properties myText_txt.html = true; myText_txt.wordWrap = true; myText_txt.multiline = true; //create the html string we want to use var myString:String = "<p align='center'><b>Extra Extra</b> <br> Read <u>all</u> about it< /p>"; //now set the text to the text field myText_txt.htmlText = myString;
| Test the movie, and it should appear like Figure 15.10. Nothing too spectacular, but you can of course add as much HTML as you want, and play around to see what else you can do. Figure 15.10. Use HTML to hold content and format at the same time. 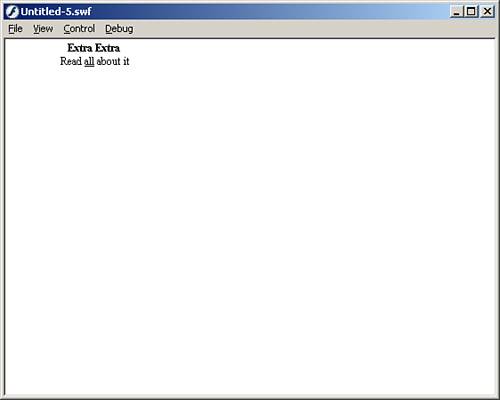
This next example will be a little more advanced. We are going to create a "mock table" with the <textformat> tag to display sets of information. We are going to create three basic field names: name, age, and location. Then we'll add four different people to the table. After that, we will set all of the text to the text field. 1. | Create a new Flash document.
| 2. | Open the Actions panel in the first frame and put these actions in:
//create the text field this.createTextField("myText_txt",1,0,0,250,200); //set its properties myText_txt.html = true; myText_txt.multiline=true; myText_txt.wordWrap = true; This created our text field and set some of the necessary properties.
| 3. | Now we create the string and add all the information we need, so add these actions in:
//now create the string to hold the headers var headers:String = "<u>Name\tAge\tLocation</u>"; //now the string to hold all the rows var rows:String = "Ben\t25\tVirginia<br>"; rows += "Lesley\t24\tBarcelona<br>"; rows += "Missy\t24\tLondon<br>"; rows += "Jen\t27\tNew Hampshire";
| 4. | Finally, create the text format tag and add all the strings to the text field by adding these actions:
//add the textformat tag myText_txt.htmlText = "<textformat tabstops='[70, 120]'>"; //add the headers myText_txt.htmlText += headers; //add the rows myText_txt.htmlText += rows; //close the text format tag myText_txt.htmlText += "</textformat>";
| Now test the movie, and it should appear like Figure 15.11. Figure 15.11. Use the <textformat> tag to create HTML text that appears to have tables. 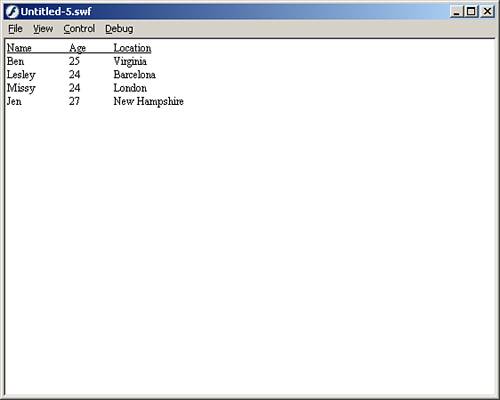
That was a pretty powerful example showing how you could display data inside HTML text fields and make it appear to be organized into tables using the <textformat> tag. Another tag that is very useful is the <img> tag. The Image Tag The image tag, <img>, was introduced in Flash 2004, and it allows the embedding of images as well as SWF files and internal movie clips directly into an HTML text field. This next example will show you how to implement it. In this example, we are simply going to load in a JPG from the outside and put some text around it. You can use any image because we are going to size it in the tag. 1. | Start a new Flash file.
| 2. | Save as imageLoader.fla.
| 3. | We are going to load a JPG image into the text field, so make sure there is one in the same directory where you just saved this file.
| 4. | Open the Actions panel in the first frame and put these actions in:
//create the text field this.createTextField("imageAndText_txt",1,0,0,300,300); //set its properties imageAndText_txt.html = true; imageAndText_txt.wordWrap = true; imageAndText_txt.multiline = true; imageAndText_txt.border = true; This created our text field and set some necessary properties.
| 5. | Now let's create the string to hold the HTML and insert it into the text field with these actions:
[View full width] //create the string to hold the html var myString_str:String = "Picture 1<br><img src='/books/3/419/1/html/2/platform.jpg' width='200' height='200'>This is a picture of platform 9 3/4 in london." //set the text in the text field imageAndText_txt.htmlText = myString_str;
| Now test the movie, and you should see something similar to Figure 15.12. Figure 15.12. You can now load images directly into a text field. 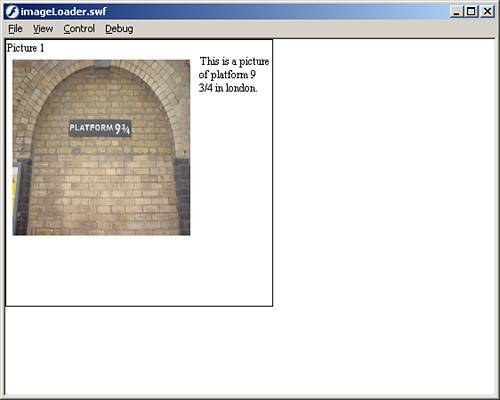
That example showed how to load a JPEG from outside the Flash file, and loading SWFs works the exact same way, but now we are going to show how to load a movie clip symbol from the Flash file into the text field. In this example, we are going to create a rectangle and then load it into the text field we create. 1. | Start a new Flash file.
| 2. | Go to Insert>New Symbol.
| 3. | Make sure it is a movie clip you are creating. Give it a Symbol name of rectangleMC and check the Export for ActionScript check box (also make sure the Linkage Identifier is the same as the Symbol name).
| 4. | When you're in this new movie clip, draw a rectangle with any stroke and fill color, but make sure it is aligned to the left, and to the top of the movie clip (its top left corner is on the cross hair) or it will not sit correctly in the text field. Make it about 150x150, but again, because we will size it with the <img> tag, it is not that important here (although the closer it is to the actual size, the clearer it will appear).
| 5. | Go back to the main timeline, open the Actions panel in the first frame, and put these actions in:
//create the text field this.createTextField("imageAndText_txt",1,0,0,300,300); //set its properties imageAndText_txt.html = true; imageAndText_txt.wordWrap = true; imageAndText_txt.multiline = true; imageAndText_txt.border = true; This will create the text field and set some of its necessary properties.
| 6. | Now that we have our text field, we will create the string and set it to the text field with these actions:
[View full width] //create the string to hold the html var myString:String = "<img src='/books/3/419/1/html/2/rectangleMC' width='150' height='150' align='right'>Now we loaded something from inside of Flash"; //put the text in the text field imageAndText_txt.htmlText = myString;
| Now test the movie to see the rectangle load into the text field. In this example, we embedded the movie clip we created earlier, and set it to the right side of the text box. But let's say we wanted to do something with the rectangle after it has been loaded into the text field. That's where the id attribute of the <img> tag comes in, as you will see as we continue. Building on our preceding example, we are going to make it so that if a user clicks on the image tag, they will open a link. 1. | Go back into the actions, and in the line of code where we create the HTML string, change the image tag to this:
<img src='/books/3/419/1/html/2/rectangleMC' id='rectangle_btn' width='150' height='150' align='right'> Now we are using the id attribute, so we can interact with the rectangle in ActionScript.
| 2. | Now that we have an id we can use in AS, let's create a function that will open a link when the user clicks on the rectangle, by adding these actions to the bottom:
//this will add the event to the rectangle imageAndText_txt.rectangle_btn.onRelease=function(){ getURL("http://www.macromedia.com","_blank"); }
| Now, when you test the movie, you can click on the rectangle, and it will open up a browser window with the link. This example showed how to interact with loaded content in HTML text fields using the id attribute, but if the truth be told, you can also hyperlink these files by using an anchor tag <a> around the image tag <img> like this: <a href="http://www.macromedia.com"> <img src='/books/3/419/1/html/2/image.jpg' ></a> We have covered the image tag, and all that can be done with it. Of course, because you can embed outside SWFs as well as movie clips you create inside, you can do a lot of interesting things, such as having a constantly running clock inside a text field. The possibilities are endless. In the next section, we are going to cover how to interface these HTML text fields with JavaScript, another Web language. HTML Text Fields and JavaScript JavaScript, like ActionScript, is an object-oriented programming language, so if you are not familiar with it, it is easy to pick up if you are comfortable with ActionScript. And JavaScript can do many things that ActionScript cannot because it is a browser language. We are going to show two examples of what can be done with JavaScript and HTML text fields in Flash. The first is a simple alert that will pop up when a link is selected. The second is a bit more powerful, and therefore more complicated; we will use JavaScript to open a custom window to load content in. This first example is fairly simple because we will use the JavaScript inside the ActionScript. 1. | Start a new Flash file.
| 2. | Open the Actions panel in the first frame and place these actions within it:
[View full width] //create the text field this.createTextField("alert_txt",1,0,0,100,20); //set its properties alert_txt.html = true; //now put the text inside the text field alert_txt.htmlText = "<a href='javascript: alert("You made an alert!");'>Alert Me</a>"
| Before you test your movie, you must load it onto a server, or change the allowScriptAccess attribute in the HTML to "always." (You can of course download the correct HTML from the website.) Once one of these two options has been met, when you test your file in a browser, an alert will pop up when you click the text. In this next example, we are actually going to publish the HTML and add some JavaScript to it so we can create custom pop-up windows. But we will build the Flash part first. 1. | Start a new Flash document.
| 2. | Save the document as popup.fla.
| 3. | Open the Actions panel in the first frame, and place these actions in it:
[View full width] //create the text field this.createTextField("popUp_txt",1,0,0,100,20); //set some properties popUp_txt.html = true; //place the html in the text field popUp_txt.htmlText = "<a href=\"javascript: popUp('http://www.sams.com',500,500)\">Open POP-UP</a>";
Now that we have all the script we need for Flash, we are calling a JavaScript function (that we have yet to build) and passing a few parameters to the function.
| 4. | Go to File, Publish (Shift+F12), which will create the .swf file as well as the .html file.
| 5. | Open the .html file we just created in a text editor (like Notepad or SciTe).
| 6. | The first thing you will want to do is change the line of HTML code that says
<param name="allowScriptAccess" value="sameDomain" /> to
<param name="allowScriptAccess" value="always" />
| 7. | Now, between the <head> tags, place this JavaScript:
[View full width] <script language="JAVASCRIPT" type="TEXT/JAVASCRIPT"> <! if(screen){ topPos=0 leftPos=0 } function popUp(thePage,wt,ht){ leftPos= (screen.width-wt)/2 topPos = (screen.height-ht)/2 newWin1 = window.open(thePage,'aWin','toolbars=no, resizeable=no, scrollbars=no ,left='+leftPos+',top='+topPos+',width='+wt+',height='+ht) } // > </script>
| When that text is in the HTML file, you can save the file and close it. Then launch it in a browser and you will see the text we put in the text field. When you click on it, it will open a custom window 500x500 in the center of the screen. You have seen how to create text fields, add text to them, and style them many different ways. You have also seen how to talk directly to JavaScript from them, which allows even more interaction with the end user. Now we are briefly going to go over how to help make your files multi-lingual. |