The first place you will use a custom event is for browsing products by categories. If you remember how the application was left at the end of Lesson 7, there was a tight coupling between the CategoryView component and the e-commerce application. When a user chose a category, this method fired: private function displayProdByCategory():void{ var prodArray:Array=catProds.getProdsForCat(catView.catSelected); prodByCategory=new ArrayCollection(prodArray); } The bolded code shows the main application file reaching into the CategoryView component to pull out data. Again, this tight coupling is undesirable. A far better solution is for the CategoryView to notify its controller (in this, case the EComm application) that a category had been selected. This event will want to carry an instance of the Category class to indicate which one was selected. 1. | Right-click on the flexGrocer project and create a folder named events. Right-click the events folder and create a new ActionScript class. Name the class CategoryEvent, and set its superclass to Event.
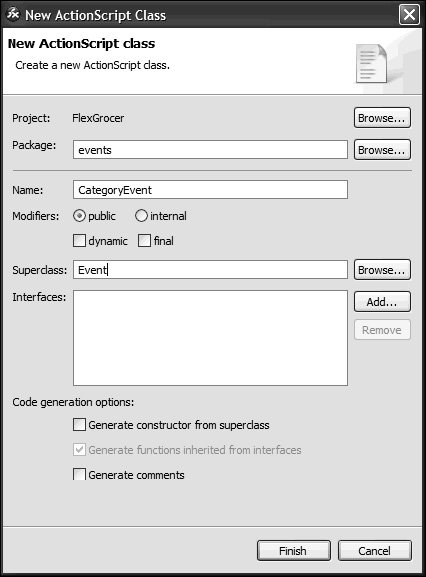 package events{ import flash.events.Event; public class CategoryEvent extends Event { } } Filling out the dialog box automatically creates the skeleton of the class seen here.
| 2. | Inside the class definition, create a public property named cat to hold an instance of the valueObjects.Category class.
public var cat:Category; If you use the Code completion feature, the import for the Category class will be automatically added; if not, you need to manually add the following:
import valueObjects.Category; | | | 3. | Create a constructor, which takes a Category and a string, defining the type as arguments. Pass the type to the superclass and set the cat property with the passed-in Category.
public function CategoryEvent(cat:Category, type:String){ super(type); this.cat = cat; } Like all constructors in ActionScript 3.0, this one is also public. The two arguments will be used to populate the event. The cat property will be used to hold the data about the Category on which the event is acting. The type defines what is happening with the Category in this event. Because the constructor of the Event class is defined as accepting an event type as an argument, you can pass the type directly to the superclass to set it.
| 4. | Override the clone() method so it returns a new instance of the CategoryEvent class.
public override function clone():Event{ return new CategoryEvent(cat, type); } When you override a method in ActionScript 3.0, the method must be defined exactly like the method of the superclass and must include the override keyword. Therefore the clone() method needs to be defined as public override, it must take no arguments, and return an instance of the Event class.
The complete CategoryEvent class should look like the following code block:
package events{ import flash.events.Event; import valueObjects.Category; public class CategoryEvent extends Event { public var cat:Category; public function CategoryEvent(cat:Category, type:String){ super(type); this.cat = cat; } public override function clone():Event{ return new CategoryEvent(cat, type); } } } | 5. | Open CategoryView.mxml from your views/ecomm directory.
Alternatively, you can open it from your Lesson09/start/views/ecomm and save it in your flexGrocer/views/ecomm directory.
| | | 6. | Inside the <mx:Script> block, find the method called categorySelect(), delete its contents, and write a line that builds a CategoryEvent based on the selectedItem from the HorizontalList, cast as a Category. As a second argument, pass the string categorySelect as the event name.
private function categorySelect():void{ var e:CategoryEvent = new CategoryEvent(this.selectedItem as Category, "categorySelect"); this.dispatchEvent(e); } The class definition for the selectedItem of a HorizontalList declares that it is of type Object. You need to tell the compiler that this particular Object is a Category, so that it will accept the value as a valid argument to the CategoryEvent constructor. If you used the code-completion features, imports for both Category and CategoryEvent will automatically be added to your class; otherwise, you will need to manually add imports for both of them.
import valueObjects.Category; import events.CategoryEvent; | 7. | Before the <mx:Script> block, use metadata to declare the new event for the CategoryView component.
<mx:Metadata> [Event(name="categorySelect",type="events.CategoryEvent")] </mx:Metadata> Because the event is an instance of events.CategoryEvent, not directly an instance of flash.events.Event, the type declaration of the metadata is required.
Your CategoryView component is now fully equipped to be loosely coupled as it broadcasts an instance of the CategoryEvent class. All that remains to use it is to have the EComm.mxml application listen for and handle this event.
| 8. | Open EComm.mxml from your flexGrocer directory and ecomm.as from your flexGrocer/as directory.
| | | 9. | Find the instantiation of the CategoryView component. Remove the click handler and replace it with a categorySelect handler, passing the event object to the displayProdByCategory() method.
<v:CategoryView width="600" left="100" cats="{categories}" categorySelect="displayProdByCategory(event)"/> Here you are instructing the EComm application to listen for the categorySelect event from CategoryView. When the event is heard, the resulting event object is passed to the displayProdByCategory() method.
| 10. | Find the displayProdByCategory() method in ecomm.as. Accept an argument containing a CategoryEvent. Remove the reference to catView.catSelected and replace it with the id of the selected Category in the CategoryEvent.
private function displayProdByCategory(event:CategoryEvent):void{ var prodArray:Array=catProds.getProdsForCat(event.cat.catID); prodByCategory=new ArrayCollection(prodArray); } If the import statement for the CategoryEvent class was not automatically imported, then you will need to explicitly import that class.
import events.CategoryEvent; | 11. | Save and run the EComm application. It should run as it did earlier.
| |