A custom User control eventually ends up as a part of a DLL (Dynamic Link Library) file. Components contained in a DLL file, when registered on another user's machine, can be added to a Visual Basic project or displayed on a Web page in Internet Explorer. However, during the development process, you need a way to test and debug your Windows controls within the Visual Basic .NET design environment. This process is slightly more complicated than debugging a normal Windows Application project because you have to deal with two separate sets of running code: the new control itself and the project using the new control. Building the Control Project To make the new control available for use by other projects and applications, you must build (compile) the MySampleControls project, which contains the NameText control. Simply right-click MySampleControls in the Solution Explorer window, then choose Build from the pop-up menu. Testing with a Multi-Project Solution The easiest way to test a new component is to use a multi-project solution. Throughout most of the samples in this book, you will open projects one at a time; that is, when you choose New, Project or Open, Project from Visual Basic's File menu, any projects already loaded close. However, the Visual Basic .NET development environment allows you to have multiple projects loaded simultaneously as part of a solution, which is a container for projects. Tip Each time you create a new project, a solution is created to contain that project, unless you are adding a new project to an existing solution. Notice in the Solution Explorer that a solution named MySampleControls just like the project was created. This solution is contained in a file named MySampleControls.sln. For the current scenario, where we are creating a new control and want to debug it, a typical solution consists of the project in which we are creating the control itself plus a "throw-away" test project (usually a Windows Application project). Adding a Test Project to the Solution To begin testing the NameText control component, you need to add a Windows Application project to the current MySampleControls solution. Just choose File, Add Project, New Project from the menu system, select the Windows Application project template from the Add Project dialog box, and name the project MyControlTest. The Solution Explorer window is updated to show both projects, as shown in Figure 16.5. Figure 16.5. You can add a standard project to your solution to test your new control. 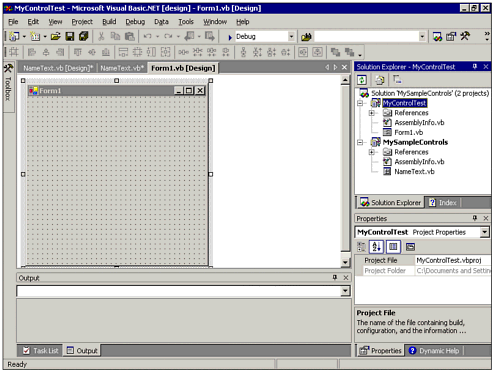 While you're at it, you may want to change the Text property of Form1 to something descriptive, like NameText Control Test Project. Referring to the New Control Project For our test project (MyControlTest) to be able to utilize the new NameText control in the MySampleControls project, MyControlTest needs to contain a reference to MySampleControls. The reference was probably created automatically when you added the test project to the open solution. Check the References folder under the MyControlTest project in the Solution Explorer window to see whether there is a reference to the MySampleControls project. If there is, you don't need to do anything. Otherwise, you'll need to create the reference manually. If you do need to add the reference, you can create it as follows: In the Solution Explorer window, right-click the References folder under the MyControlTest project, then choose Add Reference from the pop-up menu. You will be presented with the Add Reference window. Click the Projects tab, which will give you a list of all the currently open projects that may be referred to. Click the MySampleControls project (which should be the only project listed), then click the Select button to add MySampleControls to the Selected Components box. The Add Reference window should then look like Figure 16.6; click the OK button to complete the reference. Figure 16.6. The Add Reference window allows you to create references from one project to another. 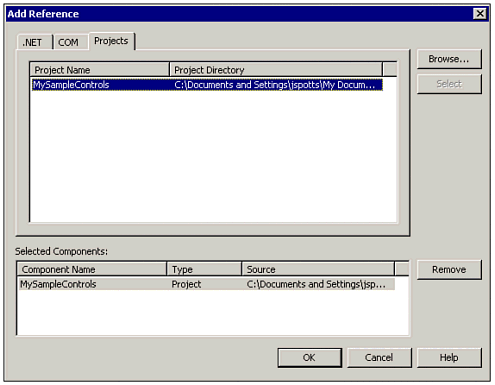 Shutting Down the Designer A user control cannot be used within another project as long as its Designer window is open. If the NameText.vb [Design] tab is visible, click it to bring NameText.vb's designer window to the front, then click the X in the upper right to close it. Setting Up the Test Project If you cannot see the Designer window for our test project's Form1.vb, right-click Form1.vb in the Solution Explorer window, then choose View Designer from the pop-up window. Bring up the Toolbox, then scroll down to the bottom of its Windows Forms section. A Toolbox icon for your NameText user control should now be available, as shown in Figure 16.7. Figure 16.7. The new NameText custom control is now available to use in applications. 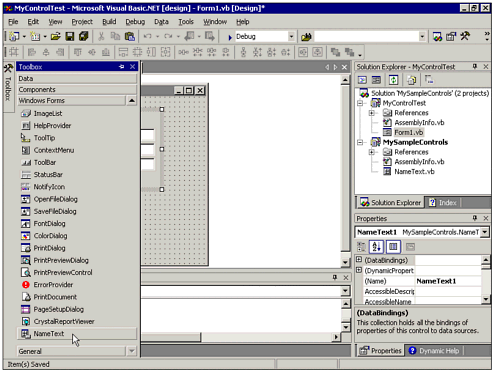 Now your custom Windows control is available. Go ahead and draw an instance on the form, just as you would with any other control. Notice that it appears just as you drew it in its Designer window, but it acts like a single control; all the text boxes within the control move together as you move the control itself; they cannot be selected individually. The control is given a default Name property of NameText1. At this point, your NameText control object is loaded and running, so try resizing it on your form. As you change its size with the mouse, the code in its Resize event handler keeps the text boxes sized appropriately, as shown in Figure 16.8. If you try to reduce the height of the Address control too much, the control springs back to its minimum size. Figure 16.8. As the NameText control is widened, the Resize event code causes the width of the text boxes to increase. 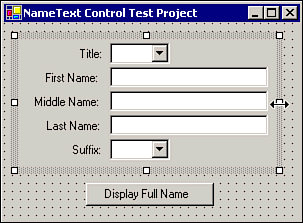 Select the NameText1 control, then check out the Properties window. The custom FullName property that we created is listed in the Properties window; however, because it is a read-only property, it is "grayed out" and cannot be modified. Now, think ahead to when you will be running the test project. How will you know if the NameText control is working properly? We need a way to verify that the NameText control's FullName property is being provided properly. We will do so by adding a Button control to the form; when the Button control is clicked, it will display a message box containing the current value of the NameText1 control's FullName property. Add a Button control to the form. Set its Name property to btnDisplay, and its Text property to Display Full Name. Enter the following line of code into btnDisplay's Click event handler: MessageBox.Show(NameText1.FullName) Notice when you type the dot (.) after NameText1, the FullName property is available from the Auto Display Members feature of the code editor, as shown in Figure 16.9. Figure 16.9. The Auto Display Members feature lists the custom FullName property that was created for the NameText control. 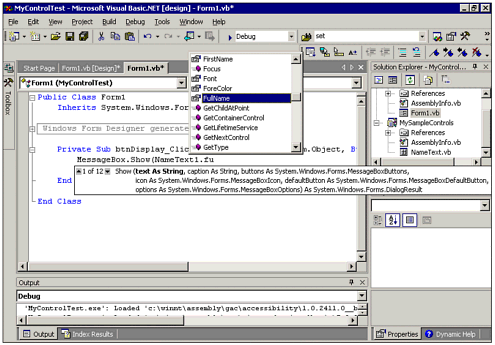 Now when we run the test program and click the button, the current value of the NameText control's FullName property will be displayed in a message box. Setting the Startup Project Now that you have drawn an instance of your control on the form, you will want to run the test project. When you are using a multi-project solution, you must specify which project is the startup project. Visual Basic .NET gives that project the focus, causing it to be executed when the Start command is given, and starting the other projects automatically as needed. By default, the MySampleControls project is set to be the startup project by virtue of having been the first member of the solution. The startup project is indicated by boldface letters in the Solution Explorer window. Because the NameText control is designed to be used within a project other than the Control Library project in which it was created, you need to set the startup project for the current solution to be the test project, MyControlTest. To do so, right-click MyControlTest in the Solution Explorer window, and select Set as Startup Project from the context menu that appears, as shown in Figure 16.10. The MyControlTest project changes to boldface in the Solution Explorer window to indicate that it is the startup project. Figure 16.10. Set the startup project to be the test Windows Application project. The custom control project starts automatically. 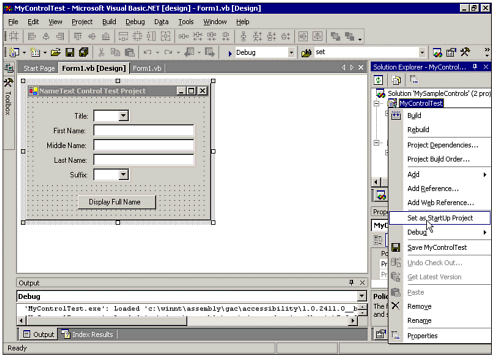 Running the Test Application Now that you have set up the test program, you are ready to run it to ensure that the NameText control works correctly. because you set MyControlTest to be the startup project in the previous section, pressing F5 or clicking the Start button causes MyControlTest to run. To start the test project, press F5; choose Debug, Start from the menu system; or click the Start button on the Toolbar. The form will load, and the topmost combo box in the NameText control will get the focus. Fill out some or all the name component fields. When you click the Display Full Name button, a message box should appear to report the current value of the FullName property. Your custom NameText control is working properly! Figure 16.11 shows an example of the completed NameText control generating the FullName property. Figure 16.11. The completed NameText control assembles a full name from component fields. 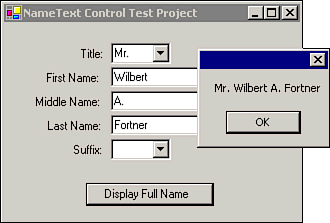 If you notice any problems; say, if you made a constituent control too small or left out a space in the concatenation of the name fields, simply stop the running program, open the Designer or Code window for the NameText control, and make any necessary changes. Run the test program again (which will cause the control to be rebuilt) to verify that your changes fixed the problems. If you want to learn more about debugging a program, see Chapter 26, "Debugging and Performance Tuning." You can use the methods described there to step through the code in the FullName property procedure, examining the contents of the variables to verify that they are correct. For more information on dealing with unexpected errors in your programs, p. 718 |