For a successful rocket launch to occur, a number of conditions must be met. One of the most important variables affecting a rocket launch is weather, which helps determine not only how the rocket is propelled but how it's controlled. In the following exercise, we'll begin scripting a project so that the rocket will react differently based on randomly generated weather conditions. Open rocketLaunch1.fla in the Lesson08/Assets folder. This file contains a single scene made up of eight layers, named according to their contents. Let's look at the various elements in the scene. The Weather layer contains a movie clip instance the size of the movie itself. This movie clip, named weather_mc, represents the background sky in the scene. Its timeline contains three frame labels: Sunny, Rainy, and Night. At each of these frame labels, the sky graphic changes to match the name of the label. At Sunny, the sky appears sunny; at Rainy, it appears overcast; at Night, it looks dark. The Ground/Central Graphics layer contains the mountains, ground, and main Central Command graphics. There is also a small movie clip instance of a red dot, which blinks to indicate the launch location on the globe. The Rocket layer obviously contains our rocket that is, a movie clip instance named rocket_mc. Its timeline contains two frame labels: off and on. At the off label, the rocket doesn't show a flame; at the on label, it does, providing a visual indicator of the rocket in motion. The Control Panel layer contains several elements, the most conspicuous of which is the red Launch button named launch_btn. This button will be used not only to launch the rocket but also to reset the scene after a launch has been attempted. Below this button is a movie clip instance named thrustBoost_mc that includes the text "Thrusters." This clip also contains two frame labels: on and off. At the on label, the text appears big and red to provide a visual indication that thrust is being applied to the rocket. This layer's last element is a text field in the lower-right portion of the stage with an instance name of weatherText_txt. This text field provides a textual indication of current weather conditions. The Status layer contains a movie clip instance named status_mc that appears as a small circle in the middle of the sky. It looks this way because the first frame of its timeline contains no graphical content. This clip provides a text message about the launch's success or failure. There are three frame labels on this movie clip's timeline: off, abort, and success. At the off frame label, the status displays no text. At the abort label, a text message appears: "Launch Aborted." At the success label, a different text message appears: "Launch Successful." The Launch Window layer contains a movie clip instance named launchWindow_mc, showing two red bars. The space between the red bars represents the launch window the area the rocket must pass through to have a successful launch. The Sound Clips layer contains a movie clip instance named sounds_mc that also appears as a small circle just above the stage, on the left side. Its timeline contains frame labels named intro, launch, abort, and success. At each of these labels is an appropriate sound clip (the launch label has a launch sound, the abort label has an abort sound, and so on). Various scripts will send this clip to these frame labels to provide audio effects for the project. The Actions layer will contain all the scripts for this project. Let's begin.
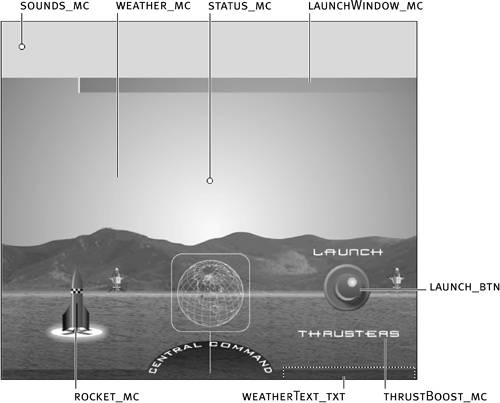
With the Actions panel open, select Frame 1 of the Actions layer and add the following script: var noThrust:Number; var thrust:Number; function setWeatherConditions(){ var randomWeather:Number = random (3); var conditions:String; if (randomWeather == 0) { conditions = "Sunny"; noThrust = 3; thrust = 6; } else if (randomWeather == 1) { conditions = "Rainy"; noThrust = 2; thrust = 4; } else { conditions = "Night"; noThrust = 1; thrust = 2; } } The first two lines of this script declare two variables: noThrust and thrust. (We'll elaborate on the purposes of these two variables in a moment.) This function is used to make the project react to random weather conditions. The function's first action sets the value of the randomWeather variable, based on a randomly generated number (from three possibilities: 0, 1, or 2). The next line declares the conditions variable, which will be used later in the function. NOTE You may wonder why the first two variables (noThrust and thrust) are declared outside the function definition, yet randomWeather and conditions are declared within the function definition. The randomWeather and condition variables will be used only within this function definition, so they need to exist only as local variables of the function. The noThrust and thrust variables will be used later, by other parts of the script. Because noThrust and thrust must exist as timeline variables, they're created outside the function definition, and the function is used to set their values. For more information on functions, see Lesson 5, "Using Functions." Next, an if statement within the function definition analyzes the value of randomWeather so that the values of three other variables can be set. If randomWeather has a value of 0, the variable named conditions is created on this timeline and assigned a string value of "Sunny". In addition, the values of noThrust and thrust are set to 3 and 6, respectively. If the value of randomWeather isn't 0, that part of the statement is ignored, at which point an else if statement checks whether randomWeather has a value of 1. If so, the variables are instead assigned values of "Rainy", 2, and 4, respectively. If the value of randomWeather isn't 1, that part of the statement is ignored, at which point the actions following the else statement are executed. Remember that an else statement enables you to define what happens if none of the previous conditions in the statement has proven true but you still want something to happen. In this case, if the previous conditions proved false, the actions under the else statement are executed, setting the value of the variables we've been discussing to "Night", 1, and 2, respectively. The value of the conditions variable will be used in a moment to set how the weather will appear graphically in the scene. The other two variables will soon be used to establish how fast the rocket_mc instance will move based on the weather condition generated.
Add this script after the end of the if statement (but within the function definition): weather_mc.gotoAndStop (conditions); weatherText_txt.text = "Weather: " + conditions; These two actions occur as soon as the if statement is analyzed, and both use the value of conditions to react in unique ways. The first action moves the weather_mc movie clip instance to a frame label based on the value of conditions. If conditions has a value of "Rainy", the weather_mc movie clip instance moves to that frame label, where the weather appears rainy. The next action determines what will be displayed in the weatherText_txt text field in the lower-right portion of the stage. If conditions has a value of "Rainy", this text field displays Weather: Rainy.
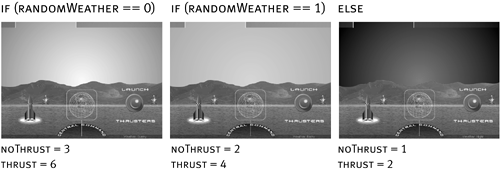
Add this script at the end of the function definition: setWeatherConditions(); This script calls the setWeatherConditions() function as soon as the movie begins to play.
Choose Control > Test Movie to test the functionality of the project at this point. As soon as the movie plays, the script we just added in the preceding steps is executed. The graphical representation of the weather, as well as the text displayed in the lower-right portion of the screen, are affected by the random number generated in the script.
NOTE Repeated testing may give you different results each time. While still in the testing environment, choose Debug > List Variables. This step opens the Output window, allowing you to see the values of variables on different timelines. A quick glance reveals the values of the thrust and noThrust variables in the main timeline. We'll use these values shortly.
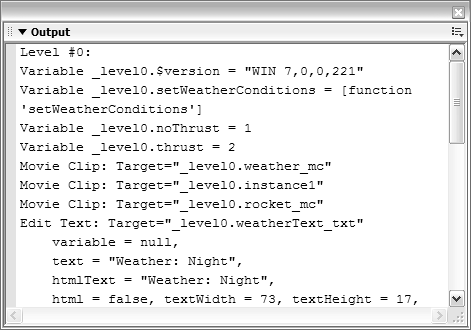
Close the test environment to return to the authoring environment, and save your work as rocketLaunch2.fla. We'll build on this file in the next exercise.
|