The CarPartRequest Web Service The CarPartRequest Web service is a simple Web service that exposes a method called getCarPartRequest that reports the status of a specified engine type. This method takes a string specifying the name of the engine type as its only parameter, and returns a string describing the status of the engine type. A remote client of the CarPartRequest Web service will connect to the Web service and do an RPC on the getCarPartRequest method. To create the Web service and its remote client, the following files need to be created: -
The service definition interface file, called CarPartRequestIF.java. -
The service definition implementation file, called CarPartRequestImpl.java. -
The config.xml file. This is the configuration file read by the xrpcc tool to generate the stubs and ties. -
The web.xml file. This is the deployment descriptor for the Web service. -
The CarPartRequestClient.java file. This is the remote client that connects to the Web service and does a RPC on the getPartRequest method. Creating the Service Definition Interface A service definition interface is a standard J2SE interface definition file that declares the methods that a remote client can execute. A definition file has to abide by the following rules: -
It must extend the java.rmi.Remote interface. -
There should be no constant declarations. -
All the methods must necessarily throw the java.rmi.RemoteException or one of its subclasses. Additionally, the methods can also throw service-specific exceptions. -
The parameters and return types must be the data types supported by the JAX-RPC specifications. With the rules defined, let's now create the CarPartRequestIF service definition interface: -
Create an empty file called CarPartRequestIF.java. -
You want to put the Web service classes in a package called CarPartReq, so specify the package definition. -
Import the Remote and RemoteException classes. -
Specify the declaration for getCarPartRequest method. Open the text editor of your choice and create an empty file called CarPartRequestIF.java. Enter the following lines of code: package CarPartReq; import java.rmi.Remote; import java.rmi.RemoteException; public interface CarPartRequestIF extends Remote { public String getCarPartRequest(String s) throws RemoteException; } Next you need to define the implementation class for the service definition interface. Creating the Service Definition Interface Implementation Class The implementation class will basically define the getCarPartRequest method. The method takes the name of the engine as its only parameter. If the engine name is Engine 1, the getCarPartRequest method should report that Engine 1 is out of stock. For all other engine types, it should say that the engine has been shipped. To create the implementation class, select the text editor of your choice and create a file called CarPartRequestImpl.java. In that file, enter the following lines of code: package CarPartReq; public class CarPartRequestImpl implements CarPartRequestIF { public String getCarPartRequest(String s) { if (s.equals("Engine 1")) { return new String("Engine 1 is currently out of stock"); } else { return new String("Your requested engine is in stock. It will be shipped shortly"); } } } Now you need to create the config.xml file. Creating the config.xml File As mentioned earlier, the config.xml file is the configuration file that the xrpcc tool reads to create the stubs, ties, and the WSDL document for the Web service. To create the config.xml file, select a text editor and create a file called config.xml. In that file, enter the following lines of code: <?xml version="1.0" encoding="UTF-8"?> <configuration xmlns="http://java.sun.com/xml/ns/jax-rpc/ri/config"> <service name="CarPartRequest" packageName="CarPartReq" targetNamespace="http://carpartsheaven.org/wsdl" typeNamespace="http://carpartsheaven.org/types"> <interface name="CarPartReq.CarPartRequestIF" servantName="CarPartReq.CarPartRequestImpl"> </interface> </service> </configuration> Let's take a closer look at this file. The name parameter of the service element is CarPartRequest. The xrpcc tool will use this name as the prefix to the implementation class file that is generated when you run the xrpcc tool for creating stubs and ties. This implementation class file is different from the one that you created earlier. The value of the packageName parameter is the name of the package of the classes generated by xrpcc. This should match the package name that you specified in the service definition interface and its corresponding implementation class. The value in the name parameter of the interface element is the fully qualified name of the service definition interface, which is CarPartReq.CarPartRequestIF in this case. The value of the servantName parameter has to be the fully qualified name of the implementation class of the service definition interface. Now let's generate the stubs, ties, and the WSDL document: -
Generate the class file for the Web service definition (compile the CarPartRequestIF.java file). -
Generate the class file for the Web service definition implementation (compile the CarPartRequestImpl.java file). -
Run the xrpcc tool to generate the stubs, ties, and the WSDL document. To generate the class file for the Web service definition interface, enter the following line of code at the command prompt: javac -d . CarPartRequestIF.java To generate the class file for the service definition interface implementation, enter the following line of code at the command prompt: javac -classpath . CarPartRequestImpl.java Next you need to run the xrpcc tool. This will generate the stubs, ties, and the WSDL file for the Web service. To run the xrpcc tool, enter the following command at the command prompt: xrpcc -both -classpath . -d . config.xml The -both option creates the stubs, ties, and the WSDL document describing the Web service. The following files will be created under the CarPartReq folder: -
CarPartRequest.class -
CarPartRequestIF.class -
CarPartRequestIF_Stub.class -
CarPartRequestIF_Tie.class -
CarPartRequestImpl.class -
CarPartRequest_Impl.class -
CarPartRequest_SerializerRegistry.class -
CarPartRequestIF_GetCarPartRequest_RequestStruct.class -
CarPartRequestIF_GetCarPartRequest_RequestStruct_SOAPSerializer.class -
CarPartRequestIF_GetCarPartRequest_ResponseStruct.class -
CarPartRequestIF_GetCarPartRequest_ResponseStruct_SOAPSerializer.class Additionally, it will also create the CarPartRequest_Config.properties file in the location where you ran the xrpcc tool. As you will see shortly, the CarPartRequest_Config.properties file is read by the deployment descriptor (web.xml) file while loading the Web service. Next you will create the web.xml file for the Web service. Creating the web.xml File The web.xml file is an XML file that provides configuration information about a Web application to the Web server. Because the Web service you are creating is installed as a servlet, the deployment descriptor will contain information pertaining to the Web service. To create the deployment descriptor, create an empty file called web.xml. In the web.xml file, enter the following lines of code: <?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.2//EN" "http://java.sun.com/j2ee/dtds/web-app_2_2.dtd"> <web-app> <display-name>CarPartRequest</display-name> <description>Car Parts Order System</description> <servlet> <servlet-name>CarPartRequest</servlet-name> <display-name>CarPartRequest</display-name> <description>Servlet to handle Car Part Request</description> <servlet-class>com.sun.xml.rpc.server.http.JAXRPCServlet</servlet-class> <init-param> <param-name>configuration.file</param-name> <param-value>/WEB-INF/CarPartRequest_Config.properties</param-value> </init-param> <load-on-startup>0</load-on-startup> </servlet> <servlet-mapping> <servlet-name>CarPartRequest</servlet-name> <url-pattern>/jaxrpc/*</url-pattern> </servlet-mapping> <session-config> <session-timeout>60</session-timeout> </session-config> </web-app> Let's look at the parts of the web.xml file that pertain to the CarPartRequest Web service. The value of the servlet-class element refers to the servlet that can support the JAX-RPC-based Web service. For this example, you'll use the servlet that's shipped with the reference implementation. The value of the init-param element is the CarPartRequest_Config.properties file that was generated by the xrpcc tool. Next the url-pattern element of the web.xml file determines the URL of the service's endpoint. Finally, you put a timeout of 60 seconds if the Web service fails to respond within that time. Deploying the Web Service Next you need to install the Web service on the Web server. To do so, create a folder called CarPartRequestApp. In the CarPartRequestApp folder, create a folder called WEB-INF. Inside the WEB-INF folder, copy over the web.xml and the CarPartRequest_Config.properties files and create a folder called classes. In the classes folder, copy over the CarPartReq folder that was created when you compiled the Web service interface and definition files and ran the xrpcc tool. Your directory structure should now look like the one shown in Figure 11.3. 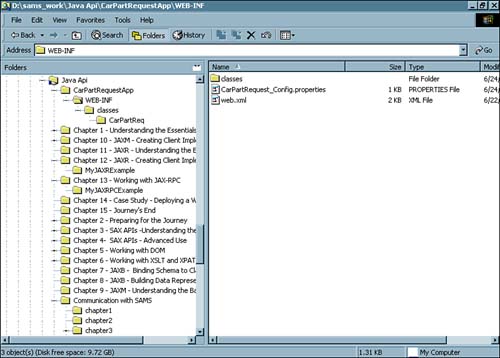 After the files are copied over, you will create a Web application archive WAR file and deploy it with Tomcat. To create the WAR file, go to the CarPartRequestApp folder and enter the following command at the command prompt: jar cvf CarPartRequest.war * This will create the Tomcat-deployable Web application archive for you. To run the Web service, copy the CarPartRequest.war file into the webapps folder. Now start up Tomcat. Next launch a Web browser and go to http://localhost:8080/CarPartRequest/jaxrpc to verify the installation. The browser should show the contents as displayed in Figure 11.4. 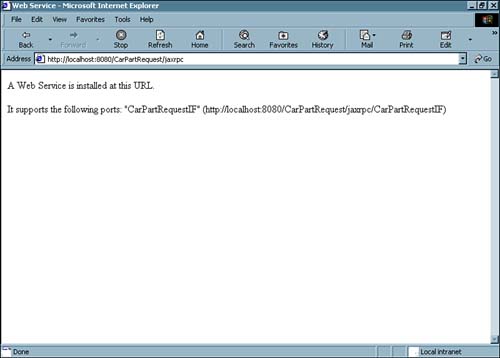 Let's summarize what has been done so far. You created the Web service endpoint interface and its implementation class. Then you created the configuration file for the xrpcc tool. After that, you compiled the service endpoint interface and its implementation class, and ran the xrpcc tool to generate the stubs, ties, and WSDL document. Finally, you created the Web application archive for the CarPartRequest Web service and deployed it in the Tomcat Web server. Now you will create the client application for the CarPartRequest Web service. |