The XML Style Sheet Language Transformation (XSLT) API is used for transforming an XML document into any other form. The most common use of XSLT for Web development is transforming XML documents into HTML for presentation in a Web browser. The JAXP defines an XSLT Transformer that reads an XML document and applies the rules specified in a style sheet to it to produce a resultant document. Figure 25.3 shows this graphically. Figure 25.3. XSLT process. 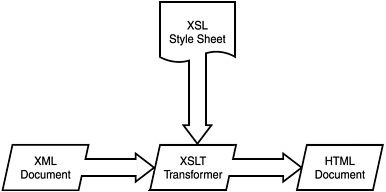 The JAXP provides XSLT support through the javax.xml.transform package. The javax.xml.Transformer class performs the actual transformation and similar to obtaining a SAX parser or a DOM document builder, a Transformer is obtained through a factory, or more specifically a javax.xml.TransformerFactory. An instance of the TransformerFactory class can be obtained by calling the TransformerFactory's newInstance() method: TransformerFactory factory = TransformerFactory.newInstance(); From the TransformerFactory, a Transformer can be obtained by calling one of its newTransformer() methods, see Table 25.11. Table 25.11. TransformerFactory newTransformer() Methods Method | Description |
---|
Transformer newTransformer() | Create a new Transformer object that performs a copy of the source to the result. | Transformer newTransformer(javax.xml.transform.Source source) | Process the Source into a Transformer object. | The latter of the newTransformer() methods is used when transforming an XML document into another form. It accepts a stylesheet in the form of a class implementing the javax.xml.transform.Source interface; three classes are provided as sources in the JAXP: javax.xml.transform.SAXSource javax.xml.transform.DOMSource javax.xml.transform.StreamSource Together these source objects allow a Transformer to be built with an XML stylesheet from an existing DOM document, a SAX parser, or a stream, which includes a file, a java.io.InputStream, or a java.io.Reader. The Transformer is then used by calling its transform() method to transform a source document to a result document: void transform( Source xmlSource, Result outputTarget ) The XML source document is another instance of a class implementing the javax.xml.transform.Source interface, which includes SAX, DOM, or Stream inputs) and the output target is an instance of a class implementing the javax.xml.transform.Result interface. Similar to the Source interface, the Result interface has three implementations: javax.xml.transform.SAXResult javax.xml.transform.DOMResult javax.xml.transform.StreamResult The resultant transformation can be in the form of SAX events, a DOM document, or any java.io.OutputStream, java.io.File, or java.io.Writer variation. To summarize the steps in transforming an XML document to another form using XSLT: -
Get an instance of the TransformerFactory by calling its static newInstance() method. -
Create a javax.xml.transform.Source reference to the stylesheet used in the transformation by building a SAXSource, DOMSource, or StreamSource. -
Get a Transformer from the TransformerFactory using the XSL source by calling the newTransformer() method. -
Create a javax.xml.transform.Source reference to the source XML document. -
Create a javax.xml.transform.Result reference to the output target. -
Call the Transformer's transform() method. XSL Stylesheets The Java code to apply a stylesheet to an XML document to produce an output document is trivial; the real work is in defining the stylesheet. Exhaustive references to XSL and the corresponding XPath specification can be found both in print (see Special Edition Using XSLT, by Que Publishing) and on the World Wide Web at http://www.w3c.org. At its core, XSL files contain processing instructions that are organized by XPath expressions; the XSLT processor traverses the source XML document looking for matching XPath expressions defined in the stylesheet. An XSL stylesheet always starts by defining the XSL namespace and version: <xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"> This header defines the XSL stylesheet language and fully qualifies the xsl prefix that will be used later in the document with the qualifying URL: http://www.w3.org/1999/XSL/Transform From this point forward, XSL-specific tags will be represented with the XSL prefix: <xsl:command>. The most common XSL command is the match command; this is used to match patterns in the XML file. For example, the following statement matches the root of the XML file: <xsl:template match="/"> ... </xsl:template> The statements enclosed between the <xsl:template> start and end tags will be written to the result document. Another common tag is the <xsl:apply-templates> tag, which tells the XSLT transformer to execute the other template match expressions and place their output at the location of the <xsl:apply-templates> tag. For example: <xsl:template match="/"> <HTML> <BODY> <table> <xsl:apply-templates/> </table> </BODY> </HTML> </xsl:template> <xsl:template match="books/book"> <tr><td>...</td></tr> </xsl:template> This example creates a new HTML document that contains a table when it sees the root of the XML document. The contents of the table are handled by other patterns, in this case the pattern books/book, or in XML terms this looks for all <book> tags that appear inside of a <books> tag. The books/book handler creates table rows and table cells that presumably describe a book. For more information on XPath, XSL, and XSLT fundamentals, please refer to the World Wide Web Consortium Web Site at http://www.w3c.org. An Example Using XSLT This example transforms the book.xml file that we've been working with throughout this chapter and generates an HTML representation of it. The HTML is purposely simple so as not to confuse the technical issues. Listing 25.9 shows the contents of the XSL file that contains the instructions for the transformation, and Listing 25.10 shows the Java code that performs the transformation. Listing 25.9 book.xsl 001:<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"> 002: 003:<xsl:template match="/"> 004: <HTML> 005: <HEAD> 006: <TITLE>My Books</TITLE> 007: </HEAD> 008: <BODY> 009: <TABLE> 010: <TR> 011: <TH>Category</TH> 012: <TH>Title</TH> 013: <TH>Author</TH> 014: <TH>Price</TH> 015: </TR> 016: <xsl:apply-templates select="books/book"> 017: <xsl:sort select="@category"/> 018: </xsl:apply-templates> 019: </TABLE> 020: </BODY> 021: </HTML> 022:</xsl:template> 023: 024:<xsl:template match="books/book"> 025: <TR> 026: <TD><xsl:value-of select="@category" /></TD> 027: <TD><xsl:value-of select="./title" /></TD> 028: <TD><xsl:value-of select="./author" /></TD> 029: <TD><xsl:value-of select="./price" /></TD> 030: </TR> 031:</xsl:template> 032: 033:</xsl:stylesheet> Line 1 defines the version of XSL stylesheet that this stylesheet is using and it defines the xsl namespace. Lines 3 22 handle the root element: It creates an HTML document with a table, a table header, and then it delegates the contents of the table to the books/book element. The select clause in the <xsl:apply-template> tag tells the XSLT transformer what patterns to match; if it is omitted, it matches all patterns. Line 17 tells the transformer to sort the results by the <book> element's category attribute (the at sign @ denotes category). Lines 24 31 handle the <book> nodes by creating a table record and four table rows. It obtains the value of the category attribute by using the <xsl:value-of> tag and passing its select clause @category (again the @ means attribute). Similarly, it uses the <xsl:value-of> tag passing its select clause of the child nodes to get the title, author, and price. Listing 25.10 XSLTTest.java 001:import javax.xml.transform.*; 002:import javax.xml.transform.stream.*; 003:import java.io.File; 004: 005:public class XSLTTest 006:{ 007: public static void main( String[] args ) 008: { 009: try 010: { 011: StreamSource source = new StreamSource( new File( "book.xml" ) ); 012: StreamResult result = new StreamResult( System.out ); 013: TransformerFactory factory = TransformerFactory.newInstance(); 014: Transformer transformer = factory.newTransformer( new StreamSource( new File( "books.xsl" ) ) ); 015: transformer.transform( source, result ); 016: } 017: catch( Exception e ) 018: { 019: e.printStackTrace(); 020: } 021: } 022:} Line 11 creates a StreamSource to the file book.xml and line 12 creates a StreamResult to the standard output; this application will transform the book.xml file to the screen. Line 13 obtains a new instance of the TransformerFactory and Line 14 uses it to create a new Transformer for the books.xsl file (as a StreamSource). Finally, line 15 transforms the source document to the result (screen). The output should look something like the following: <HTML> <HEAD> <META http-equiv="Content-Type" content="text/html; charset=UTF-8"> <TITLE>My Books</TITLE> </HEAD> <BODY> <TABLE> <TR> <TH>Category</TH><TH>Title</TH><TH>Author</TH><TH>Price</TH> </TR> <TR> <TD>Computer Programming</TD><TD>Java 2 From Scratch</TD><TD>Steven Haines</TD><TD>39.95</TD> </TR> <TR> <TD>fiction</TD><TD>Left Behind</TD><TD>Tim Lahaye</TD><TD>14.95</TD> </TR> </TABLE> </BODY> </HTML> |