Servlets that don't accept input from the client are of very little practical value outside of testing. Real-world servlets often receive data and additional instructions from clients in the form of parameters. The most common way for these parameters to be entered by the user is by using HTML forms. Numerous HTML books are on the market, so I won't try to replace them here. You will, however, learn just enough HTML to be able to do data entry so that you can pass it to your servlets. Listing 21.9 shows an HTML page that enables the user to enter data bound for a servlet. Listing 21.9 The DataEntry.html File <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <HTML> <HEAD> <TITLE>Passing Parameters to Java Servlets</TITLE> </HEAD> <BODY BGCOLOR="#FDF5E6"> <H1 align="CENTER"> Enter Values for all three Parameters </H1> <FORM action='/examples/servlet/PassParam'> Name: <INPUT TYPE="TEXT" NAME="UserName"><BR><BR> Age: <INPUT TYPE="TEXT" NAME="UserAge"><BR><BR> Favorite Sport: <INPUT TYPE="TEXT" NAME="UserSport"><BR><BR> <CENTER> <INPUT TYPE="SUBMIT"> </CENTER> </FORM> </BODY> </HTML> The most interesting part of this HTML file is the <FORM> tag. The design of this feature predates Java servlets by several years. In the formative years of Web development, forms were heavily used with CGI and Perl on the server. The basic flow is fairly intuitive. The FORM tag contains an action value that tells which script the Web server is to run when the SUBMIT button is clicked. In this case, the action is to run a servlet stored in the examples application, called PassParam. On Tomcat, this means that this servlet will be stored in a classes directory immediately under the WEB-INF directory. Other Web servers use other naming conventions. <FORM action='/examples/servlet/PassParam'> This form tag contains three data entry boxes, along with some text that tells you what belongs in each box. Name: <INPUT TYPE="TEXT" NAME="UserName"><BR><BR> Age: <INPUT TYPE="TEXT" NAME="UserAge"><BR><BR> Favorite Sport: <INPUT TYPE="TEXT" NAME="UserSport"><BR><BR> Following these, a SUBMIT button is defined. When the user clicks on this button, the action is to be triggered. The browser processes this form and creates the HTTP containing the request. <CENTER> <INPUT TYPE="SUBMIT"> </CENTER> To execute this example, store this file in the webapps\examples\ directory and type the following line in the address box of a browser: http://localhost:1776/examples/DataEntry.html The browser will look like Figure 21.9. Figure 21.9. . The Form tag causes data entry fields to be created on a Web page. 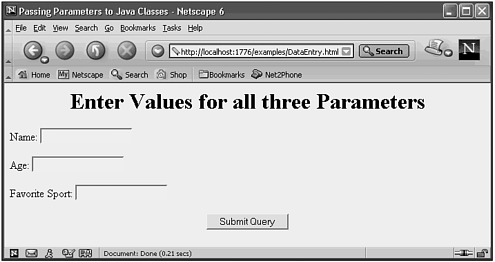 When you enter the data into the form and click the SUBMIT button, the following line will appear in the address line of the browser: http://localhost:1776/examples/servlet/PassParam? [ic:ccc]UserName=Joe&UserAge=15&UserSport=Soccer Notice that the parameters are passed right on the command line, providing proof that a GET HTTP command has been issued. We could have written this HTML page with the following FORM tag: <FORM action='/examples/servlet/PassParam' method='GET'> The result would have been the same, however, because GET is the default form-handling method. The syntax of the method says that we want to call a servlet in the examples application called PassParam. As mentioned earlier in this section, the Web server will look for the file ...\webapps\examples\WEB_INF\classes\PassParam.class. Listing 21.10 shows the .java file that was used to create the PassParam.class file. Listing 21.10 The PassParam.java Class /* * PassParam.java * * Created on June 21, 2002, 5:25 PM */ import javax.servlet.*; import javax.servlet.http.*; /** * * @author Stephen Potts * @version */ public class PassParam extends HttpServlet { /** Initializes the servlet. */ public void init(ServletConfig config) throws ServletException { super.init(config); } /** Destroys the servlet. */ public void destroy() { } /** Processes requests for both HTTP <code>GET</code> * and <code>POST</code> methods. * @param request servlet request * @param response servlet response */ protected void processRequest(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { response.setContentType("text/html"); java.io.PrintWriter out = response.getWriter(); // output your page here out.println("<html>"); out.println("<head>"); out.println("<title>" + "Parameter Passing" + "</title>"); out.println("</head>"); out.println("<body BGCOLOR=\"#FDF5E6\"\n>"); out.println("<h1 ALIGN=CENTER>"); out.println("Here are the Parameters"); out.println("</h1>"); out.println("<B>The UserName is </B>"); out.println(request.getParameter("UserName") + "<BR>"); out.println(" "); out.println("<B>The UserAge is </B>"); out.println(request.getParameter("UserAge") + "<BR>"); out.println(" "); out.println("<B>The UserSport is </B>"); out.println(request.getParameter("UserSport") + "<BR>"); out.println(" "); out.println("</body>"); out.println("</html>"); out.close(); } /** Handles the HTTP <code>GET</code> method. * @param request servlet request * @param response servlet response */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { processRequest(request, response); } /** Handles the HTTP <code>POST</code> method. * @param request servlet request * @param response servlet response */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { processRequest(request, response); } /** Returns a short description of the servlet. */ public String getServletInfo() { return "Short description"; } } This servlet looks like the simple servlets already described in this chapter, but it has some new features. As usual, the real work is done in the processRequest() method, which is called by both the doGet() and doPost() methods. This method is passed to the HttpServletRequest and HttpServletResponse objects. protected void processRequest(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException The response object is how we will communicate back to the client's browser. The Web server will magically communicate anything printed to this object back to the client's browser. response.setContentType("text/html"); java.io.PrintWriter out = response.getWriter(); Much of the output consists of simple static HTML statements, but there are several dynamic statements. The request object contains quite a bit of information that has been passed to the servlet from the browser. The most important parts of this information are the values of the parameters that were passed in from the HTML. out.println("<B>The UserName is </B>"); out.println(request.getParameter("UserName") + "<BR>"); out.println(" "); Notice how the request object retrieves these values by the exact string names that were specified in the HTML. The HTML line for the age was Age: <INPUT TYPE="TEXT" NAME="UserAge"><BR><BR> The parameter that the getParameter() passed is the same name, "UserAge". The value that it returns will be a String containing exactly what the user entered into the browser. out.println("<B>The UserAge is </B>"); out.println(request.getParameter("UserAge") + "<BR>"); out.println(" "); out.println("<B>The UserSport is </B>"); out.println(request.getParameter("UserSport") + "<BR>"); The result of running the DataEntry.HTML page and clicking SUBMIT is shown in Figure 21.10. Figure 21.10. The HttpServletRequest contains information that is being passed from the browser to the servlet. 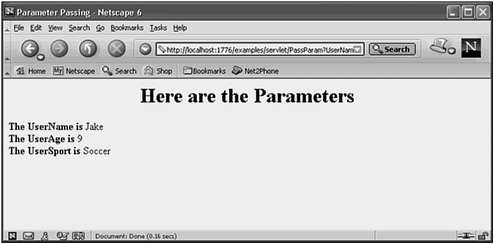 The DataEntry.HTML page used the default HTTP GET command to communicate with the server. We can change that very easily to use the POST command instead. All that we have to do is modify the HTML as shown in Listing 21.11. Listing 21.11 The DataEntryPost.HTML File <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <HTML> <HEAD> <TITLE>Posting Parameters to Java Servlets</TITLE> </HEAD> <BODY BGCOLOR="#FDF5E6"> <H1 align="CENTER"> Enter Values for all three Parameters </H1> <FORM action='/examples/servlet/PassParam' method='post'> Name: <INPUT TYPE="TEXT" NAME="UserName"><BR><BR> Age: <INPUT TYPE="TEXT" NAME="UserAge"><BR><BR> Favorite Sport: <INPUT TYPE="TEXT" NAME="UserSport"><BR><BR> <CENTER> <INPUT TYPE="SUBMIT"> </CENTER> </FORM> </BODY> </HTML> Notice that only one line has changed: <FORM action='/examples/servlet/PassParam' method='post'> The post method is telling the browser to generate a different set of HTTP commands to send to the server. This new version passes the parameters in the content of the HTTP communication, not in the address as we saw earlier. The URL being sent to the server now looks like the following: http://localhost:1776/examples/servlet/PassParam Notice that no changes were made to the servlet. The reason for this is that the Web server prepares the same request object regardless of which method is specified. A second reason is that we wrote the servlet with the same processing for both the doGet() and the doPost() methods. Be aware that the GET method will only allow 255 bytes to be passed. Anything longer will be truncated, therefore longer parameters must use the POST method. |