Armed with an understanding of the basics, we are ready to create a simple JavaBean. The program that we will create is going to be a progress bar, which grows and shrinks to indicate progress and regress. The code for this class is shown here in Listing 15.1: Listing 15.1 The ProgressBar.java /* * ProgressBar.java * * Created on August 27, 2002, 11:18 AM */ package com.samspublishing.jpp.ch15; import java.awt.*; import java.awt.event.*; import java.beans.*; import java.io.Serializable; /** * * @author Stephen Potts */ public class ProgressBar extends Canvas implements Serializable { private float scaleSize; private float currentValue; //Default constructor public ProgressBar() { this(100, 50); } //Constructor public ProgressBar(float scaleSize, float currentValue) { super(); this.scaleSize = scaleSize; this.currentValue = currentValue; setBackground(Color.lightGray); setForeground(Color.magenta); //sets the initial size of the bean on a canvas setSize(100, 25); } public float getScaleSize() { return scaleSize; } public void setScaleSize(float sSize) { //The scale size can never set to a value lower than //the current value this.scaleSize = Math.max(0.0f, sSize); if (this.scaleSize < this.currentValue) { this.scaleSize = this.currentValue; } } public float getCurrentValue() { return currentValue; } public void setCurrentValue(float cVal) { //The current value can not be set negative //nor can it be set greater than the scale size this.currentValue = Math.max(0.0f, cVal); if (this.currentValue > this.scaleSize) { this.currentValue = this.scaleSize; } } //The paint method is called by the container public synchronized void paint(Graphics g) { int width = getSize().width; int height = getSize().height; g.setColor(getBackground()); g.fillRect(1, 1, width-2, height-2); g.draw3DRect(0,0, width-1, height-1, true); g.setColor(getForeground()); g.fillRect(3,3,(int)((currentValue * (width-6))/scaleSize), height-6); } //The grow method makes the current value larger public void grow() { setCurrentValue( this.currentValue + 1.0f); } //The shrink method makes the current value smaller public void shrink() { setCurrentValue( this.currentValue - 1.0f); } }//class The ProgressBar class extends Canvas, which is a simple rectangular area with a paint() method that can be overridden to create the appearance of the JavaBean. The Serializable interface is a marker with no methods to implement. It simply marks this class as one that can store and retrieve its state properly, in the opinion of the programmer. public class ProgressBar extends Canvas implements Serializable This Bean has only two unique class-level variables (properties) and about a dozen more that it inherits by extending the Canvas class. (foreground and background colors, height, width, and so on). The scaleSize property shows the value that will fill the progress bar to the max. The currentValue represents how much progress has been achieved thus far. If a gas tank holds 20 gallons, and you fill it half way, the scaleSize would be set to 20 and the currentValue would be set to 10. private float scaleSize; private float currentValue; The default constructor is the constructor that contains no parameters. In this instance, it calls another constructor via the this() method, and passes it the values of 100 and 50. These values are the scaleSize and currentValue parameters. Most GUI tools use this constructor when you first drag and drop a component onto a form. //Default constructor public ProgressBar() { this(100, 50); } The other constructor sets some unique property values, and then sets some of the inherited properties also. Note that the initial size of the Bean is set using the setSize() method. This size is the height and width of the Bean itself, not to be confused with the scaleSize and currentValue of the progress bar. setBackground(Color.lightGray); setForeground(Color.magenta); //sets the initial size of the bean on a canvas setSize(100, 25); } The paint() method is used to create the appearance of the Bean. A Canvas object is just a rectangle with a background color of gray displayed. The word synchronized means that this method is threadsafe. Thread safety is important when you are creating multiple instances of the same object at the same time because GUI screens often contain multiple instances of the same JavaBean. //The paint method is called by the container public synchronized void paint(Graphics g) { int width = getSize().width; int height = getSize().height; g.setColor(getBackground()); g.fillRect(1, 1, width-2, height-2); g.draw3DRect(0,0, width-1, height-1, true); g.setColor(getForeground()); g.fillRect(3,3,(int)((currentValue * (width-6))/scaleSize), height-6); } Two methods exist that other classes and components can use to cause the progress bar to grow gracefully or to shrink. The only increments and decrements are by 1 to make the growth smooth. public void grow() { setCurrentValue( this.currentValue + 1.0f); } JavaBean classes do explicitly inherit from the Canvas class. They also inherit implicitly from the Component class, however. This inherited functionality does not provide everything that GUI builder tools need to manage the component, however. They still need some toolbox icons so that the users can drag and drop the component into an application. The SimpleBeanInfo class provides these icons. In Listing 15.2, we extend the SimpleBeanInfo class and override the getIcon method as shown here: Listing 15.2 The ProgressBarBeanInfo.java File /* * ProgressBarBeanInfo.java * * Created on August 27, 2002, 11:54 AM */ package com.samspublishing.jpp.ch15; import java.beans.*; import java.awt.*; /** * * @author Stephen Potts * @version */ public class ProgressBarBeanInfo extends SimpleBeanInfo { public Image getIcon(int iconKind) { if(iconKind == BeanInfo.ICON_COLOR_16x16) { Image img = loadImage("ProgressBarIcon16.gif"); return img; } if(iconKind == BeanInfo.ICON_COLOR_32x32) { Image img = loadImage("ProgressBarIcon32.gif"); return img; } return null; } } Because JavaBeans are programmed to a careful specification, GUI builder tools can figure out which properties a Bean exposes to programs by looking at the structure of the class file. This is called introspection. Because of introspection, we don't need to write any special code to handle properties. The reason that we have two different sizes of icons is that some GUI tools prefer one size and some the other. Deploying JavaBeans The whole strategy of writing Bean classes is very logical and straightforward. You just package up the classes and icons into a jar file and place the jar file in a special directory with a special document called a manifest. We will work an example to show you how to do this. This example will be deployed using the Bean Development Kit (BDK) that is available from java.sun.com. The BDK is a testing environment that enables programmers to test their JavaBeans before deploying them to other environments such as Forte or JBuilder. Let's step through the deployment of the ProgressBar example on a Windows machine. The process for Unix is identical except for the directory names. Follow these steps: -
Create a directory called c:\com\samspublishing\jpp\ch15. -
Copy the following files from the Web site to this directory: ProgressBar.java ProgressBarIcon32.gif ProgressBarIcon16.gif ProgressBarBeanInfo.java
-
Compile the two .java files. -
Create a file called ProgressBar.mf that contains the following lines: Manifest-Version: 1.0 Created-By: 1.4.0 (Sun Microsystems Inc.) Name: com/samspublishing/jpp/ch15/ProgressBar.class Java-Bean: True -
cd up to c:\. -
Type the following command: jar cfm com\samspublishing\jpp\ch15\ProgressBar.jar [ic:ccc] com\samspublishing\jpp\ch15\ProgressBar.mf [ic:ccc] com\samspublishing\jpp\ch15\*.class com\samspublishing\jpp\ch15\*.gif -
Look in c:\com\samspublishing\jpp\ch15 and see that a file named ProgressBar.jar exists. Open it with a zip program and verify that it contains the files shown in Figure 15.1: Figure 15.1. The ProgressBar.jar file contains all the code needed to add this JavaBean to a GUI development tool. 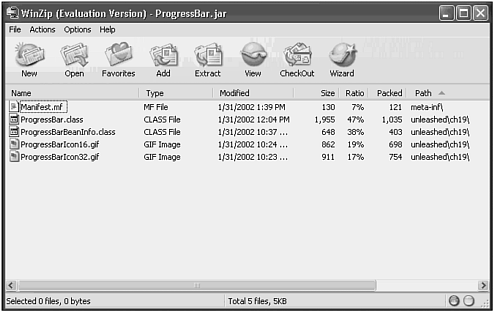 -
Download the Bean Development Kit, the BDK from the http://java.sun.com/ Web site. (Type BDK in the search box, and it will take you to the download page.) You will find instructions on how to install this product on the Web site. During installation, specify c:\BDK as the location in which to install. -
Copy the ProgressBar.jar file into the C:\BDK\jars directory. -
Move to the c:\BDK\beanbox directory, and type run . This will open four windows. The first window is the ToolBox window that is shown in Figure 15.2: Figure 15.2. The ToolBox contains all the Beans that have jar files in c:\BDK\jars. 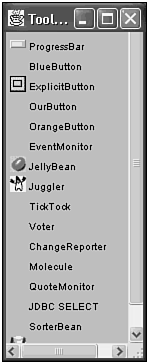 -
Notice that one of the Beans in the ToolBox is our very own ProgressBar. -
Click the ProgressBar icon in the ToolBox. Next, click the middle of the BeanBox window. An instance of the ProgressBar Bean appears in the window, as shown in Figure 15.3: Figure 15.3. The BeanBox application allows programmers to test their Beans before placing them into other, more complex, development tools. 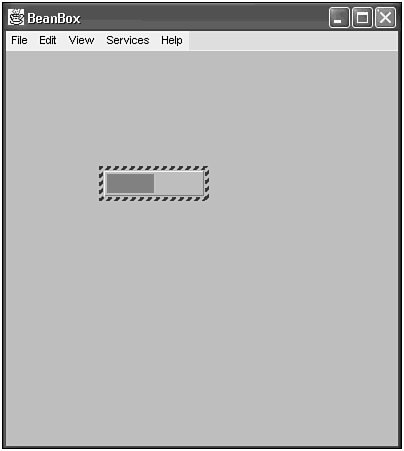 -
Next, examine the contents of the Properties window that is shown in Figure 15.4: Figure 15.4. The Properties window provides a user interface for changing the bean-specific set of properties. 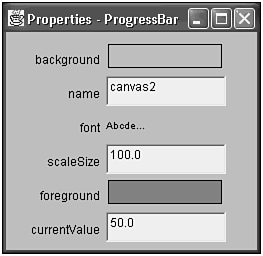 -
Notice that all the properties are listed, including the inherited ones. Click the colored rectangle and notice the dialog box that appears in Figure 15.5: Figure 15.5. The foreground property takes a Color object as its value. A built-in property dialog exists for changing the Color object. 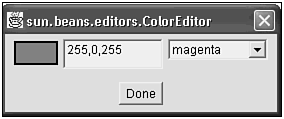 -
Observe that the color of the rectangle in the Properties dialog has changed along with the color of the ProgressBar that is being displayed in the BeanBox. This dialog appears automatically because the return type of the setForgroundColor() method is of class Color. Automatic property change dialogs exist for the intrinsic types, Strings, Fonts, and other common return types. If you return an object that you have defined, you will be responsible for implementing this. The Bean Development Kit is a good way to start the development process. Eventually you will need to learn how to deploy JavaBeans in commercial development tools. |