10.6. Sessions and Process Groups In Linux, as in other Unix systems, users normally interact with groups of related processes. Although they initially log in to a single terminal and use a single process (their shell, which provides a command-line interface), users end up running many processes as a result of actions such as Running noninteractive tasks in the background Switching among interactive tasks via job control, which is discussed more fully in Chapter 15 Starting multiple processes that work together through pipes Running a windowing system, such as the X Window System, which allows multiple terminal windows to be opened In order to manage all of these processes, the kernel needs to group the processes in ways more complicated than the simple parent-child relationship we have already discussed. These groupings are called sessions and process groups. Figure 10.1 shows the relationship among sessions, process groups, and processes. Figure 10.1. Sessions, Process Groups, and Processes 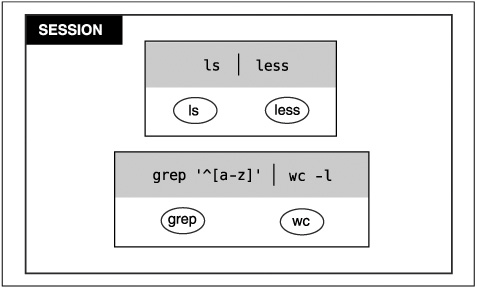 10.6.1. Sessions When a user logs out of a system, the kernel needs to terminate all the processes the user had running (otherwise, users would leave a slew of old processes sitting around waiting for input that can never arrive). To simplify this task, processes are organized into sets of sessions. The session's ID is the same as the pid of the process that created the session through the setsid() system call. That process is known as the session leader for that session group. All of that process's descendants are then members of that session unless they specifically remove themselves from it. The setsid() function does not take any arguments and returns the new session ID. #include <unistd.h> pid_t setsid(void); 10.6.2. Controlling Terminal Every session is tied to a terminal from which processes in the session get their input and to which they send their output. That terminal may be the machine's local console, a terminal connected over a serial line, or a pseudo terminal that maps to an X window or across a network (see Chapter 16 for information on pseudo terminal devices). The terminal to which a session is related is called the controlling terminal (or controlling tty) of the session. A terminal can be the controlling terminal for only one session at a time. Although the controlling terminal for a session can be changed, this is usually done only by processes that manage a user's initial logging in to a system. Information on how to change a session's controlling tty appears in Chapter 16, on pages 338-339. 10.6.3. Process Groups One of the original design goals of Unix was to construct a set of simple tools that could be used together in complex ways (through mechanisms like pipes). Most Linux users have done something like the following, which is a practical example of this philosophy: ls | grep "^[aA].*\.gz" | more Another popular feature added to Unix fairly early was job control. Job control allows users to suspend the current task (known as the foreground task) while they go and do something else on their terminals. When the suspended task is a sequence of processes working together, the system needs to keep track of which processes should be suspended when the user wants to suspend "the" foreground task. Process groups allow the system to keep track of which processes are working together and hence should be managed together via job control. Processes are added to a process group through setpgid(). int setpgid(pid_t pid, pid_t pgid); pid is the process that is being placed in a new process group (0 may be used to indicate the current process). pgid is the process group ID the process pid should belong to, or 0 if the process should be in a new process group whose pgid is the same as that process's pid. Like sessions, a process group leader is the process whose pid is the same as its process group ID (or pgid). The rules for how setpgid() may be used are a bit complicated. A process may set the process group of itself or one of its children. It may not change the process group for any other process on the system, even if the process calling setpgid() has root privileges. A session leader may not change its process group. A process may not be moved into a process group whose leader is in a different session from itself. In other words, all the processes in a process group must belong to the same session. setpgid() call places the calling process into its own process group and its own session. This is necessary to ensure that two sessions do not contain processes in the same process group. A full example of process groups is given when we discuss job control in Chapter 15. When the connection to a terminal is lost, the kernel sends a signal (SIGHUP; see Chapter 12 for more information on signals) to the leader of the session containing the terminal's foreground process group, which is usually a shell. This allows the shell to terminate the user's processes unconditionally, notify the processes that the user has logged out (usually, through a SIGHUP), or take some other action (or inaction). Although this setup may seem complicated, it lets the session group leader decide how closed terminals should be handled rather than putting that decision in the kernel. This allows system administrators flexibile control over account policies. Determining the process group is easily done through the getpgid() and getpgrp() functions. pid_t getpgid(pid_t pid) Returns the pgid of process pid. If pid is 0, the pgid of the current process is returned. No special permissions are needed to use this call; any process may determine the process group to which any other process belongs. pid_t getpgrp(void) Returns the pgid of the current process (equivalent to getpgid(0)). 10.6.4. Orphaned Process Groups The mechanism for how processes are terminated (or allowed to continue) when their session disappears is quite complicated. Imagine a session with multiple process groups in it (Figure 10.1 may help you visualize this). The session is being run on a terminal, and a normal system shell is the session leader. When the session leader (the shell) exits, the process groups are left in a difficult situation. If they are actively running, they can no longer use stdin or stdout as the terminal has been closed. If they have been suspended, they will probably never run again as the user of that terminal cannot easily restart them, but never running means they will not terminate either. In this situation, each process group is called an orphaned process group. POSIX defines this as a process group whose parent is also a member of that process group, or is not a member of that group's session. This is another way of saying that a process group is not orphaned as long as a process in that group has a parent in the same session but a different group. While both definitions are complicated, the concept is pretty simple. If a process group is suspended and there is not a process around that can tell it to continue, the process group is orphaned.[18] [18] Chapter 15 makes all of this much clearer, and it may be worth rereading this section after reading that chapter. When the shell exits, any of its child programs become children of init, but stay in their original session. Assuming that every program in the session is a descendant of the shell, all of the process groups in that session become orphaned. [19] When a process group is orphaned, every process in that process group is sent a SIGHUP, which normally terminates the program. Programs that have chosen not to terminate on SIGHUP are sent a SIGCONT, which resumes any suspended processes. This sequence terminates most processes and makes sure that any processes that are left are able to run (are not suspended).[20] [19] But maybe not right away. There may be process groups that contain processes who have parents in other process groups in that session. As the parent-child relationship between processes is a tree, there will eventually be a process group that contained only processes whose parents were the shell, and when that process group exits another one becomes orphaned.
[20] This discussion will make much more sense after you read the chapters on signals (Chapter 12) and job control (Chapter 15). Once a process has been orphaned, it is forcibly disassociated from its controlling terminal (to allow a new user to make use of that terminal). If programs that continue running try to access that terminal, those attempts result in errors, with errno set to EIO. The processes remain in the same session, and the session ID is not used for a new process ID until every program in that session has exited. |