In addition to the formatting tags provided by JSTL, a second set of tags is available for parsing. In JSTL, parsing refers to tags that are used to access data entered by a user. As a result, it is quite possible for errors to occur if the data that is to be parsed is not in the format that the JSTL tag expects. In this section, we show you how to use the JSTL parsing tags. Using the <fmt:parseNumber> Tag The <fmt:parseNumber> tag is used to parse numbers, percentages, and currencies. There are two forms of this tag: // Syntax 1: Without a body <fmt:parseNumber value="numericValue" [type="{number|currency|percent}"] [pattern="customPattern"] [parseLocale="parseLocale"] [integerOnly="{true|false}"] [var="varName"] [scope="{page|request|session|application}"]/> // Syntax 2: With a body to specify the numeric value to be parsed <fmt:parseNumber [type="{number|currency|percent}"] [pattern="customPattern"] [parseLocale="parseLocale"] [integerOnly="{true|false}"] [var="varName"] [scope="{page|request|session|application}"]> numeric value to be parsed </fmt:parseNumber> The attributes accepted by the <fmt:parseNumber> tag include the following: integerOnly | N | Specifies whether just the integer portion of the given value should be parsed. This attribute defaults to true. | parseLocale | N | Specifies the locale whose default formatting pattern (for numbers, currencies, or percentages) is to be used during the parse operation, or to which the pattern specified via the pattern attribute (if present) is applied. | pattern | N | The custom formatting pattern that determines how the string in the value attribute is to be parsed. Applied only when parsing numbers (that is, if type is missing or is equal to number); ignored otherwise. | scope | N | The scope of var. This attribute defaults to page. | type | N | Specifies whether the string in the value attribute should be parsed as a number, currency, or percentage. This attribute defaults to number. | value | N | The string to be parsed. | var | N | The name of the exported scoped attribute that stores the parsed result (of type java.lang.Number). | In the first syntax, the value that is to be parsed is passed in using the value attribute. Once parsed, the number will be written to the page if a var attribute is not specified. If a var attribute is specified, it will receive the parsed number. The second syntax uses the body of the tag to specify the number that is to be parsed. You should not provide a value attribute if you are using the tag with a body (syntax 2 in our example). In addition to these standard JSTL attributes, several parse attributes are available specific to number formatting. A type attribute is provided to specify what type of number is to be parsed. Valid values for the type attribute are number, currency, and percent. The default is number. A pattern attribute is provided that works just like the pattern attribute for the <fmt:formatNumber> tag. However, in the case of parsing, the pattern attribute tells the parser what format to expect. Table 6.1 (earlier in the chapter) contains a complete list of pattern codes. The integerOnly attribute allows you to request that decimal places should not be parsed. Decimal places will not cause an error; they will simply be truncated. The program in Listing 6.4 shows some examples of formatting for different types. Listing 6.4 Parsing Numbers, Currency, and Percents (parseNumber.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <%@ taglib uri="http://java.sun.com/jstl/fmt" prefix="fmt" %> <html> <head> <title>Parse Number</title> </head> <body> <form method="POST"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="62%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4">Number Formatting</font> </b> </p> </td> </tr> <tr> <td width="47%">Enter a number to be parsed:</td> <td width="53%"> <input type="text" name="num" size="20" /> </td> </tr> <tr> <td width="100%" colspan="2"> <p align="center"> <input type="submit" value="Submit" name="submit" /> <input type="reset" value="Reset" name="reset" /> </p> </td> </tr> </table> <p> </p> </form> <c:if test="${pageContext.request.method=='POST'}"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="63%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4">Formatting: <c:out value="${param.num}" /> </font> </b> </p> </td> </tr> <tr> <td width="51%">type="number"</td> <td width="49%"> <c:catch var="e"> <fmt:parseNumber var="i" type="number" value="${param.num}" /> <c:out value="${i}" /> </c:catch> <c:out value="${e}" escapeXml="false" /> </td> </tr> <tr> <td width="51%">type="currency"</td> <td width="49%"> <c:catch var="e"> <fmt:parseNumber var="i" type="currency" value="${param.num}" /> <c:out value="${i}" /> </c:catch> <c:out value="${e}" escapeXml="false" /> </td> </tr> <tr> <td width="51%">type="percent"</td> <td width="49%"> <c:catch var="e"> <fmt:parseNumber var="i" type="percent" value="${param.num}" /> <c:out value="${i}" /> </c:catch> <c:out value="${e}" escapeXml="false" /> </td> </tr> <tr> <td width="51%">type="number" integerOnly="true"</td> <td width="49%"> <c:catch var="e"> <fmt:parseNumber var="i" integerOnly="true" type="number" value="${param.num}" /> <c:out value="${i}" /> </c:catch> <c:out value="${e}" escapeXml="false" /> </td> </tr> </table> </c:if> </body> </html> The program in Listing 6.4 works by asking the user to enter a number. This number will be then parsed in several ways. Because it is possible that the user might enter an invalid number, each call to the <fmt:parseNumber> tag is bounded in <c:catch> tags. The following code shows how this is done: <c:catch var="e"> <fmt:parseNumber var="i" integerOnly="true" type="number" value="${param.num}" /> <c:out value="${i}" /> </c:catch> This code attempts to pass the scoped variable i as a number. If the scoped variable i cannot be parsed as a variable, an exception is thrown. This exception, if thrown, will be placed in the e scoped variable. If any exception is thrown, the following line of code prints it out: <c:out value="${e}" escapeXml="false" /> Our program also attempts to parse as a percent and currency. Usually, several of the parses in this program will throw an exception, since it is impossible for one number to satisfy all types. The output from this program, on a typical number, is shown in Figure 6.4. 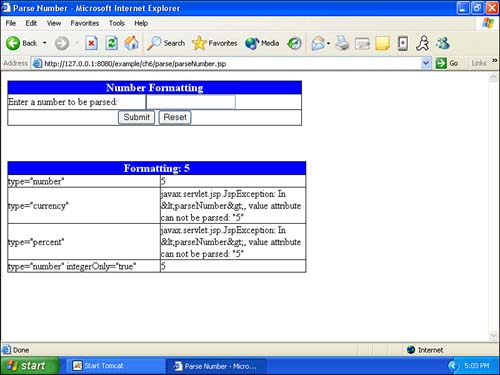 Using the <fmt:parseDate> Tag There are many occasions when a program must display dates. There are also many formats that a date can be displayed in. By using the <fmt:parseDate> tag, you can express your program's dates in a variety of ways. The two forms of the <fmt:parseDate> tag are as follows: // Syntax 1: Without a body <fmt:parseDate value="dateString" [type="{time|date|both}"] [dateStyle="{default|short|medium|long|full}"] [timeStyle="{default|short|medium|long|full}"] [pattern="customPattern"] [timeZone="timeZone"] [parseLocale="parseLocale"] [var="varName"] [scope="{page|request|session|application}"]/> // Syntax 2: With a body to specify the date value to be parsed <fmt:parseDate [type="{time|date|both}"] [dateStyle="{default|short|medium|long|full}"] [timeStyle="{default|short|medium|long|full}"] [pattern="customPattern"] [timeZone="timeZone"] [parseLocale="parseLocale"] [var="varName"] [scope="{page|request|session|application}"]> date value to be parsed </fmt:parseDate> The attributes that are accepted by the <fmt:parseDate> tag include the following: dateStyle | N | The style for the date. Should be default, short, long, medium, or full. The default is default. This attribute uses DateFormat semantics. | parseLocale | N | Specifies the locale that should be used. This attribute should be of type String or java.util.Locale. | pattern | N | Specifies how the date is to be parsed using a pattern. | timeStyle | N | The style for the time. Should be default, short, long, medium, or full. The default is default. | timeZone | N | The zone that the parsed time is from. This value should be of type String or java.util.TimeZone. | type | N | The type of date to parse. Should be time, date, or both. The default is date. | value | N | The string to be parsed. | var | N | The name of the exported scoped attribute that stores the parsed result (of type java.lang.Number). | In the first syntax, the value that is to be parsed is passed using the value attribute. The parsed date will be written to the page if a var attribute is not specified. If a var attribute is specified, it will receive the parsed date. The second syntax uses the body of the tag to specify the date that is to be parsed. You should not provide a value attribute if you are using the tag with a body (syntax 2 in our example). In addition to these standard JSTL attributes, several formatting attributes are available specific to date parsing. The type attribute allows you to specify whether a time, date, or both will be parsed. The valid values for the type attribute are time, date, and both. A pattern attribute is provided that works just like the pattern attribute for the <fmt:formatDate> tag. However, in the case of parsing, the pattern attribute tells the parser what format to expect. A complete list of pattern codes can be found in Table 6.2. A timeZone attribute is available to specify the time zone; we cover time zones in the next section. The dateStyle and timeStyle attributes are specified, just as with the <fmt:formatDate> tag. These tags indicate which format the date will be in. Refer to Figure 6.3 to see the various formats available. Listing 6.5 shows a program that demonstrates parsing dates and times. Listing 6.5 Parsing Dates (parseDate.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <%@ taglib uri="http://java.sun.com/jstl/fmt" prefix="fmt" %> <html> <head> <title>Parse Date</title> </head> <body> <form method="POST"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="62%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4">Date Formatting</font> </b> </p> </td> </tr> <tr> <td width="47%">Enter a date to be parsed:</td> <td width="53%"> <input type="text" name="num" size="20" /> </td> </tr> <tr> <td width="100%" colspan="2"> <p align="center"> <input type="submit" value="Submit" name="submit" /> <input type="reset" value="Reset" name="reset" /> </p> </td> </tr> </table> <p> </p> </form> <c:if test="${pageContext.request.method=='POST'}"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="63%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4">Formatting: <c:out value="${param.num}" /> </font> </b> </p> </td> </tr> <tr> <td width="51%">type="date" dateStyle="short"</td> <td width="49%"> <c:catch var="e"> <fmt:parseDate var="i" type="date" dateStyle="short" value="${param.num}" /> <c:out value="${i}" /> </c:catch> <c:out value="${e}" escapeXml="false" /> </td> </tr> <tr> <td width="51%">type="date" dateStyle="medium"</td> <td width="49%"> <c:catch var="e"> <fmt:parseDate var="i" type="date" dateStyle="medium" value="${param.num}" /> <c:out value="${i}" /> </c:catch> <c:out value="${e}" escapeXml="false" /> </td> </tr> <tr> <td width="51%">type="date" dateStyle="long"</td> <td width="49%"> <c:catch var="e"> <fmt:parseDate var="i" type="date" dateStyle="long" value="${param.num}" /> <c:out value="${i}" /> </c:catch> <c:out value="${e}" escapeXml="false" /> </td> </tr> <tr> <td width="51%">type="date" dateStyle="full"</td> <td width="49%"> <c:catch var="e"> <fmt:parseDate var="i" type="date" dateStyle="full" value="${param.num}" /> <c:out value="${i}" /> </c:catch> <c:out value="${e}" escapeXml="false" /> </td> </tr> </table> </c:if> </body> </html> The program in Listing 6.5 works by asking the user to enter a date. This date will be then parsed in several ways. Because it is possible that the user might enter an invalid date, each call to the <fmt:parseDate> tag is bounded in <c:catch> tags. The following code shows how this is done: <c:catch var="e"> <fmt:parseDate var="i" type="date" dateStyle="full" value="${param.num}" /> <c:out value="${i}" /> </c:catch> This code attempts to pass the scoped variable i as a date. If the scoped variable i cannot be parsed as a date, then an exception is thrown. This exception, if thrown, will be placed in the e scoped variable. The following line of code prints it out: <c:out value="${e}" escapeXml="false" /> This program also attempts to parse several formats. Usually, several of the parses in the program will throw an exception, since it is impossible for one date to satisfy all types. The output from this program, on a typical number, is shown in Figure 6.5. 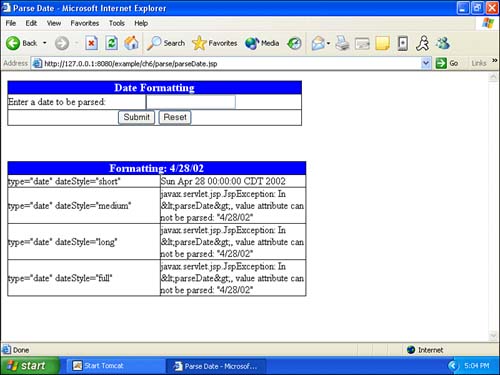 |